routes和router的区别
router
写路由的时候的Router对象
通过调用 app.use(router),我们可以在任意组件中以 this.
r
o
u
t
e
r
的形式访问它,并且以
t
h
i
s
.
router 的形式访问它,并且以 this.
router的形式访问它,并且以this.route 的形式访问当前路由:
export default new Router({
mode: 'history',
scrollBehavior: () => ({ y: 0 }),
routes: [
{
path: '/redirect',
component: Layout,
hidden: true,
children: [
{
path: '/redirect/:path(.*)',
component: () => import('@/views/redirect')
}
]
}
]
})
写路由的时候的router文件夹
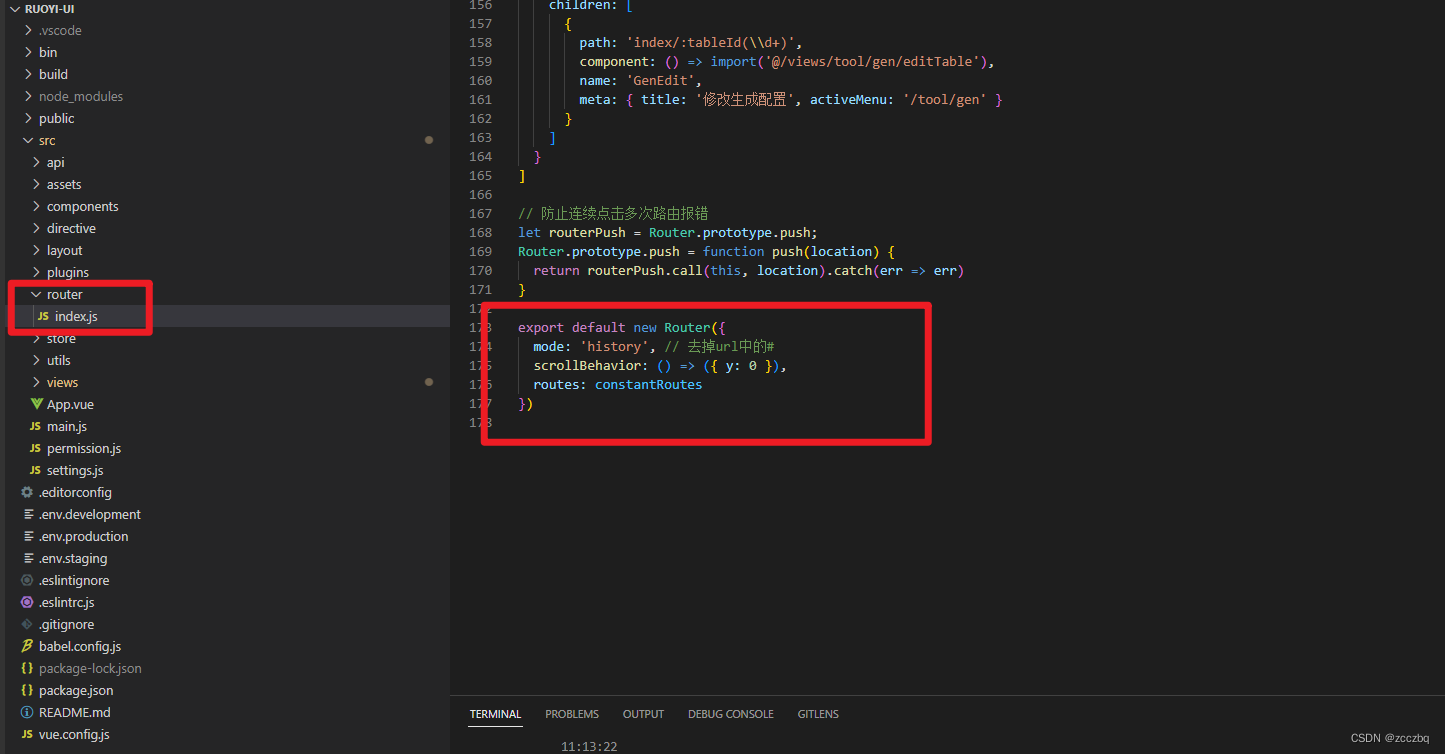
router-link和router-view
我们没有使用常规的 a 标签,而是使用一个自定义组件 router-link 来创建链接。这使得 Vue Router 可以在不重新加载页面的情况下更改 URL,处理 URL 的生成以及编码。我们将在后面看到如何从这些功能中获益。
<router-link to="/user/profile">
<el-dropdown-item>个人中心</el-dropdown-item>
</router-link>
将显示与 url 对应的组件。你可以把它放在任何地方,以适应你的布局。
<template>
<div id="app">
<router-view />
</div>
</template>
routes(路由规则)
写路由的时候,Router对象下面的属性
<script>
import Home from './Home.vue'
import About from './About.vue'
import NotFound from './NotFound.vue'
const routes = {
'/': Home,
'/about': About
}
export default {
data() {
return {
currentPath: window.location.hash
}
},
computed: {
currentView() {
return routes[this.currentPath.slice(1) || '/'] || NotFound
}
},
mounted() {
window.addEventListener('hashchange', () => {
this.currentPath = window.location.hash
})
}
}
</script>
<template>
<a href="#/">Home</a> |
<a href="#/about">About</a> |
<a href="#/non-existent-path">Broken Link</a>
<component :is="currentView" />
</template>
$route和$router区别
$route
当前路由对象,可以获取当前路由的 path, name, params, query 等属性。
很多时候,我们需要将给定匹配模式的路由映射到同一个组件。例如,我们可能有一个 User 组件,它应该对所有用户进行渲染,但用户 ID 不同。在 Vue Router 中,我们可以在路径中使用一个动态字段来实现,我们称之为 路径参数 :
const User = {
template: '<div>User</div>',
}
const routes = [
{ path: '/users/:id', component: User },
]
现在像 /users/johnny 和 /users/jolyne 这样的 URL 都会映射到同一个路由。
路径参数 用冒号 : 表示。当一个路由被匹配时,它的 params 的值将在每个组件中以 this.$route.params 的形式暴露出来。因此,我们可以通过更新 User 的模板来呈现当前的用户 ID:
const User = {
template: '<div>User {{ $route.params.id }}</div>',
}
$router
$router全局路由对象,this.$router 与直接使用通过 createRouter 创建的 router 实例完全相同。我们使用 this.$router 的原因是,我们不想在每个需要操作路由的组件中都导入路由。 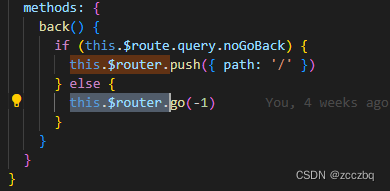
export default {
computed: {
username() {
return this.$route.params.username
},
},
methods: {
goToDashboard() {
if (isAuthenticated) {
this.$router.push('/dashboard')
} else {
this.$router.push('/login')
}
},
},
}
|