项目仓库: https://github.com/chenfenbgin/vue3code vue3 官网学习地址:https://staging-cn.vuejs.org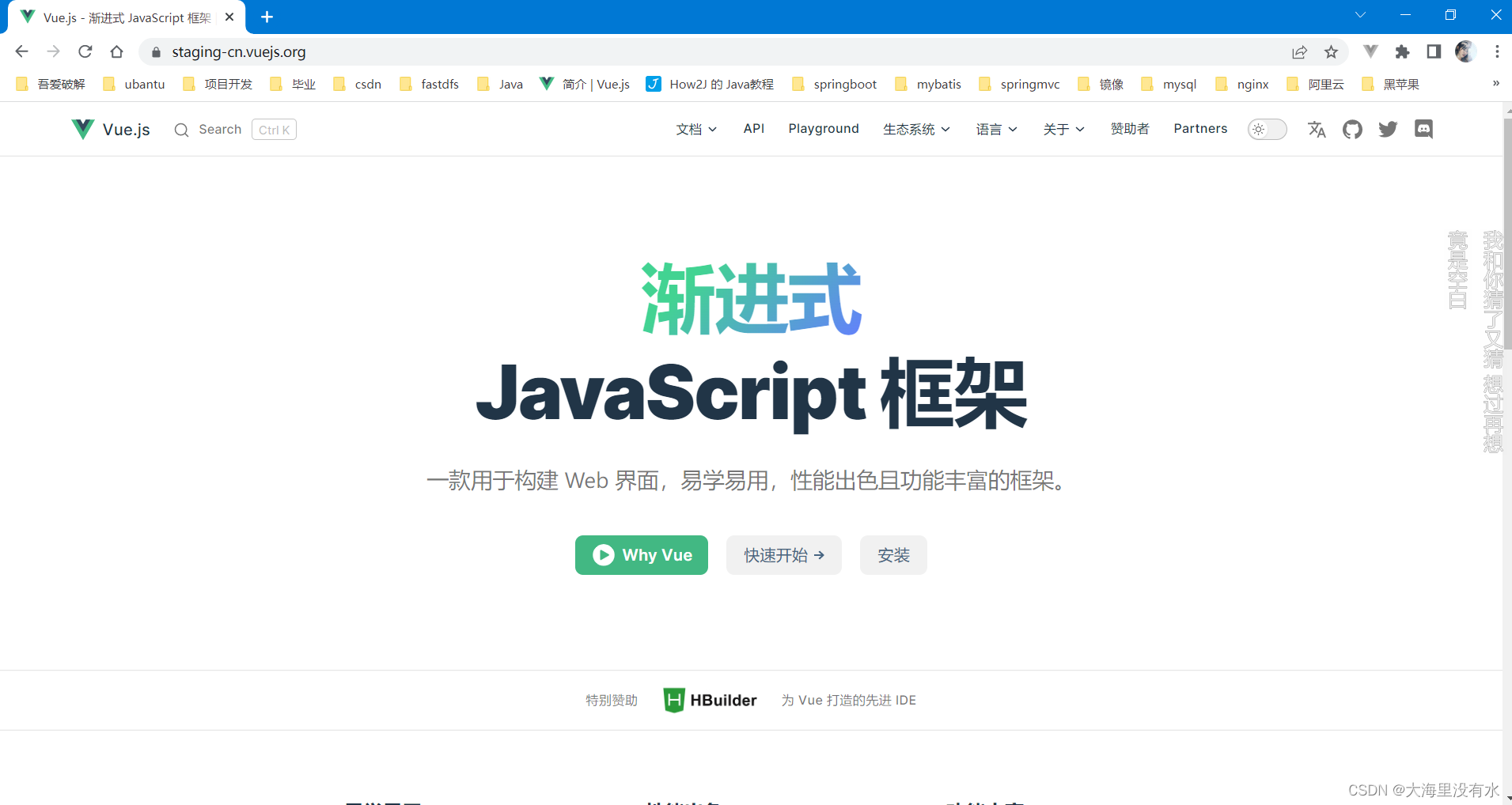
一、vue3基础
1、vue3改变
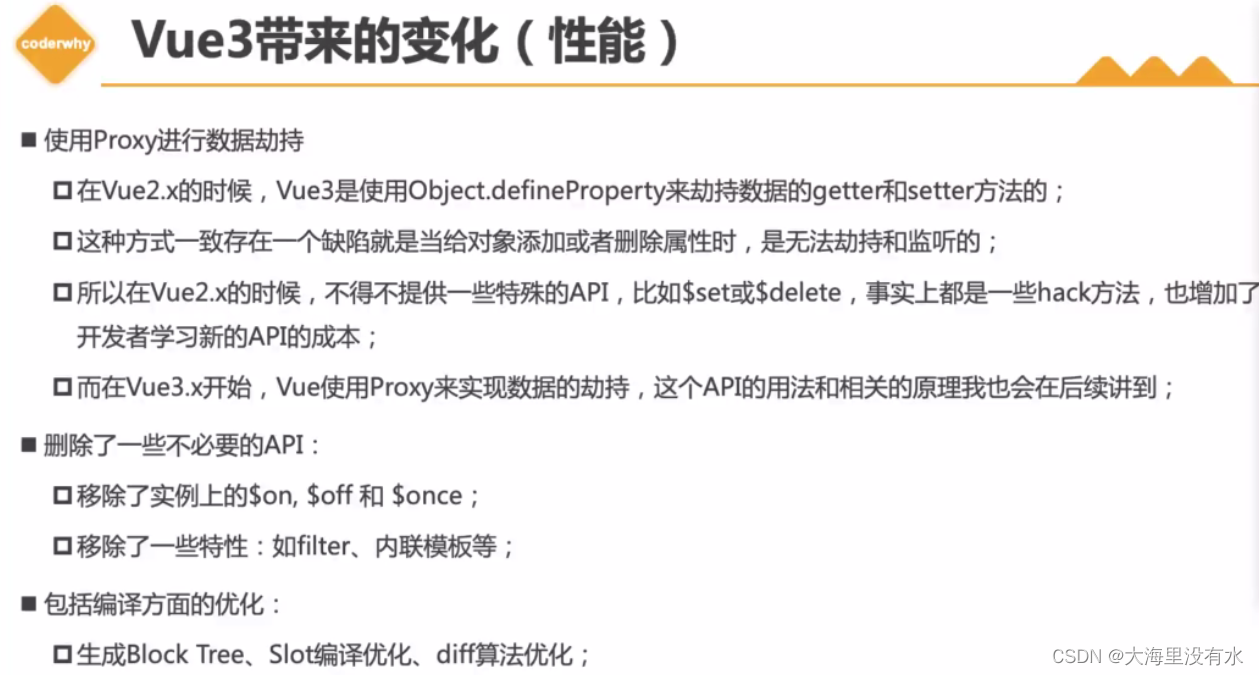 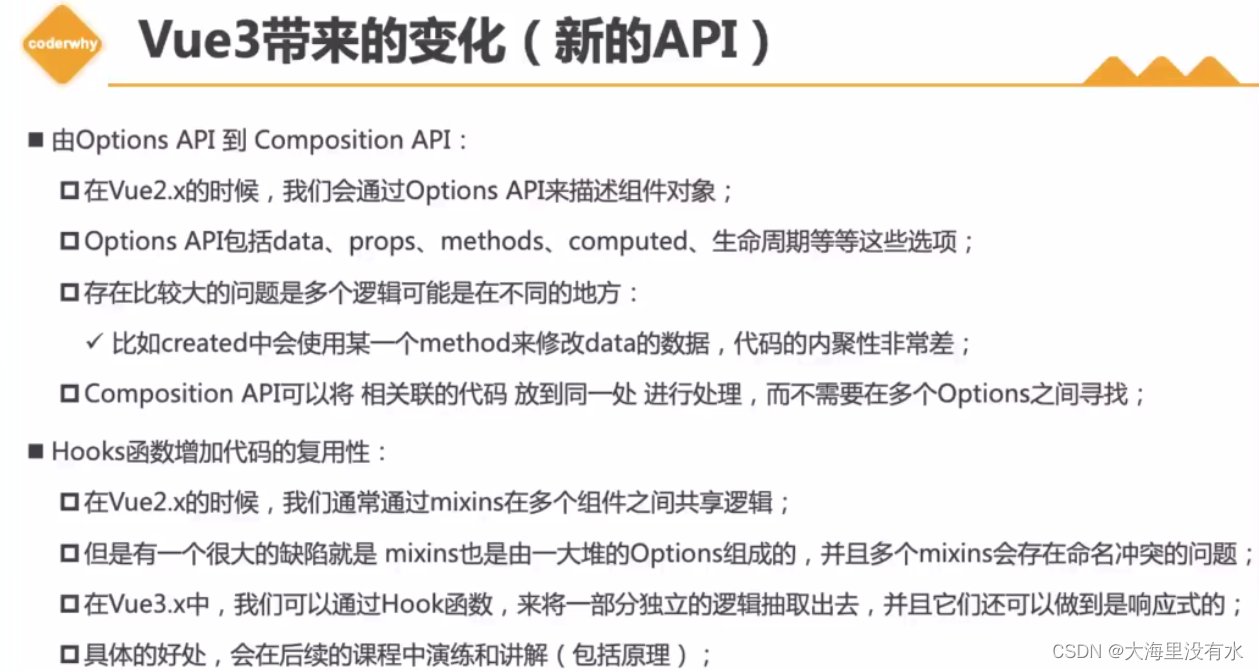
2、vue引入
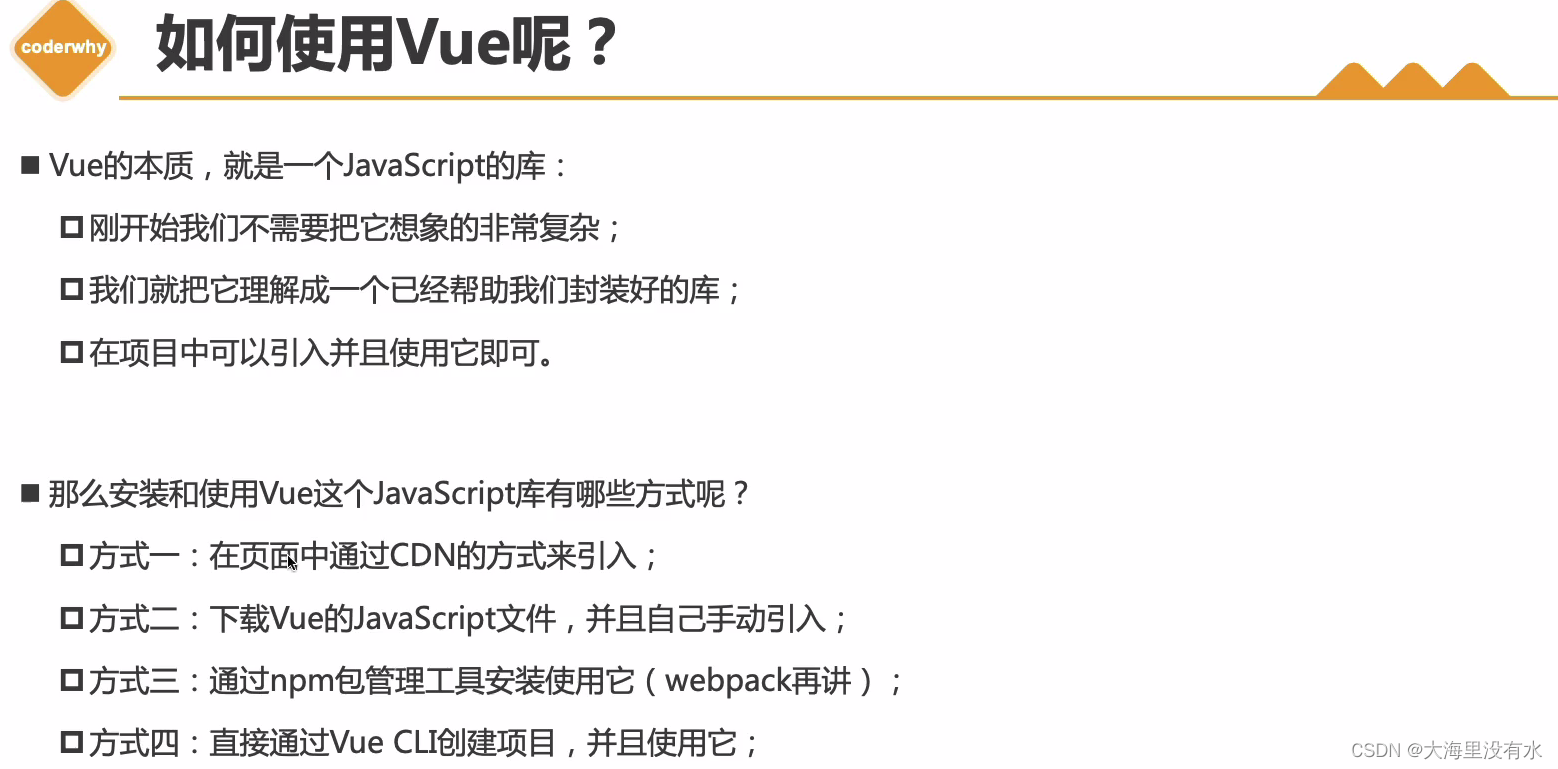
2.1、CDN引入
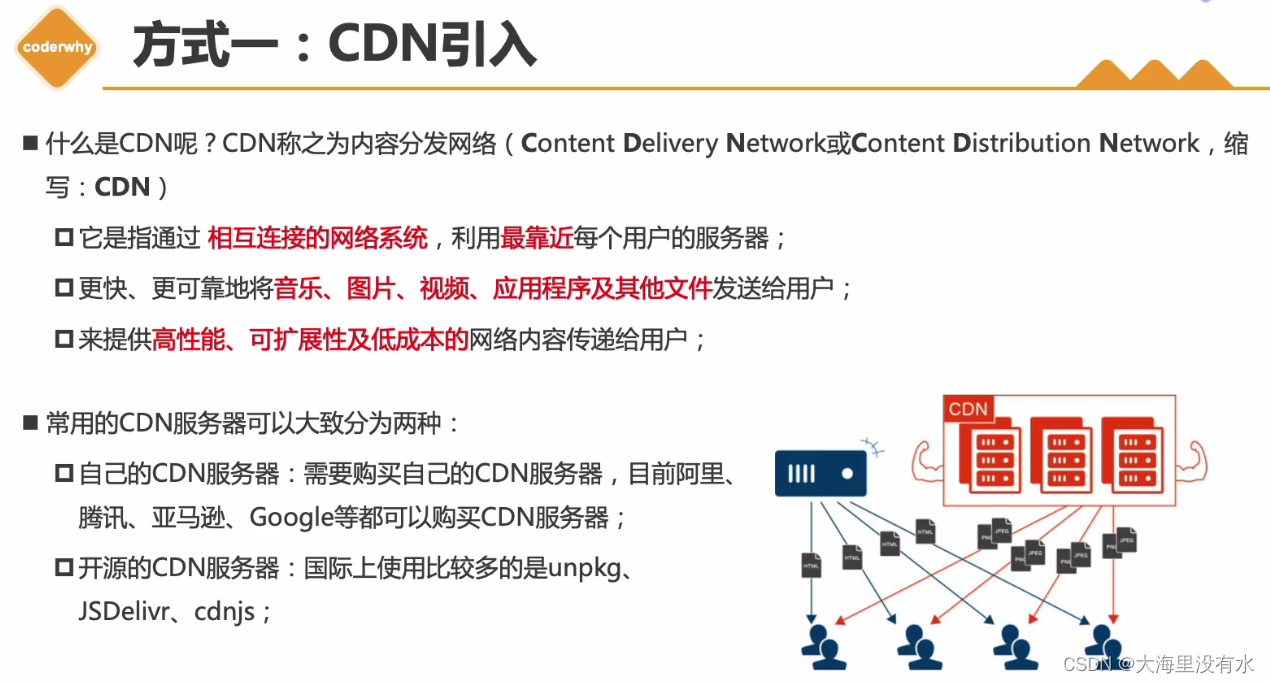
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<script src="https://unpkg.com/vue@3"></script>
<script>
const chen = {
template: "<h2>hello world</h2>",
};
const app = Vue.createApp(chen);
app.mount("#app");
</script>
</body>
</html>
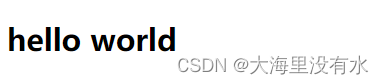
2.2、下载 - 本地引入
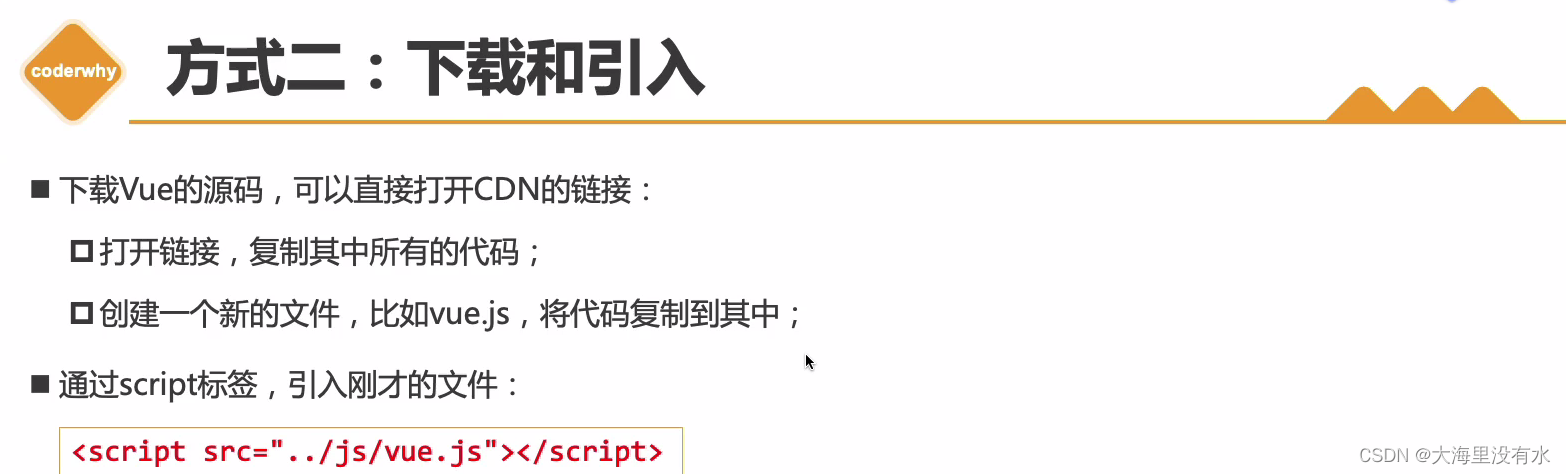
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<script src="./js/vue.js"></script>
<script>
Vue.createApp({
template: "<h2>hello world2</h2",
}).mount("#app");
</script>
</body>
</html>
3、计数器
3.1、原生实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h2 class="counter">0</h2>
<button class="increment" @click="increment">+1</button>
<button class="decrement" @click="decrement">-1</button>
<script>
const counterEL = document.querySelector(".counter");
const incrementEL = document.querySelector(".increment");
const decrementEL = document.querySelector(".decrement");
let counter = 100;
counterEL.innerHTML = counter;
incrementEL.addEventListener("click", () => {
counter += 1;
counterEL.innerHTML = counter;
});
decrementEL.addEventListener("click", () => {
counter -= 1;
counterEL.innerHTML = counter;
});
</script>
</body>
</html>
3.2、vue实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app">
哈哈哈
</div>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: `
<div>
<h2>{{message}}</h2>
<h2>{{counter}}</h2>
<button @click="increment">+1</button>
<button @click="decrement">-1</button>
</div>
`,
data: function () {
return {
message: "hello world vue.js",
counter: 100,
};
},
methods: {
increment() {
console.log("点击了加1");
this.counter++;
},
decrement() {
console.log("点击了减1");
this.counter--;
},
},
}).mount("#app");
</script>
</body>
</html>
4、声明式和命令式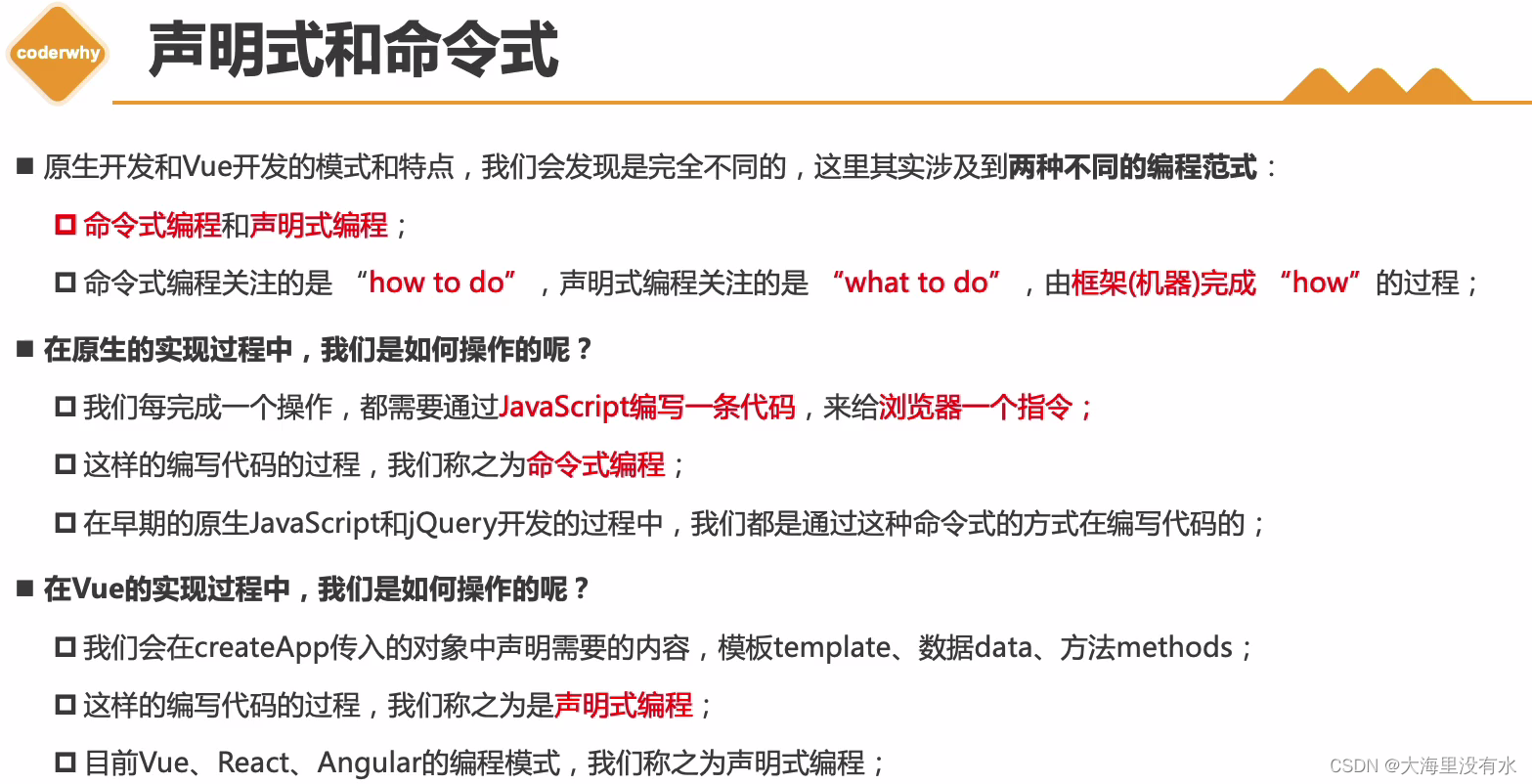
5、MVVM
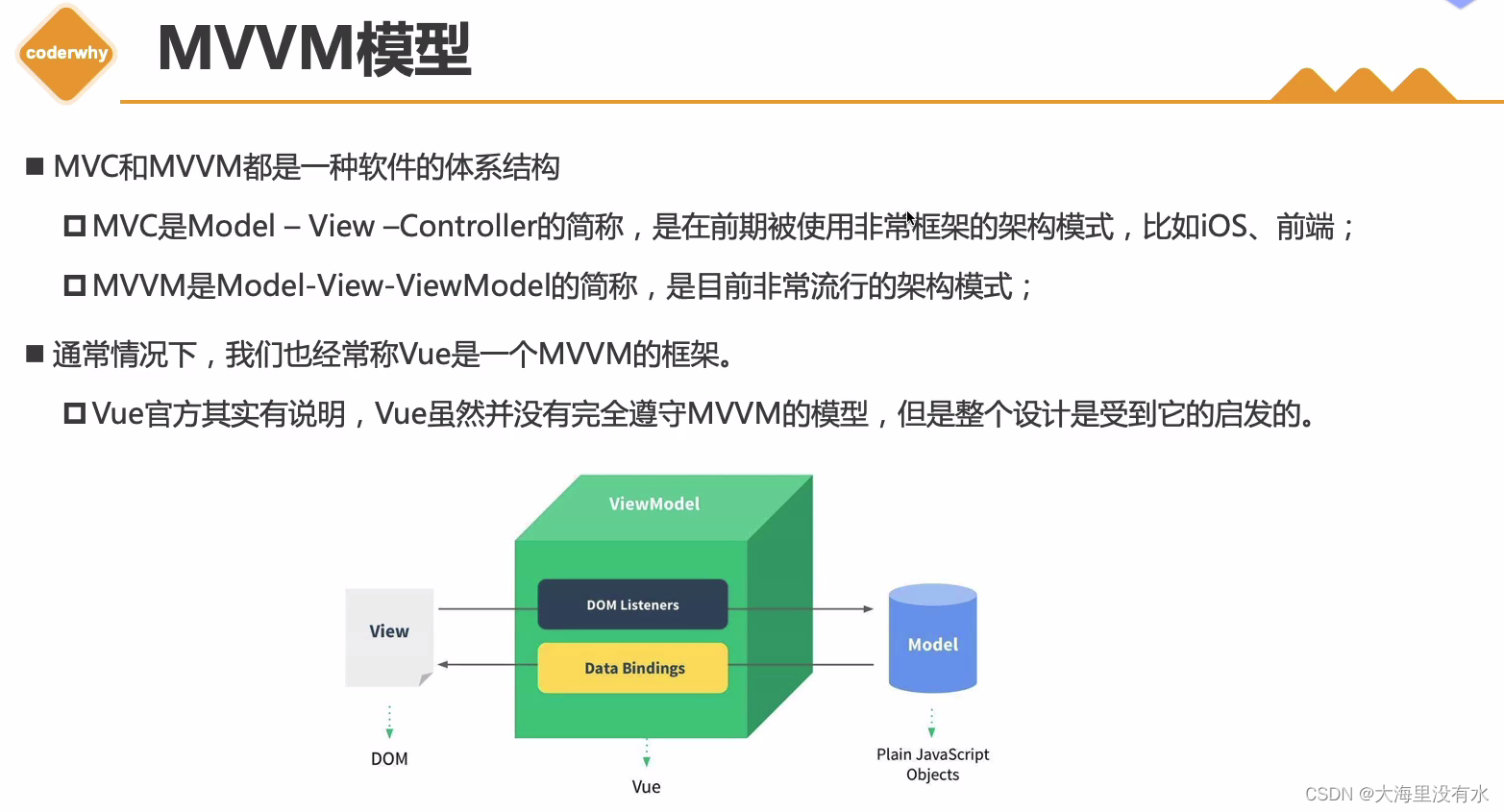
6、template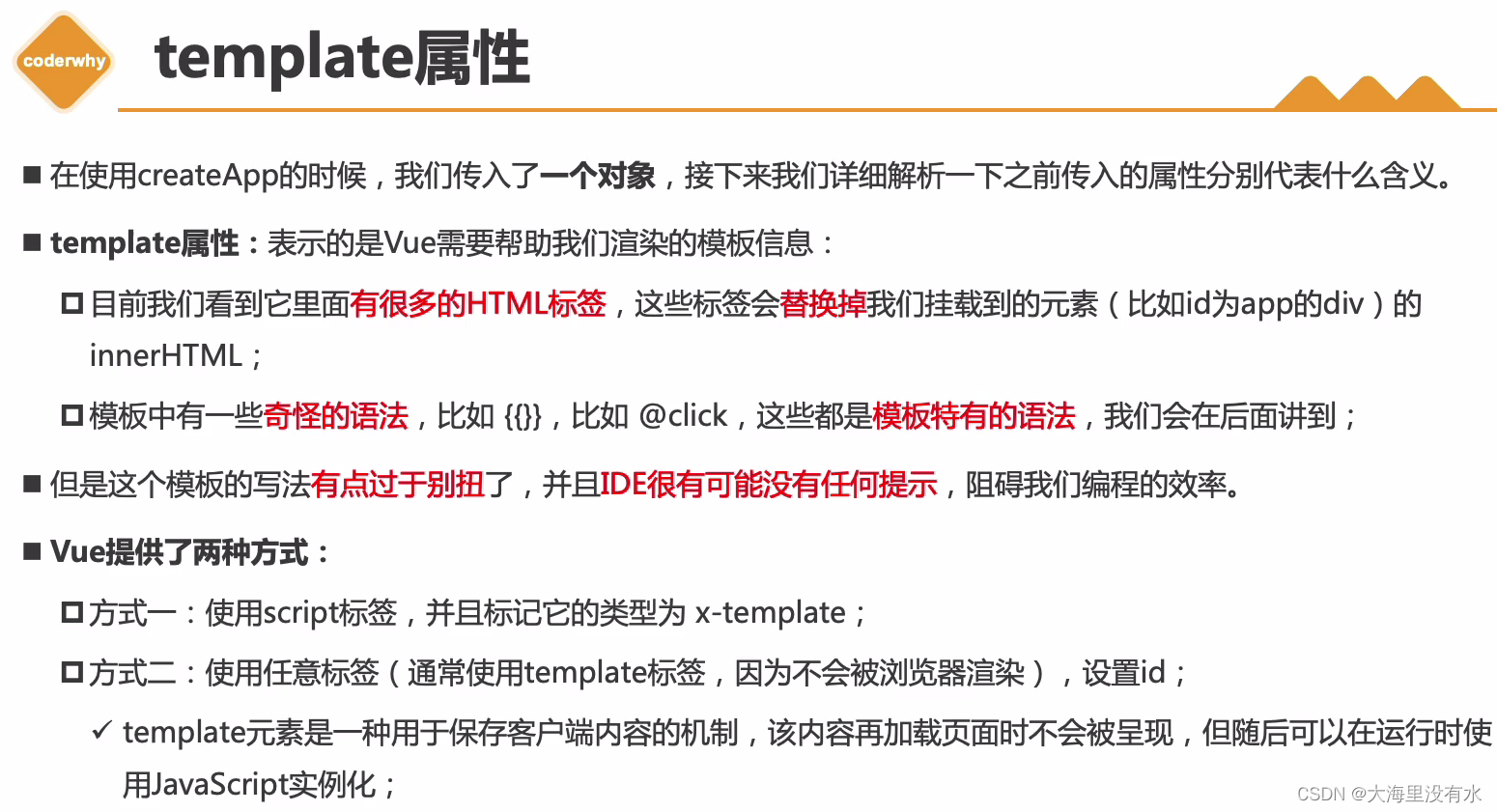
6.1、template写法一:
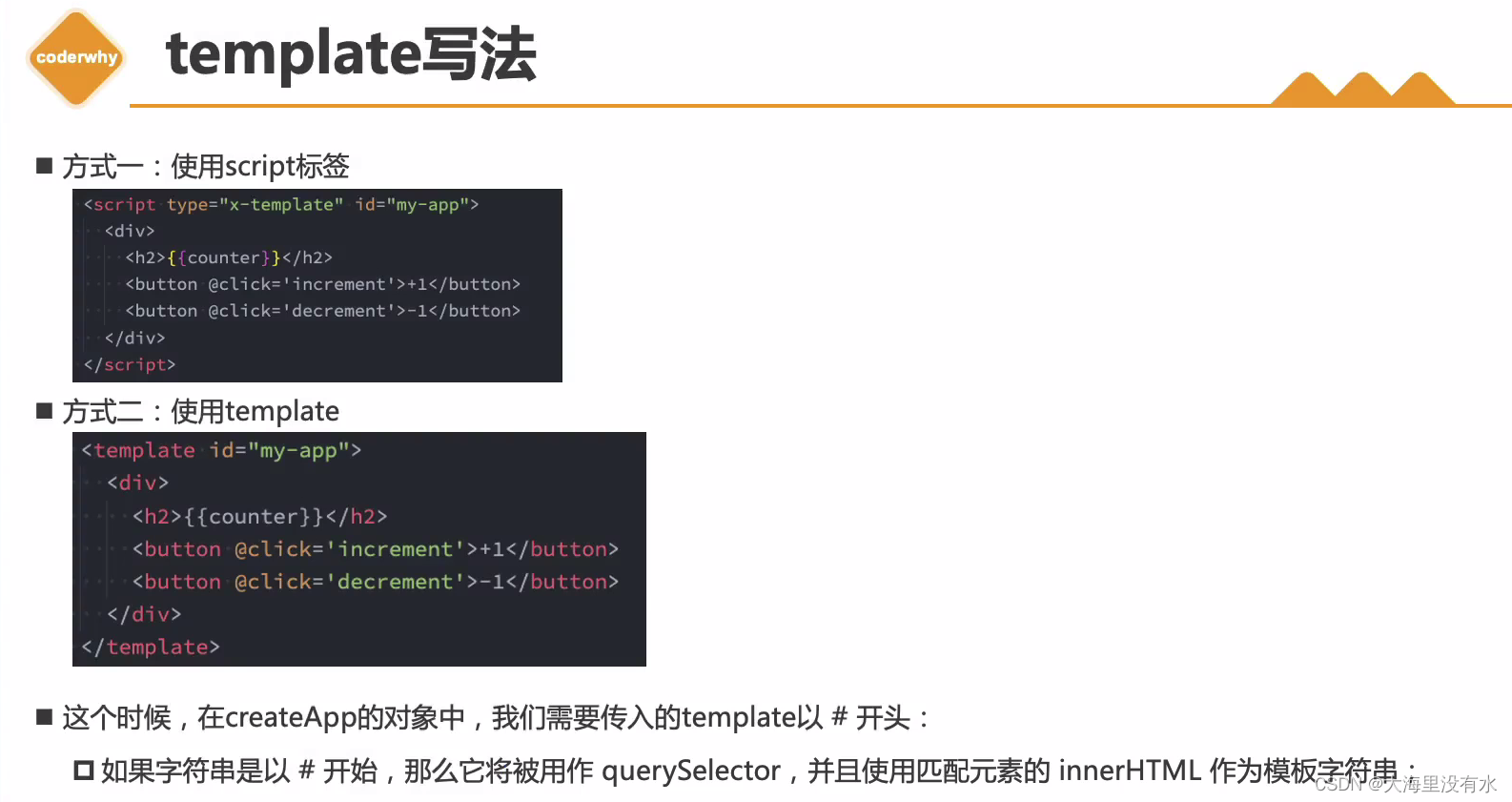
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app">哈哈哈</div>
<script type="x-template" id="chen">
<div>
<h2>{{message}}</h2>
<h2>{{counter}}</h2>
<button @click="increment">+1</button>
<button @click="decrement">-1</button>
</div>
</script>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
message: "hello world vue.js",
counter: 100,
};
},
methods: {
increment() {
console.log("点击了加1");
this.counter++;
},
decrement() {
console.log("点击了减1");
this.counter--;
},
},
}).mount("#app");
</script>
</body>
</html>
6.2、template写法2:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app">哈哈哈</div>
<template id="chen">
<div>
<h2>{{message}}</h2>
<h2>{{counter}}</h2>
<button @click="increment">+1</button>
<button @click="decrement">-1</button>
</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
message: "hello world vue.js",
counter: 100,
};
},
methods: {
increment() {
console.log("点击了加1");
this.counter++;
},
decrement() {
console.log("点击了减1");
this.counter--;
},
},
}).mount("#app");
const foo = function () {
console.log(this);
};
foo();
const obj = { bar: foo };
obj.bar();
</script>
</body>
</html>
7、data
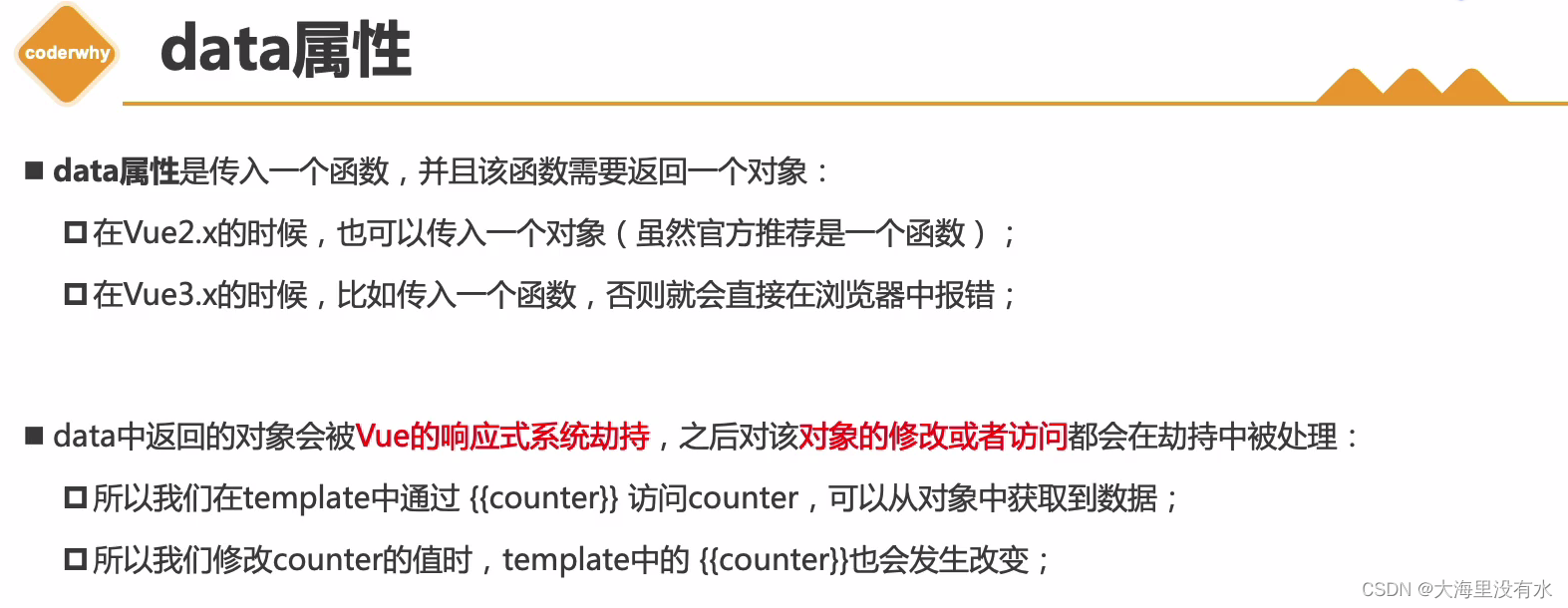
8、method
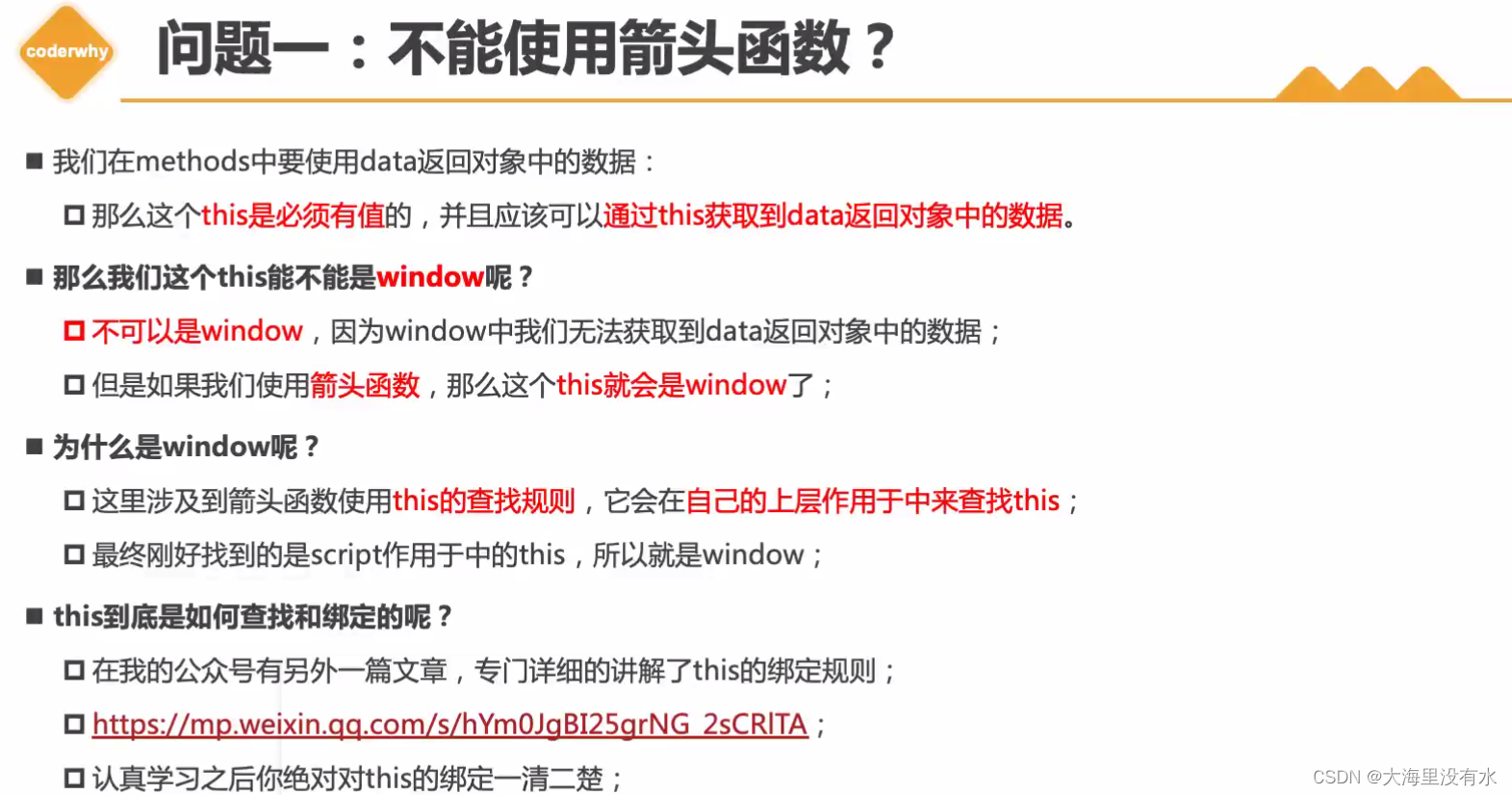
注:this绑定是就是我们组件对应的代理对象了 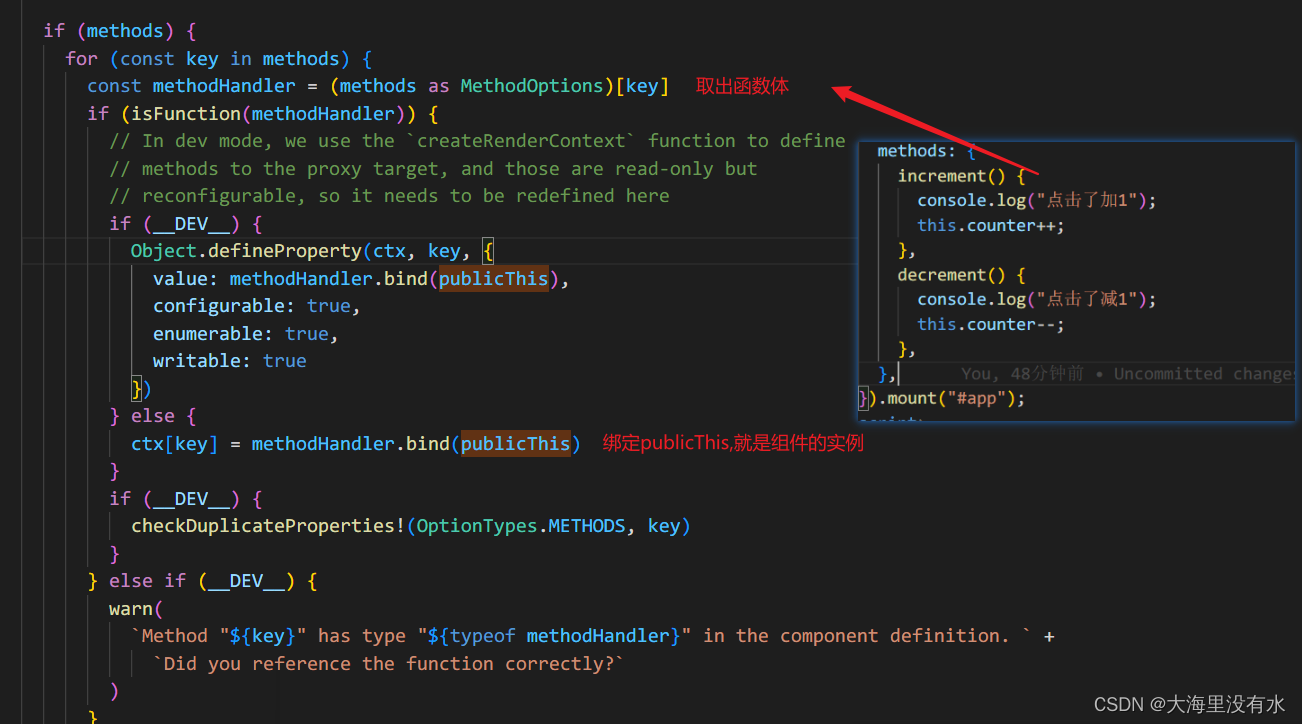
9、语法
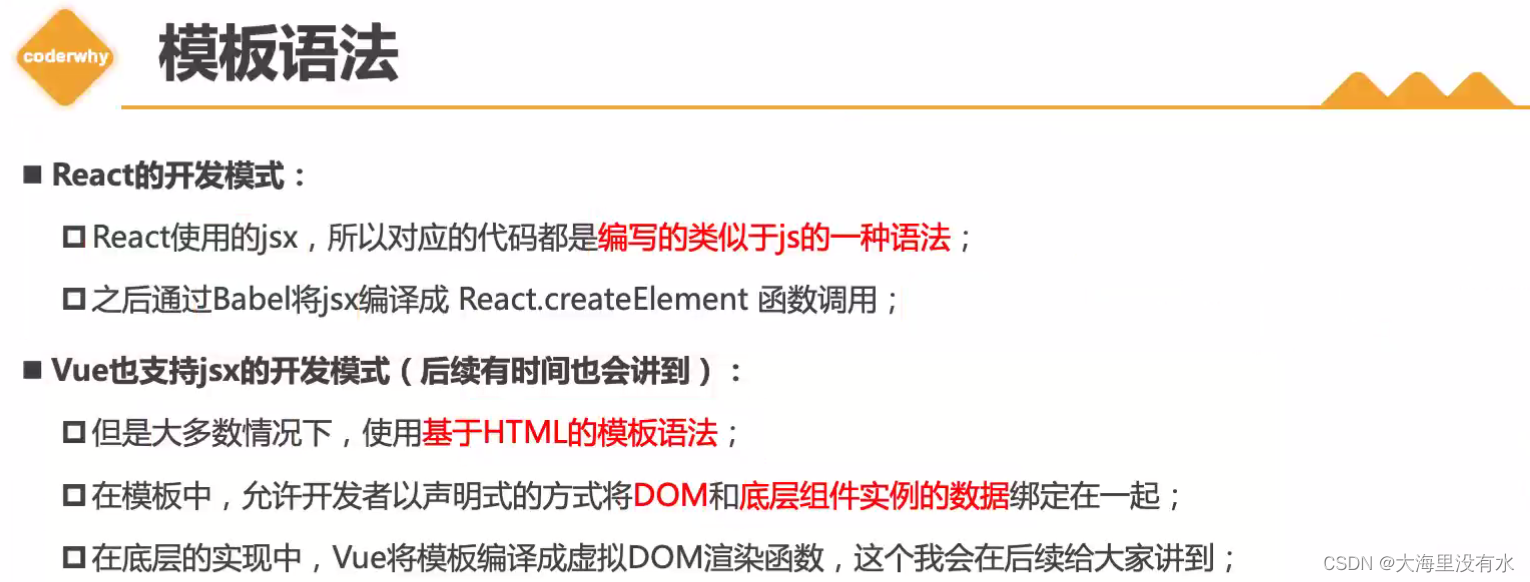
9.1、Mustche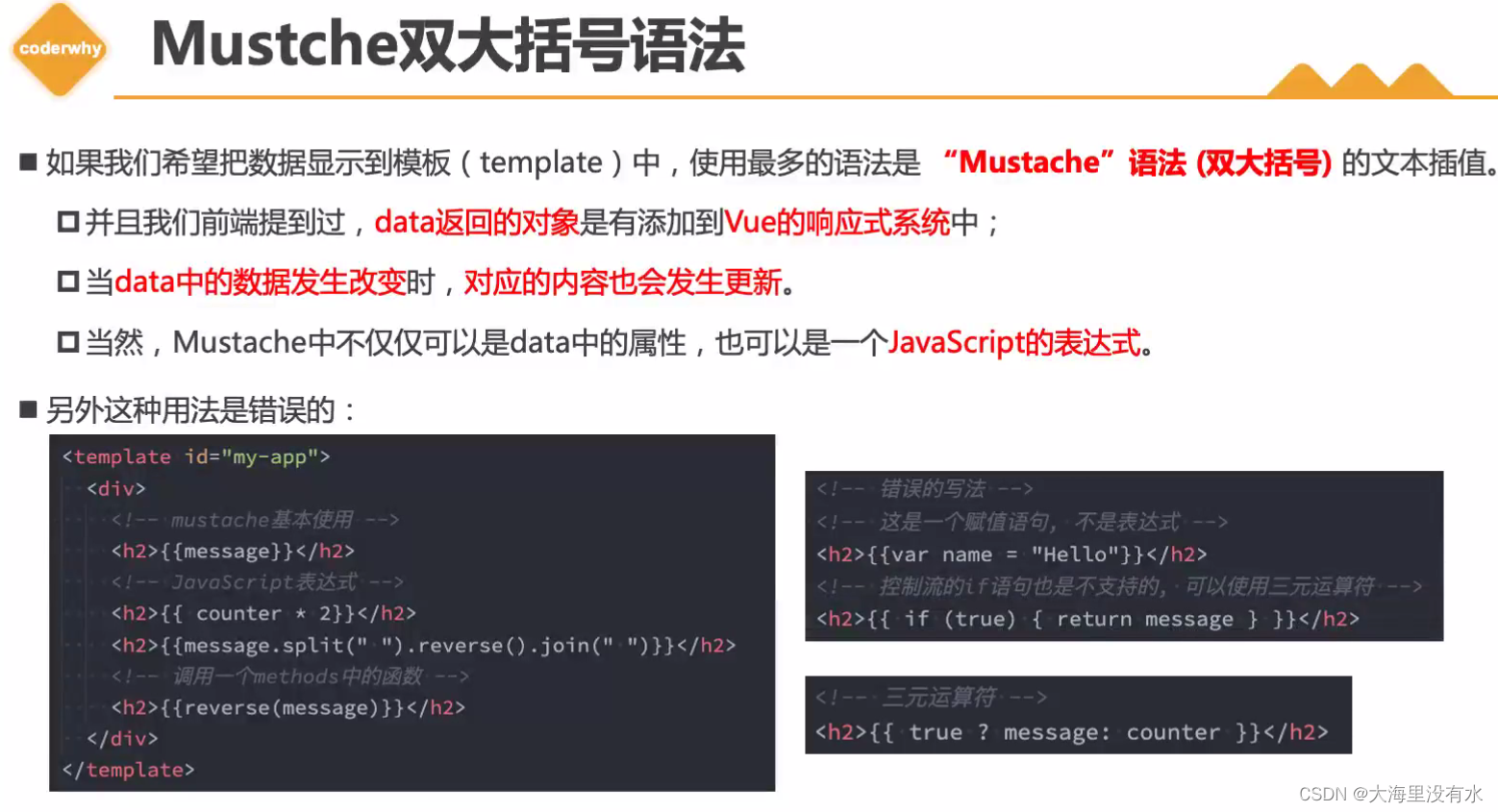
9.2、v-once
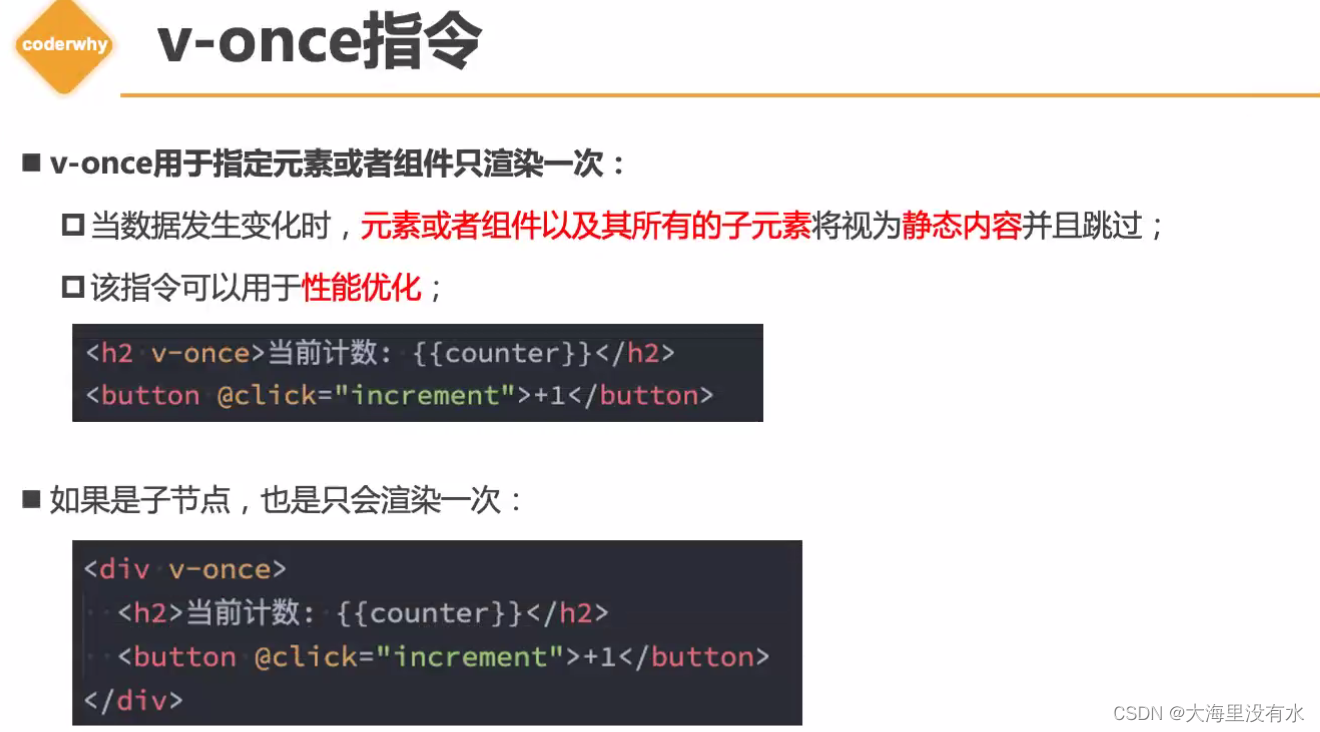
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<h2>{{counter}}</h2>
<div v-once>
<h2>{{counter}}</h2>
<h2>{{message}}</h2>
</div>
<button @click="increment">+1</button>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
counter: 100,
message: "hello",
};
},
methods: {
increment() {
this.counter++;
},
},
}).mount("#app");
</script>
</body>
</html>
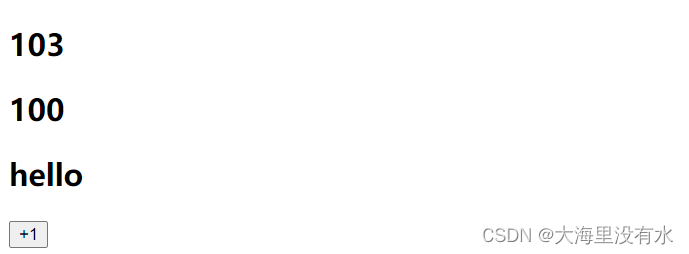
9.3、v-html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<div>
{{message}}
</div>
<div v-html="message"></div>
</template>
<script src="../js/vue.js"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
message:
'<span style="color: red; background: bule">哈哈哈哈</span>',
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>

9.4、v-pre
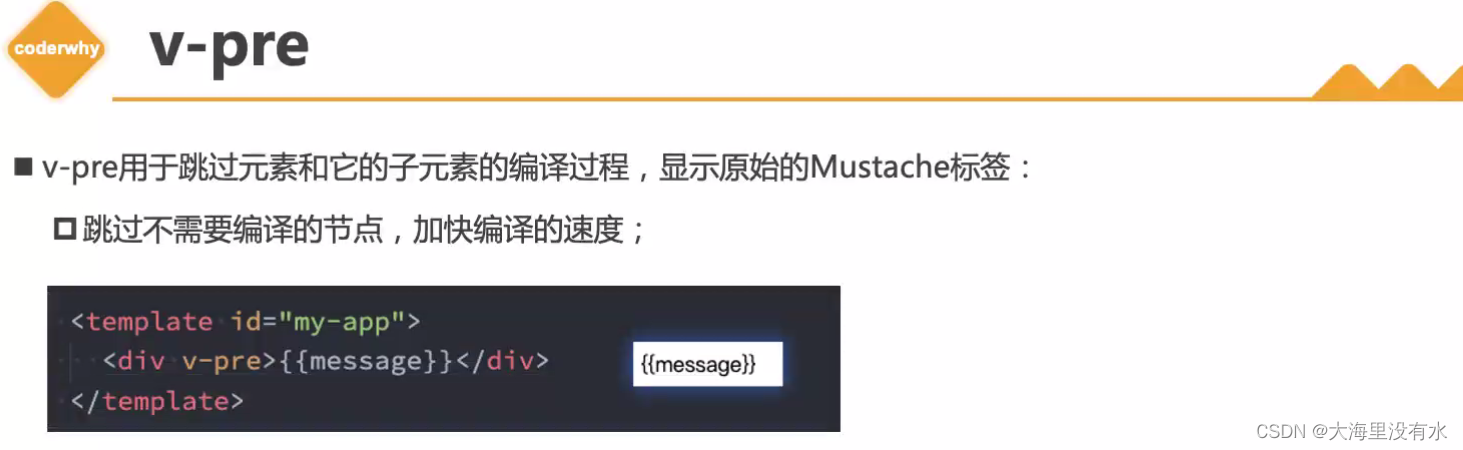
9.5、v-bind
9.5.1、基本使用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<img :src="imgUrl" />
<a :href="link">百度一下</a>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
imgUrl: "https://avatars.githubusercontent.com/u/54337779?s=40&v=4",
link: "https://www.baidu.com",
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>

9.5.2、v-bind绑定class - 对象语法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
.active {
color: red;
}
</style>
</head>
<body>
<div id="app"></div>
<template id="chen">
<div :class="{'active': isActive, 'title': true}">小陈必胜</div>
<div class="abc dde" :class="{'active': isActive, 'title': true}">
小陈必胜
</div>
<button @click="toggle">切换</button>
<div class="abc dde" :class="classObj">小陈必胜</div>
<div class="abc dde" :class="getClassObj()">小陈必胜</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
message: "hello world",
isActive: false,
classObj: { active: true, title: true },
};
},
methods: {
toggle() {
this.isActive = !this.isActive;
},
getClassObj() {
return {
active: true,
title: true,
};
},
},
}).mount("#app");
</script>
</body>
</html>
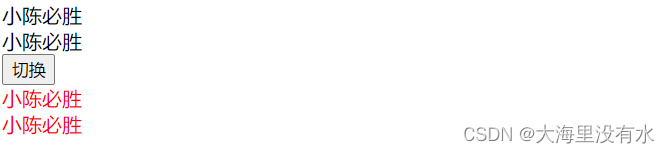
9.5.3、v-bind绑定class - 数组语法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
.active {
color: red;
}
</style>
</head>
<body>
<div id="app"></div>
<template id="chen">
<div :class="['abc', title]">哈哈哈</div>
<div :class="['abc', title, isActive ? 'active': '', {active: isActive}]">
哈哈哈a
</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
title: "ddd",
isActive: true,
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>

9.5.4、v-bind绑定style- 对象语法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<div style="color: red">嘻嘻嘻</div>
<div :style="{color: 'red'}">哈哈哈</div>
<div :style="{color: finalColor}">哈哈哈</div>
<div :style="{color: finalColor, 'font-size': '59px'}">哈哈哈</div>
<div :style="finalStyleObj">呵呵黑</div>
<div :style="getfinalStyleObj()">呵呵黑2</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
finalColor: "red",
finalStyleObj: {
fontSize: "20px",
backgroundColor: "red",
},
};
},
methods: {
getfinalStyleObj() {
return {
fontSize: "20px",
backgroundColor: "blue",
};
},
},
}).mount("#app");
</script>
</body>
</html>
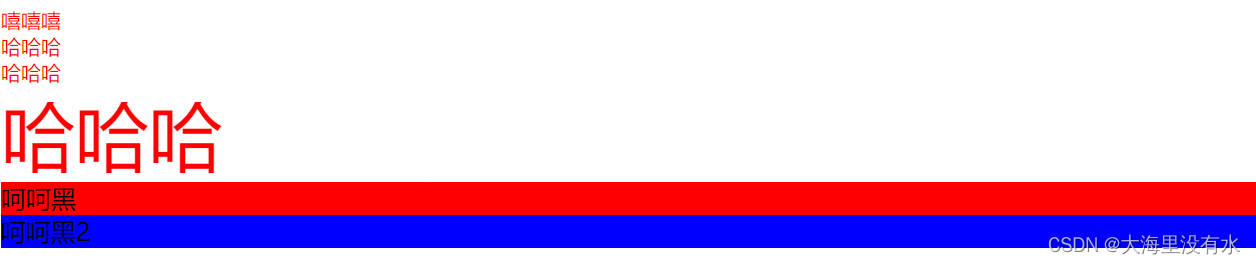
9.5.5、 v-bind绑定style- 数组语法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<div :style="[styleObj, styleObj1]">hhh</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
styleObj: {
color: "red",
fontSize: "23px",
},
styleObj1: {
textDecoration: "underline",
},
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
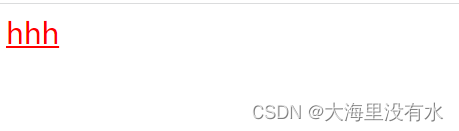
9.5.6、v-bind 动态绑定属性名称
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<div :[name]="value">呵呵黑</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
name: "abcde",
value: "keobd",
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
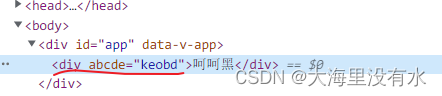
9.5.7、v-bind 属性直接绑定一个对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<div :class="info">陈氏史实</div>
<div :="info">陈氏史实2</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
info: {
name: "chen",
age: 23,
height: 2.4,
},
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
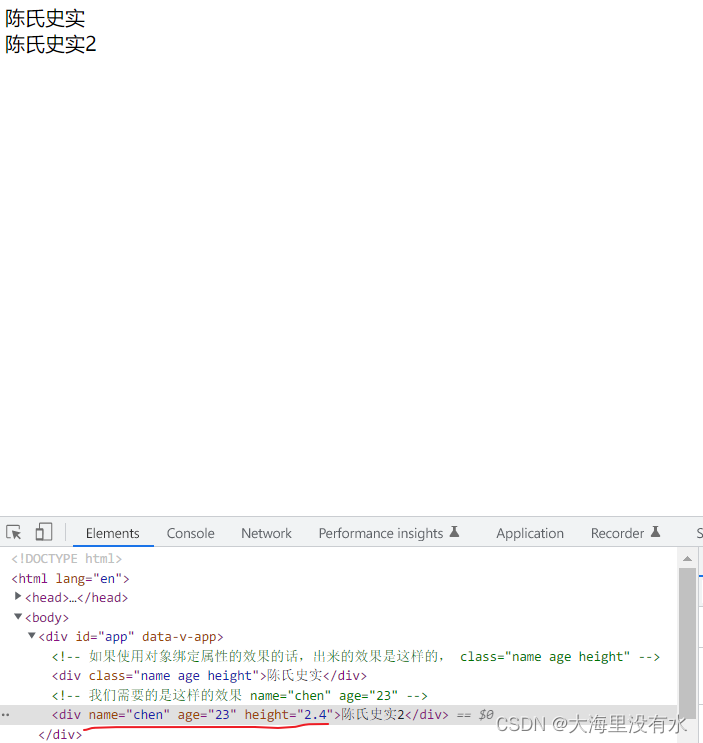
9.6、v-on绑定事件
9.6.1、v-on基本使用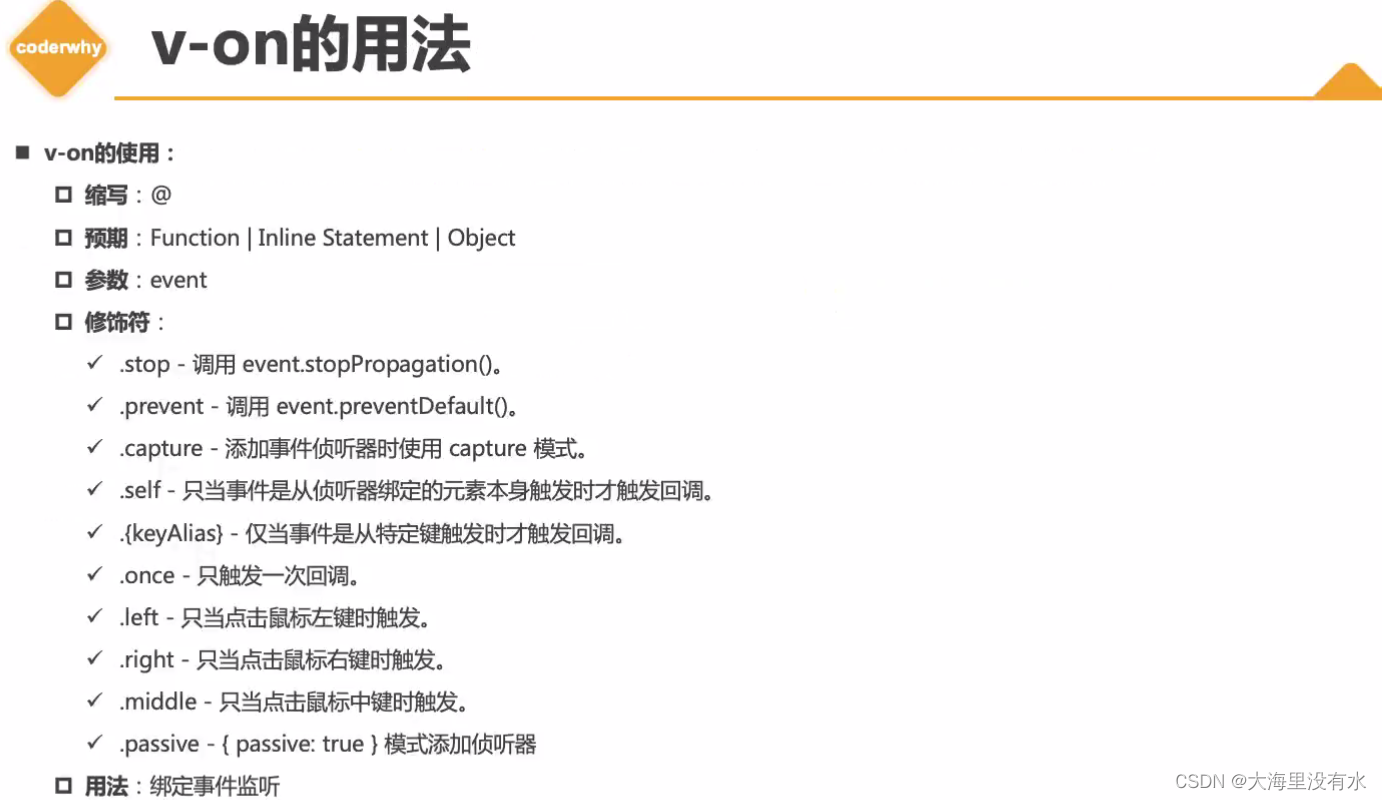
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
.area {
width: 300px;
height: 300px;
background: red;
}
</style>
</head>
<body>
<div id="app"></div>
<template id="chen">
<button @click="btnClick">按钮1</button>
<div class="area" @mousemove="mouse">区域</div>
<button @click="counter++">{{counter}}</button>
<div class="area" v-on="{click: btnClick, mousemove: mouse}">
<div class="area" @="{click: btnClick, mousemove: mouse}">
{{counter}}
</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
counter: 1100,
};
},
methods: {
btnClick() {
console.log("点击了按钮1");
},
mouse() {
console.log("在area里面移动");
},
},
}).mount("#app");
</script>
</body>
</html>
9.6.2、v-on 传递参数
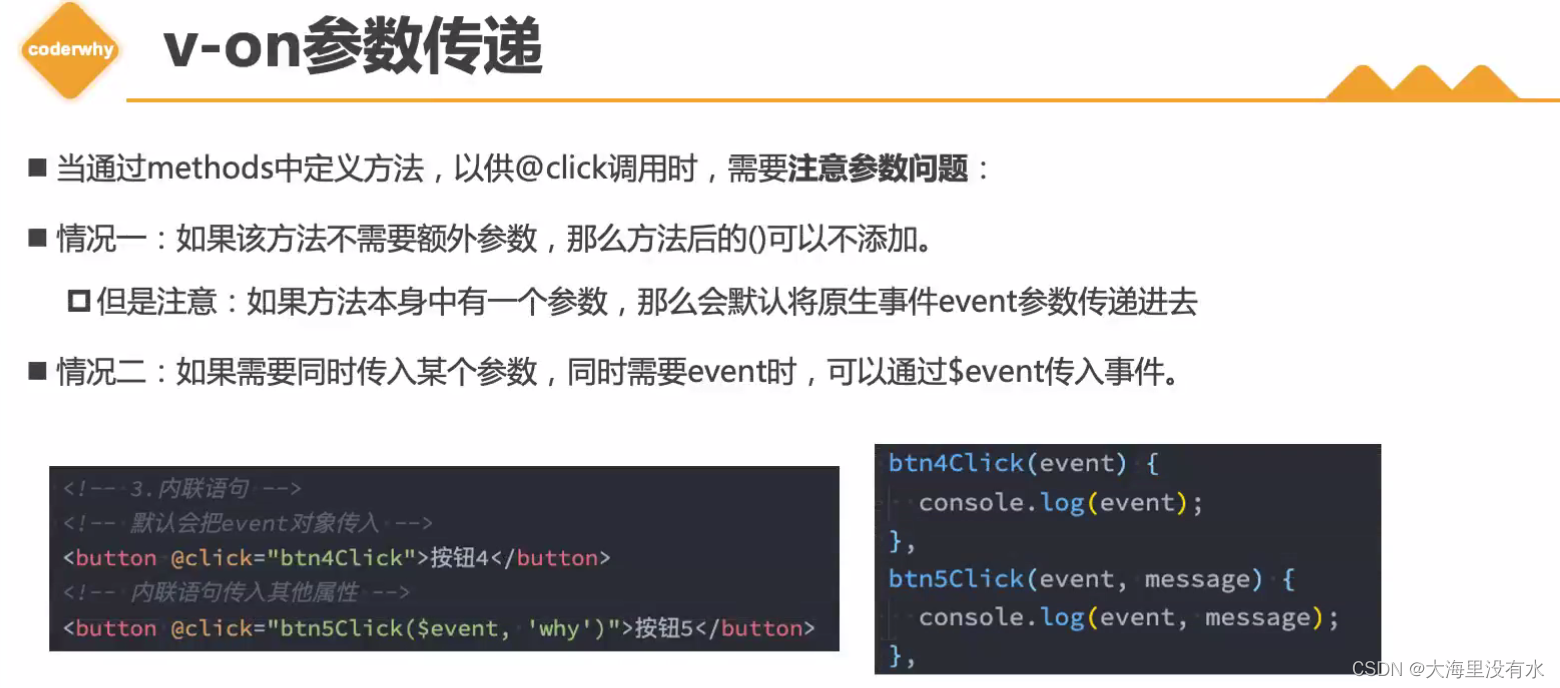
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<button @click="btnClick">按钮1</button>
<button @click="btnClick1($event, 'chen')">按钮1</button>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {};
},
methods: {
btnClick() {
console.log(event);
},
btnClick1(event, name) {
console.log(event);
console.log(name);
},
},
}).mount("#app");
</script>
</body>
</html>
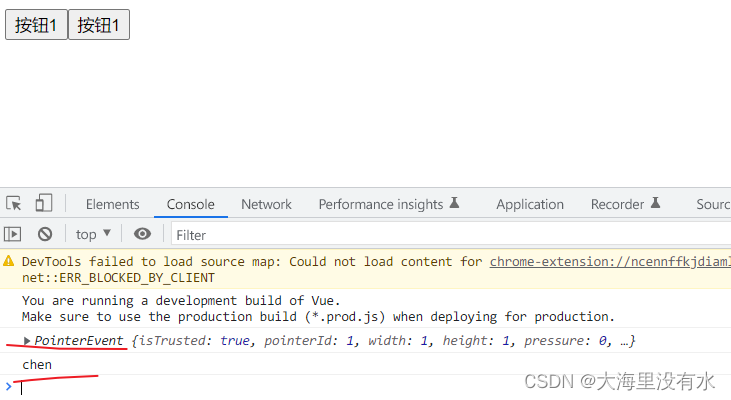
9.6.3、v-on 修饰符
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<div @click="divClick">
<button @click.stop="btnClick">按钮</button>
</div>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {};
},
methods: {
divClick() {
console.log("divclick");
},
btnClick() {
console.log("btnclick");
},
},
}).mount("#app");
</script>
</body>
</html>
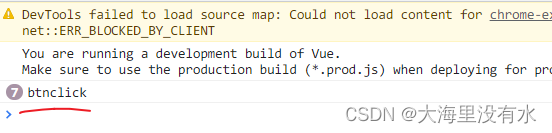
9.7、条件渲染
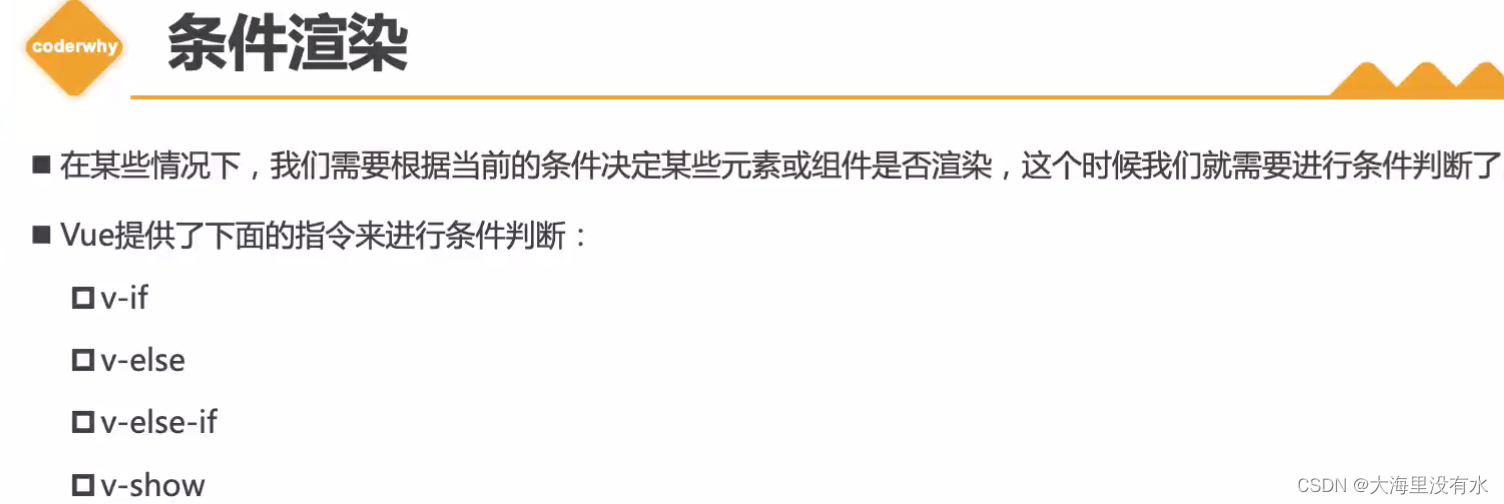 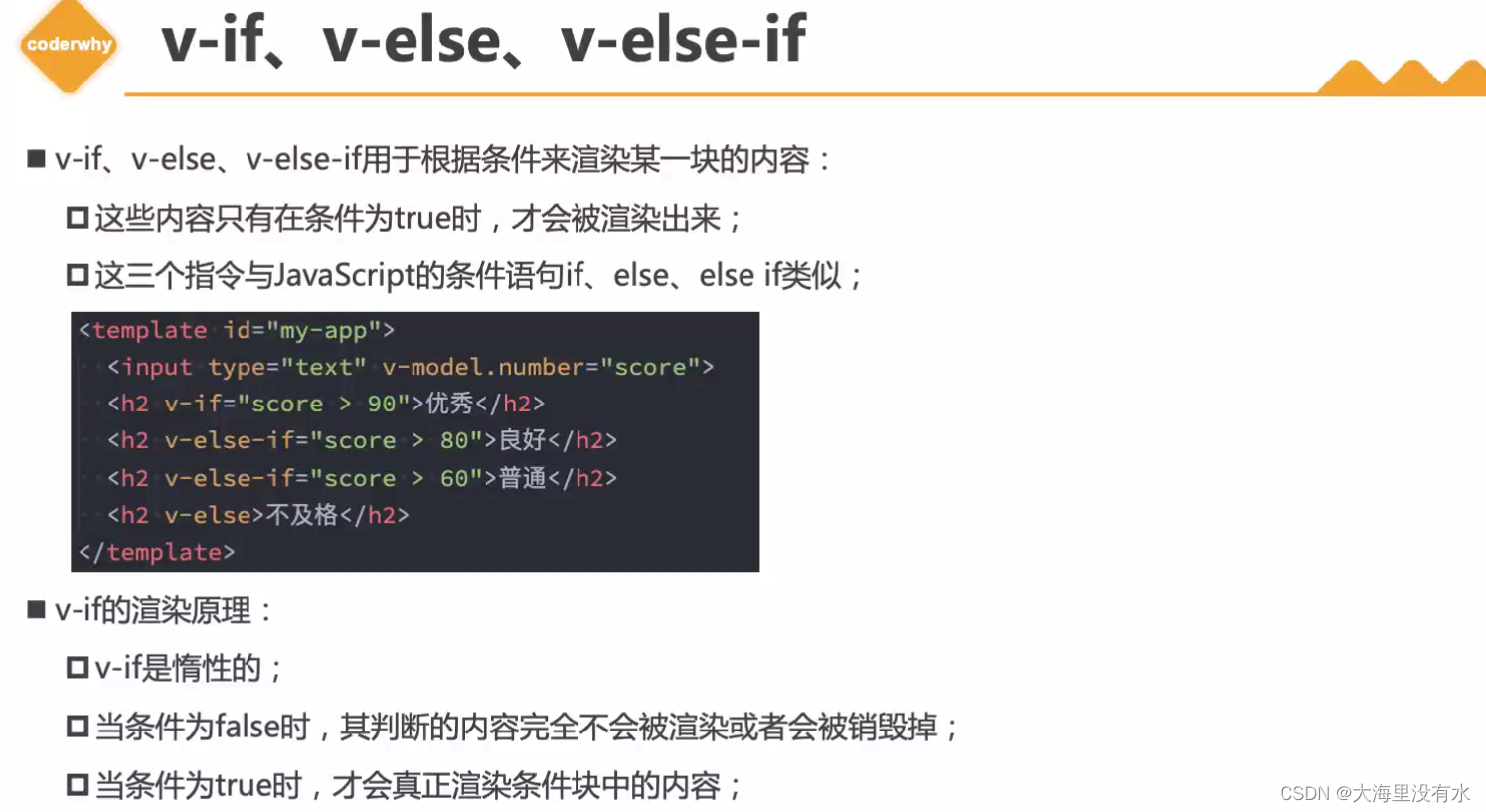
9.7.1、v-if多条件渲染
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<h2 v-if="score > 90">优秀</h2>
<h2 v-else-if="score > 60">良好</h2>
<h2 v-else>不及格</h2>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
score: 90,
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
9.7.2、v-if结合template
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<template v-if="isShowHa">
<h2>哈哈哈</h2>
<h2>哈哈哈</h2>
<h2>哈哈哈</h2>
</template>
<template v-else>
<h2>呵呵呵</h2>
<h2>呵呵呵</h2>
<h2>呵呵呵</h2>
</template>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
isShowHa: true,
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
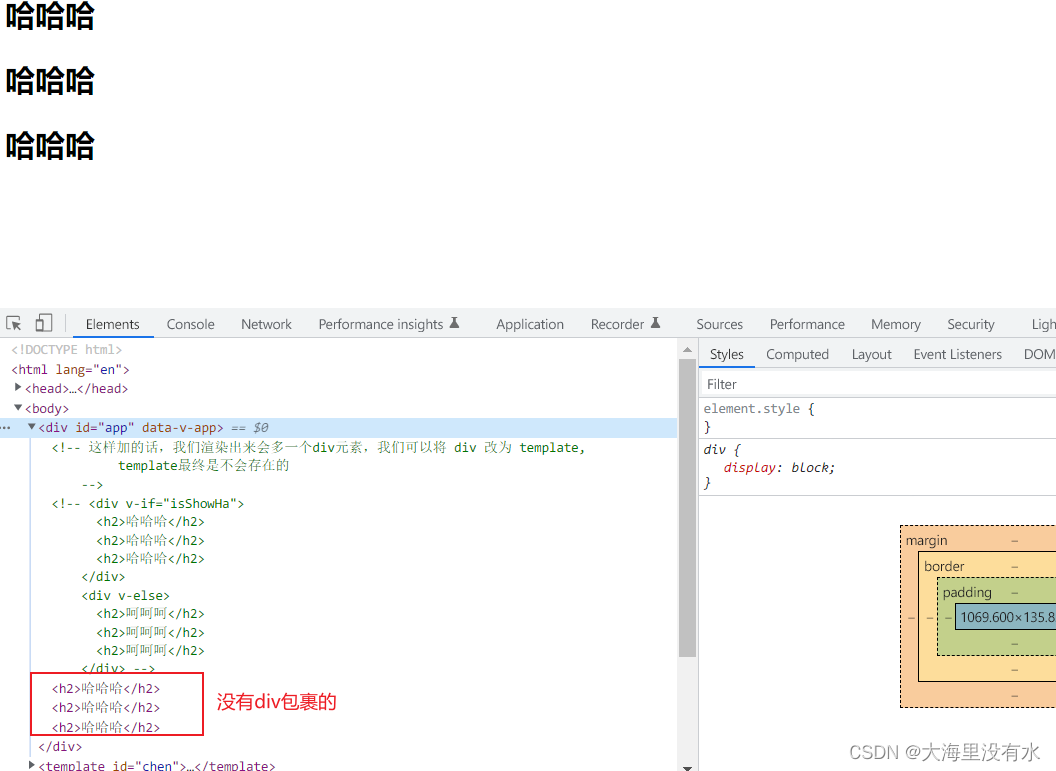
9.7.3、v-show
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<h2 v-if="isShow">哈哈哈哈</h2>
<h2 v-show="isShow">哈哈哈哈</h2>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
isShow: !true,
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
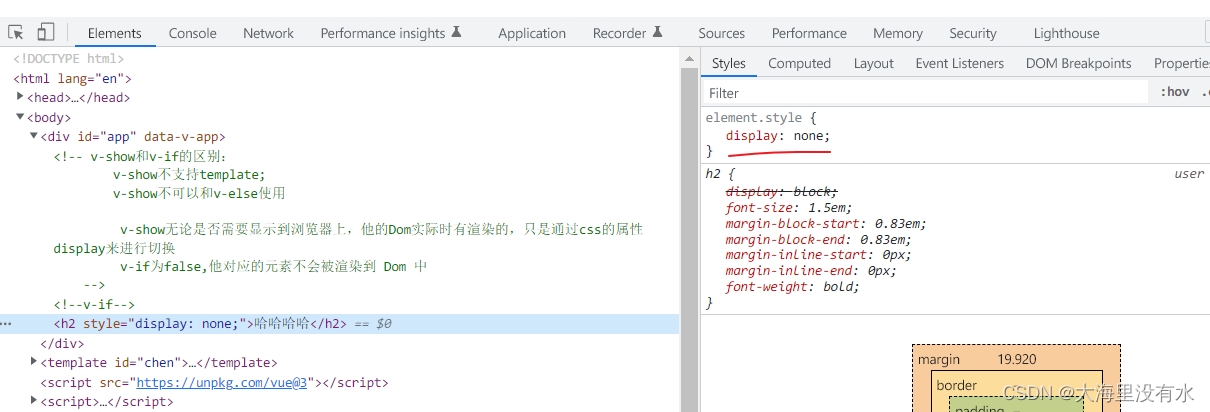 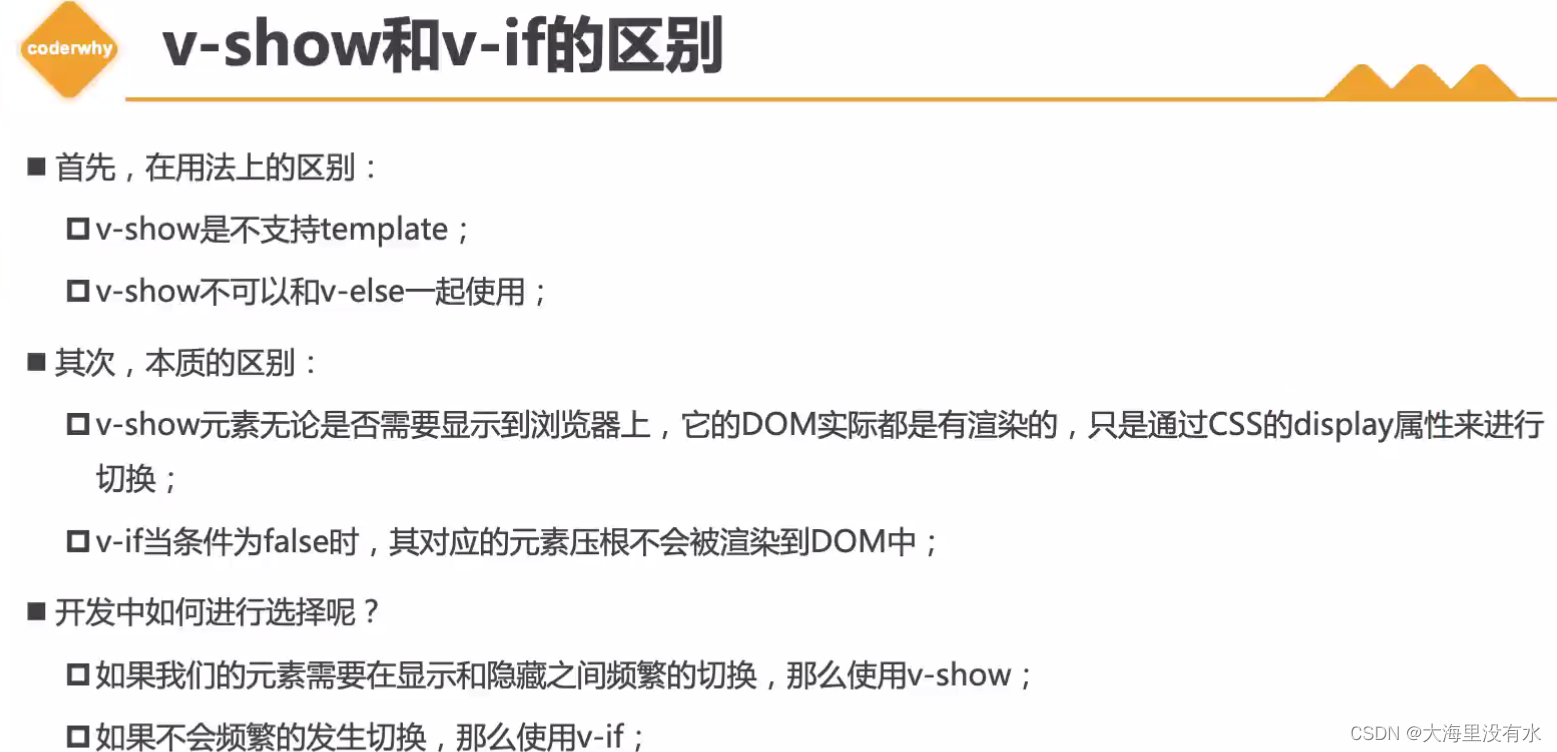
9.8、v-for
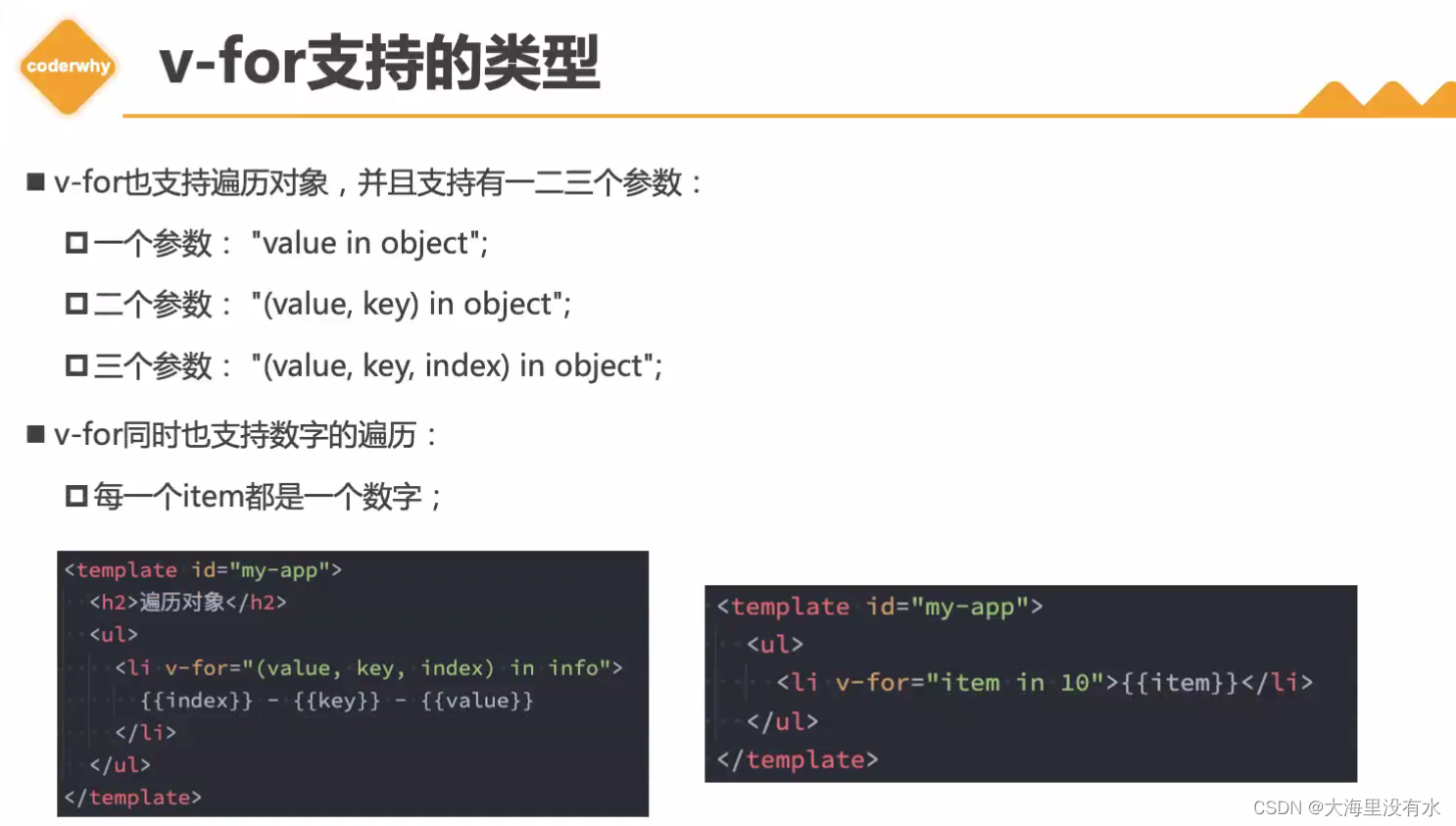
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<h2>动漫列表</h2>
<ul>
<li v-for="(item,index) in movies">{{index + 1}}.{{item}}</li>
</ul>
<h2>个人中心</h2>
<ul>
<li v-for="value in info">{{value}}</li>
</ul>
<ul>
<li v-for="(value,key) in info">{{value}}-{{key}}</li>
</ul>
<ul>
<li v-for="(value, key, index) in info">{{value}}-{{key}}-{{index}}</li>
</ul>
<ul>
<li v-for="(num, index) in 10">{{num}}-{{index}}</li>
</ul>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
movies: ["火影忍者", "斗罗大陆", "斗破苍穹"],
info: {
name: "Chen",
age: 12,
height: 12.2,
},
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
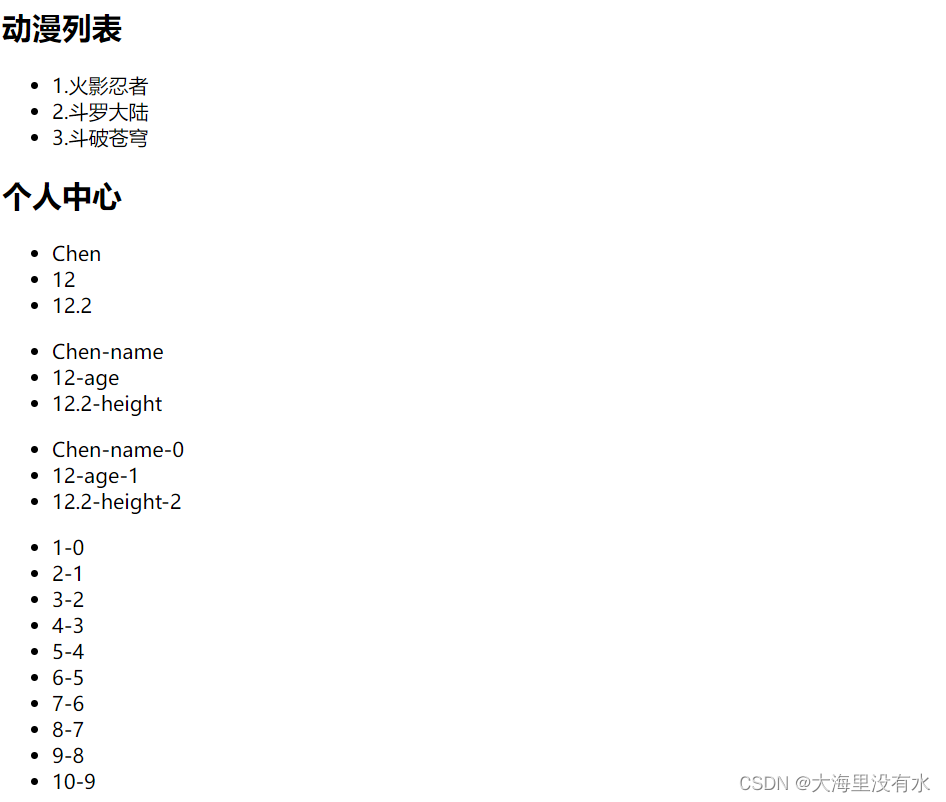
9.8.1 v-for与template结合使用
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
<style>
.line {
list-style: none;
border-top: 1px solid black;
margin-bottom: 10px;
}
</style>
</head>
<body>
<div id="app"></div>
<template id="chen">
<ul>
<div v-for="(value, key) in info">
<li>{{key}}</li>
<li>{{value}}</li>
<hr />
</div>
</ul>
<ul>
<template v-for="(value, key) in info">
<li>{{key}}</li>
<li>{{value}}</li>
<li class="line"></li>
</template>
</ul>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
info: {
name: "Chen",
age: 12,
height: 12.2,
},
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
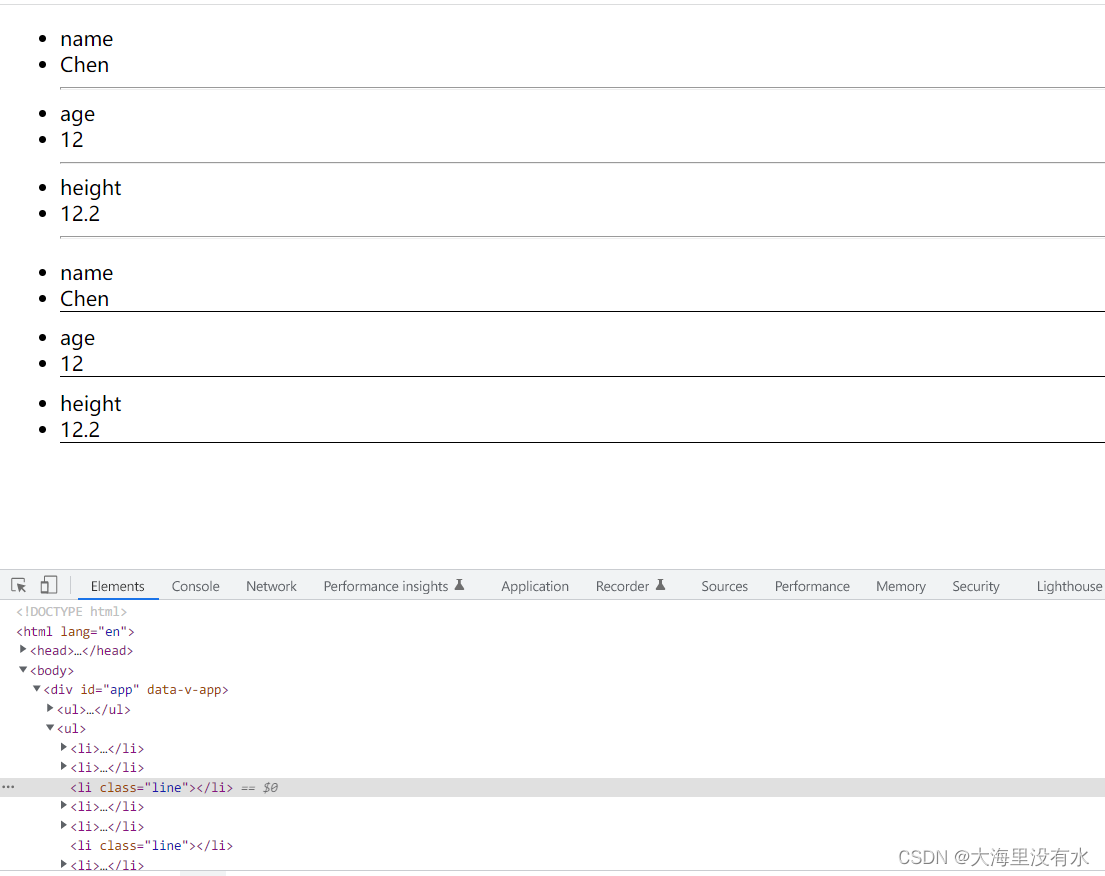
9.8.2、 v-for中key的作用
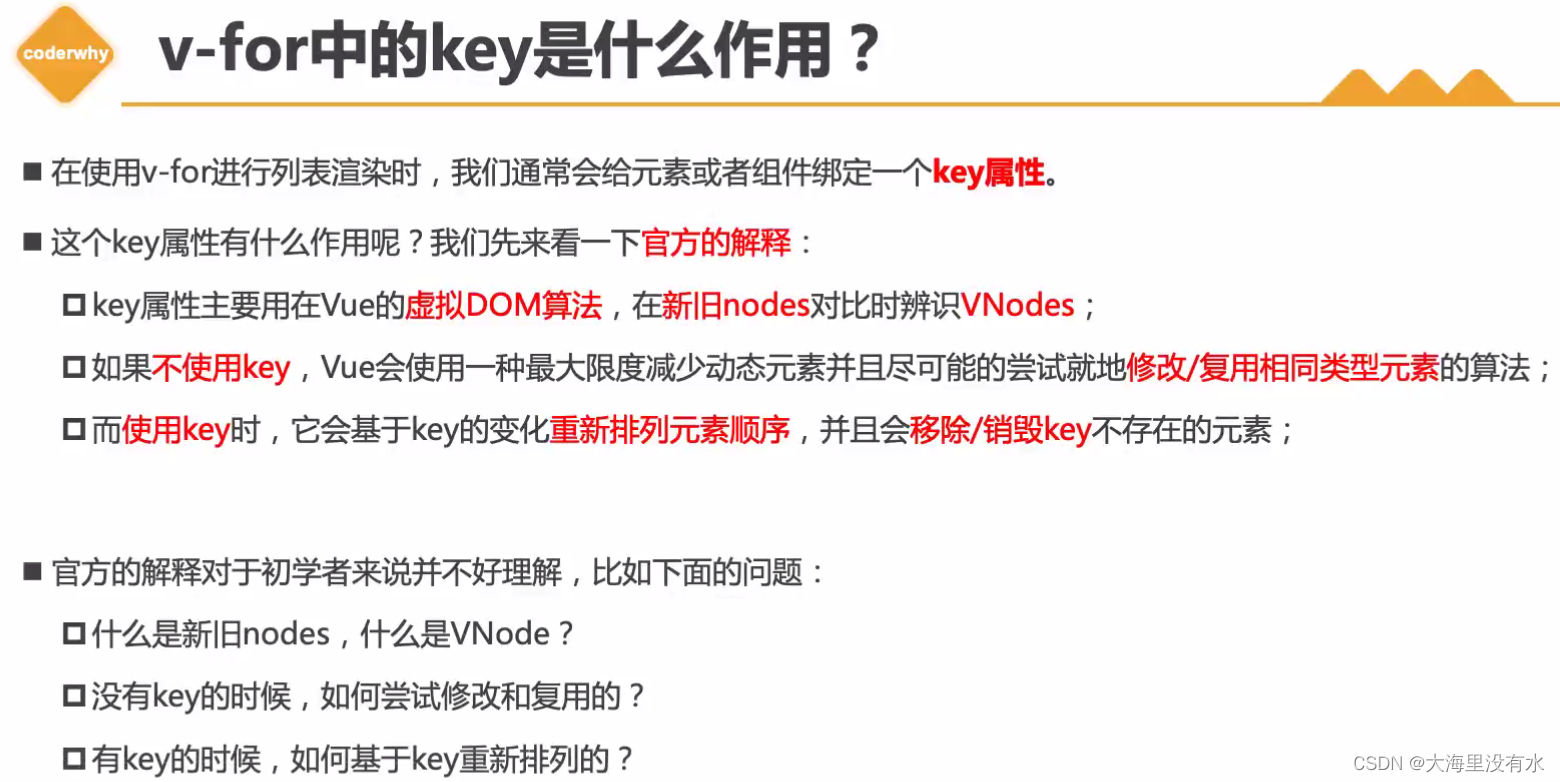
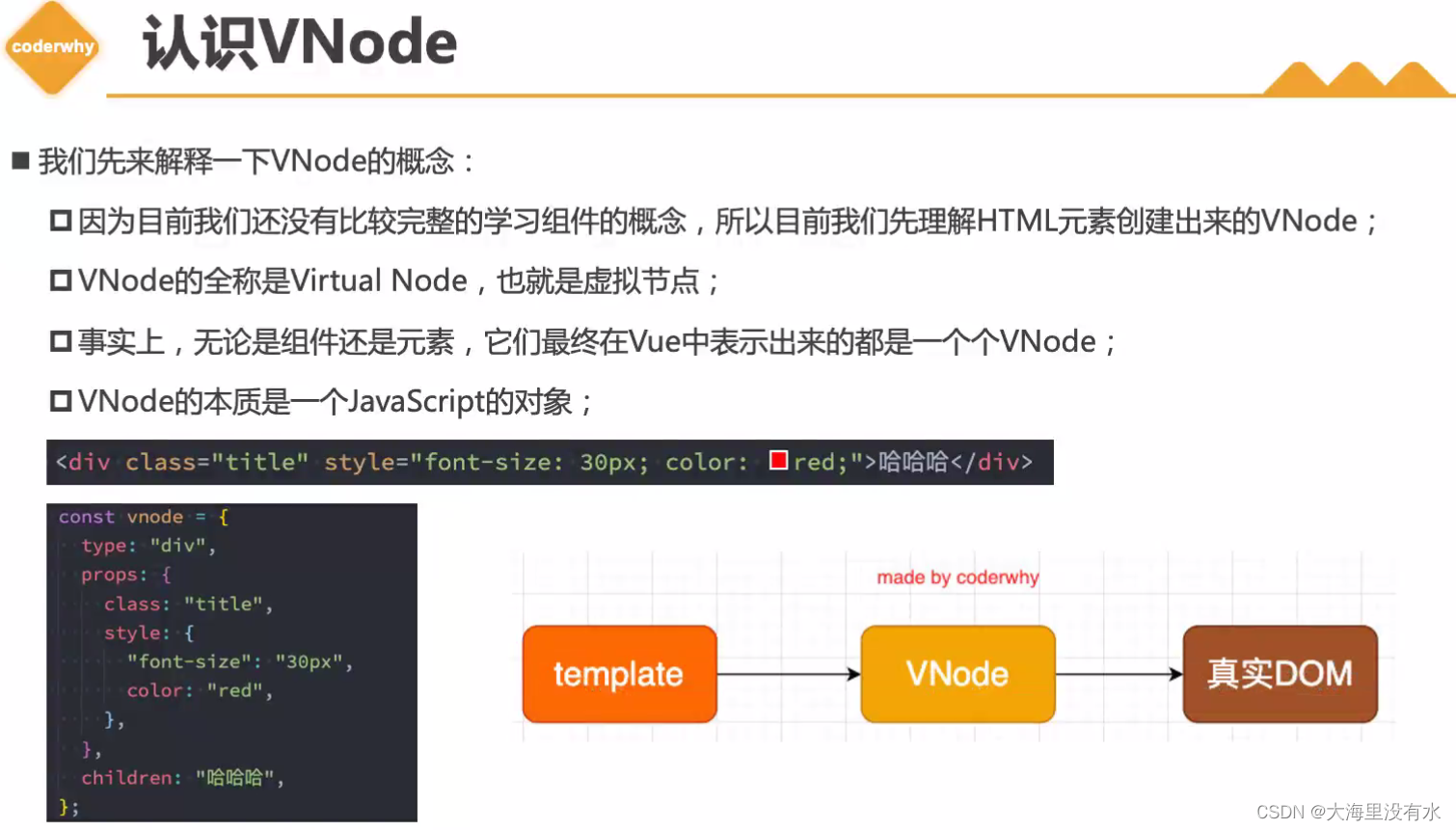
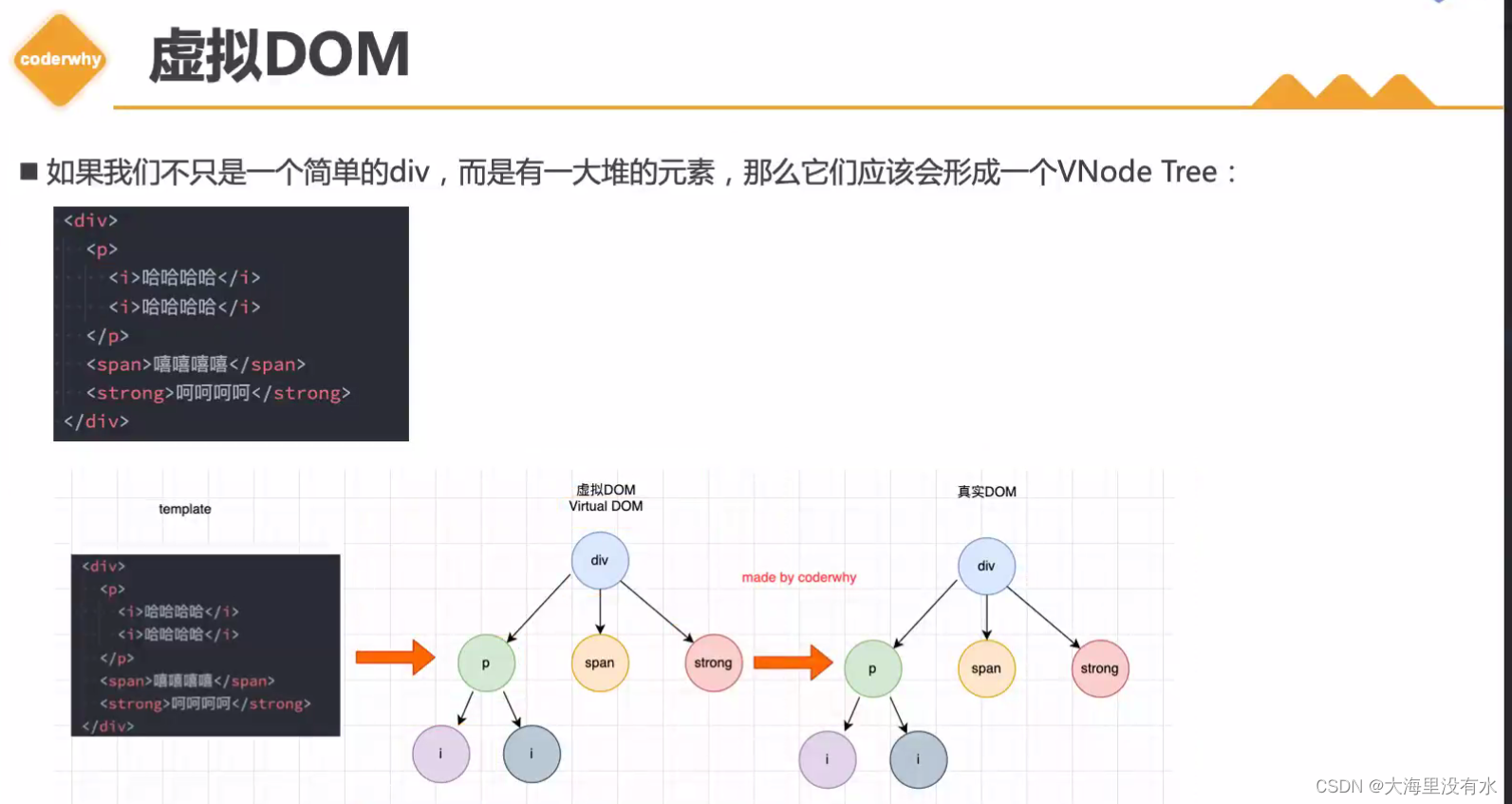 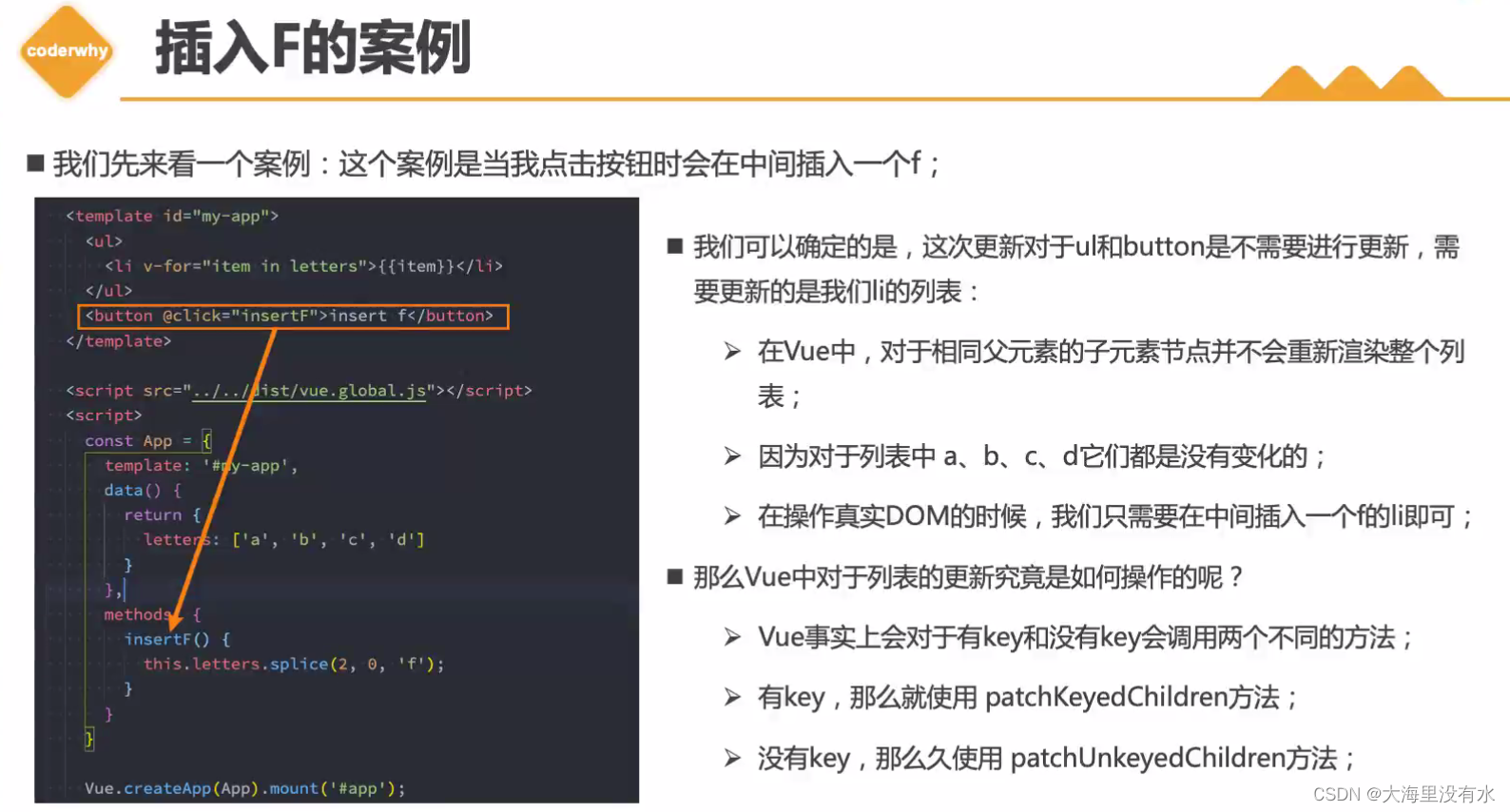
没有key的算法, 源码如下 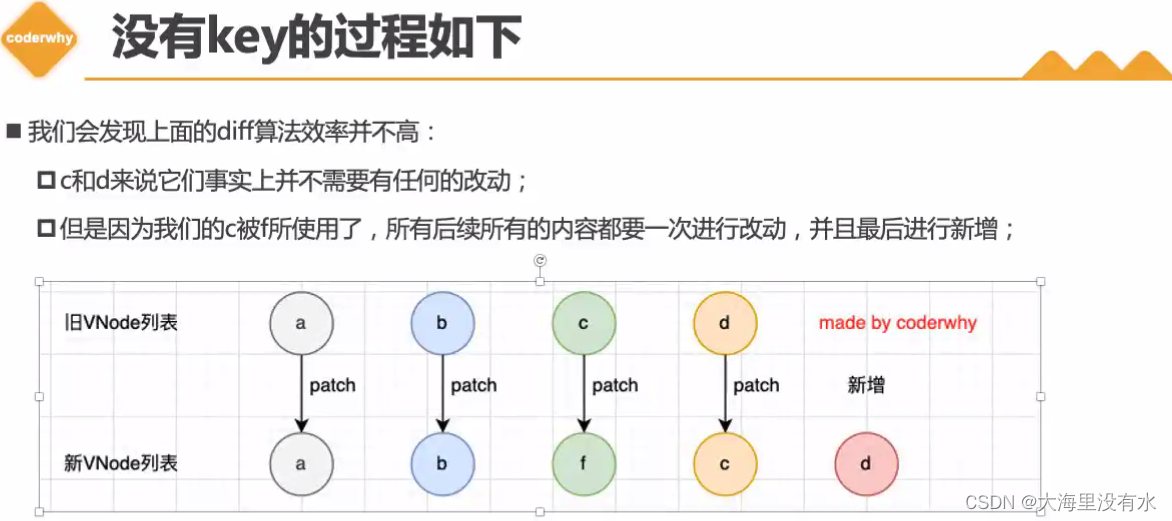
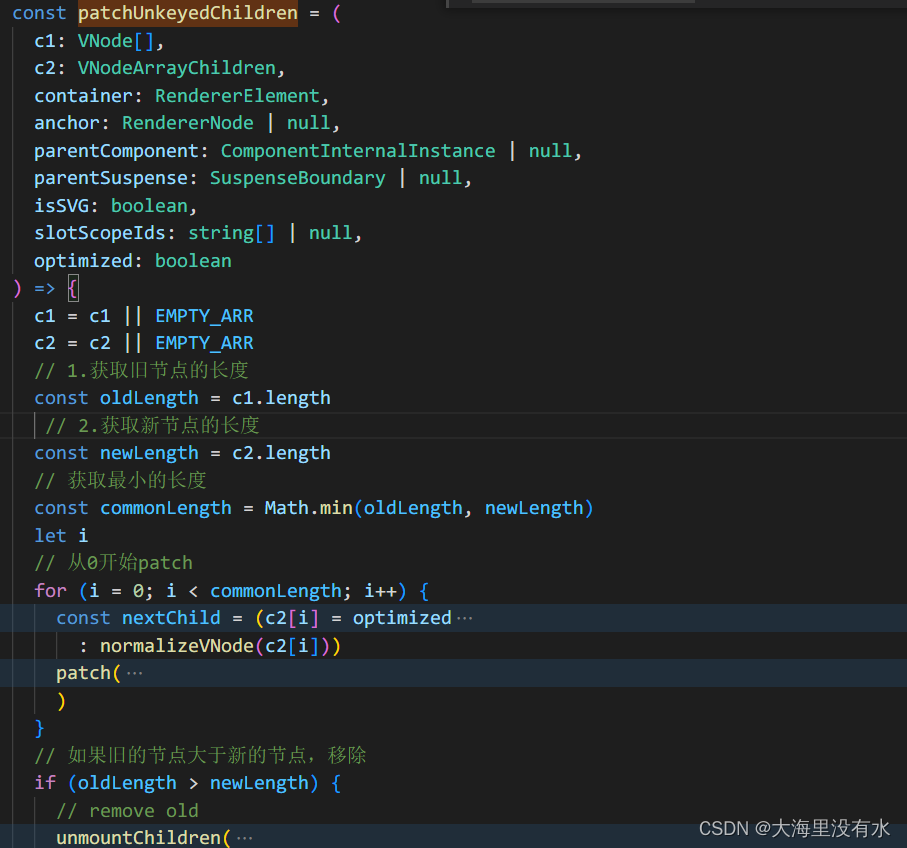
有key的算法, 源码如下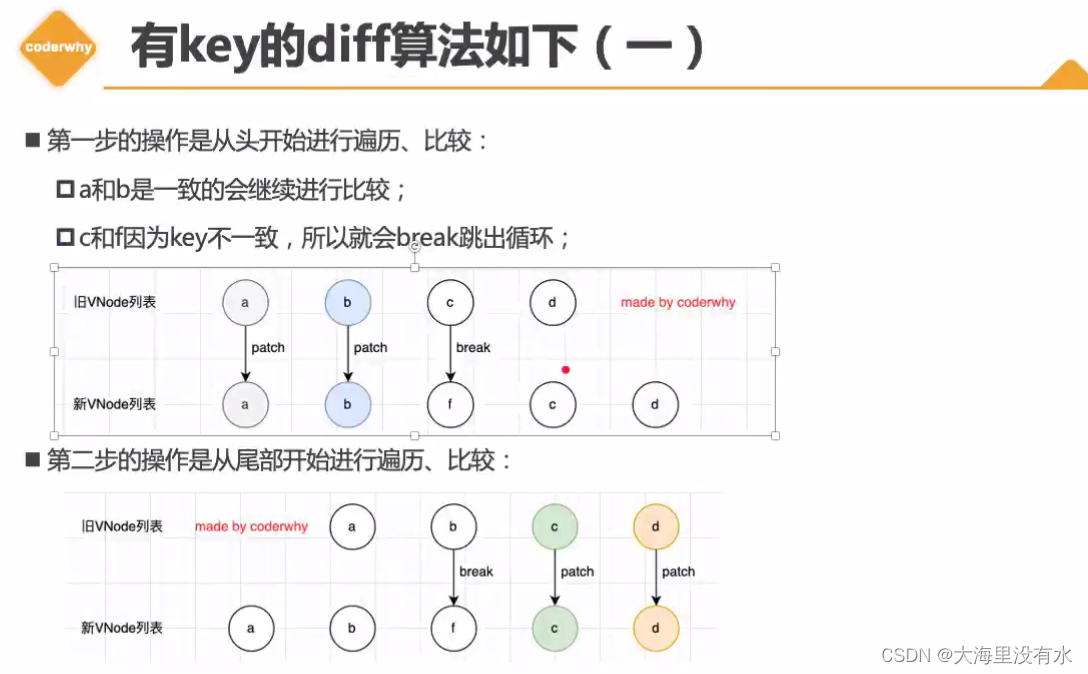 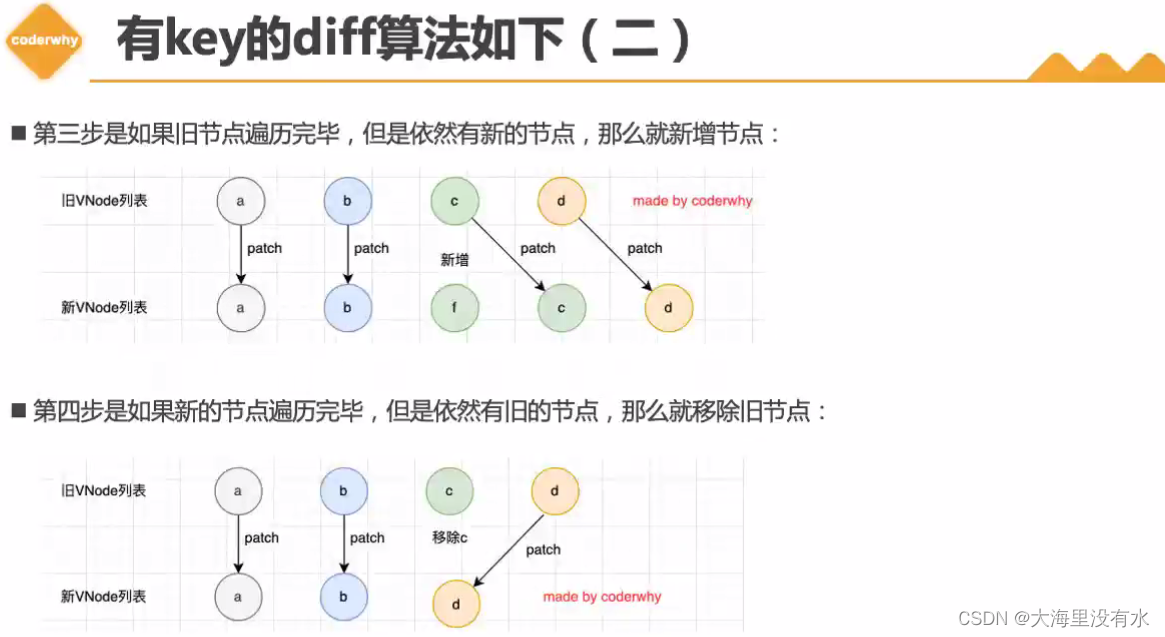 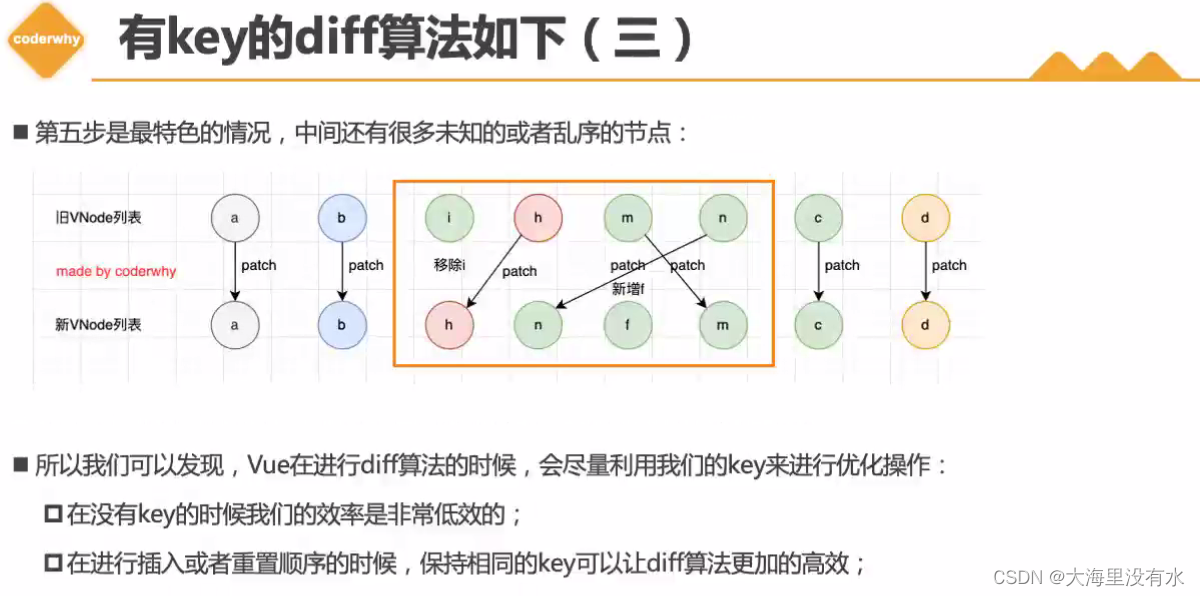
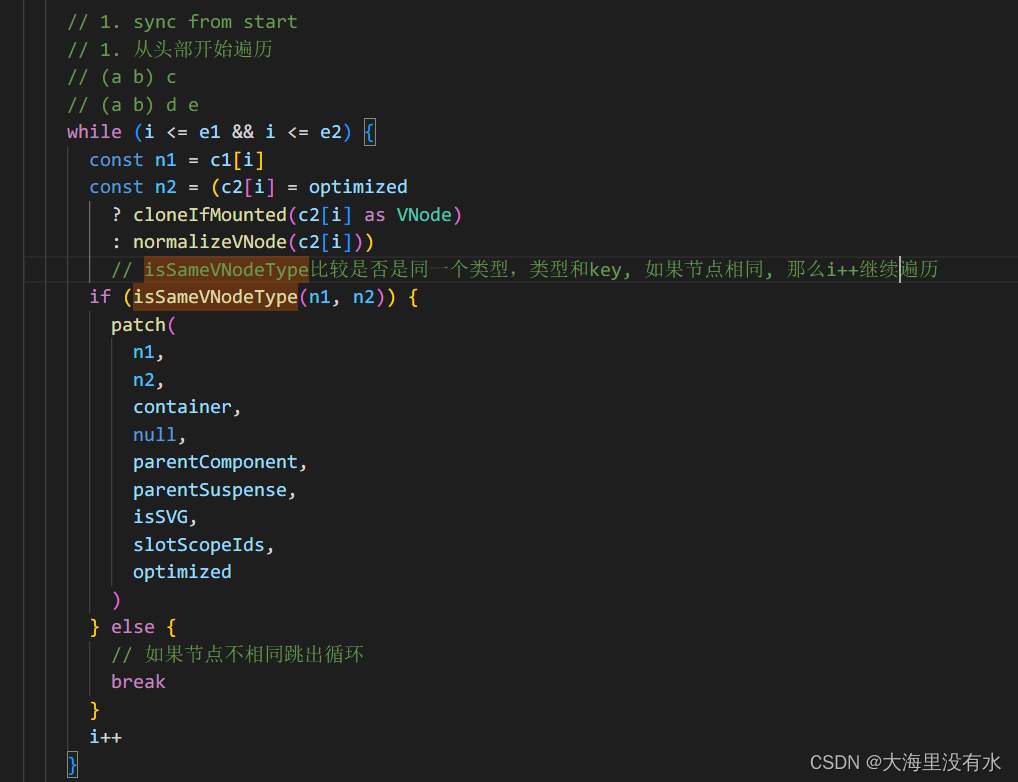 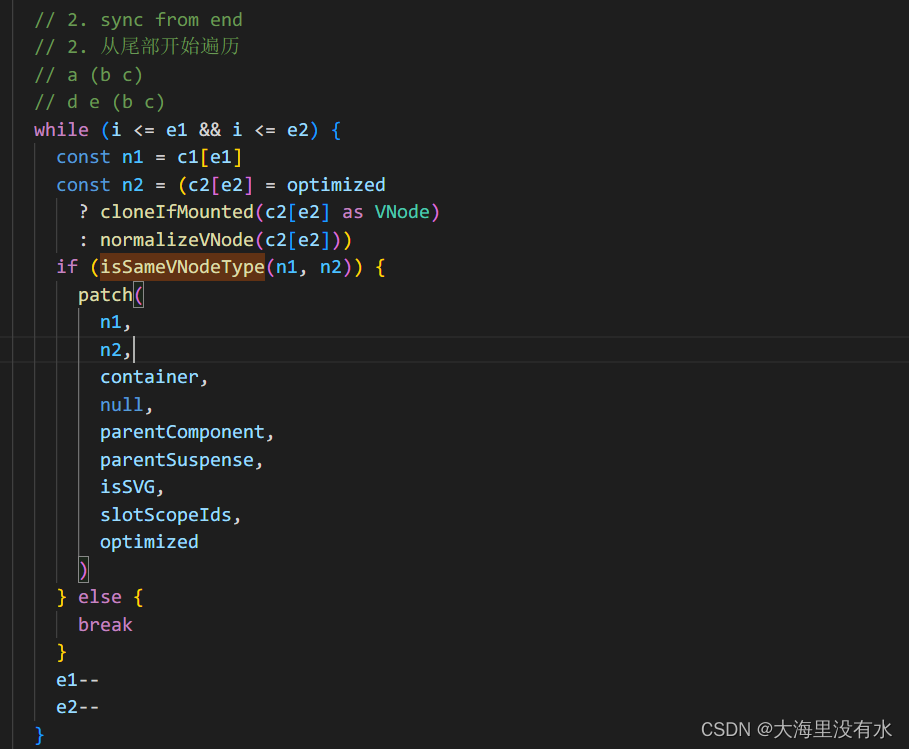
10、method 和 计算属性(对于任何包含响应式的数据)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<button @click="changeFirstName">修改按钮</button>
<h2>{{ fullName}}</h2>
<h2>{{ fullName}}</h2>
<h2>{{ fullName}}</h2>
<h2>{{ getFullName() }}</h2>
<h2>{{ getFullName() }}</h2>
<h2>{{ getFullName() }}</h2>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
firstName: "chen",
lastName: "ping",
};
},
computed: {
fullName() {
console.log("computed");
return this.firstName + " " + this.lastName;
},
},
methods: {
getFullName() {
console.log("methods");
return this.firstName + " " + this.lastName;
},
changeFirstName() {
this.firstName = "madongmei";
},
},
}).mount("#app");
</script>
</body>
</html>
10.1、计算属性的setter/getter
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<button @click="changeFullName">修改按钮</button>
<h2>{{ fullname }}</h2>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
firstName: "chen",
lastName: "ping",
};
},
computed: {
fullname: {
get: function () {
return this.firstName + " " + this.lastName;
},
set: function (newValue) {
const names = newValue.split(" ");
this.firstName = names[0];
this.lastName = names[1];
console.log("设置计算属性的值", newValue);
},
},
},
methods: {
changeFullName() {
this.fullname = "chen bairen";
},
},
}).mount("#app");
</script>
</body>
</html>
源码computed 对传入函数 和 对象两种情况的判断 :NOOP是空的函数实现 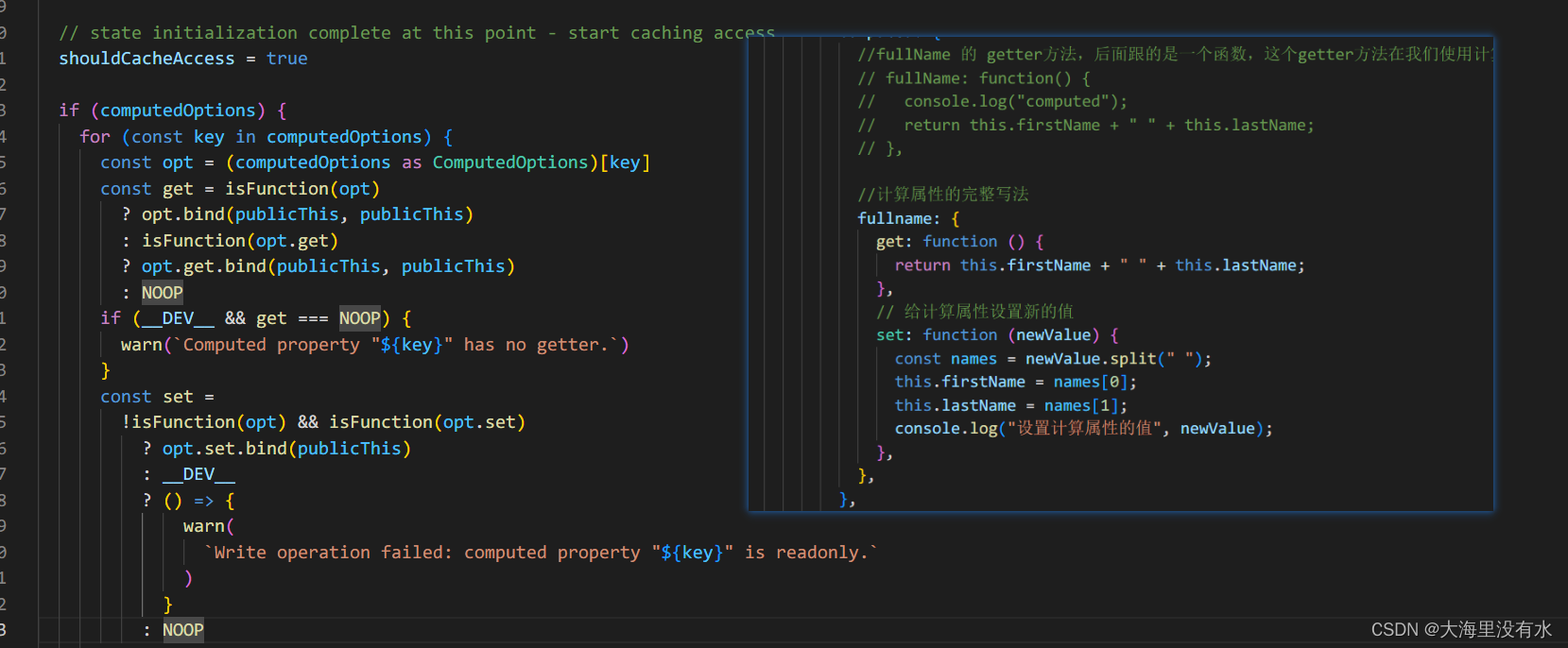
11、侦听器watch
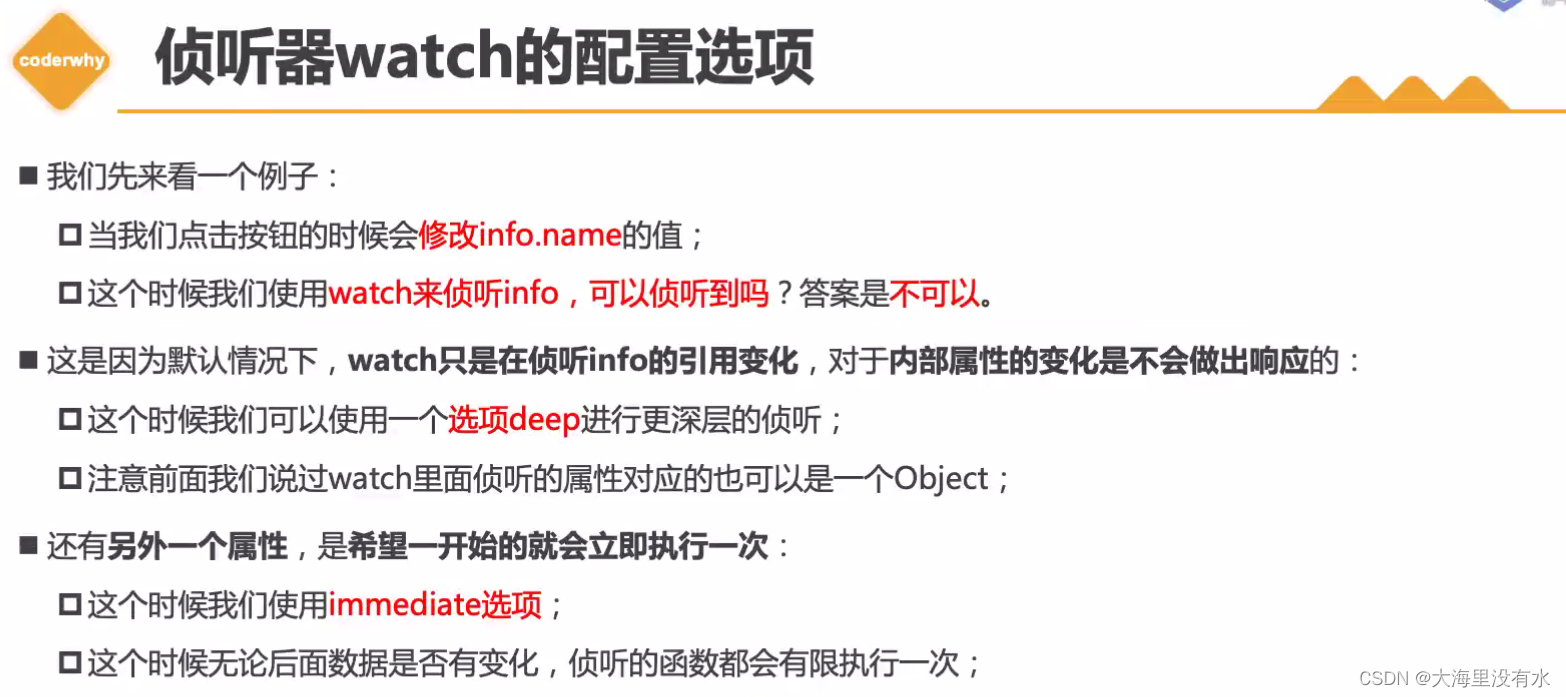
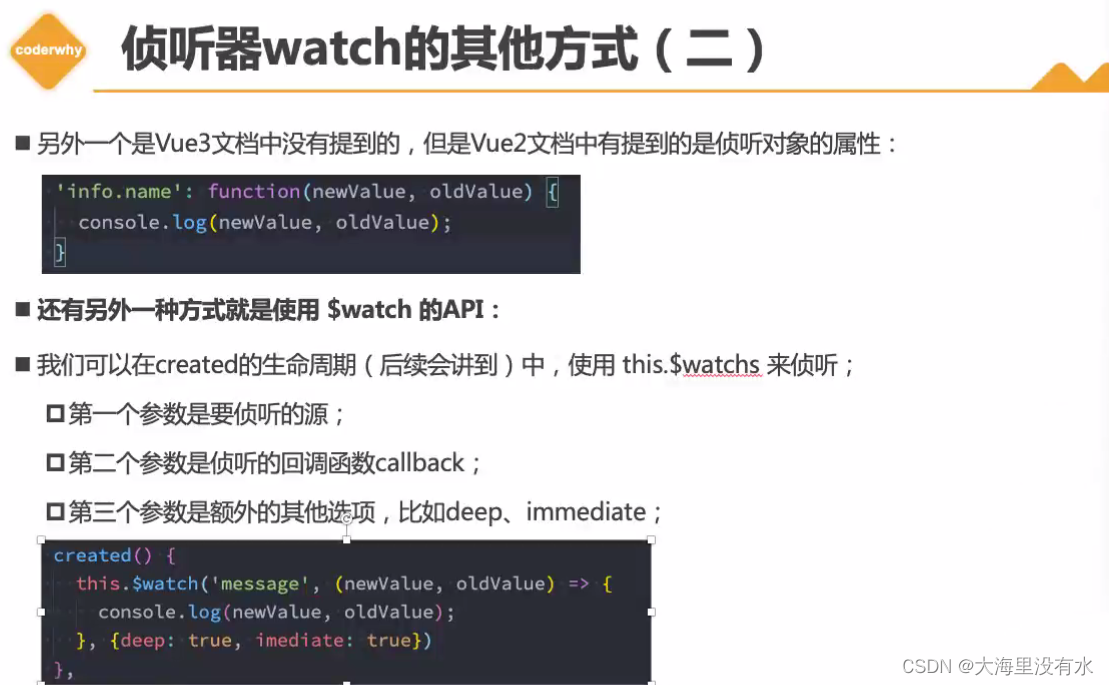
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<h2>{{ info.name}}</h2>
<button @click="changeInfo">改变info</button>
<button @click="changeInfoName">改变info中的name</button>
<button @click="changeInfoNbaName">改变info.nba.中的name</button>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data() {
return {
info: { name: "chen", age: 13, nba: { name: "xiao" } },
};
},
watch: {
"info.name": function (newValue, oldValue) {
console.log("newValue, oldValue", newValue, oldValue);
},
},
methods: {
changeInfo() {
this.info = { name: "ping" };
},
changeInfoName() {
this.info.name = "hahha";
},
changeInfoNbaName() {
this.info.nba.name = "辰";
},
},
created() {
const unwatch = this.$watch(
"info.name",
function (newValue, oldValue) {
console.log("------", newValue, oldValue);
},
{
deep: true,
immediate: true,
}
);
},
}).mount("#app");
</script>
</body>
</html>
12、v-model
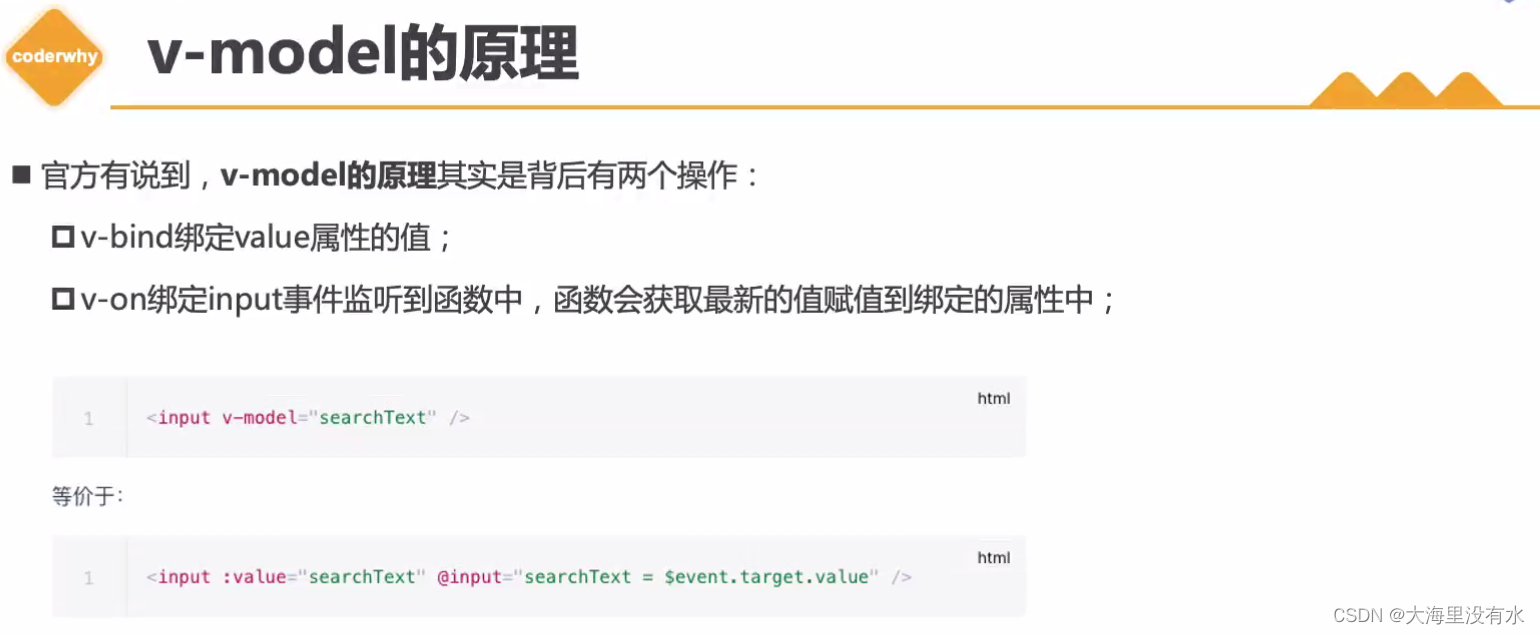
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<input type="text" :value="message" @input="inputChange" />
<input
type="text"
:value="message"
@input="message = $event.target.value"
/>
<input type="text" v-model="message" />
<h2>{{message}}</h2>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
message: "hell world",
};
},
methods: {
inputChange(event) {
this.message = event.target.value;
},
},
}).mount("#app");
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<label for="intro">
自我介绍
<textarea
name="intro"
id="intro"
cols="30"
rows="10"
v-model="intro"
></textarea>
</label>
<h2>intro: {{ intro }}</h2>
<label for="agree">
<input id="agree" type="checkbox" v-model="isAgree" />同意协议
<h2>isAgree: {{isAgree}}</h2>
</label>
<span>你的爱好: </span>
<label for="basketball">
<input
id="basketball"
type="checkbox"
v-model="hobbies"
value="basketball"
/>篮球
</label>
<label for="football">
<input
id="football"
type="checkbox"
v-model="hobbies"
value="football"
/>足球
</label>
<label for="tennis">
<input
id="tennis"
type="checkbox"
v-model="hobbies"
value="tennis"
/>网球
</label>
<h2>hobbies: {{hobbies}}</h2>
<span>你的性别: </span>
<label for="male">
<input id="male" type="radio" v-model="gender" value="male" />男
</label>
<label for="female">
<input id="female" type="radio" v-model="gender" value="female" />女
</label>
<h2>gender: {{gender}}</h2>
<span>喜欢的水果:</span>
<select v-model="fruit" multiple size="2">
<option v-for="item of fruits" :value="item">{{item.value}}</option>
<option value="apple">苹果</option>
<option value="orange">橘子</option>
<option value="banana">香蕉</option>
</select>
<h2>fruit: {{fruit}}</h2>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
intro: "hello world",
isAgree: false,
hobbies: [],
gender: "",
fruit: "orange",
};
},
methods: {},
}).mount("#app");
</script>
</body>
</html>
12.1 v-model修饰符
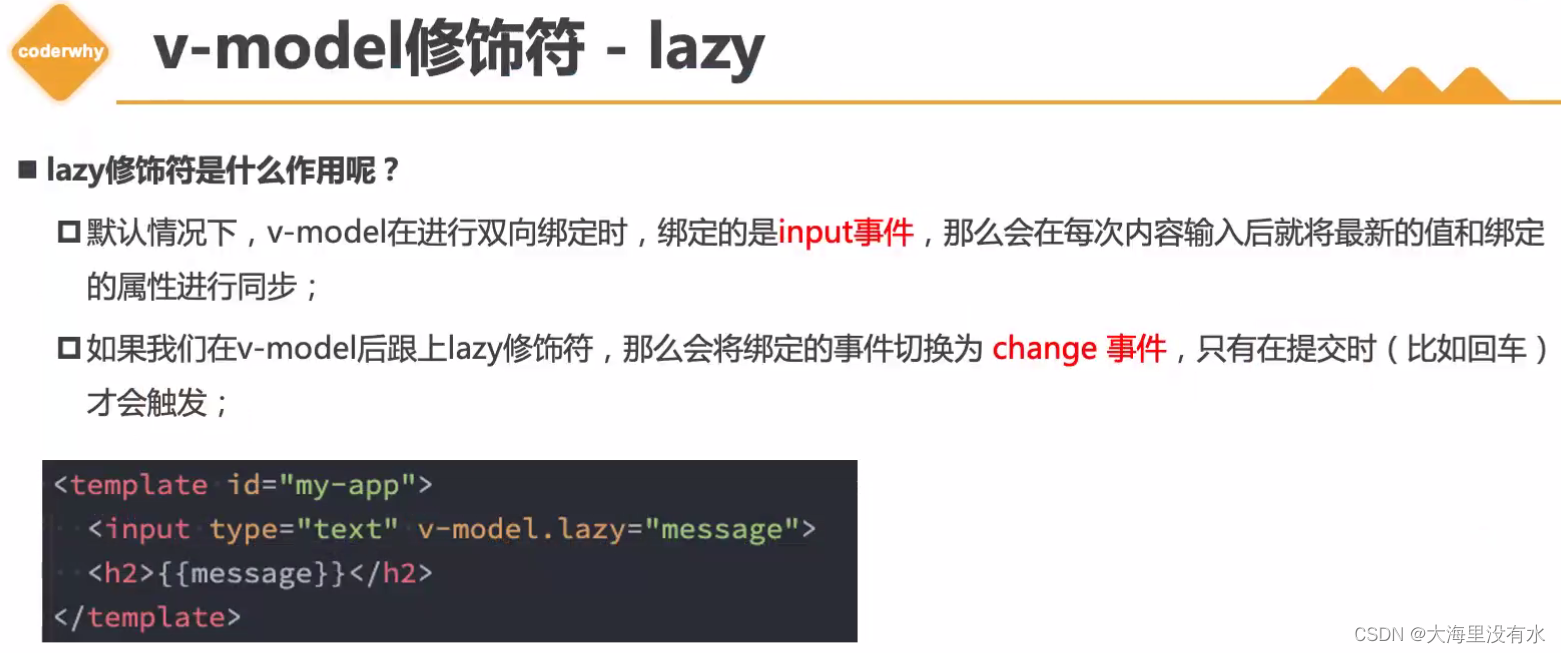
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<input type="text" v-model.trim="message" />
<h2>{{message}}</h2>
<button @click="getType">查看数据类型</button>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
Vue.createApp({
template: "#chen",
data: function () {
return {
message: 1000,
};
},
methods: {
getType() {
console.log(this.message, typeof this.message);
},
},
}).mount("#app");
</script>
</body>
</html>
二、vue组件
1、组件树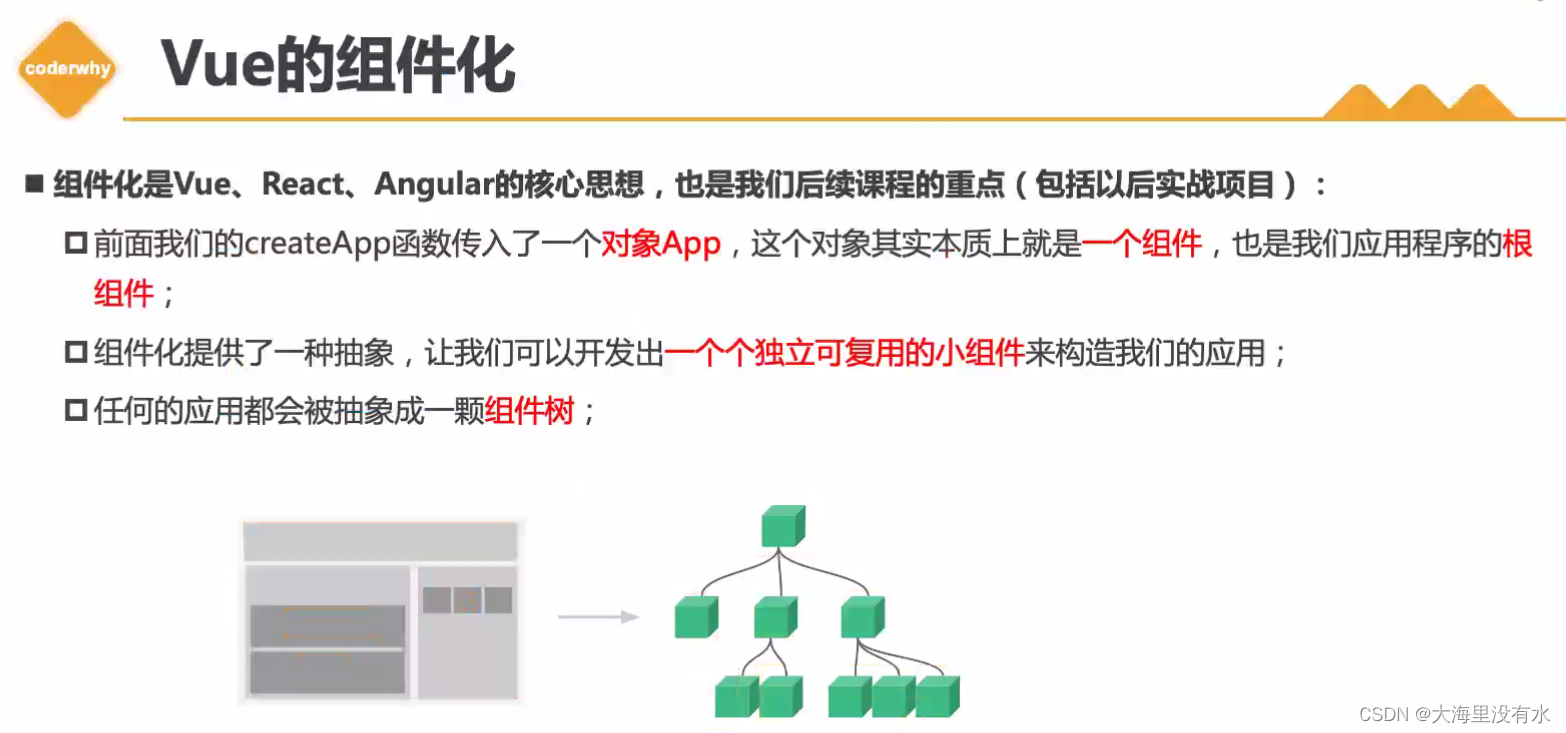
2、注册局部组件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<div id="app"></div>
<template id="chen">
<h2>{{message}}</h2>
<component-a></component-a>
</template>
<template id="component-a">
<h2>我是组件a</h2>
<p>我是内容</p>
</template>
<script src="https://unpkg.com/vue@3"></script>
<script>
const ComponentA = {
template: "#component-a",
};
const App = {
template: "#chen",
components: {
ComponentA: ComponentA,
},
data() {
return {
message: "hello world",
};
},
};
const app = Vue.createApp(App);
app.mount("#app");
</script>
</body>
</html>
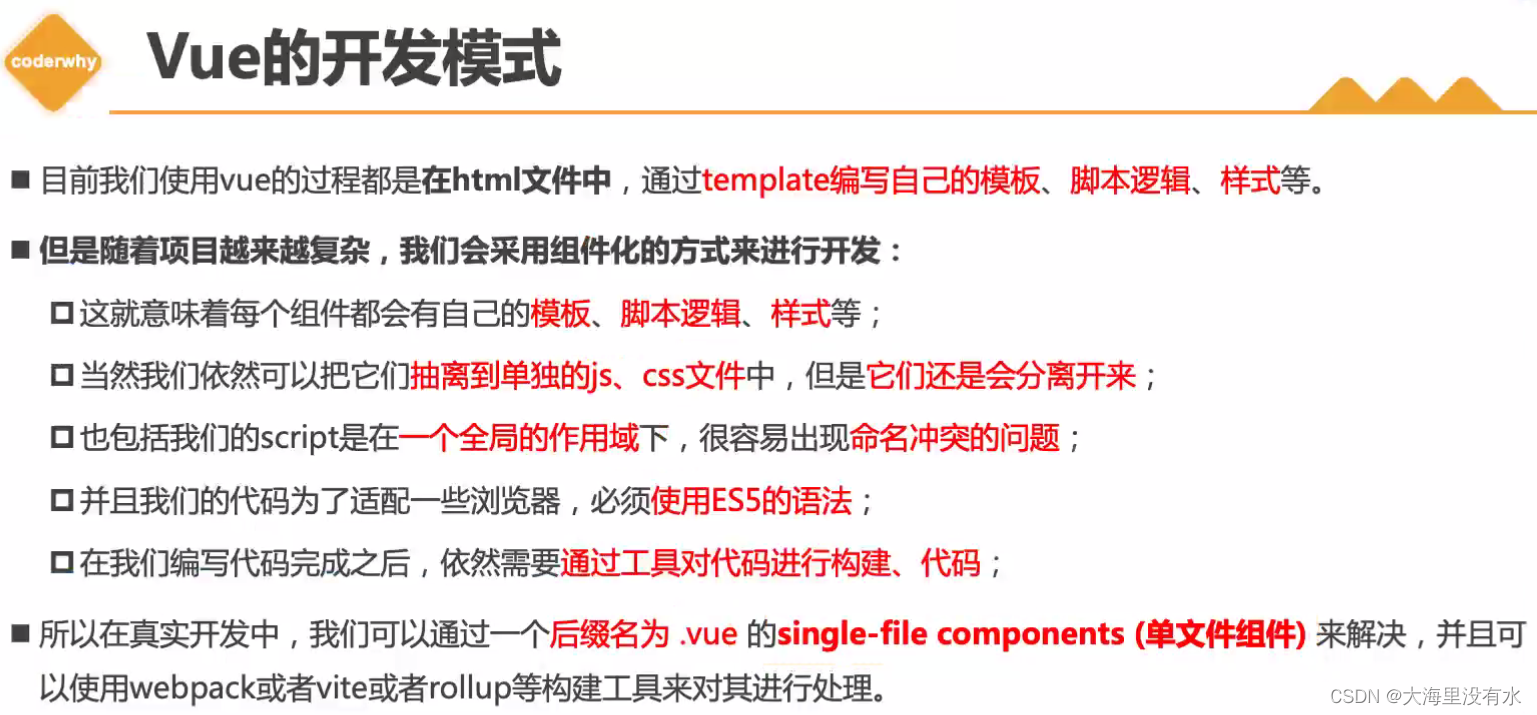 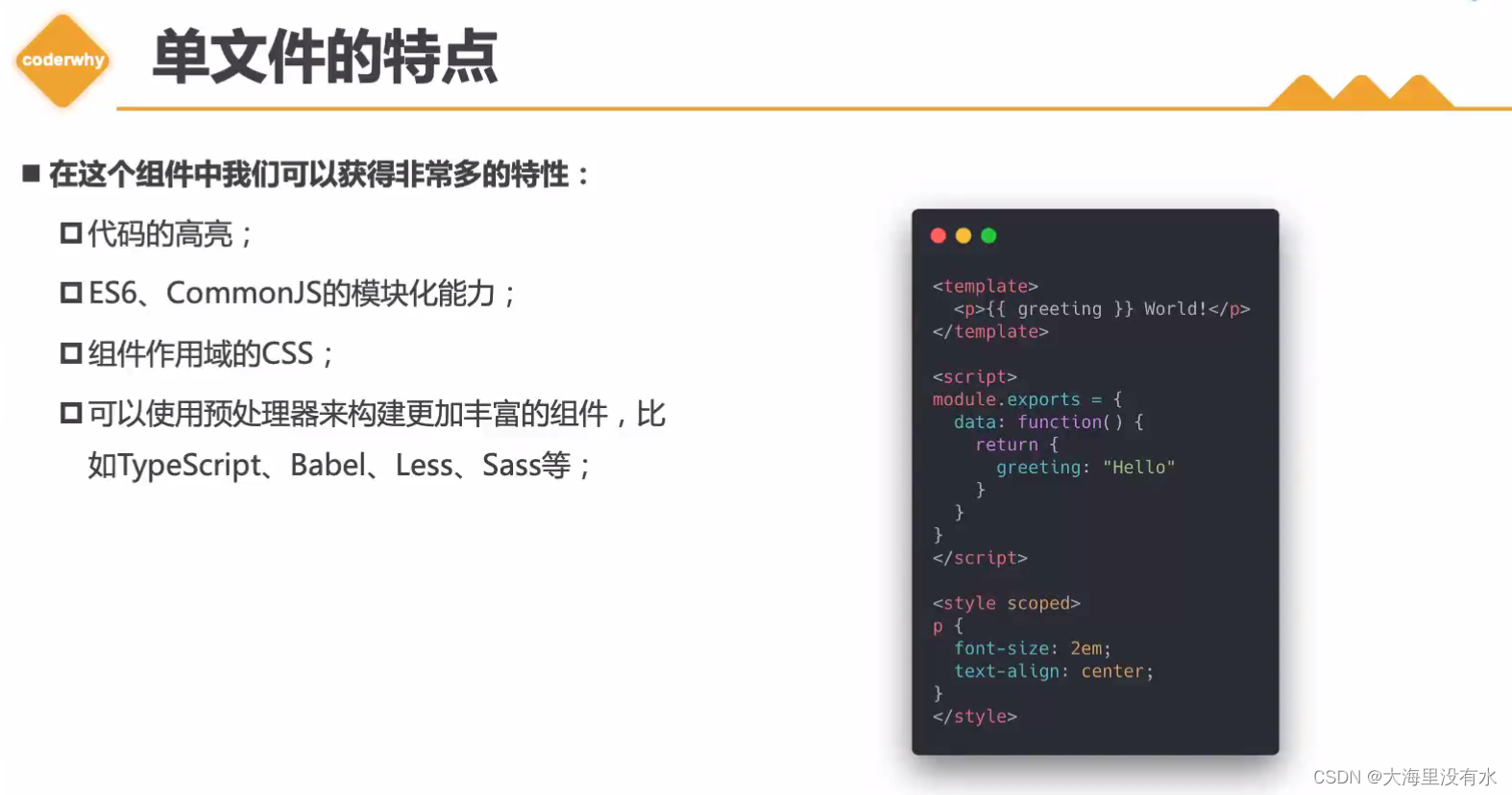
|