1.Ajax
1.1前后端分离
????????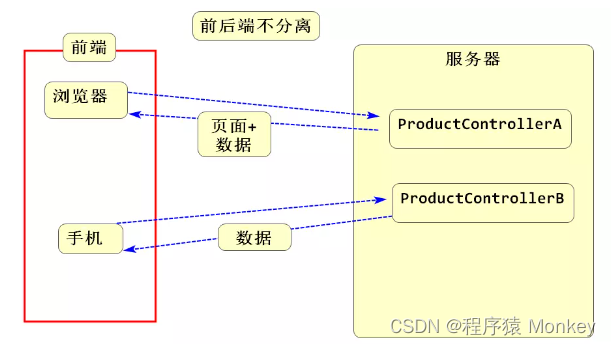
?
- 如果前后端不分离, 指的是在后端Controller代码中需要处理页面相关的代码, 这样的话手机端发请求时需要单独再创建一个Controller提供数据, 这样加大了后端程序员的工作量,需要准备两套处理相同业务的Controller
????????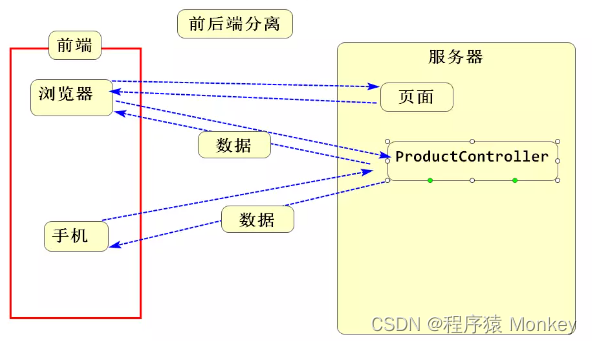
?
- 前后端分离后, 将页面相关代码从Controller中去掉, Controller只负责提供数据,这样不管前端是浏览器还是手机端都用相同的处理方式, 浏览器的请求流程变成先请求页面,页面显示后再发请求获取数据,得到数据后把数据显示到原页面中,这个过程属于页面局部刷新,所以只能使用异步请求来实现
- 由于以后工作中都是前后端分离的架构,所以以后发请求都是使用异步请求.?
1.2服务器给客户端传递复杂数据过程
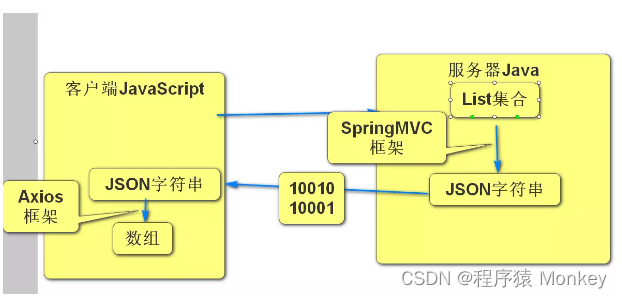
1.3JSON
- 轻量级的数据交换格式(数据封装格式)
- 当客户端和服务器之间进行数据传输时, 如果数据相对复杂,则需要按照一定的格式保存到字符串中,再进行传输, JSON就是一个通用的格式.
????????[{"id":1,"title":"小米手机","price":5000.0,"num":100},{"id":7,"title":"猫屎咖啡","price":100.0,"num":30},{"id":8,"title":"雀巢咖啡","price":20.0,"num":500},{"id":9,"title":"洗衣机","price":2000.0,"num":50},{"id":10,"title":"键盘","price":50.0,"num":1000}]
1.4商品管理系统
- 准备工作: 创建boot4工程 打3个√
- 在application.properties里面添加连接数据库的信息
- 创建index.html页面 里面添加两个超链接: 添加商品 和 商品列表,请求的地址分别为 /insert.html和 /select.html
- 添加商品步骤:
- 创建insert.html页面 , 通过vue管理页面, 三个文本框进行双向绑定, 给按钮添加点击事件, 点击后向/insert发出异步请求,请求成功后返回到首页
- 创建controller.ProductController,添加insert方法处理/insert请求, 创建Product实体类, 并且声明在参数列表处接收参数, 控制台输出接收到的参数 测试是否有数据,
- 创建ProductMapper在里面添加insert方法
- 在Controller里面把ProductMapper装配进来, 在insert方法中调用mapper的insert方法把product传递进去.
- 商品列表步骤:
- 创建select.html页面, 在页面中添加一个table表格 和data里面arr数组中的数据进行绑定,在vue里面添加created方法在方法中向/select发出异步请求 把请求回来得到的数据给到data里面的arr数组, 页面会自动发生改变
- 在Controller里面添加select方法处理/select请求,在里面调用mapper的select方法 返回值为List集合里面装着Product对象, 把得到的list集合直接return出去
- 实现mapper里面的select方法
- 删除商品步骤:
- 在select.html页面中的表格里面添加操作一列 ,里面添加删除超链接, 废掉超链接的跳转功能, 然后给超链接添加点击事件, 点击时把商品的id传递到方法里面, 在方法里面向/delete?id=xxx发出删除的异步请求
- 在ProductController里面添加delete方法处理/delete请求接收传递过来的id, 先测试id是否接收到了, 在方法中调用mapper的deleteById方法
- 实现mapper里面的deleteById方法
- 修改商品步骤:
- 在select.html页面中的表格里面操作一列里面 ,里面添加修改超链接,请求地址为/update.html?id=xxx
- 创建update.html页面,页面中四个文本框和vue里面的对象进行双向绑定, 添加created方法,在里面向/selectById?id=xxx发出请求, 把得到的数据赋值给vue里面的对象,页面会自动发生改变
- 在Controller里面添加selectById方法处理/selectById方法,接收传递过来的id,测试id是否接收到, 在方法中调用mapper的selectById方法返回值为Product对象, 把对象return出去
- 实现mapper里面的selectById方法
- 给update.html页面中的修改按钮添加点击事件, 点击时向/update发出异步请求,把vue里面的对象传递过去
- 在Controller里面添加update方法处理/update请求,方法中声明Product对象接收传递过来的商品信息, 调用mapper里面的update方法把接收到的product对象传递过去
- 实现mapper中的update方法.
1.4.1代码展示
1.4.1.1ProductController.java(商品接收响应页面)
package cn.tedu.boot03.collection;
import cn.tedu.boot03.entity.Product;
import cn.tedu.boot03.mapper.ProductMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class ProductController{
@Autowired
ProductMapper mapper;
@RequestMapping("/insert")
public void insert(@RequestBody Product product){
mapper.insert(product);
}
@RequestMapping("/select")
public List<Product> select(){
return mapper.select();
}
@RequestMapping("/delete")
public void delete(int id){
mapper.delete(id);
}
@RequestMapping("/selectById")
public Product selectById(int id){
return mapper.selectById(id);
}
@RequestMapping("/update")
public void update(@RequestBody Product product){
mapper.update(product);
}
}
1.4.1.2Product.java(商品实体类)
package cn.tedu.boot03.entity;
public class Product {
private Integer id;
private String title;
private Double price;
private Integer num;
@Override
public String toString() {
return "Product{" +
"id=" + id +
", title='" + title + '\'' +
", price=" + price +
", num=" + num +
'}';
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Double getPrice() {
return price;
}
public void setPrice(Double price) {
this.price = price;
}
public Integer getNum() {
return num;
}
public void setNum(Integer num) {
this.num = num;
}
}
1.4.1.3ProductMapper.java(商品Sql操作页面)
package cn.tedu.boot03.mapper;
import cn.tedu.boot03.entity.Product;
import org.apache.ibatis.annotations.*;
import java.util.List;
@Mapper
public interface ProductMapper {
@Insert("insert into product values(null,#{title},#{price},#{num})")
public void insert(Product product);
@Select("select * from product")
List<Product> select();
@Delete("delete from product where id = #{id}")
void delete(int id);
@Select("select * from product where id = #{id}")
Product selectById(int id);
@Update("update product set title = #{title},price = #{price} ,num = #{num} where id = #{id}")
void update(Product product);
}
1.4.1.4index.html(首页)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>商品管理系统</h1>
<a href="/insert.html">添加商品</a>
<a href="/select.html">商品展示</a>
</body>
</html>
1.4.1.5insert.html(商品添加页面)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<h1>添加商品</h1>
<input type="text" v-model="product.title" placeholder="商品标题">
<input type="text" v-model="product.price" placeholder="商品价格">
<input type="text" v-model="product.num" placeholder="商品库存">
<input type="button" value="添加" @click="insert()">
</div>
<script type="text/javascript" src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script type="text/javascript">
let v = new Vue({
el:"body>div",
data:{
product:{
title:"",price:"",num:""
}
},
methods:{
insert(){
axios.post("/insert",v.product).then(function () {
alert("添加成功");
location.href = "/";
})
}
}
})
</script>
</body>
</html>
1.4.1.6select.html(商品展示页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<table border="1">
<caption>商品信息</caption>
<tr>
<th>编号</th>
<th>标题</th>
<th>价格</th>
<th>库存</th>
<th>操作</th>
</tr>
<tr v-for="p in arr">
<td>{{p.id}}</td>
<td>{{p.title}}</td>
<td>{{p.price}}</td>
<td>{{p.num}}</td>
<td>
<a :href="'/update.html?id='+p.id">修改</a>
<a href="javascript:void(0)" @click="del(p.id)">删除</a>
</td>
</tr>
</table>
</div>
<script type="text/javascript" src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script type="text/javascript">
let v = new Vue({
el:"body>div",
data:{
arr:[
{id:1,title:"asd",price:233,num:34},
{id:2,title:"axcd",price:12233,num:314},
]
},
created:function (){
axios.post("/select").then(function (response) {
v.arr = response.data;
})
},
methods:{
del(id){
axios.get("/delete?id="+id).then(function () {
alert("删除成功");
location.reload()
})
}
}
})
</script>
</body>
</html>
1.4.1.7update.html(商品修改页面)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<h1>修改商品信息</h1>
<input type="text" v-model="product.id" readonly>
<input type="text" v-model="product.title">
<input type="text" v-model="product.price">
<input type="text" v-model="product.num">
<input type="button" value="修改商品" @click="update()">
</div>
<script type="text/javascript" src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script type="text/javascript">
let v = new Vue({
el:"body>div",
data:{
product:{
id:1,
title:"hehe",
price:12,
num:10
}
},
created:function (){
axios.get("/selectById"+location.search).then(function (response) {
v.product = response.data;
})
},
methods:{
update(){
axios.post("/update",v.product).then(function () {
alert("修改成功")
location.href="/select.html"
})
}
}
})
</script>
</body>
</html>
1.5扩展练习:命令行中创建一个员工表myemp 包含:id,name,salary,job字段 创建boot4-1工程实现员工管理系统(员工的增删改查)
1.5.1代码展示
1.5.1.1sql代码展示
create database empdb;
use empdb;
create table myemp(
id int primary key auto_increment,
name varchar(40),
salary int,
job varchar(40)
);
1.5.1.2empController
package cn.tedu.boot41.controller;
import cn.tedu.boot41.entity.Emp;
import cn.tedu.boot41.mapping.EmpMapping;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.List;
@RestController
public class empController {
@Autowired
EmpMapping mapping;
@RequestMapping("/insert")
public void insert(@RequestBody Emp emp){
mapping.insert(emp);
}
@RequestMapping("/select")
public List<Emp> select(){
return mapping.select();
}
@RequestMapping("/delete")
public void delete(int id){
mapping.delete(id);
}
@RequestMapping("/selectById")
public Emp selectById(int id){
return mapping.selectById(id);
}
@RequestMapping("/update")
public void update(@RequestBody Emp emp){
mapping.update(emp);
}
}
1.5.1.3Emp
package cn.tedu.boot41.entity;
public class Emp {
private Integer id;
private String name;
private Integer salary;
private String job;
@Override
public String toString() {
return "Emp{" +
"id=" + id +
", name='" + name + '\'' +
", salary=" + salary +
", job='" + job + '\'' +
'}';
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getSalary() {
return salary;
}
public void setSalary(Integer salary) {
this.salary = salary;
}
public String getJob() {
return job;
}
public void setJob(String job) {
this.job = job;
}
}
1.5.1.4EmpMapping.java
package cn.tedu.boot41.mapping;
import cn.tedu.boot41.entity.Emp;
import org.apache.ibatis.annotations.*;
import java.util.List;
@Mapper
public interface EmpMapping {
@Insert("insert into myemp values(null,#{name},#{salary},#{job})")
void insert(Emp emp);
@Select("select * from myemp")
List<Emp> select();
@Delete("delete from myemp where id = #{id}")
void delete(int id);
@Select("select * from myemp where id = #{id}")
Emp selectById(int id);
@Update("update myemp set name = #{name},salary = #{salary},job = #{job} where id = #{id}")
void update(Emp emp);
}
1.5.1.5index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>员工管理系统</h1>
<a href="/insert.html">添加员工</a>
<a href="/select.html">查看员工信息</a>
</body>
</html>
1.5.1.6insert.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<h1>添加员工</h1>
<input type="text" v-model="myemp.name" placeholder="员工姓名">
<input type="text" v-model="myemp.salary" placeholder="员工工资">
<input type="text" v-model="myemp.job" placeholder="员工单位">
<input type="button" value="添加" @click="insert()">
</div>
<script type="text/javascript" src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script type="text/javascript">
let v = new Vue({
el:"body>div",
data:{
myemp:{
name:"",
salary:"",
job:""
}
},
methods:{
insert(){
axios.post("/insert",v.myemp).then(function (){
alert("添加成功");
location.href="/";
})
}
}
})
</script>
</body>
</html>
1.5.1.7select.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<table border="1">
<caption>员工信息</caption>
<tr>
<th>编号</th>
<th>姓名</th>
<th>工资</th>
<th>部门</th>
<th>操作</th>
</tr>
<tr v-for="e in emp">
<td>{{e.id}}</td>
<td>{{e.name}}</td>
<td>{{e.salary}}</td>
<td>{{e.job}}</td>
<td>
<a :href="'/update.html?id='+e.id">修改</a>
<a href="javascript:void(0)" @click="del(e.id)">删除</a>
</td>
</tr>
</table>
</div>
<script type="text/javascript" src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script type="text/javascript">
let v = new Vue({
el:"body>div",
data:{
emp:[
{id:1,name:"张三",salary:13333,job:"天上"},
{id:2,name:"李四",salary:14323,job:"地上"},
]
},
created:function () {
axios.get("/select").then(function (response) {
v.emp = response.data;
})
},
methods:{
del(id){
axios.get("/delete?id="+id).then(function () {
alert("删除成功")
location.reload();
})
}
}
})
</script>
</body>
</html>
1.5.1.8update.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<div>
<h1>修改员工数据</h1>
<input type="text"readonly v-model="emp.id">
<input type="text" v-model="emp.name">
<input type="text" v-model="emp.salary">
<input type="text" v-model="emp.job">
<input type="button" value="修改" @click="update()">
</div>
<script type="text/javascript" src="https://cdn.staticfile.org/vue/2.2.2/vue.min.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script type="text/javascript">
let v = new Vue({
el:"body>div",
data:{
emp:{
id:1,
name:"张三",
salary:10030,
job:"天上"
}
},
created:function (){
axios.get("/selectById"+location.search).then(function (request){
v.emp = request.data;
})
},
methods:{
update(){
axios.post("/update",v.emp).then(function(response){
alert("修改成功")
location.href = "/select.html";
})
}
}
})
</script>
</body>
</html>
2.文件上传
2.1项目目录
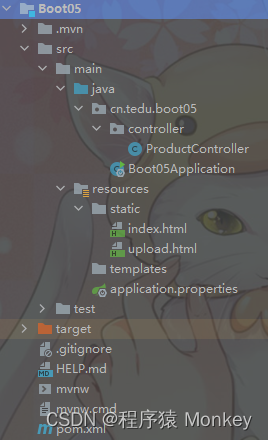
?2.2代码展示
2.2.1ProductController
package cn.tedu.boot05.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import java.io.File;
import java.io.IOException;
import java.util.UUID;
@RestController
public class ProductController {
@RequestMapping("/upload")
public void upload(MultipartFile picFile) throws IOException {
//得到原始文件名
String fileName = picFile.getOriginalFilename();
System.out.println(fileName);
//得到文件后缀 a.jpg .jpg 从.的位置开始截取 截取到最后
String suffix = fileName.substring(fileName.lastIndexOf("."));
//得到唯一的文件名 UUID.randomUUID()得到一个唯一标识符
fileName = UUID.randomUUID()+suffix;
System.out.println(fileName);
String dirPath = "E:/files";
File dirFile = new File(dirPath);
if (!dirFile.exists()){
dirFile.mkdirs();
}
//E:/files/xxxxx.jpg
String filePath = dirPath+"/"+fileName;
//吧文件保存到指定的路径 异常抛出
picFile.transferTo(new File(filePath));
}
}
2.2.2index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
<h1>文件上传练习首页</h1>
<a href="/upload.html">上传文件</a>
</body>
</html>
2.2.3upload.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<!-- import CSS -->
<link rel="stylesheet" href="https://unpkg.com/element-ui/lib/theme-chalk/index.css">
</head>
<body>
<div id="app">
<el-upload
action="/upload"
name="picFile"
list-type="picture-card"
:on-preview="handlePictureCardPreview"
:on-remove="handleRemove">
<i class="el-icon-plus"></i>
</el-upload>
<el-dialog :visible.sync="dialogVisible">
<img width="100%" :src="dialogImageUrl" alt="">
</el-dialog>
</div>
</body>
<!-- import Vue before Element -->
<script src="https://cdn.staticfile.org/vue/2.6.14/vue.min.js"></script>
<!-- import JavaScript -->
<script src="https://unpkg.com/element-ui/lib/index.js"></script>
<script src="https://cdn.bootcdn.net/ajax/libs/axios/0.21.1/axios.min.js"></script>
<script>
let v = new Vue({
el: '#app',
data: function() {
return {//在这里面定义变量
dialogImageUrl: '',
dialogVisible: false
}
},
methods: {
handleRemove(file, fileList) {
console.log(file, fileList);
},
handlePictureCardPreview(file) {
this.dialogImageUrl = file.url;
this.dialogVisible = true;
}
}
})
</script>
</html>
|