以本人写的一个vue抽奖页面为例:
<template>
<div id="indexDiv">
<div id="header">
<h1 id="title">{{ msg }}</h1>
</div>
<div id="body">
<h1 id="title">{{randomList}}</h1>
</div>
<div id="foot">
<button @click="setTimer()">开始</button>
<button @click="cleanTimer()">停止</button>
</div>
</div>
</template>
<script>
import {getArray} from '../../api/randomList.js'
export default {
name: 'HelloWorld',
props: {
msg: String
},
data () {
return {
randomList: '点击按钮开始抽奖',
timer: ''
}
},
methods: {
beginRandomList(list){
console.log("开始抽奖");
let num = parseInt(Math.random()*list.length);
if(num < 0){
num = 0;
}else if(num > list.length -1){
num = list.length - 1;
}
this.randomList = list[num];
},
setTimer(){
this.timer = setInterval(() => {this.beginRandomList(getArray());}, 50);
},
cleanTimer(){
clearInterval(this.timer);
}
},
components: {
},
created () {
},
mounted () {
console.log("indexVue");
console.log(getArray());
},
watch: {
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style scoped>
#indexDiv {
background-image: url(../../assets/random.jpeg);
background-size: 100% 100%;
width: 100%;
height: 100%;
position: fixed;
top: 0;
bottom: 0;
margin: 0;
padding: 0;
}
#title {
color: yellow;
}
#header {
height: 20%;
}
#body {
height: 60%;
font-size: 40px;
}
#foot {
height: 20%;
}
button {
background-image: url(../../assets/button.png);
background-size: 100% 100%;
margin: 10px;
color: gray;
font-weight:500;
font-size: 25px;
padding: 10px;
border: 1px solid white;
border-radius: 10px;
outline: none;
width: 100px;
}
</style>
一、template标签
其中可以放置页面的主要内容,例如增加div标签、h1标签等。
二、style标签
其中可以放置本页面需要用到的样式。 <style scoped> ,使用scoped后,可以确保样式只用于本vue文件。 基本语法是,# 是按id指定样式;. 是按class指定样式;直接用文字的话是按标签指定样式(例如上方指定button 标签的样式)。
其中,#indexDiv里是with:100%,height:100%的用法,需要position: fixed后才会生效。
三、script标签
其中可以放置本页面需要用到的js内容。 大部分都配置在了export default 中。其中:
1.name
自己起的vue组件的名称,其它地方需要名称时用。
2.props
声明其它页面传入这个页面的参数;页面使用时可以用{{}}。
3.data
声明这个页面需要用到的参数;页面使用时可以用{{}},方法中使用时可以用this。
4.methods
声明这个页面需要用到的js方法;如果一个方法中需要调用另一个方法,用this指定另一个方法。
5.components
声明这个页面需要用到的其它页面组件;可以先import一个vue文件,然后在这里申明,然后就可以在页面中使用了。 例如:
import HelloWorld from './pages/index/Index.vue'
export default {
name: 'App',
components: {
HelloWorld
}
}
<template>
<HelloWorld msg="MyVue"/>
</template>
6.created
页面刚创建时,就会执行这个方法;可以在其中放些初始化用的js方法。
7.mounted
页面刚出现时,就会执行这个方法。(区别是,例如,返回上一个页面时,如果有缓存,那么上一个页面就不会执行created方法,但是会执行mounted方法)
8.watch
可以监听某个数据是否发生了变化,如果变化后就会执行这个方法。
9.引入其它js文件中的方法
例如,上方有:
import {getArray} from '../../api/randomList.js'
这个的意思是从randomList.js中,引入getArray方法; 然后就可以使用了:
console.log(getArray());
对应的randomList.js中,需要使用export 语句导出这个方法,才能被其它页面import到:
export function getArray(){
var arr = ["a","b","c","d","e","f","g"];
return arr;
}
10.附:vue抽奖页面效果图
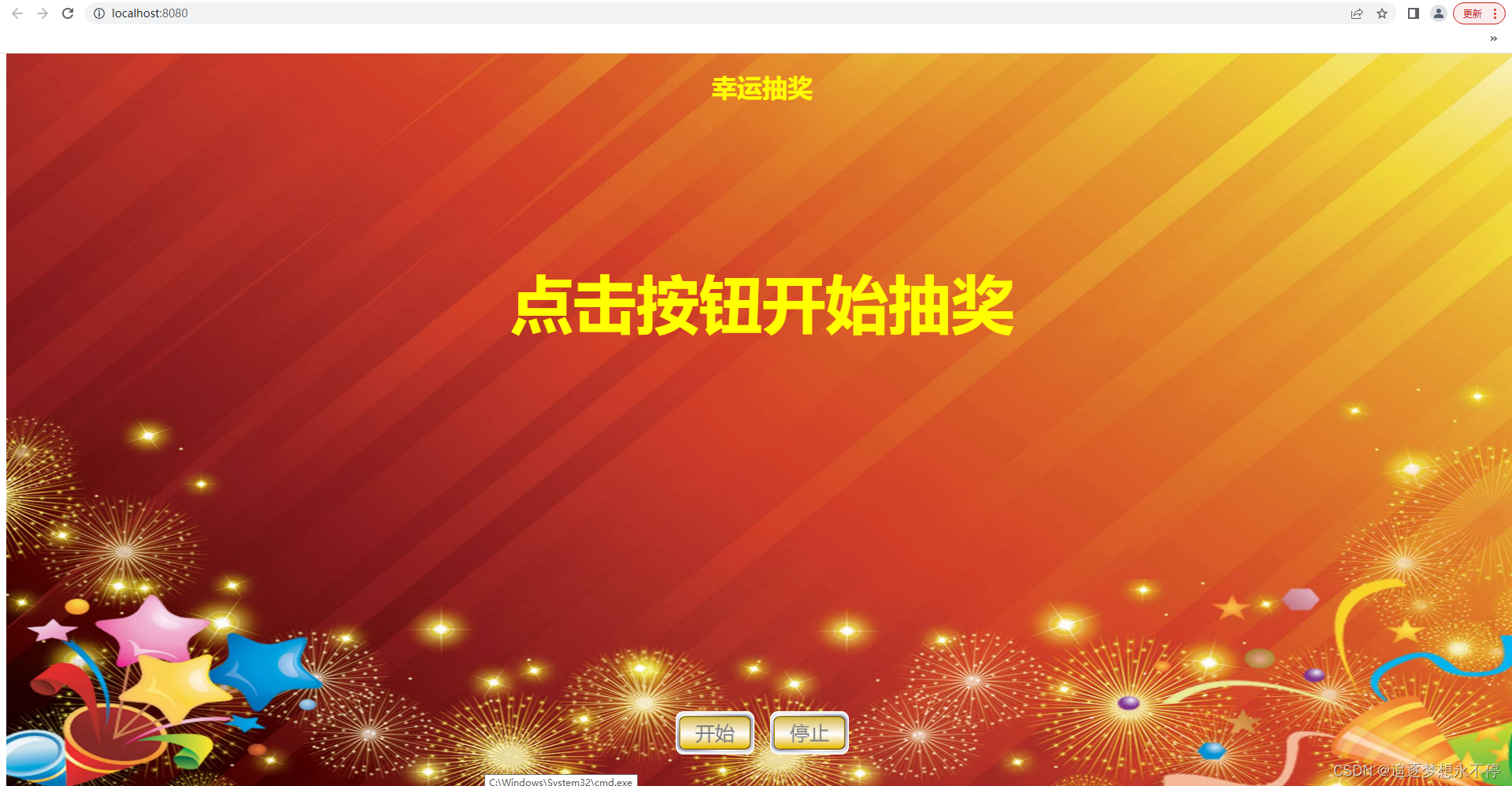
|