vue-router:vue的一个插件库,专门用来实现SPA应用
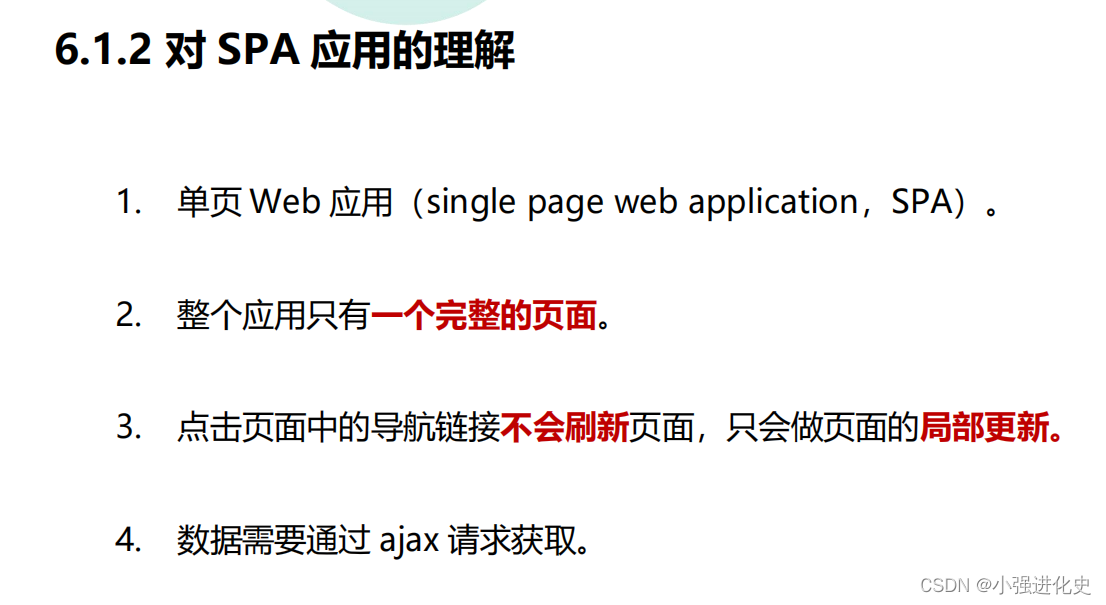
1、vue-router属于插件库,要在Terminal中npm i vue-router,并在main.js文件中引入vue-router,并use一下VueRouter 2、在components文件夹下创建router文件夹,并在router文件夹下创建index.js,用于创建整个应用的路由器,引入需要显示的组件及路由,创建并暴露一个路由器 router\index.js
//该文件专门用于创建整个应用的路由器
import VueRouter from "vue-router";
import About from "@/components/About";
import Home from "@/components/Home";
//创建并暴露一个路由器
export default new VueRouter({
routes:[
{
path:'/about',
component:About
},
{
path:'/home',
component:Home
}
]
})
3、在main.js中导入创建的路由器,在App.vue文件中,
<!-- vue中借助router-link标签实现路由的切换-->
<router-link class="list-group-item" active-class="active" to="/about">About</router-link>
<router-link class="list-group-item" active-class="active" to="/home">Home</router-link>
main.js
import Vue from 'vue'
import App from './App.vue'
import 'bootstrap/dist/css/bootstrap.css'
//引入vue-router
import VueRouter from "vue-router";
//引入路由器
import router from "@/router";
Vue.config.productionTip = false
//应用插件
Vue.use(VueRouter)
new Vue({
render: h => h(App),
router:router
}).$mount('#app')
App.vue
<template>
<div>
<div class="row">
<div class="col-xs-offset-2 col-xs-8">
<div class="page-header"><h2>Vue Router Demo</h2></div>
</div>
</div>
<div class="row">
<div class="col-xs-2 col-xs-offset-2">
<div class="list-group">
<!-- 原始html中我们使用a标签实现页面的跳转-->
<!-- <a class="list-group-item active" href="./about.html">About</a>-->
<!-- <a class="list-group-item" href="./home.html">Home</a>-->
<!---->
<!-- vue中借助router-link标签实现路由的切换-->
<router-link class="list-group-item" active-class="active" to="/about">About</router-link>
<router-link class="list-group-item" active-class="active" to="/home">Home</router-link>
</div>
</div>
<div class="col-xs-6">
<div class="panel">
<div class="panel-body">
<!-- 路由器的视图,类似插槽作用——指定组建的呈现位置-->
<router-view></router-view>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
import Home from "@/components/Home";
import About from "@/components/About";
import HelloWorld from './components/HelloWorld.vue'
export default {
name: 'App',
components: {
Home,
About
}
}
</script>
<style>
</style>
SPA页面前进后退??? 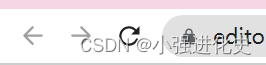 (一)可通过栈,指针的移动来往返页面,将页面push压栈。【router默认】 (二)replace模式,在新页面进栈的同时,将就页面销毁,替换 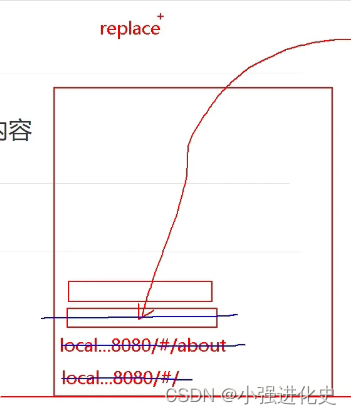 修改replace模式,直接在中写入replace 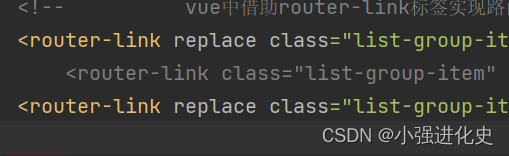
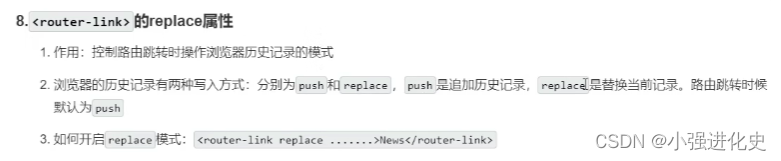
编程式路由导航
即不使用 
<router-link active-class="active" :to="{
path:'/home/news/denews',
query:{
title:item.title,
content:item.content
}
预防有其他需求要求,及并非时使用  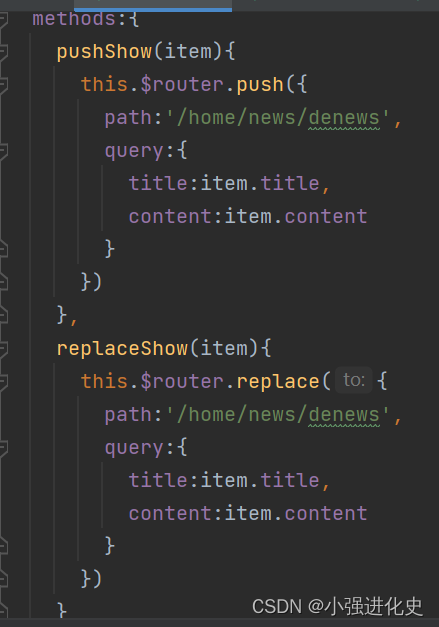 相关API: (一) this.
r
o
u
t
e
r
.
b
a
c
k
(
)
后退(二)
t
h
i
s
.
router.back() 后退 (二) this.
router.back()后退(二)this.router.forward() 前进 (三) this.$router.go(1) // 里面参数如果参数为正数n,则前进n步,若为负数,则后退n步 (四) 入栈式网页,不销毁
this.$router.push({
path:'/home/news/denews',
query:{
title:item.title,
content:item.content
}
})
(五) 点击下一网页时,销毁前一网页
this.$router.replace({
path:'/home/news/denews',
query:{
title:item.title,
content:item.content
}
})
vue-router特有的生命周期
组件被激活 activated() {} 组件失活 deactivated() {} 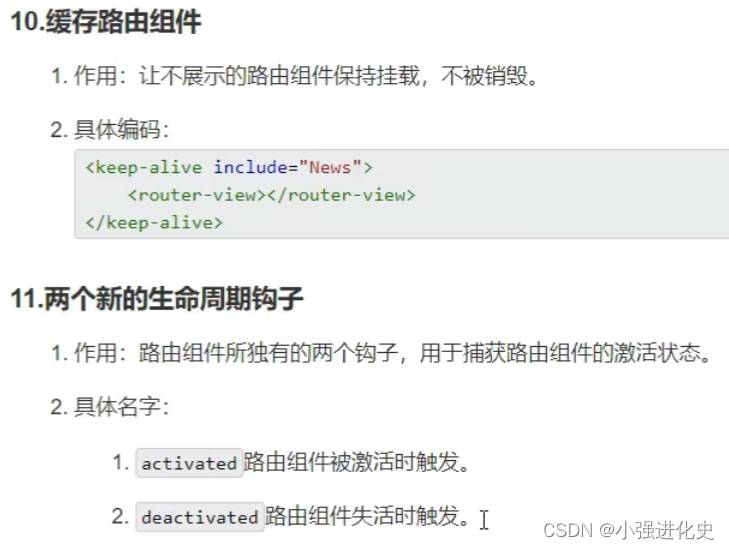
全局路由守卫
在路由配置文件内改写:router/index.js
//该文件专门用于创建整个应用的路由器
import VueRouter from "vue-router";
import About from "@/pages/About";
import Home from "@/pages/Home";
import News from "@/pages/News";
import Message from "@/pages/Message";
import Detail from "@/pages/Detail";
import Denews from "@/pages/Denews";
import Novels from "@/pages/Novels";
import Articials from "@/pages/Articials";
import NovelModule from "@/pages/NovelModule";
//创建并暴露一个路由器
const router=new VueRouter({
routes:[
//一级路由
{
name:'guanyu',
path:'/about',
component:About,
meta:{title:'关于'},
children:[
{
name:'xiaoshuo',
path: 'novels',
component: Novels,
meta:{
isAuth:false,
title:'小说'
},
children: [
{
path:'novelmoudle',
component:NovelModule
}
]
},
{
name:'wenzhang',
path: 'articials',
component: Articials,
meta:{
isAuth:false,
title:'文章'
},
}
]
},
{
path:'/home',
component:Home,
children:[
//子路由path 不需要加/
{
path:'news',
component:News,
children:[
{
path: 'denews',
component: Denews
}
]
},
{
path:'message',
component:Message,
children:[
{
// name:'xiangqing',
//params传参需要先在path中占位
path:'detail/:id/:title',
component:Detail,
//props的第一种配置方法,值为对象,该对象中的所有key-value都会以props的形式传给Detail组件
/*props:{
a:1,b:'hello'
}*/
//props的第二种配置方法,值为布尔值,若布尔值为真,就会把该路由组件收到的所有params参数,以props的形式传给Detail组件
// props:true
//props的第三种配置方法,值为函数该路由组件收到的所有params参数,以props的形式传给Detail组件
props($route){
return {id:$route.id,title:$route.title}}
}
]
}
]
}
]
})
//全局前置路由守卫——初始化的时候被调用、每次路由切换之前被调用
router.beforeEach((to,from,next)=>{
console.log('前置路由守卫',to,from)
if (to.meta.isAuth){//判断是否需要权限
if (localStorage.getItem('school')==='atguigu'){
next()
}
}else {
next()
}
})
//全局后置路由守卫——初始化的时候被调用、每次路由切换之后被调用
router.afterEach((to,from)=>{
console.log('后置路由守卫')
document.title=to.meta.title || '啥?'
})
export default router
独享路由守卫
在路由配置文件内改写:router/index.js 只有Novels组件判断是否设置权限 独享路由守卫只有前置,没有后置
name:'guanyu',
path:'/about',
component:About,
meta:{title:'关于'},
children:[
{
name:'xiaoshuo',
path: 'novels',
component: Novels,
meta:{
isAuth:false,
title:'小说'
},
beforeEnter:(to, from, next) =>{
console.log('前置路由守卫',to,from)
if (to.meta.isAuth){//判断是否需要权限
if (localStorage.getItem('school')==='atguigu'){
next()
}
}else {
next()
}
},
组件内路由守卫
在组件内写 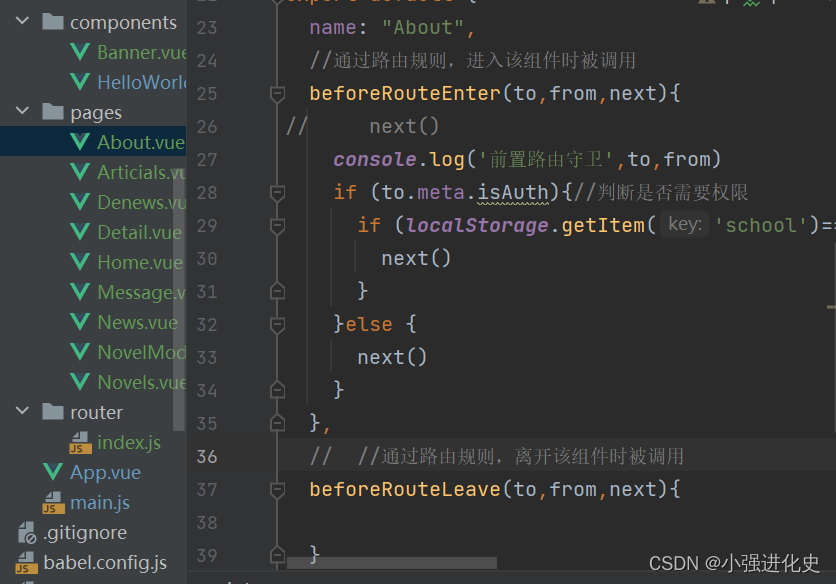
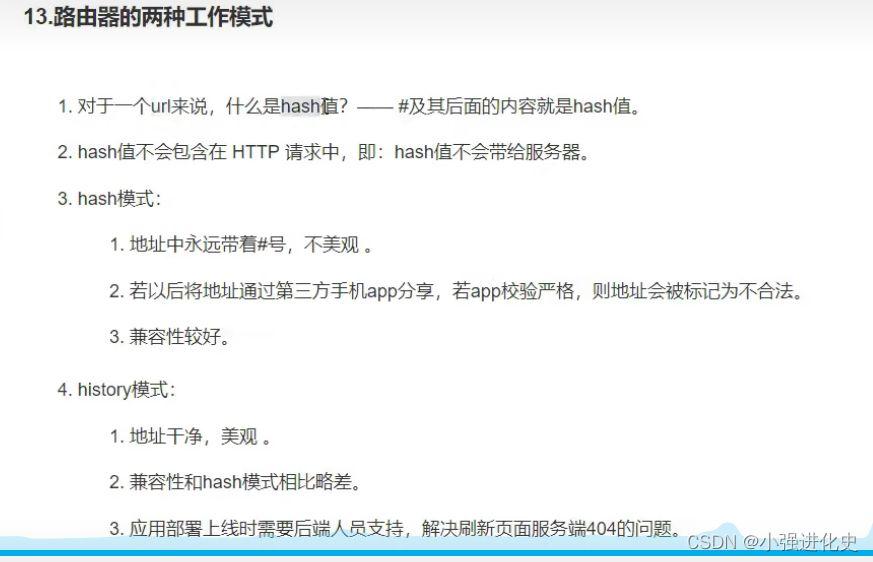
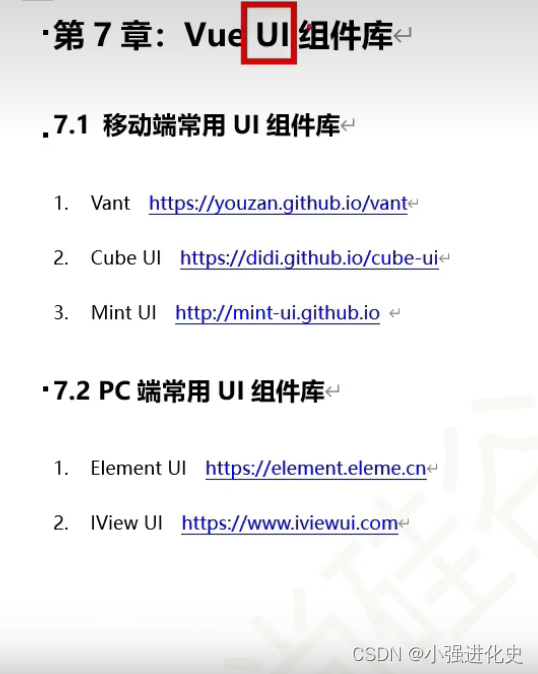
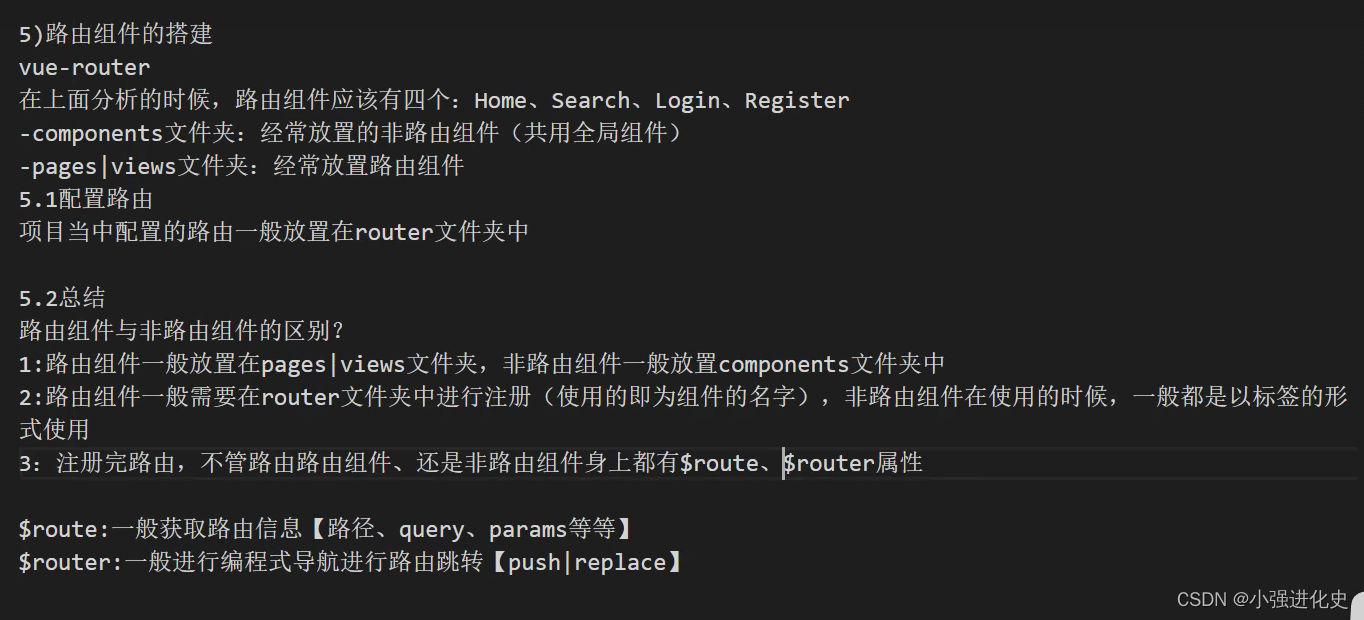 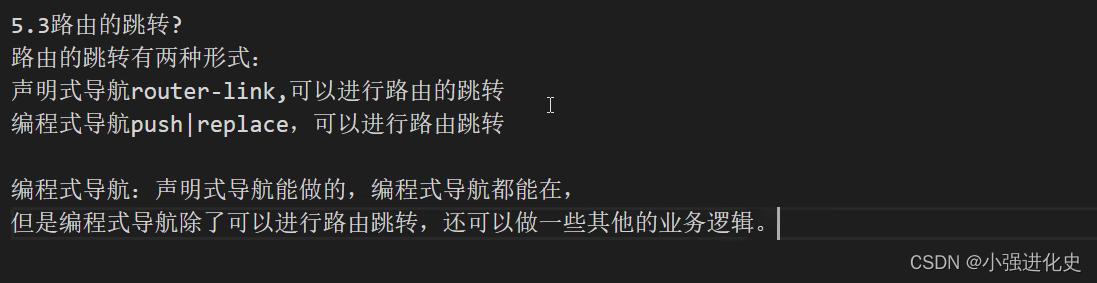 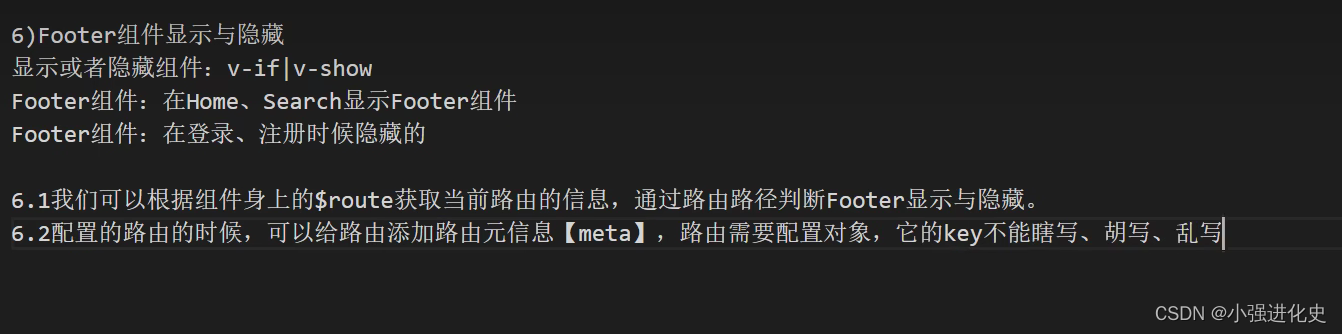 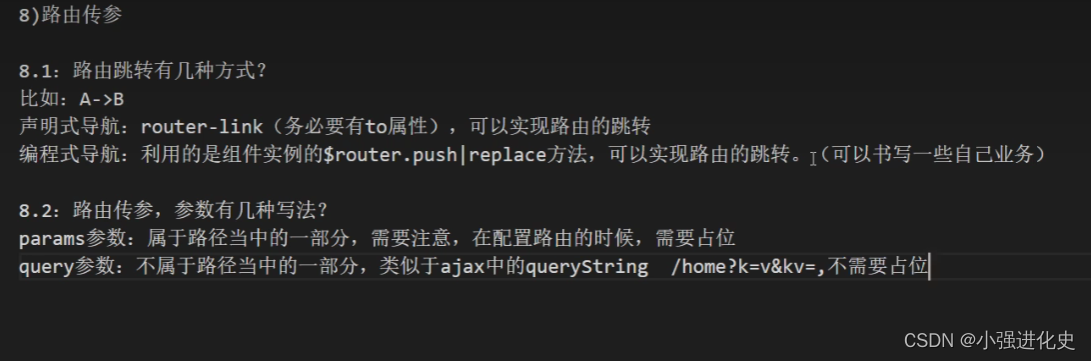
|