Promise基本概念
-
Promise是一个构造函数
-
我们可以创建Promise的实例
const p = new Promise()
-
new 出来的Promise实例对象,代表一个异步操作
-
Promise.prototype上包含一个
.then() 方法
-
每一次new Promise()构造函数得到的实例对象,
-
都可以
通过原型链的方式 访问到.then()方法,例如p.then()
-
.then()方法用来预先指定成功和失败的回调函数
-
p.then(
成功的回调函数 ,
失败的回调函数 )
p.then(
result => { },
error => {})
调用.then()方法时,成功的回调函数是必选的、失败的回调函数是可选的
then-fs基本使用测试Promise
基本使用
import thenFs from 'then-fs'
thenFs.readFile('./files/1.txt', 'utf8').then(r1 =>{console.log(r1)})
thenFs.readFile('./files/2.txt', 'utf8').then(r2 =>{console.log(r2)})
thenFs.readFile('./files/3.txt', 'utf8').then(r3 =>{console.log(r3)})
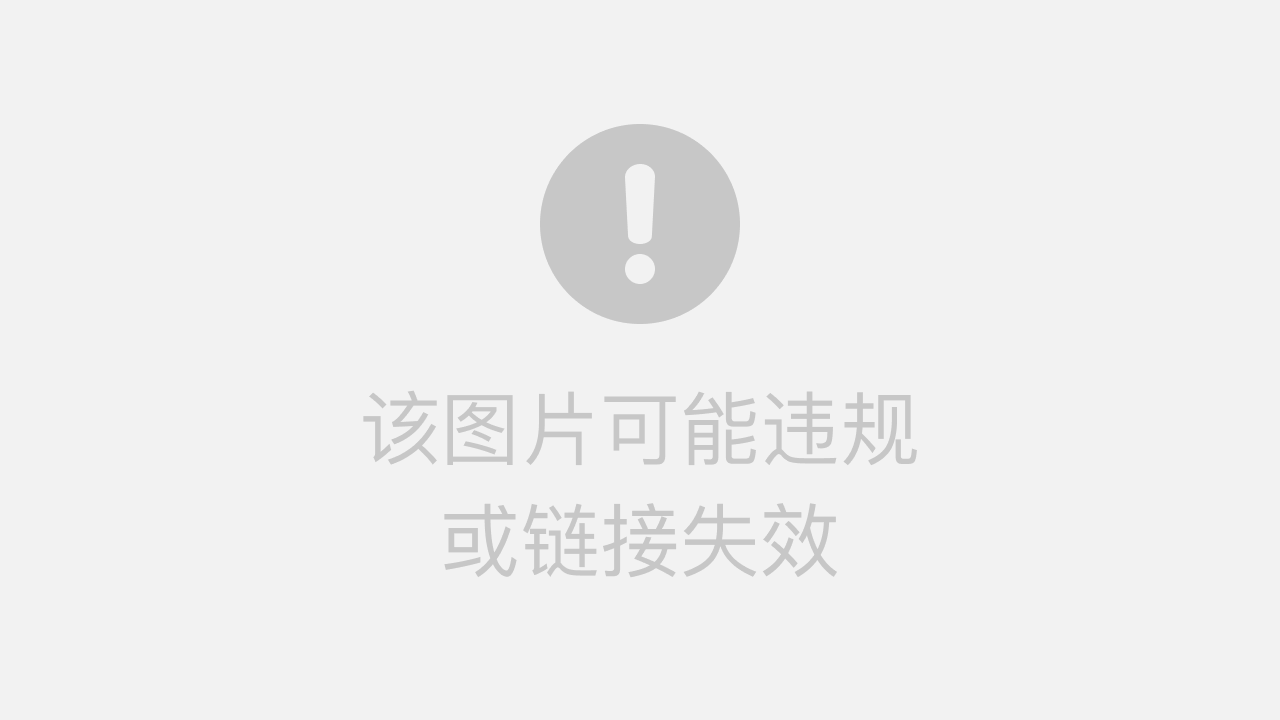
基于Promise的链式顺序调用
import thenFs from 'then-fs'
thenFs.readFile('./files/1.txt', 'utf8')
.then(r1 =>{
console.log(r1)
return thenFs.readFile('./files/2.txt', 'utf8')
})
.then(r2 => {
console.log(r2)
return thenFs.readFile('./files/3.txt', 'utf8')
})
.then(r3 =>{
console.log(r3)
})
通过.catch捕获错误
- 可以通过catch捕捉错误
- 不希望错误影响程序的后续执行,则可以
将catch往前提
import thenFs from 'then-fs'
thenFs.readFile('./files/111.txt', 'utf8')
.catch(err =>{
console.log(err)
})
.then(r1 =>{
console.log(r1)
return thenFs.readFile('./files/2.txt', 'utf8')
})
.then(r2 => {
console.log(r2)
return thenFs.readFile('./files/3.txt', 'utf8')
})
.then(r3 =>{
console.log(r3)
})
.catch(err =>{
console.log(err)
})
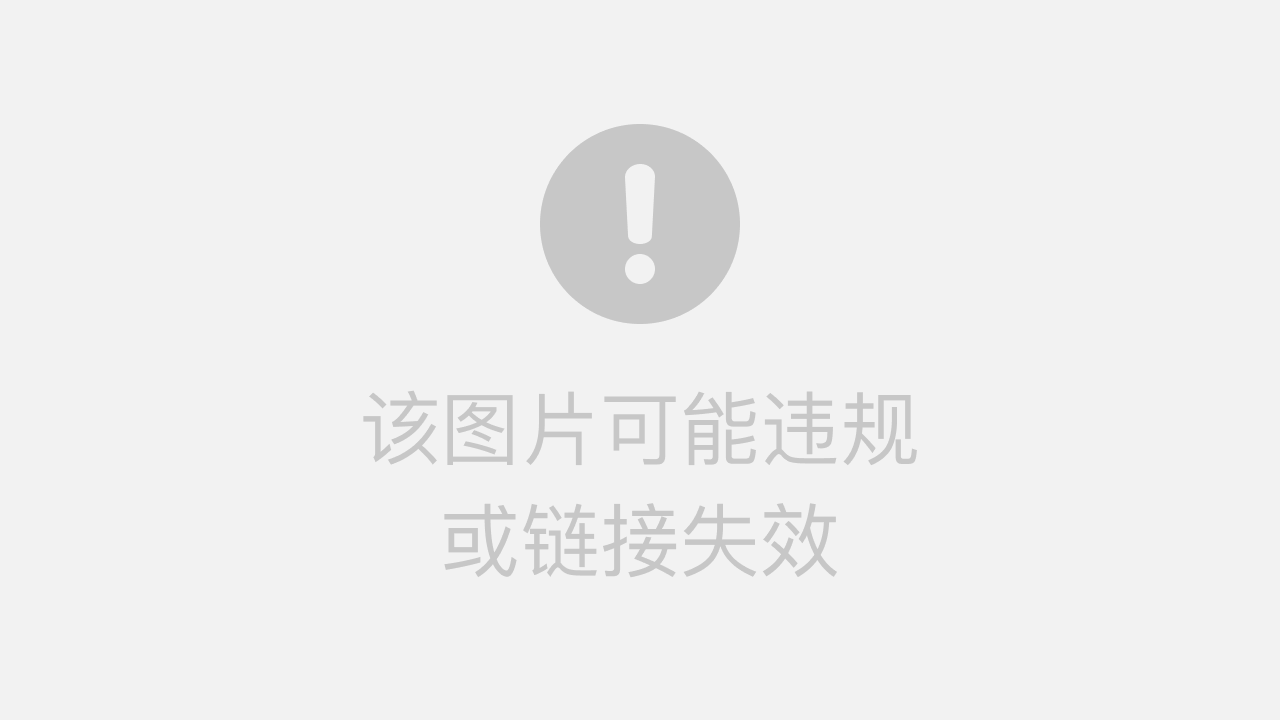
Promise.all()方法
- 会发起并行的Promise异步操作,等
所有异步操作全部结束后 才会执行下一步的.then操作(等待机制)
import thenFs from 'then-fs'
const promiseArr = [
thenFs.readFile('./files/2.txt', 'utf8'),
thenFs.readFile('./files/1.txt', 'utf8'),
thenFs.readFile('./files/3.txt', 'utf8')
]
Promise.all(promiseArr).then(res =>{
console.log(res)
})
.catch(err =>{
console.log(err)
})
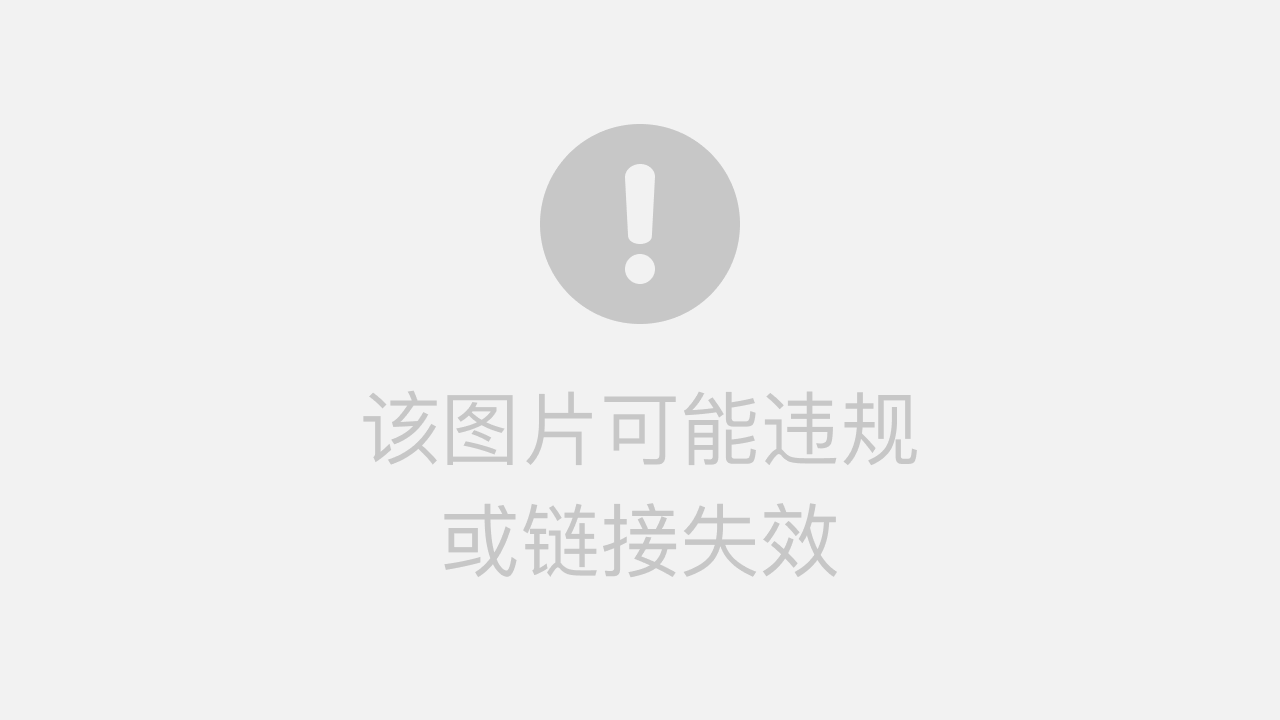
Promise.race()方法
- 也会发起并行的Promise操作,
任何一个异步操作完成,就立即执行下一步.then 赛跑机制
import thenFs from 'then-fs'
const promiseArr = [
thenFs.readFile('./files/2.txt', 'utf8'),
thenFs.readFile('./files/1.txt', 'utf8'),
thenFs.readFile('./files/3.txt', 'utf8')
]
Promise.race(promiseArr).then(res =>{
console.log(res)
})
.catch(err =>{
console.log(err)
})
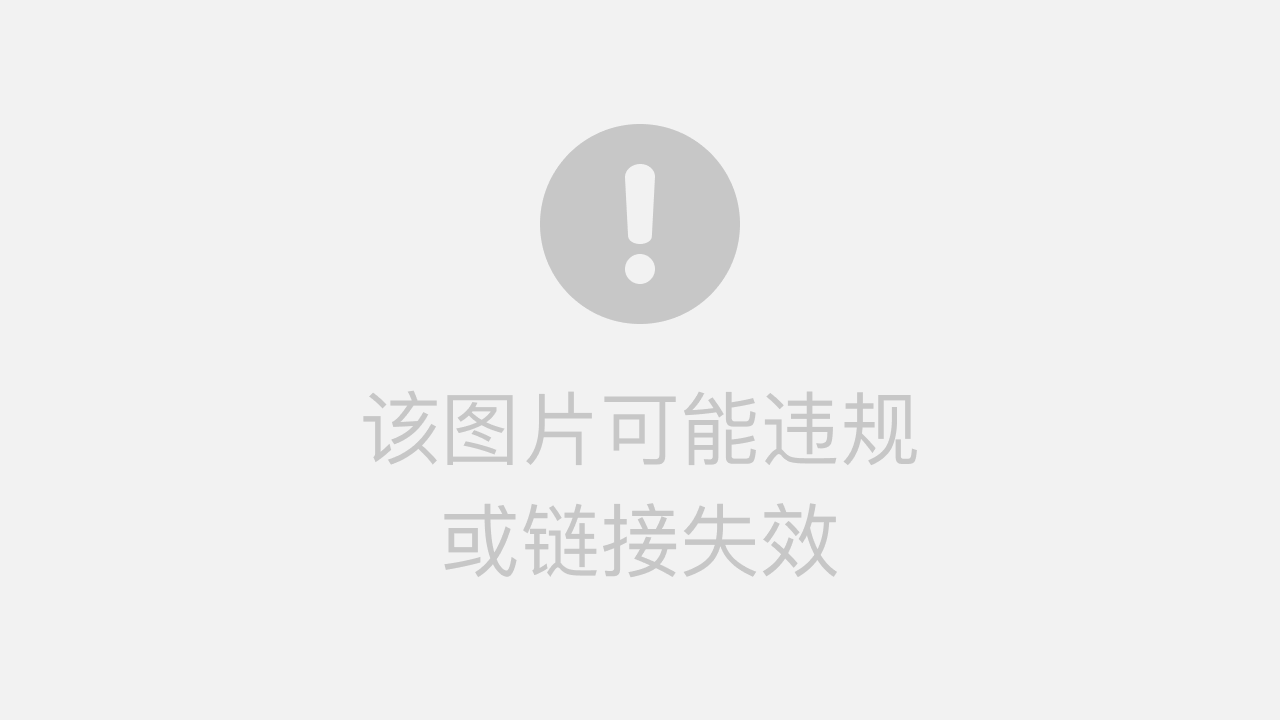
基于Promise封装读文件的方法
import thenFs from 'then-fs'
function getFile (fpath){
return new Promise(function (resolve, reject){
thenFs.readFile(fpath, 'utf8', (res, err) => {
if(err) return reject(err)
return resolve(res)
})
})
}
getFile('./files/1.txt').then(r1 => {
console.log(r1)
}, err =>{
console.log(err)
})
getFile('./files/11.txt').then(r1 => {
console.log(r1)
}).catch(err => {
console.log(err)
})

async和await基本使用
一个方法内使用了await则方法必须被async修饰 - 不使用async和await修饰则拿到的时实例对象
- 使用async和await直接拿到对应的结果
import thenFs from 'then-fs'
function getAllFile (){
const r1 = thenFs.readFile('./files/1.txt', 'utf8')
console.log(r1)
}
getAllFile()
async function getAllFile1 (){
const r1 = await thenFs.readFile('./files/1.txt', 'utf8')
console.log(r1)
const r2 = await thenFs.readFile('./files/2.txt', 'utf8')
console.log(r2)
const r3 = await thenFs.readFile('./files/3.txt', 'utf8')
console.log(r3)
}
getAllFile1()
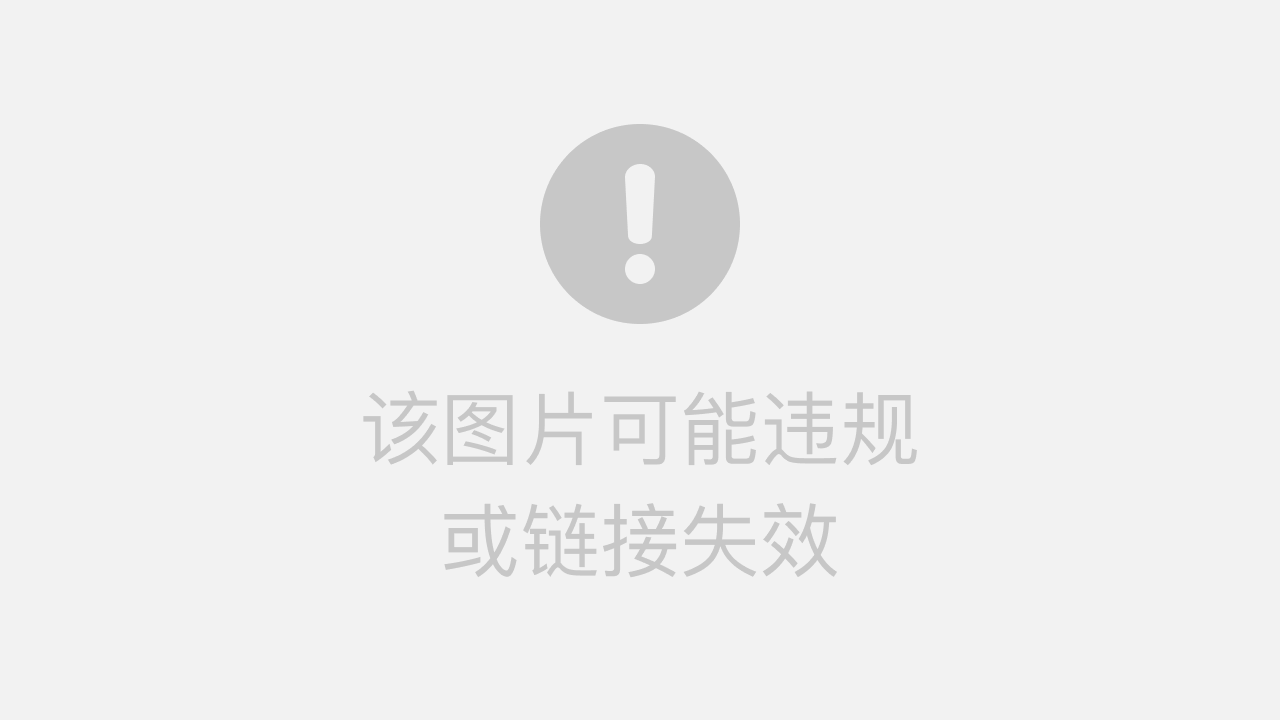
async和await注意事项
- async方法中,
第一个await之前的代码会同步执行 ,await之后的代码会异步执行
console.log('A')
async function getAllFile1 (){
console.log('B')
const r1 = await thenFs.readFile('./files/1.txt', 'utf8')
console.log(r1)
const r2 = await thenFs.readFile('./files/2.txt', 'utf8')
console.log(r2)
const r3 = await thenFs.readFile('./files/3.txt', 'utf8')
console.log(r3)
setTimeout(function (){
console.log('E')
}, 1000)
console.log('D')
}
getAllFile1()
console.log('C')
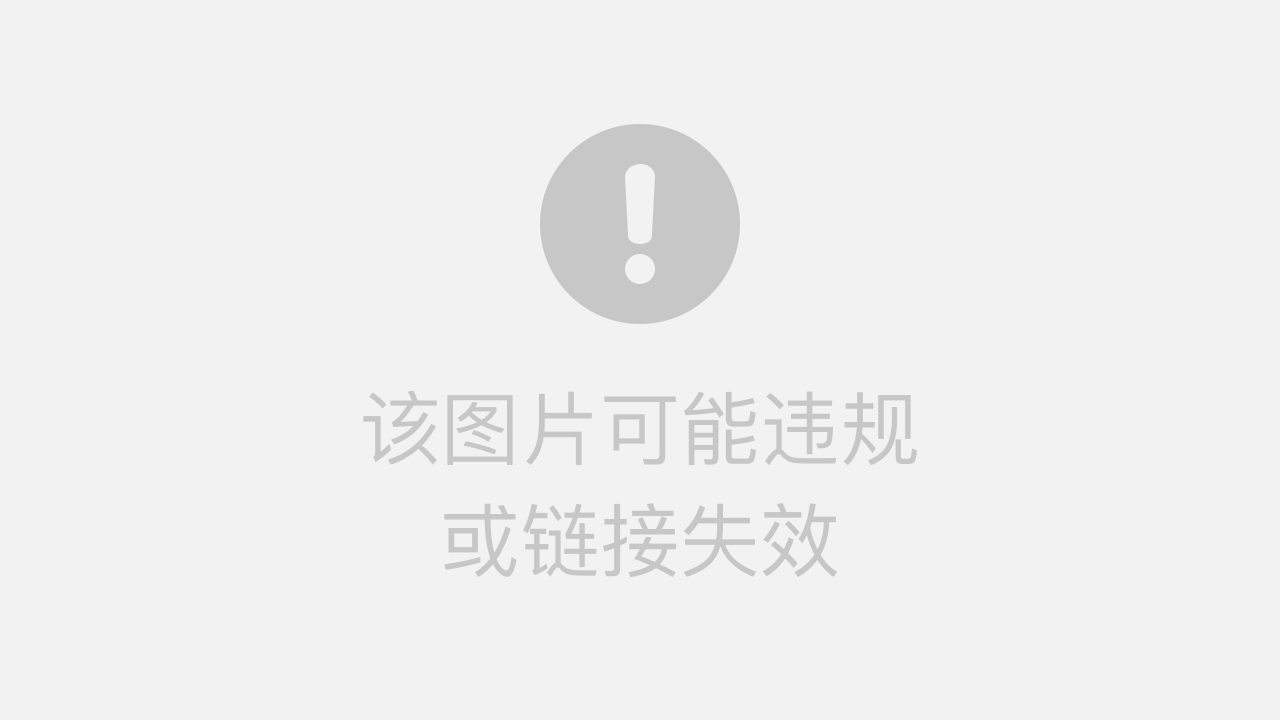
|