看完文章会收获满满哦!
?一、类的定义和声明
class Person {} // 类的声明
const Girl = class {} // 类的表达式
?二、类中的方法
1.构造器方法
?一个类只能有一个构造函数 ?通过new关键字操作类时会调用constructor函数,如果不写也会调用默认的constructor
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
const p1 = new Person('wyy',22)
console.log(p1)
2.实例方法--通过创建的实例进行访问
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
// 实例方法
eating() {
console.log(this.name, "eating");
}
}
const p1 = new Person('wyy',22)
p1.eating() // wyy eating
3.访问器方法
?可对类的属性操作进行访问和响应拦截
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
this._sex = "boy";
}
// 类的访问器方法
get sex() {
console.log("拦截访问操作");
return this._sex;
}
set sex(newVal) {
console.log("拦截设置操作");
this._sex = newVal;
}
}
const p1 = new Person('wyy',22)
p1.sex = '男'
console.log(p1.sex)
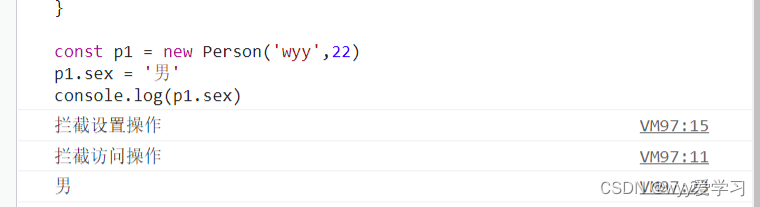
?4.静态方法-通过类名访问
如Promise.all()
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
this._sex = "boy";
}
// 静态方法
static creatP() {
console.log("访问了类的静态方法");
}
}
Person.creatP();
三、类的继承
类的继承使用extends关键字
class Boy {}
class Student extends Boy {}
super:调用父类的构造器函数
注:在子类的构造函数中在使用this之前必须调用父类的构造方法(super)
? ? ? ? 使用位置:子类(派生类)的构造函数,实例方法,静态方法
1.继承父类的属性?
// 类的继承
class Boy {
constructor(name, age) {
this.name = name;
this.age = age;
}
}
class Student extends Boy {
constructor(name, age, sno) {
super(name, age); //调用父类的构造函数
this.sno = sno;
}
}
const s1 = new Student("hhh", 18, "2339929");
console.log(s1);
2.继承父类的方法
class Boy {
constructor(name, age) {
this.name = name;
this.age = age;
}
say() {
console.log(this.name, "say");
}
}
class Student extends Boy {
constructor(name, age, sno) {
super(name, age); //调用父类的构造函数
this.sno = sno;
}
//定义子类内部自己的方法
study() {
console.log(this.name, "study");
}
}
const s1 = new Student("hhh", 18, "2339929");
s1.say(); //方法的继承
3.对父类方法进行重写
如果对父类的方法不满意时,可根据需求进行重写
3.1 完全重写
class Boy {
constructor(name, age) {
this.name = name;
this.age = age;
}
say() {
console.log(this.name, "say");
}
}
class Student extends Boy {
constructor(name, age, sno) {
super(name, age); //调用父类的构造函数
this.sno = sno;
}
say() {
console.log(this.name, "子类重写say");
}
}
const s1 = new Student("hhh", 18, "2339929");
s1.say();
3.2 部分重写-通过super关键字实现
class Boy {
constructor(name, age) {
this.name = name;
this.age = age;
}
say() {
console.log(this.name, "say1");
console.log(this.name, "say2");
console.log(this.name, "say3");
}
}
class Student extends Boy {
constructor(name, age, sno) {
super(name, age); //调用父类的构造函数
this.sno = sno;
}
say() {
super.say()
console.log(this.name, "子类重写say1");
console.log(this.name, "say2");
}
}
const s1 = new Student("hhh", 18, "2339929");
s1.say();
3.3 对静态方法进行重写
class Boy {
static sum() {
console.log("static静态方法");
}
}
class Student extends Boy {
// 重写静态方法
static sum() {
super.sum();
console.log("子类的静态方法");
}
}
Student.sum();
|