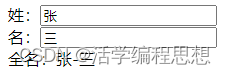 需求分析: 两个输入框进行联动,全名随着两个输入框的输入而变化拼接
使用差值语法实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_差值语法实现</title></title>
<!-- 引入vue -->
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
姓:<input type="text" v-model="firstName"><br/><br/>
名:<input type="text" v-model="lastName"><br/><br/>
全名:<span>{{firstName.slice(0, 3)}}--{{lastName}}</span>
</div>
<script type="text/javascript">
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
firstName:'张',
lastName:'三'
}
})
console.log(vm)
</script>
</body>
</html>
使用methods实现
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_methods实现</title></title>
<!-- 引入vue -->
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
姓:<input type="text" v-model="firstName"><br/><br/>
名:<input type="text" v-model="lastName"><br/><br/>
全名:<span>{{fullName()}}</span>
</div>
<script type="text/javascript">
Vue.config.productionTip = false
const vm = new Vue({
el:'#root',
data:{
firstName:'张',
lastName:'三'
},
methods: {
fullName() {
return this.firstName + '-' + this.lastName
}
}
})
console.log(vm)
</script>
</body>
</html>
computed 计算属性实现
computed计算属性
-
1、定义:要用的属性不存在,需要通过已有属性计算得来 -
2、原理:底层借助了Objcet.defineproperty()方法提供的getter和setter -
3、get函数什么时候执行? (1)初次读取时会执行一次 (2)当依赖的数据发生改变时会被再次调用 -
4、优势:与methods实现相比,内部有缓存机制(复用),效率更高,调试方便 -
5、备注 (1)计算属性最终会出现在vm上,直接读取使用即可 (2)如果计算属性要被修改,那必须写set函数去响应修改,且set中要引起计算时依赖的数据发生改变 (3)如果计算属性确定不考虑修改,可以使用计算属性的简写形式
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_计算属性实现</title>
</title>
<!-- 引入vue -->
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
姓:<input type="text" v-model="firstName"><br /><br />
名:<input type="text" v-model="lastName"><br /><br />
全名:<span>{{fullName}}</span>
</div>
<script type="text/javascript">
Vue.config.productionTip = false
const vm = new Vue({
el: '#root',
data: {
firstName: '张',
lastName: '三'
},
computed: {
fullName() {
console.log('get被调用了');
return this.firstName + '-' + this.lastName
}
}
})
console.log(vm)
</script>
</body>
</html>
监听属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>姓名案例_差值语法实现</title></title>
<!-- 引入vue -->
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<h2>今天天气很{{info}}</h2>
<!-- <button @click="isHot = !isHot">切换天气</button> -->
<button @click="changeWeather">切换天气</button>
</div>
<script type="text/javascript">
Vue.config.productionTip = false
new Vue({
el:'#root',
data:{
isHot:true
},
computed:{
info() {
return this.isHot ? '炎热' : '凉爽'
}
},
methods: {
changeWeather() {
this.isHot = !this.isHot
}
},
})
</script>
</body>
</html>
侦听属性基本用法
watch监视属性
- 1、当被监视的属性变化时,回调函数自动调用,进行相关操作
- 2、监视的属性必须存在,才能进行监视,既可以监视data,也可以监视计算属性
- 3、配置项属性**immediate:false,改为 true,**则初始化时调用一次
handler(newValue,oldValue) - 4、监视有两种写法
(1)创建Vue时传入watch: {} 配置 (2)通过vm.$watch() 监视
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气案例_监视属性</title>
</title>
<!-- 引入vue -->
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="root">
<h2>今天天气很{{info}}</h2>
<!-- <button @click="isHot = !isHot">切换天气</button> -->
<button @click="changeWeather">切换天气</button>
</div>
<script type="text/javascript">
Vue.config.productionTip = false
const vm = new Vue({
el: '#root',
data: {
isHot: true
},
computed: {
info() {
return this.isHot ? '炎热' : '凉爽'
}
},
methods: {
changeWeather() {
this.isHot = !this.isHot
}
},
})
vm.$watch('isHot', {
handler(newValue, oldValue) {
console.log('isHot 被修改了', newValue, oldValue);
}
})
</script>
</body>
</html>
深度监听
深度监听
- 1、Vue中的watch默认不监测对象内部值的改变(一层)
- 2、在watch中配置
deep:true 可以监测对象内部值的改变(多层)
注意
- 1、Vue自身可以监测对象内部值的改变,但Vue提供的watch默认不可以
- 2、使用watch时根据监视数据的具体结构,决定是否采用深度监视
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气案例_深度监视</title>
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<div id="root">
<h3>a的值是:{{ numbers.a }}</h3>
<button @click="numbers.a++">点我让a+1</button>
<h3>b的值是:{{ numbers.b }}</h3>
<button @click="numbers.b++">点我让b+1</button>
<button @click="numbers = {a:666,b:888}">彻底替换掉numbers</button>
{{numbers.c.d.e}}
</div>
</body>
<script type="text/javascript">
Vue.config.productionTip = false
const vm = new Vue({
el: '#root',
data: {
isHot: true,
numbers: {
a: 1,
b: 1,
c: {
d: {
e: 100
}
}
}
},
watch: {
numbers: {
deep: true,
handler() {
console.log('numbers改变了')
}
}
}
})
</script>
</html>
监听的简写方法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>天气案例_深度监视</title>
<script type="text/javascript" src="../js/vue.js"></script>
</head>
<body>
<div id="root">
<h2>今天天气很好{{info}}</h2>
<button @click="changeWeather">切换天气</button>
</div>
</body>
<script type="text/javascript">
Vue.config.productionTip = false
const vm = new Vue({
el: '#root',
data: {
isHot: true,
},
computed: {
info() {
return this.isHot ? '炎热' : '凉爽'
}
},
methods: {
changeWeather() {
this.isHot = !this.isHot
}
},
watch: {
isHot: {
handler(newValue, oldValue) {
console.log('isHot 被修改了', newValue, oldValue);
}
},
isHot(newValue, oldValue) {
console.log('isHot 被修改了', newValue, oldValue);
}
}
})
vm.$watch('isHot', {
handler(newValue, oldValue) {
console.log('isHot 被修改了', newValue, oldValue);
}
})
vm.$watch('isHot', function(newValue, oldValue) {
console.log('isHot 被修改了', newValue, oldValue);
})
</script>
</html>
|