第 3 章:使用 Vue 脚手架 3.1 初始化脚手架
3.1.1 说明
- Vue 脚手架是 Vue 官方提供的标准化开发工具(开发平台)。
- 最新的版本是 4.x。
- 文档: https://cli.vuejs.org/zh/。
3.1.2 具体步骤
第一步(仅第一次执行):全局安装@vue/cli。 npm install -g @vue/cli 第二步:切换到你要创建项目的目录,然后使用命令创建项目 vue create xxxx //xxxx为自己项目名 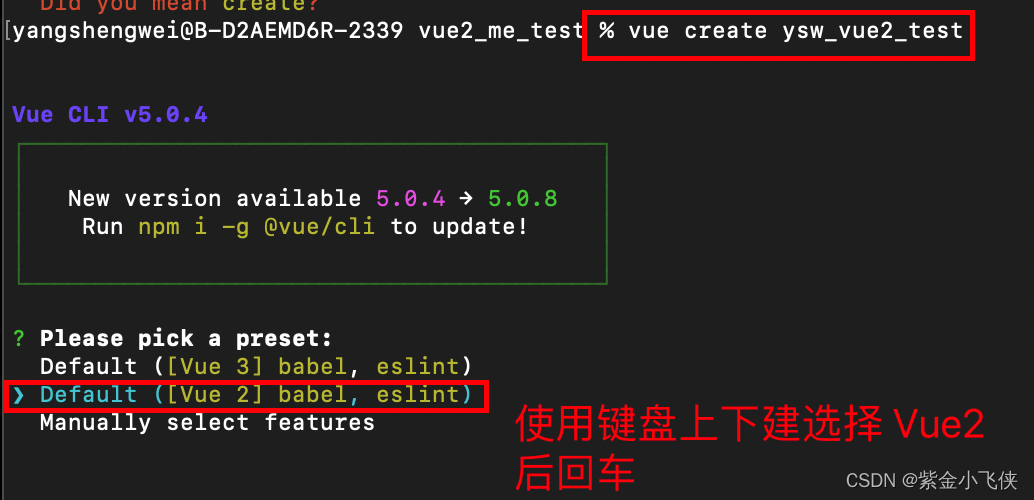 开下载构建脚手架需要的文件 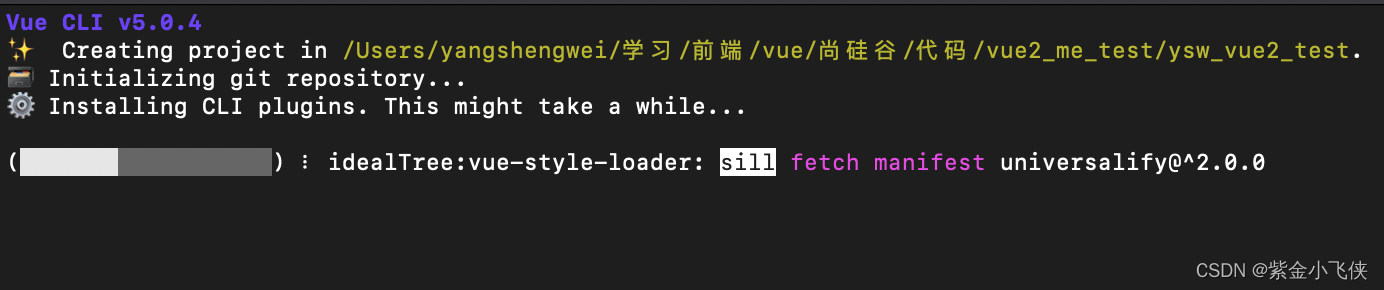
第三步:启动项目 npm run serve /Users/yangshengwei/学习/前端/vue/尚硅谷/代码/vue2_me_test 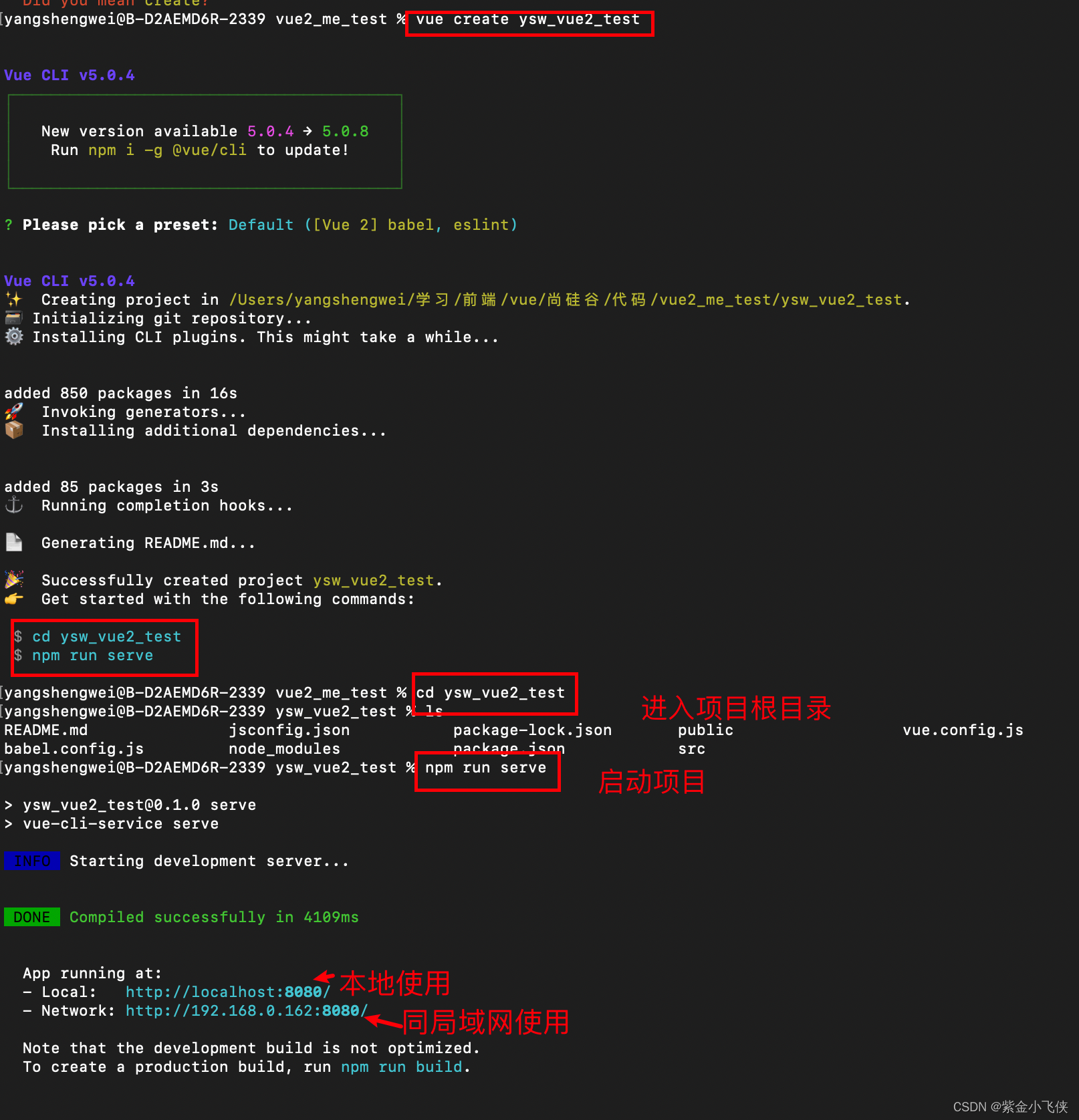 复制地址浏览打开 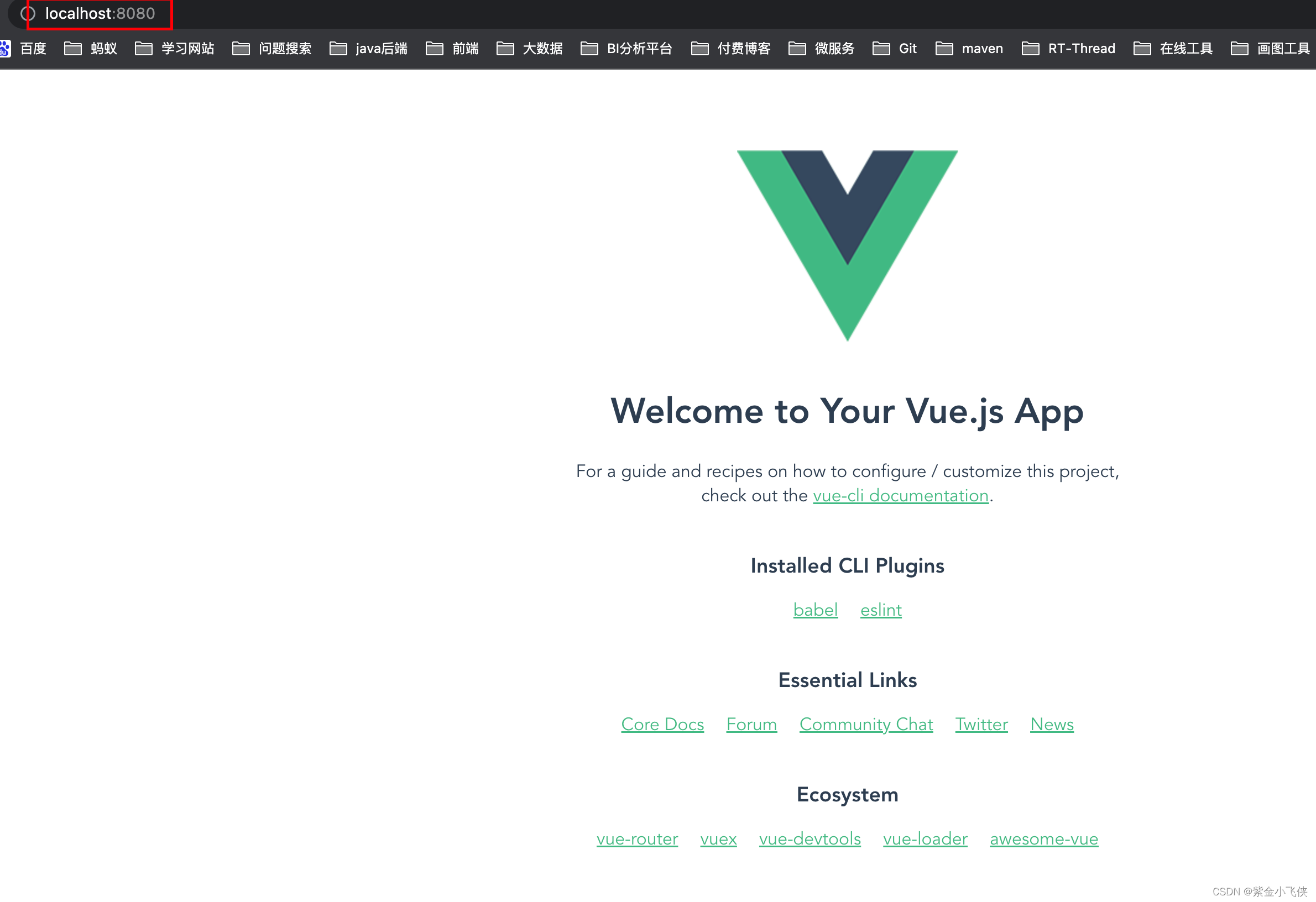 同局域网手机打开 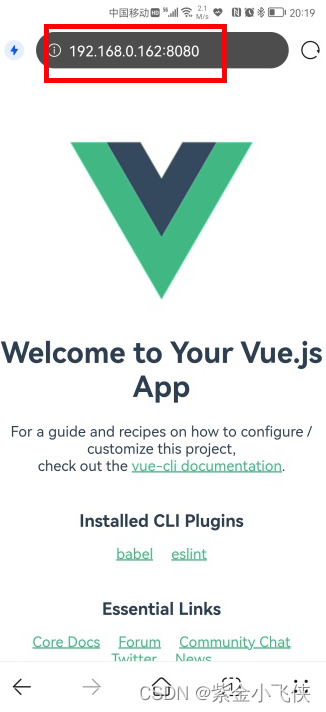 第四步退出 control +c 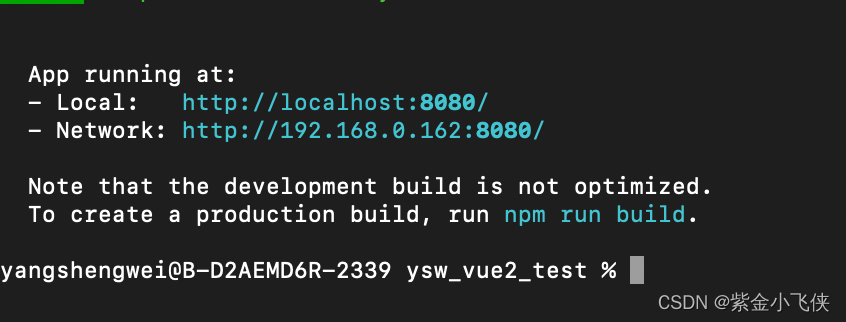 vscode 中打开项目运行
vscode 中修改代码,使用control+s 保存 项目会自动编辑更新执行 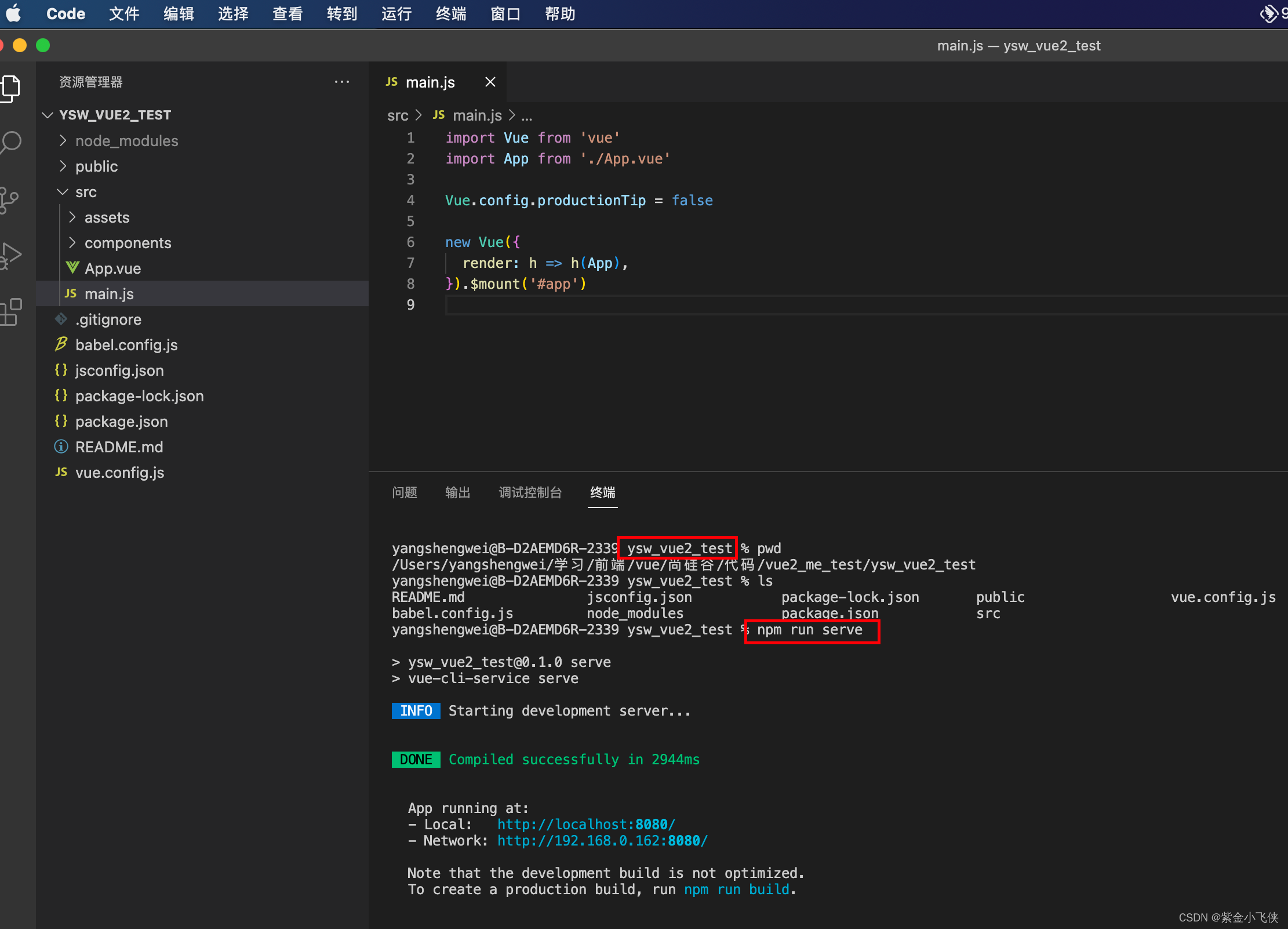
vue的项目结构
脚手架文件结构
├── node_modules
├── public
│ ├── favicon.ico: 页签图标
│ └── index.html: 主页面
├── src
│ ├── assets: 存放静态资源
│ │ └── logo.png
│ │── component: 存放组件
│ │ └── HelloWorld.vue
│ │── App.vue: 汇总所有组件
│ │── main.js: 入口文件
├── .gitignore: git版本管制忽略的配置
├── babel.config.js: babel的配置文件
├── package.json: 应用包配置文件
├── README.md: 应用描述文件
├── package-lock.json:包版本控制文件
main.js
npm run serve运行后首先进入 main.js 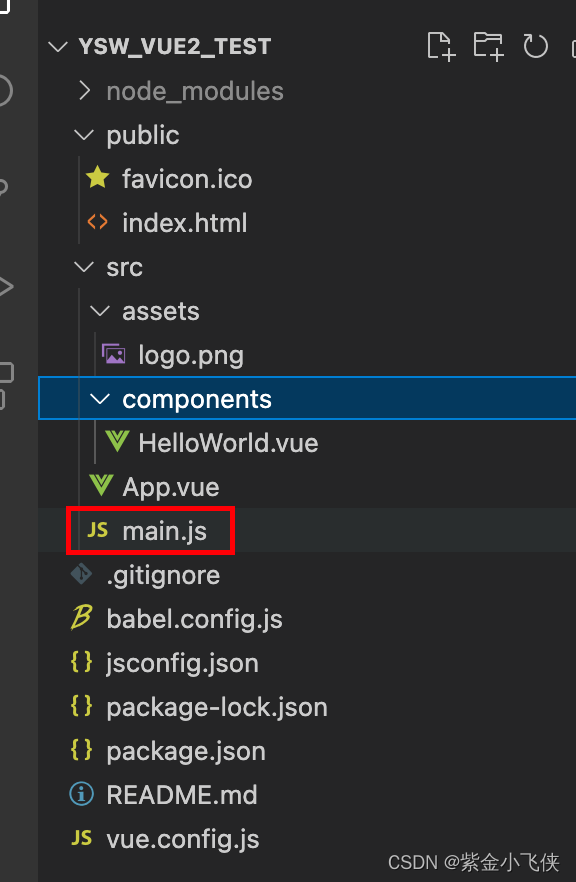 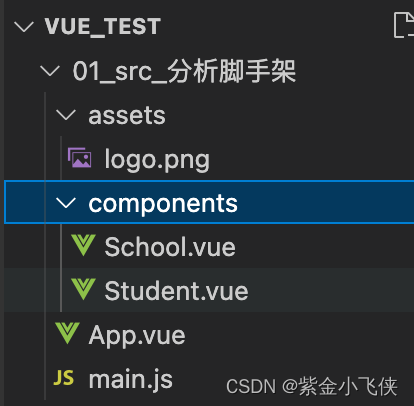
/*
该文件是整个项目的入口文件
*/
//引入Vue
import Vue from 'vue'
//引入App组件,它是所有组件的父组件
import App from './App.vue'
//关闭vue的生产提示
Vue.config.productionTip = false
/*
关于不同版本的Vue:
1.vue.js与vue.runtime.xxx.js的区别:
(1).vue.js是完整版的Vue,包含:核心功能+模板解析器。
(2).vue.runtime.xxx.js是运行版的Vue,只包含:核心功能;没有模板解析器。
2.因为vue.runtime.xxx.js没有模板解析器,所以不能使用template配置项,需要使用
render函数接收到的createElement函数去指定具体内容。
*/
//创建Vue实例对象---vm
new Vue({
el:'#app',
//render函数完成了这个功能:将App组件放入容器中
render: h => h(App),
// render:q=> q('h1','你好啊')
// template:`<h1>你好啊</h1>`,
// components:{App},
})
App.vue
<template>
<div>
<img src="./assets/logo.png" alt="logo">
<School></School>
<Student></Student>
</div>
</template>
<script>
import School from './components/School'
import Student from './components/Student'
export default {
name:'App',
components:{
School,
Student
}
}
</script>
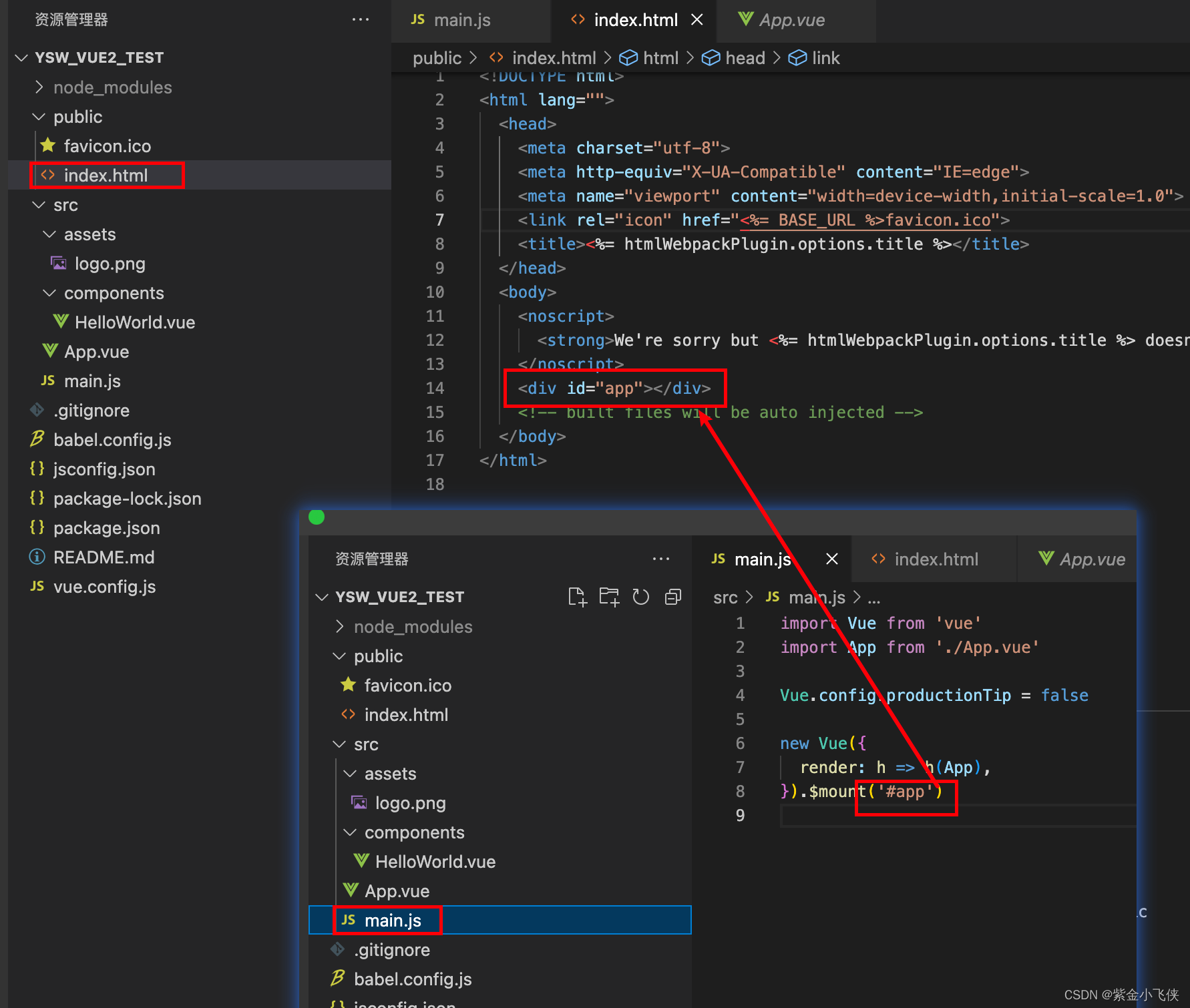
index.html
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<link rel="icon" href="<%= BASE_URL %>favicon.ico">
<link rel="stylesheet" href="<%= BASE_URL %>css/bootstrap.css">
<title>硅谷系统</title>
</head>
<body>
<noscript>
<strong>We're sorry but <%= htmlWebpackPlugin.options.title %> doesn't work properly without JavaScript enabled. Please enable it to continue.</strong>
</noscript>
<div id="app"></div>
</body>
</html>
设置页面图表和标题
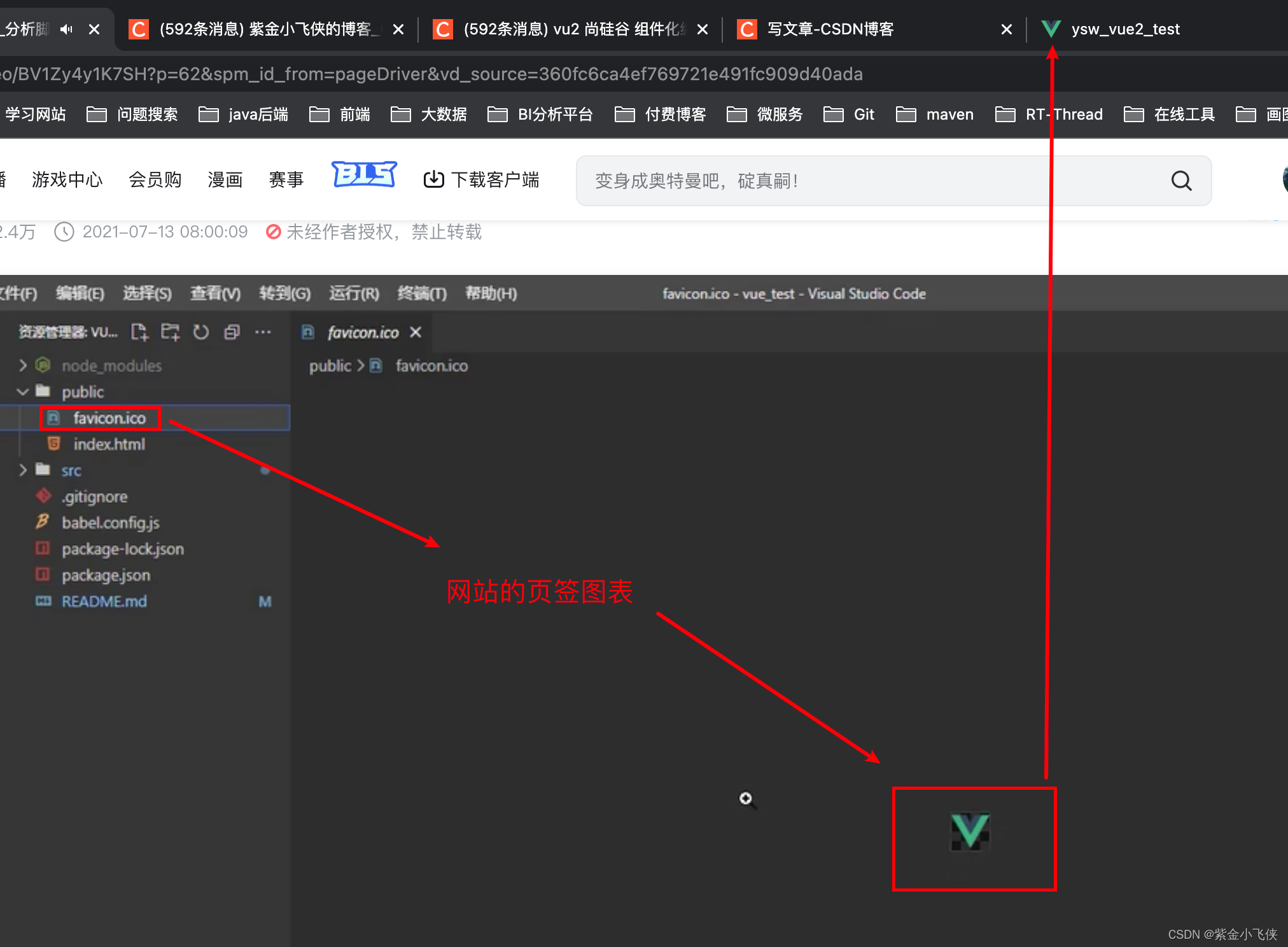
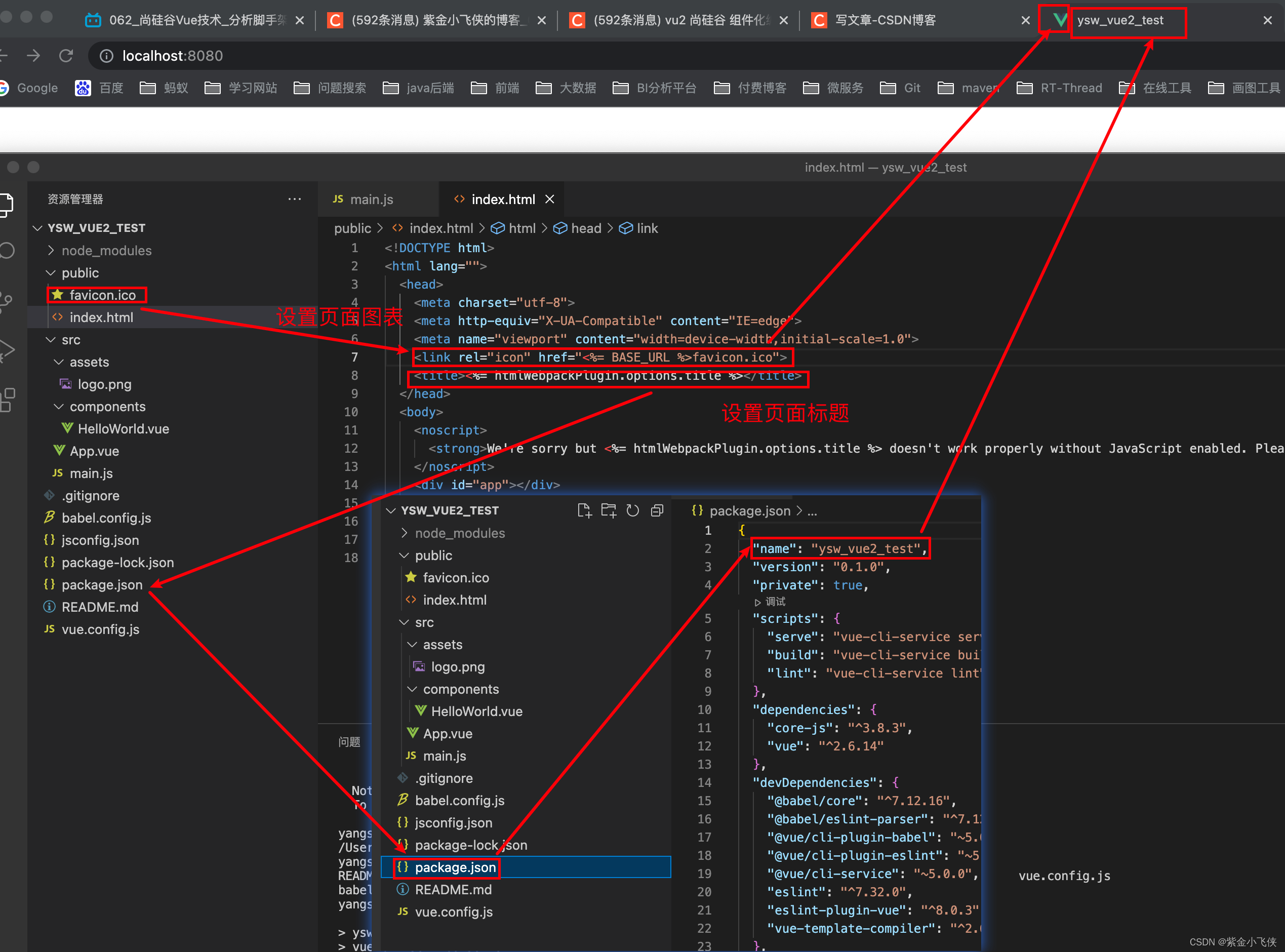
关于不同版本的Vue
- vue.js与vue.runtime.xxx.js的区别:
- vue.js是完整版的Vue,包含:核心功能 + 模板解析器。
- vue.runtime.xxx.js是运行版的Vue,只包含:核心功能;没有模板解析器。
- 因为vue.runtime.xxx.js没有模板解析器,所以不能使用template这个配置项,需要使用render函数接收到的createElement函数去指定具体内容。
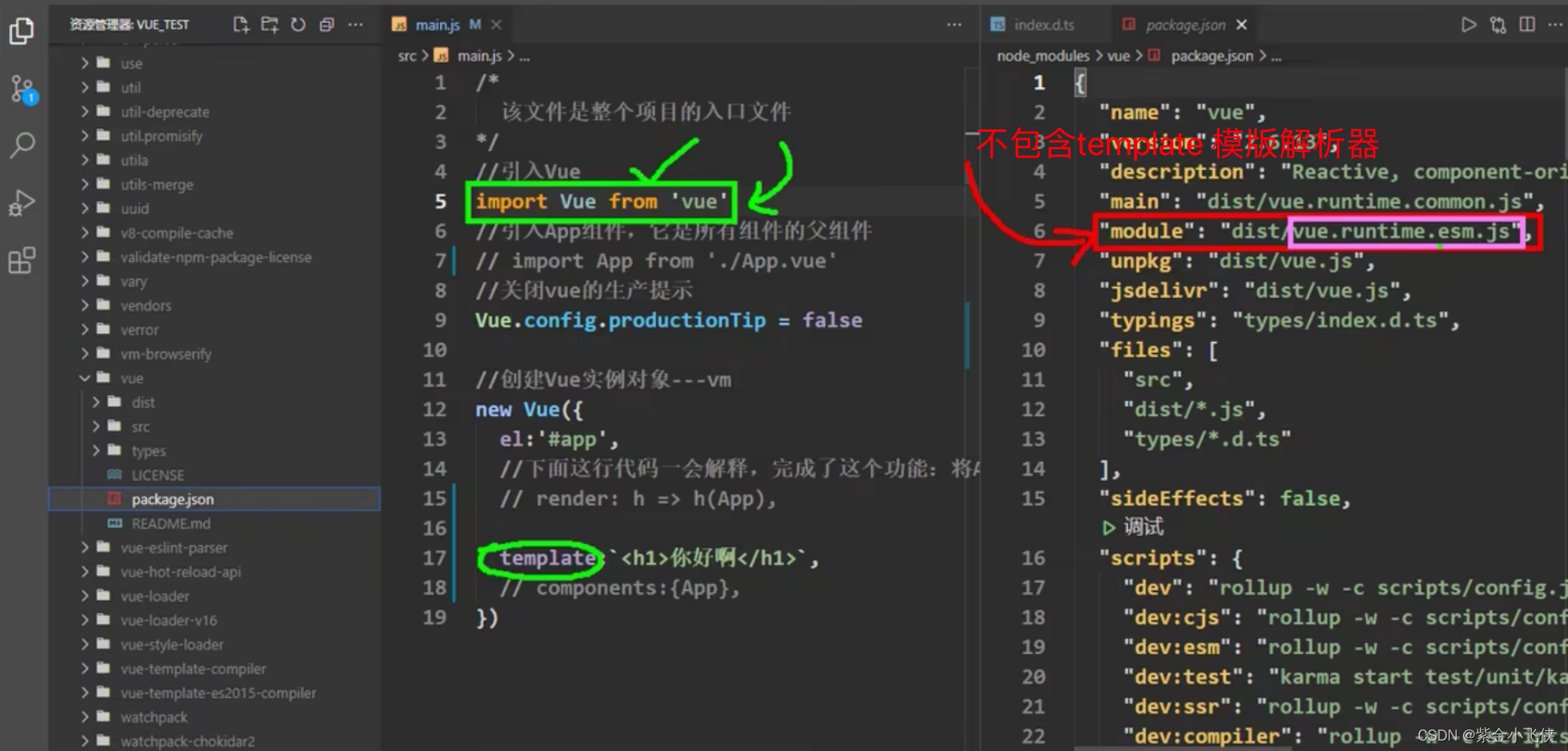 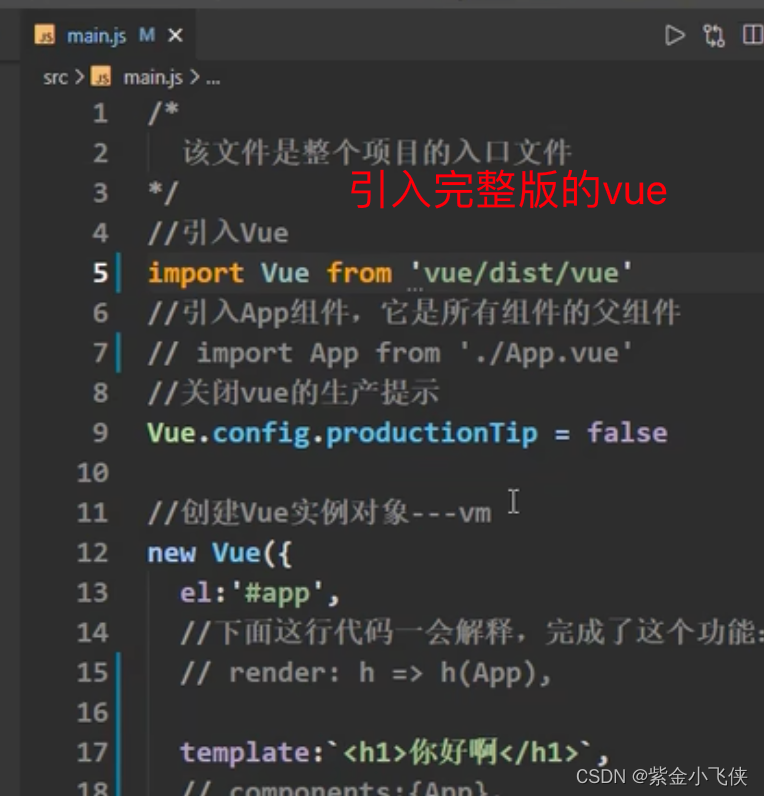 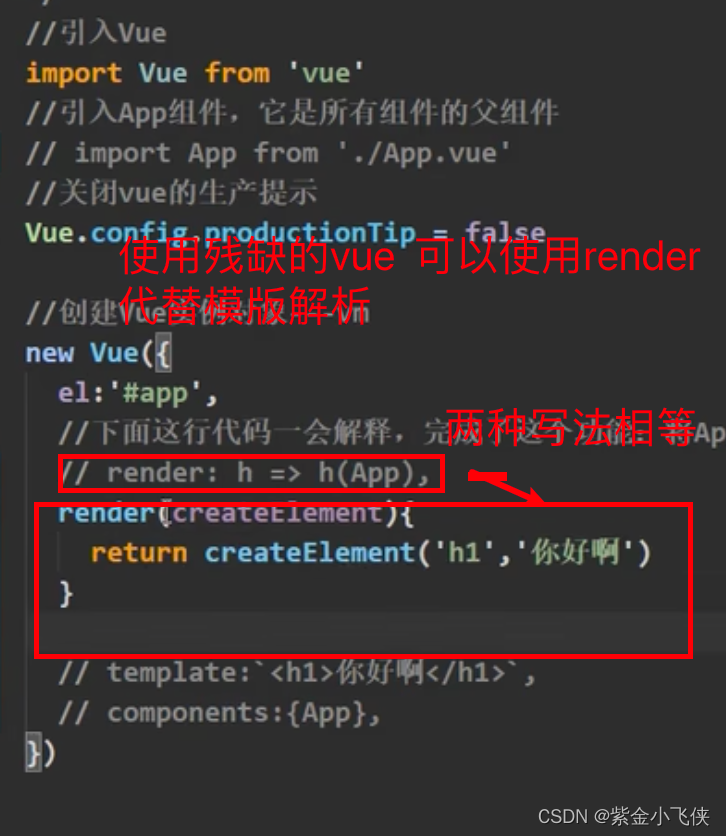
props配置项
-
功能:让组件接收外部传过来的数据 -
传递数据:<Demo name="xxx"/> -
接收数据:
-
第一种方式(只接收):props:['name'] -
第二种方式(限制类型):props:{name:String} -
第三种方式(限制类型、限制必要性、指定默认值):
props:{
name:{
type:String,
required:true,
default:'老王'
}
}
> 备注:props是只读的,Vue底层会监测你对props的修改,如果进行了修改,就会发出警告,若业务需求确实需要修改,那么请复制props的内容到data中一份,然后去修改data中的数据。
<template>
<div>
<Student name="李四" sex="女" :age="18"/>
</div>
</template>
<script>
import Student from './components/Student'
export default {
name:'App',
components:{Student}
}
</script>
<template>
<div>
<h1>{{msg}}</h1>
<h2>学生姓名:{{name}}</h2>
<h2>学生性别:{{sex}}</h2>
<h2>学生年龄:{{myAge+1}}</h2>
<button @click="updateAge">尝试修改收到的年龄</button>
</div>
</template>
<script>
export default {
name:'Student',
data() {
console.log(this)
return {
msg:'我是一个尚硅谷的学生',
myAge:this.age
}
},
methods: {
updateAge(){
this.myAge++
}
},
props:{
name:{
type:String,
required:true,
},
age:{
type:Number,
default:99
},
sex:{
type:String,
required:true
}
}
}
</script>
|