最近遇到一个需求:要把swiper的pagination分页器调整到右下角,同时修改分页器颜色。 被这个很简单的需求困了好久,所以本文就讲一下如何实现这个需求,给后面遇到同样问题的朋友排排坑。
运行环境
"next": "12.1.6",
"react": "18.2.0",
"react-dom": "18.2.0",
"swiper": "^8.2.4"
Swiper8基本使用
总所周知,Next.js是基于React的SSR框架。 在官网可以看到Swiper团队针对React专门封装了对应的组件。 英文官方文档:https://swiperjs.com/react (建议直接看英文文档,中文文档更新不及时,甚至现在还没有出Swiper8的)
- 安装
yarn add swiper 目前我安装的最新版本为"swiper": “^8.2.4” - 基本使用
封装轮播图组件
import { Pagination } from "swiper";
import { Swiper, SwiperSlide } from "swiper/react";
import "swiper/css";
import "swiper/css/pagination";
const Carousel = () => {
return (
<Swiper
modules={[Pagination]}
spaceBetween={0}
slidesPerView={1}
pagination={{ clickable: true }}
onSlideChange={() => console.log("slide change")}
onSwiper={(swiper) => console.log(swiper)}
>
<SwiperSlide style={{ background: "lightblue", height: "200px" }}>
Slide 1
</SwiperSlide>
<SwiperSlide style={{ background: "lightblue", height: "200px" }}>
Slide 2
</SwiperSlide>
<SwiperSlide style={{ background: "lightblue", height: "200px" }}>
Slide 3
</SwiperSlide>
<SwiperSlide style={{ background: "lightblue", height: "200px" }}>
Slide 4
</SwiperSlide>
</Swiper>
);
};
export default Carousel;
在页面中引入组件
import Carousel from "../components/Carousel";
const Home = () => {
return (
<>
<Carousel></Carousel>
</>
);
};
export default Home;
- 基本效果展示
输入yarn dev 启动项目后在http://localhost:3000 访问到如下页面 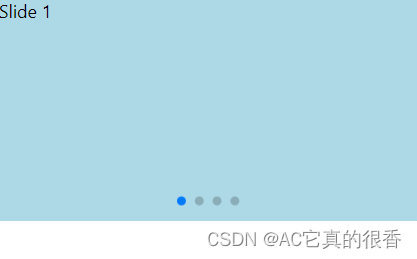
修改样式
因为Next.js不支持直接导入你自己写的外部样式(只有在globals.css中才能导入),而需要使用module模块化的样式才能导入。模块化的样式隔离了作用域,导致我编写的样式无法穿透到swiper组件。
如果在globals.css中直接导入样式是可以修改的,但会在不需要此样式的页面中会出现不必要的加载。
最后在官方文档中发现可以在js中通过styled-jsx直接编写css:CSS-in-JS 使用global 关键字可设置样式的作用域
全局样式
export default () => (
<div>
<style jsx global>{`
body {
background: red;
}
`}</style>
</div>
)
某一个样式全局化
使用:global() 包裹选择器
import Select from 'react-select'
export default () => (
<div>
<Select optionClassName="react-select" />
<style jsx>{`
/* "div" will be prefixed, but ".react-select" won't */
div :global(.react-select) {
color: red;
}
`}</style>
</div>
)
修改分页器样式
import { Pagination } from "swiper";
import { Swiper, SwiperSlide } from "swiper/react";
import "swiper/css";
import "swiper/css/pagination";
const Carousel = () => {
return (
<>
<Swiper
modules={[Pagination]}
spaceBetween={0}
slidesPerView={1}
pagination={{ clickable: true }}
onSlideChange={() => console.log("slide change")}
onSwiper={(swiper) => console.log(swiper)}
>
<SwiperSlide style={{ background: "lightblue", height: "200px" }}>
Slide 1
</SwiperSlide>
<SwiperSlide style={{ background: "lightblue", height: "200px" }}>
Slide 2
</SwiperSlide>
<SwiperSlide style={{ background: "lightblue", height: "200px" }}>
Slide 3
</SwiperSlide>
<SwiperSlide style={{ background: "lightblue", height: "200px" }}>
Slide 4
</SwiperSlide>
</Swiper>
<style jsx global>
{`
.swiper-pagination {
text-align: right;
padding-right: 10px;
}
.swiper-pagination-bullet {
border-radius: 0;
}
.swiper-pagination-bullet-active {
background: green;
}
`}
</style>
</>
);
};
export default Carousel;
修改后的样式如下: 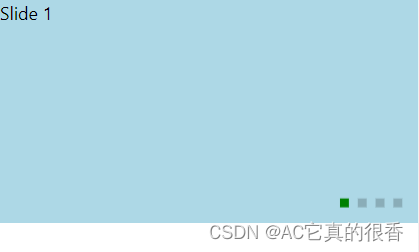
|