vue2源码浅读(三):数据响应式
数据响应式入口
- 紧接着上一篇,在
initState 中初始化data,调用了initData() 方法: - src\core\instance\state.js
function initData (vm) {
let data = vm.$options.data
data = vm._data = typeof data === 'function'
? getData(data, vm)
: data || {}
if (!isPlainObject(data)) {
data = {}
process.env.NODE_ENV !== 'production' && warn(
'data functions should return an object:\n' +
'https://vuejs.org/v2/guide/components.html#data-Must-Be-a-Function',
vm
)
}
const keys = Object.keys(data)
const props = vm.$options.props
const methods = vm.$options.methods
let i = keys.length
while (i--) {
const key = keys[i]
if (process.env.NODE_ENV !== 'production') {
if (methods && hasOwn(methods, key)) {
warn(
`Method "${key}" has already been defined as a data property.`,
vm
)
}
}
if (props && hasOwn(props, key)) {
process.env.NODE_ENV !== 'production' && warn(
`The data property "${key}" is already declared as a prop. ` +
`Use prop default value instead.`,
vm
)
} else if (!isReserved(key)) {
proxy(vm, `_data`, key)
}
}
observe(data, true )
}
- vue 设计, 不希望访问 _ 开头的数据,_ 开头的数据是私有数据
- 那么,经过proxy后, data挂载vm的实例上,访问app.xxx就相当于访问app._data.xxx
const sharedPropertyDefinition = {
enumerable: true,
configurable: true,
get: noop,
set: noop
}
export function proxy (target: Object, sourceKey: string, key: string) {
sharedPropertyDefinition.get = function proxyGetter () {
return this[sourceKey][key]
}
sharedPropertyDefinition.set = function proxySetter (val) {
this[sourceKey][key] = val
}
Object.defineProperty(target, key, sharedPropertyDefinition)
}
- 找到observe方法,这里才是响应式处理入口
- src\core\observer\index.js
function observe (value, asRootData) {
if (!isObject(value) || value instanceof VNode) {
return
}
let ob;
if (hasOwn(value, '__ob__') && value.__ob__ instanceof Observer) {
ob = value.__ob__
} else if (
shouldObserve &&
!isServerRendering() &&
(Array.isArray(value) || isPlainObject(value)) &&
Object.isExtensible(value) &&
!value._isVue
) {
ob = new Observer(value)
}
if (asRootData && ob) {
ob.vmCount++
}
return ob
}
响应式处理
- Vue的响应式数据分为两类:对象和数组
- 遍历对象的所有属性,并为每个属性设置getter和setter,以便将来的获取和设置,如果属性的值也是对象,则递归为属性值上的每个key设置getter和setter
- 接下来进入正题, Observer 类的具体实现
- src\core\observer\index.js
class Observer {
value;
dep;
vmCount: number;
constructor (value) {
this.value = value
this.dep = new Dep()
this.vmCount = 0
def(value, '__ob__', this)
if (Array.isArray(value)) {
if (hasProto) {
protoAugment(value, arrayMethods)
} else {
copyAugment(value, arrayMethods, arrayKeys)
}
this.observeArray(value)
} else {
this.walk(value)
}
}
walk (obj: Object) {
const keys = Object.keys(obj)
for (let i = 0; i < keys.length; i++) {
defineReactive(obj, keys[i])
}
}
observeArray (items: Array<any>) {
for (let i = 0, l = items.length; i < l; i++) {
observe(items[i])
}
}
}
数组响应式处理
- 众所周知,
Object.defineProperty 不能监听数组的变化,具体为使用push、unshift、pop、shift、splice, sort, revers ,是触发不了set的。 - 通过重写数组操作方法实现数组监听
- src\core\observer\array.js
const arrayProto = Array.prototype
export const arrayMethods = Object.create(arrayProto)
const methodsToPatch = [
'push',
'pop',
'shift',
'unshift',
'splice',
'sort',
'reverse'
]
methodsToPatch.forEach(function (method) {
const original = arrayProto[method]
def(arrayMethods, method, function mutator (...args) {
const result = original.apply(this, args)
const ob = this.__ob__
let inserted
switch (method) {
case 'push':
case 'unshift':
inserted = args
break
case 'splice':
inserted = args.slice(2)
break
}
if (inserted) ob.observeArray(inserted)
ob.dep.notify()
return result
})
})
对象响应式处理
- 在Observer类里边,walk方法里调用了defineReactive方法
- 获取数据时:在dep中添加相关的watcher
- 设置数据时:再由dep通知相关的watcher去更新
- src\core\observer\index.js
function defineReactive (obj,key,valcustomSetter,shallow) {
const dep = new Dep();
const property = Object.getOwnPropertyDescriptor(obj, key)
if (property && property.configurable === false) {
return
}
const getter = property && property.get
const setter = property && property.set
if ((!getter || setter) && arguments.length === 2) {
val = obj[key]
}
let childOb = !shallow && observe(val)
Object.defineProperty(obj, key, {
enumerable: true,
configurable: true,
get: function reactiveGetter () {
const value = getter ? getter.call(obj) : val
if (Dep.target) {
dep.depend()
if (childOb) {
childOb.dep.depend()
if (Array.isArray(value)) {
dependArray(value)
}
}
}
return value
},
set: function reactiveSetter (newVal) {
const value = getter ? getter.call(obj) : val
if (newVal === value || (newVal !== newVal && value !== value)) {
return
}
if (getter && !setter) return
if (setter) {
setter.call(obj, newVal)
} else {
val = newVal
}
childOb = !shallow && observe(newVal)
dep.notify()
}
})
}
function dependArray (value: Array<any>) {
for (let e, i = 0, l = value.length; i < l; i++) {
e = value[i]
e && e.__ob__ && e.__ob__.dep.depend()
if (Array.isArray(e)) {
dependArray(e)
}
}
}
依赖管理Dep类
- 接着找到依赖管理Dep类,主要实现提供依赖收集 ( depend ) 和 派发更新 ( notify ) 的功能。
- 每个在页面上使用了的数据都会有一个Dep 类,主要访问属性的时候 get 方法会收集对应的 watcher
- 在获取数据的时候知道自己(Dep)依赖的watcher都有谁,同时在数据变更的时候通知自己(Dep)依赖的这些watcher去执行对应update,以在页面多组件情况下实现局部渲染。
- src\core\observer\dep.js
class Dep {
static target: ?Watcher;
id: number;
subs: Array<Watcher>;
constructor () {
this.id = uid++
this.subs = []
}
addSub (sub: Watcher) {
this.subs.push(sub)
}
removeSub (sub: Watcher) {
remove(this.subs, sub)
}
depend () {
if (Dep.target) {
Dep.target.addDep(this)
}
}
notify () {
const subs = this.subs.slice()
if (process.env.NODE_ENV !== 'production' && !config.async) {
subs.sort((a, b) => a.id - b.id)
}
for (let i = 0, l = subs.length; i < l; i++) {
subs[i].update()
}
}
}
Dep.target = null
const targetStack = []
export function pushTarget (target: ?Watcher) {
targetStack.push(target)
Dep.target = target
}
export function popTarget () {
targetStack.pop()
Dep.target = targetStack[targetStack.length - 1]
}
观察者watcher
- 页面访问的属性,将该属性的watcher 关联到该属性的 dep 中
- 同时, 将 dep 也存储 watcher 中. ( 互相引用的关系 )
- 在 Watcher 调用 get 方法的时候, 将当前 Watcher 放到全局, 在 get 之前结束的时候(之后), 将这个 全局的 watcher 移除
- src\core\observer\watcher.js
class Watcher {
vm: Component;
expression: string;
cb: Function;
id: number;
deep: boolean;
user: boolean;
lazy: boolean;
sync: boolean;
dirty: boolean;
active: boolean;
deps: Array<Dep>;
newDeps: Array<Dep>;
depIds: SimpleSet;
newDepIds: SimpleSet;
before: ?Function;
getter: Function;
value: any;
constructor (
vm: Component,
expOrFn: string | Function,
cb: Function,
options?: ?Object,
isRenderWatcher?: boolean
) {
this.vm = vm
if (isRenderWatcher) {
vm._watcher = this
}
vm._watchers.push(this)
if (options) {
this.deep = !!options.deep
this.user = !!options.user
this.lazy = !!options.lazy
this.sync = !!options.sync
this.before = options.before
} else {
this.deep = this.user = this.lazy = this.sync = false
}
this.cb = cb
this.id = ++uid
this.active = true
this.dirty = this.lazy
this.deps = []
this.newDeps = []
this.depIds = new Set()
this.newDepIds = new Set()
this.expression = process.env.NODE_ENV !== 'production'
? expOrFn.toString()
: ''
if (typeof expOrFn === 'function') {
this.getter = expOrFn
} else {
this.getter = parsePath(expOrFn)
if (!this.getter) {
this.getter = noop
process.env.NODE_ENV !== 'production' && warn(
`Failed watching path: "${expOrFn}" ` +
'Watcher only accepts simple dot-delimited paths. ' +
'For full control, use a function instead.',
vm
)
}
}
this.value = this.lazy
? undefined
: this.get()
}
get () {
pushTarget(this)
let value
const vm = this.vm
try {
value = this.getter.call(vm, vm)
} catch (e) {
if (this.user) {
handleError(e, vm, `getter for watcher "${this.expression}"`)
} else {
throw e
}
} finally {
if (this.deep) {
traverse(value)
}
popTarget()
this.cleanupDeps()
}
return value
}
addDep (dep: Dep) {
const id = dep.id
if (!this.newDepIds.has(id)) {
this.newDepIds.add(id)
this.newDeps.push(dep)
if (!this.depIds.has(id)) {
dep.addSub(this)
}
}
}
cleanupDeps () {
let i = this.deps.length
while (i--) {
const dep = this.deps[i]
if (!this.newDepIds.has(dep.id)) {
dep.removeSub(this)
}
}
let tmp = this.depIds
this.depIds = this.newDepIds
this.newDepIds = tmp
this.newDepIds.clear()
tmp = this.deps
this.deps = this.newDeps
this.newDeps = tmp
this.newDeps.length = 0
}
update () {
if (this.lazy) {
this.dirty = true
} else if (this.sync) {
this.run()
} else {
queueWatcher(this)
}
}
run () {
if (this.active) {
const value = this.get()
if (
value !== this.value ||
isObject(value) ||
this.deep
) {
const oldValue = this.value
this.value = value
if (this.user) {
const info = `callback for watcher "${this.expression}"`
invokeWithErrorHandling(this.cb, this.vm, [value, oldValue], this.vm, info)
} else {
this.cb.call(this.vm, value, oldValue)
}
}
}
}
evaluate () {
this.value = this.get()
this.dirty = false
}
depend () {
let i = this.deps.length
while (i--) {
this.deps[i].depend()
}
}
teardown () {
if (this.active) {
if (!this.vm._isBeingDestroyed) {
remove(this.vm._watchers, this)
}
let i = this.deps.length
while (i--) {
this.deps[i].removeSub(this)
}
this.active = false
}
}
}
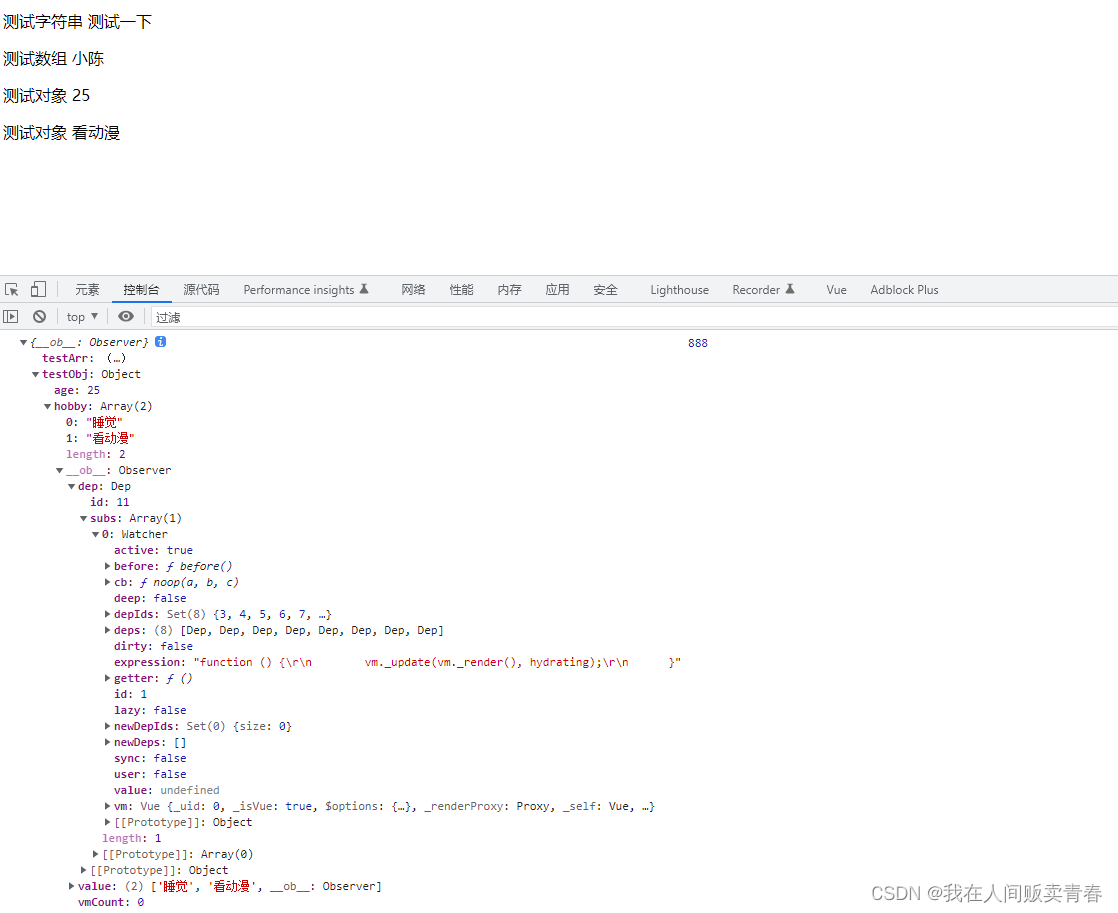
梳理整个过程
- 在initState方法中,首先是用户传入参数有没有data,没有则
observe(vm._data = {}, true /* asRootData */) ,将一个空的data代理到vm实例上,有则调用initData方法。 - 在initData方法中,主要遍历data中的属性,通过
proxy(vm, _data, key) ,并将其代理到vm实例上,然后对data进行观察observe(data, true /* asRootData */) ; - 在observe方法中,主要判断传入的value有没有
__ob__ 属性,有则说明已经被观察过了,没有则进行new Observer(value) 。 - 在Observer类中,主要实现了对数组和对象的响应式处理。
- 首先是调用
def(value, '__ob__', this) 给value添加__ob__ 属性; - 判断value如果是数组,再判断当前环境
- 如果是现代的浏览器,复杂类型数据有原型,调用arrayMethods,重写相关方法
- 将数组原型指向空对象上,对
push、unshift、pop、shift、splice, sort, revers 方法重写覆盖。具体还是执行原来的行为逻辑,主要是拦截对其做响应式处理:调用 ob.dep.notify()通知更新,如果是新插入的元素调用ob.observeArray(inserted)。 - 如果是老旧浏览器,没有原型,直接给数组上的方法给重写替换掉
- 最后调用observeArray方法,对每个数组元素进行observe
- 判断value如果是对象
- 遍历对象,对每个键调用
defineReactive(obj, keys[i]) 方法,做响应式处理 - 在defineReactive(obj, keys[i])方法中,首先对key都会实例化一个Dep。
- 在get方法中,收集依赖,把dep添加到watcher中,也将watcher添加到dep中
dep.depend() 。
- 如果有子对象,则进行嵌套对象的依赖收集
childOb.dep.depend() 。 - 如果是数组,数组内部的所有项都需要做依赖收集
dependArray(value) 。 - 在set方法中,如果新值和老值相等则不做处理直接返回,否则赋新值,且对新值做响应式处理,
dep.notify() 通知更新 - 在Dep类中,访问属性的时候 get 方法会收集对应的 watcher,在数据变更的时候通知自己(Dep)依赖的这些watcher去执行对应update,实现局部渲染。
- Watcher类中,将Dep也存储 watcher 中,形成相互引用的关系,提供了update方法
- Watcher中 不止会监听Observer,还会直接把值计算出来放在this.value上。其中get方法主要应用于computed计算和watch监听。
|