vue项目的封装引用
在vue 的项目,使用axios 进行接口访问;在引用中,时常会出现需要传递同一参数或进行同意一接口拦截的事件处理,在这的基础上,为了方便项目的统一的管理使用,采用的是对axios 的封装: 项目是基于vue3.0 与typescript 的形式创建,这里进行ts的形式封装并导出对应的请求方法: 在项目中新建一个service 的文件夹,文件的目录如下: 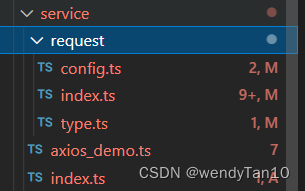
request 用于存放环境的配置与type的类型的定义
config.ts 类型的配置index.ts axios 请求类的定义type.ts typescript 类型的定义 index.ts 进行实例化类并进行导出供使用
其相对应的文件内容:
service目录下的文件
config.ts 配置环境
这里的配置采用的是:webpack 中的definePlugin 插件注入不同环境的值,从而达到区分不同的环境 (关于这里的配置可进行点击查看vue中的axios的请求(一))
let BASE_URL = ''
const TIME_OUT = 10000
if (process.env.NODE_ENV === 'development') {
BASE_URL = 'http://123.207.32.32:8000/'
} else if (process.env.NODE_ENV === 'production') {
BASE_URL = 'http://****.org/prod'
} else {
BASE_URL = 'http://****.org/test'
}
export { BASE_URL, TIME_OUT }
type.js 进行类型的定义
import type { AxiosRequestConfig, AxiosResponse } from 'axios';
export interface InRequestInterceptors<T = AxiosResponse> {
requestInterceptor?: (config: AxiosRequestConfig) => AxiosRequestConfig
requestInterceptorCatch?: (error: any) => any
responseInterceptor?: (res: T) => T
responseInterceptorCatch?: (error: any) => any
}
export interface InRequestConfig<T = AxiosResponse> extends AxiosRequestConfig {
interceptors?: InRequestInterceptors<T>
showLoading?: boolean
}
index.js 进行自定义InRequest请求类的定义
import axios from 'axios';
import type { AxiosInstance } from 'axios';
import type { InRequestInterceptors, InRequestConfig } from './type';
import { ElLoading } from 'element-plus';
import { ILoadingInstance } from 'element-plus/lib/el-loading/src/loading.type';
const DEAFULT_LOADING = true;
class InRequest{
instance: AxiosInstance
interceptors?: InRequestInterceptors
showLoading: boolean
loading?: ILoadingInstance
constructor(config: InRequestConfig) {
this.instance = axios.create(config);
this.showLoading = config.showLoading ?? DEAFULT_LOADING
this.interceptors = config.interceptors;
this.instance.interceptors.request.use(
this.interceptors?.requestInterceptor,
this.interceptors?.requestInterceptorCatch
);
this.instance.interceptors.response.use(
this.interceptors?.responseInterceptor,
this.interceptors?.responseInterceptorCatch
);
this.instance.interceptors.request.use(
(config) => {
console.log('所有的实例都有的拦截器: 请求成功拦截');
if (this.showLoading) {
this.loading = ElLoading.service({
lock: true,
text: '正在请求数据....',
background: 'rgba(0, 0, 0, 0.5)'
})
}
return config;
},
(err) => {
console.log('所有的实例都有的拦截器: 请求失败拦截');
return err;
}
)
this.instance.interceptors.response.use(
(res) => {
console.log('所有的实例都有的拦截器: 响应成功拦截')
this.loading?.close();
const data = res.data;
if (data.returnCode === '-1001') {
console.log('请求失败~, 错误信息')
} else {
return data;
}
},
(err) => {
console.log('所有的实例都有的拦截器: 响应失败拦截');
this.loading?.close();
if (err.response.status === 404) {
console.log('404的错误~');
}
return err;
}
)
}
request<T>(config: InRequestConfig<T>): Promise<T> {
return new Promise((resolve, reject) => {
if (config.interceptors?.requestInterceptor) {
config = config.interceptors.requestInterceptor(config);
}
if (config.showLoading === false) {
this.showLoading = config.showLoading;
}
this.instance
.request<any, T>(config)
.then((res) => {
if (config.interceptors?.responseInterceptor) {
res = config.interceptors.responseInterceptor(res);
}
this.showLoading = DEAFULT_LOADING;
resolve(res);
})
.catch((err) => {
this.showLoading = DEAFULT_LOADING;
reject(err);
return err;
})
})
}
get<T>(config: InRequestConfig<T>): Promise<T> {
return this.request<T>({ ...config, method: 'GET' });
}
post<T>(config: InRequestConfig<T>): Promise<T> {
return this.request<T>({ ...config, method: 'POST' });
}
delete<T>(config: InRequestConfig<T>): Promise<T> {
return this.request<T>({ ...config, method: 'DELETE' });
}
patch<T>(config: InRequestConfig<T>): Promise<T> {
return this.request<T>({ ...config, method: 'PATCH' });
}
}
export default InRequest
index.ts 定义实例类的统一接口导出;
import InRequest from './request';
import { BASE_URL, TIME_OUT } from './request/config';
const inRequest= new InRequest({
baseURL: BASE_URL,
timeout: TIME_OUT,
interceptors: {
requestInterceptor: (config) => {
const token = '';
if (token) {
config.headers.Authorization = `Bearer ${token}`;
}
console.log('请求成功的拦截');
return config;
},
requestInterceptorCatch: (err) => {
console.log('请求失败的拦截');
return err;
},
responseInterceptor: (res) => {
console.log('响应成功的拦截');
return res;
},
responseInterceptorCatch: (err) => {
console.log('响应失败的拦截');
return err;
}
}
});
export default InRequest;
在项目中直接引用index.js 文件进行使用;
|