? 今天学完了JavaScript基础,打算做个网页小游戏练练手,想来想去,想起了小时候那种游戏机里的赛车游戏,于是做了一个玩玩,效果如下:
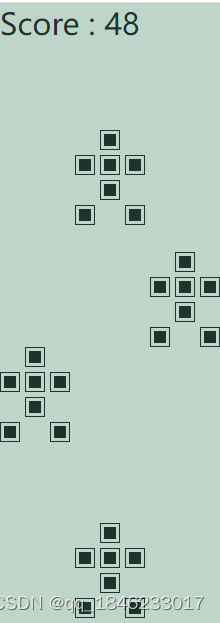
通过操纵最底下的小车来回躲避上方随机出来的三个障碍车辆从而使游戏进行,是不是找到了小时候的味道?
? 那么是怎么做的呢? 首先定义这几个小车共同的模型框架(从上图也能看见 a~g代表每个小车内部的7个方块):
<div class="car">
<div class="a">
<div class="black-block"></div>
</div>
<div class="b">
<div class="black-block"></div>
</div>
<div class="c">
<div class="black-block"></div>
</div>
<div class="d">
<div class="black-block"></div>
</div>
<div class="e">
<div class="black-block"></div>
</div>
<div class="f">
<div class="black-block"></div>
</div>
<div class="g">
<div class="black-block"></div>
</div>
</div>
然后通过绝对定位设定障碍车辆和玩家控制小车的初始位置.
接下来,通过move()函数让障碍小车向下移动:
function move(obj, attr, target, speed, callback) {
clearInterval(obj.timer);
var newValue;
obj.timer = setInterval(function () {
if (parseInt(getStyle(obj, attr)) < target) {
obj.style[attr] = parseInt(getStyle(obj, attr)) + speed + "px";
newValue = parseInt(obj.style[attr]);
if (newValue > target) {
newValue = target;
}
if (newValue == target) {
obj.style[attr] = newValue + "px";
clearInterval(obj.timer);
callback && callback();
}
}
else if (parseInt(getStyle(obj, attr)) > target) {
obj.style[attr] = parseInt(getStyle(obj, attr)) - speed + "px";
newValue = parseInt(obj.style[attr]);
if (newValue < target) {
newValue = target;
}
if (newValue == target) {
obj.style[attr] = newValue + "px";
clearInterval(obj.timer);
callback && callback();
}
}
}, 20);
}
function getStyle(obj, attr) {
return getComputedStyle(obj, null)[attr];
}
并通过碰撞检测函数实时监测己方小车是否与对面车辆相碰:
function collide(obj1, obj2) {
if (!(obj1.offsetTop + obj1.offsetHeight <= obj2.offsetTop
|| obj1.offsetLeft + obj1.offsetWidth <= obj2.offsetLeft
|| obj1.offsetLeft >= obj2.offsetLeft + obj2.offsetWidth
|| obj1.offsetTop >= obj2.offsetTop + obj2.offsetHeight)) {
alert("碰上了");
oppsiteCar1.style.top = "190px"
oppsiteCar2.style.top = "-40px"
oppsiteCar3.style.top = "95px"
speed = 2;
scores = 0;
}
}
以上就是主要的部分了,当然最重要的是,如何确实障碍小车刷新之后的间距?这点,我用了一个方法:
setInterval(function () {
if (oppsiteCar1.offsetTop != 560) {
move(oppsiteCar1, "top", 560, speed);
} else {
oppsiteCar1.style.top = parseInt(oppsiteCar2.style.top) - parseInt(Math.random() * 300 + 300) + "px";
}
}, 30);
思路就是:车辆1(图片上方左一)开始游戏的时候是在车辆2的前面先通过游戏整体的下边框,所以就让车辆1重置时候的位置通过算法保证车辆1和2之间的间距能有一个车的距离就行.
好了,以上就是游戏整体思路的介绍.
整体代码如下:
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Racing game</title>
<style>
* {
margin: 0;
padding: 0;
}
#container {
width: 220px;
height: 560px;
background-color: #bed6ca;
position: relative;
overflow: hidden;
margin-top: 60px;
}
.car {
width: 70px;
height: 95px;
position: absolute;
}
.car>div {
width: 20px;
height: 20px;
background-color: #bed6ca;
box-sizing: border-box;
border: 1px solid #1c342c;
position: absolute;
}
.black-block {
width: 12px;
height: 12px;
background-color: #1c342c;
margin-top: 3px;
margin-left: 3px;
}
.a {
left: 25px;
}
.b {
top: 25px;
}
.c {
top: 25px;
left: 25px;
}
.d {
top: 25px;
left: 50px;
}
.e {
top: 50px;
left: 25px;
}
.f {
top: 75px;
}
.g {
top: 75px;
left: 50px;
}
.car:first-child {
top: 460px;
left: 75px;
}
.car:nth-child(2) {
left: 0px;
top: 190px;
}
.car:nth-child(3) {
left: 75px;
top: -40px;
}
.car:nth-child(4) {
left: 150px;
top: 95px;
}
#score {
background-color: #bed6ca;
width: 220px;
height: 60px;
font-size: 30px;
color: #1c342c;
position: absolute;
top: 0;
}
</style>
<script>
window.onload = function () {
var myCar = document.querySelectorAll(".car")[0];
var oppsiteCar1 = document.querySelectorAll(".car")[1];
var oppsiteCar2 = document.querySelectorAll(".car")[2];
var oppsiteCar3 = document.querySelectorAll(".car")[3];
var speed = 2;
var scoreBlock = document.getElementById("score");
var scores = 0;
var dir;
document.onkeydown = function (event) {
dir = event.keyCode;
if (dir == 37 && myCar.offsetLeft) {
myCar.style.left = myCar.offsetLeft - 75 + "px";
}
if (dir == 39 && myCar.offsetLeft != 150) {
myCar.style.left = myCar.offsetLeft + 75 + "px";
}
}
setInterval(function () {
speed += 0.001;
}, 20)
setInterval(function () {
collide(myCar, oppsiteCar3);
collide(myCar, oppsiteCar2);
collide(myCar, oppsiteCar1);
scores++;
scoreBlock.innerHTML = "Score : " + scores;
}, 30)
setInterval(function () {
if (oppsiteCar1.offsetTop != 560) {
move(oppsiteCar1, "top", 560, speed);
} else {
oppsiteCar1.style.top = parseInt(oppsiteCar2.style.top) - parseInt(Math.random() * 300 + 300) + "px";
}
}, 30);
setInterval(function () {
if (oppsiteCar2.offsetTop != 560) {
move(oppsiteCar2, "top", 560, speed);
} else {
oppsiteCar2.style.top = parseInt(oppsiteCar1.style.top) - parseInt(Math.random() * 300 + 300) + "px";
}
}, 30);
setInterval(function () {
if (oppsiteCar3.offsetTop != 560) {
move(oppsiteCar3, "top", 560, speed);
} else {
oppsiteCar3.style.top = -Math.random() * 400 + "px";
}
}, 30);
function collide(obj1, obj2) {
if (!(obj1.offsetTop + obj1.offsetHeight <= obj2.offsetTop
|| obj1.offsetLeft + obj1.offsetWidth <= obj2.offsetLeft
|| obj1.offsetLeft >= obj2.offsetLeft + obj2.offsetWidth
|| obj1.offsetTop >= obj2.offsetTop + obj2.offsetHeight)) {
alert("碰上了");
oppsiteCar1.style.top = "190px"
oppsiteCar2.style.top = "-40px"
oppsiteCar3.style.top = "95px"
speed = 2;
scores = 0;
}
}
function move(obj, attr, target, speed, callback) {
clearInterval(obj.timer);
var newValue;
obj.timer = setInterval(function () {
if (parseInt(getStyle(obj, attr)) < target) {
obj.style[attr] = parseInt(getStyle(obj, attr)) + speed + "px";
newValue = parseInt(obj.style[attr]);
if (newValue > target) {
newValue = target;
}
if (newValue == target) {
obj.style[attr] = newValue + "px";
clearInterval(obj.timer);
callback && callback();
}
}
else if (parseInt(getStyle(obj, attr)) > target) {
obj.style[attr] = parseInt(getStyle(obj, attr)) - speed + "px";
newValue = parseInt(obj.style[attr]);
if (newValue < target) {
newValue = target;
}
if (newValue == target) {
obj.style[attr] = newValue + "px";
clearInterval(obj.timer);
callback && callback();
}
}
}, 20);
}
function getStyle(obj, attr) {
return getComputedStyle(obj, null)[attr];
}
}
</script>
</head>
<body>
<div id="container">
<div class="car">
<div class="a">
<div class="black-block"></div>
</div>
<div class="b">
<div class="black-block"></div>
</div>
<div class="c">
<div class="black-block"></div>
</div>
<div class="d">
<div class="black-block"></div>
</div>
<div class="e">
<div class="black-block"></div>
</div>
<div class="f">
<div class="black-block"></div>
</div>
<div class="g">
<div class="black-block"></div>
</div>
</div>
<div class="car">
<div class="a">
<div class="black-block"></div>
</div>
<div class="b">
<div class="black-block"></div>
</div>
<div class="c">
<div class="black-block"></div>
</div>
<div class="d">
<div class="black-block"></div>
</div>
<div class="e">
<div class="black-block"></div>
</div>
<div class="f">
<div class="black-block"></div>
</div>
<div class="g">
<div class="black-block"></div>
</div>
</div>
<div class="car">
<div class="a">
<div class="black-block"></div>
</div>
<div class="b">
<div class="black-block"></div>
</div>
<div class="c">
<div class="black-block"></div>
</div>
<div class="d">
<div class="black-block"></div>
</div>
<div class="e">
<div class="black-block"></div>
</div>
<div class="f">
<div class="black-block"></div>
</div>
<div class="g">
<div class="black-block"></div>
</div>
</div>
<div class="car">
<div class="a">
<div class="black-block"></div>
</div>
<div class="b">
<div class="black-block"></div>
</div>
<div class="c">
<div class="black-block"></div>
</div>
<div class="d">
<div class="black-block"></div>
</div>
<div class="e">
<div class="black-block"></div>
</div>
<div class="f">
<div class="black-block"></div>
</div>
<div class="g">
<div class="black-block"></div>
</div>
</div>
</div>
<div id="score">Score : 0</div>
</body>
</html>
|