绑定自定义事件
引例:子组件给父组件传值 -首先我们可以通过props来传递, 它的实现原理是父亲给儿子一个函数,儿子通过调用这个函数进行数据传递。 ex: 子组件
<template>
<div>
<button @click="sendName">子组件的点击按钮,点击将数据传递给父组件</button>
</div>
</template>
<script>
export default {
data() {
return {
name: "son",
sex: "nan",
};
},
mounted() {},
props: ["getName"],
methods: {
sendName() {
this.getName(this.name);
},
},
};
</script>
<style lang='less' scoped>
</style>
父组件
<template>
<div>
<div id="test">
<p>我是一个父组件</p>
我收到的儿子的名字是:{{ sonName }}
</div>
<Son :getName="getName"></Son>
</div>
</template>
<script>
import Son from "@/components/custom/Son.vue";
export default {
data() {
return {
sonName: "",
};
},
components: { Son },
methods: {
getName(name) {
this.sonName = name;
},
},
};
</script>
<style lang='less' scoped>
#test {
width: 200px;
height: 200px;
background: red;
}
</style>
点击传值按钮: 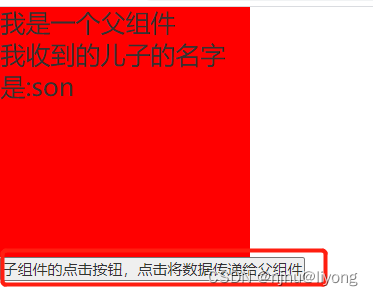
-自定义事件实现
它的原理是给组件实例对象绑定一个事件,让后在组件里面取触发,这样就可以给绑定的事件函数传递子组件的数据。 父组件
<template>
<div>
<div id="test">
<p>我是一个父组件</p>
我收到的儿子的名字是:{{ sonName }}
</div>
<Son @getName="getName"></Son>
</div>
</template>
<script>
import Son from "@/components/custom/Son.vue";
export default {
data() {
return {
sonName: "",
};
},
components: { Son },
methods: {
getName(name) {
this.sonName = name;
},
},
};
</script>
<style lang='less' scoped>
#test {
width: 200px;
height: 200px;
background: red;
font-size: 20px;
}
</style>
子组件
<template>
<div>
<button @click="sendName">子组件的点击按钮,点击将数据传递给父组件</button>
</div>
</template>
<script>
export default {
data() {
return {
name: "son",
sex: "nan",
};
},
mounted() {},
methods: {
sendName() {
this.$emit("getName", this.name);
},
},
};
</script>
<style lang='less' scoped>
</style>
运行的结果和上面通过props传递参数是同样的结果。
然后
<Son @getName="getName"></Son>
对于绑定事件我们还有一种更加灵活的写法:
<Son ref="son"></Son>
mounted() {
this.$refs.son.$on("getName", this.getName);
},
当然这里我们可以直接写回调函数
mounted() {
this.$refs.son.$on("getName", function() {}
);
},
这样我们可以在绑定自定义事件的时候添加一些其它的逻辑,比如定时操作,执行其它函数等。 如果我们只需要执行一次
<Son @getName.once="getName"></Son>
this.$refs.son.$on.$once("getName", this.getName);
如果我们需要传递多个参数:
getName(obj) {
},
getName(name, ...parms) {
},
解绑事件
this.$off("getName");
this.$off(["event1", "event2"]);
this.$off();
```
### 特别注意
组件在绑定原生事件的时候需要@click.native修饰,否则它会被当做自定义事件。
|