目录
受控组件
非受控组件
?组件应用传递
需求:获取子组件中某个DOM实例
1.类组件形式
2.函数组件形式
调用React.forwardRef()引用转发:引用地址的转发,自动传递ref的一种技术。
受控组件
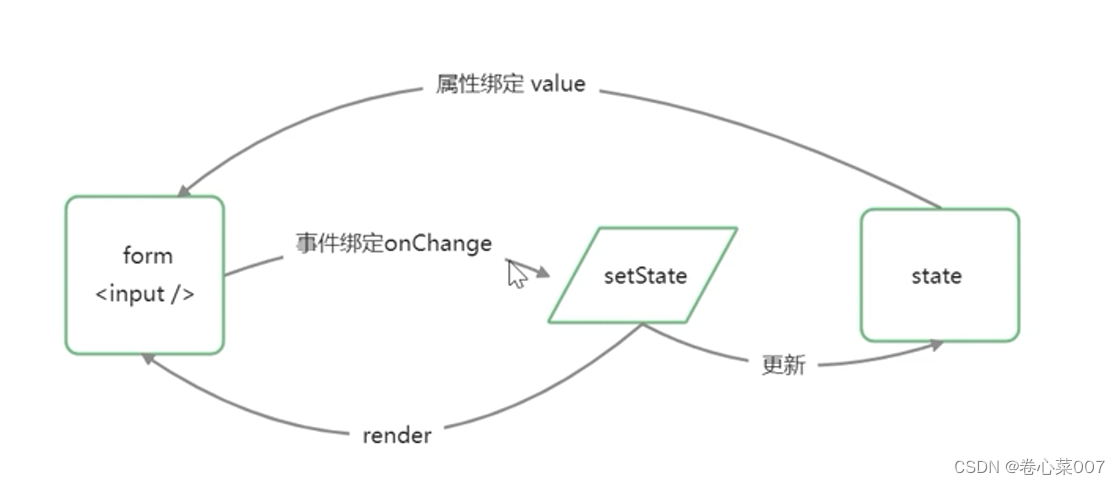
非受控组件
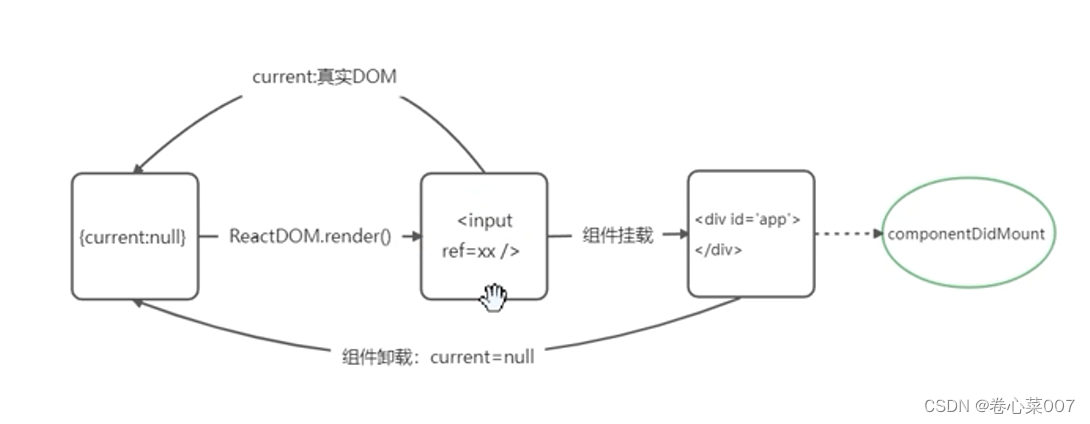
?组件应用传递
//类组件
class ChildInput extends React.Component{
? ? ? ? render(){
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <h2>child input</h2>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <inpur type="text" />
????????????????????????</div>
????????????????)
????????}
}
class FatherForm extends React.Componet{
? ? ? ? constructor(){
? ? ? ? ? ? ? ?super()
? ? ? ? ? ? ? ? this.inputRef = React.createRef() //容器:{current:null}
? ? ? ? }
? ? ? ? callbackRef=(e)=>{
? ? ? ? ? ? ? ? this.inputRef = e
????????}
? ? ? ? handleClick=()=>{
? ? ? ? ? ? ? ? console.log(this.inputRef)//获取到子组件实例

? ? ? ? ? ? ? ? console.log(this.inputRef.current) //获取不到子组件中DOM操作
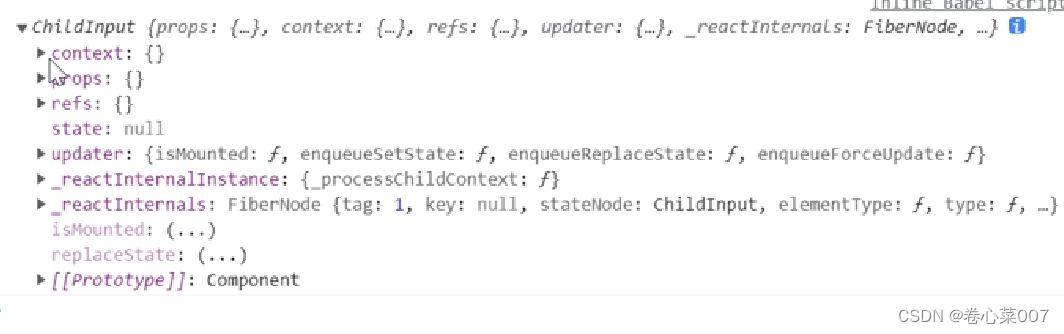
????????}
? ? ? ? render(){
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <ChildInput? callbackRef = {this.callbackRef} />
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <button onClick={this.handleClick}>commit</button>
? ? ? ? ? ? ? ? ? ? ? ? </div>
????????????????)
????????}
}
需求:获取子组件中某个DOM实例
通过回调函数的方式:给子组件传递一个回调函数callback,子组件通过ref={callback},把子组件具体DOM挂载在父组件上,使得父组件可以获取子组件的值。
1.类组件形式
class ChildInput extends React.Component{
? ? ? ? //this.props = props 自动操作
? ? ? ? render(){
? ? ? ? ? ? ? ? console.log(this.props)
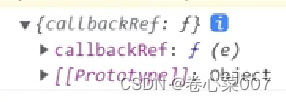
? ? ? ? ? ? ? ? ?console.log(this.props.callbackRef)
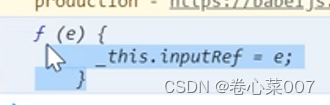
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <h2>child input</h2>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <inpur type="text" ref={this.props.callbackRef}/>
????????????????????????</div>
????????????????)
????????}
}
class FatherForm extends React.Componet{
? ? ? ? callbackRef=(e)=>{
? ? ? ? ? ? ? ? console.log(this) //父组件实例
? ? ? ? ? ? ? ? this.inputRef = e
????????}
? ? ? ? handleClick=()=>{
????????????????console.log(this) //父组件实例
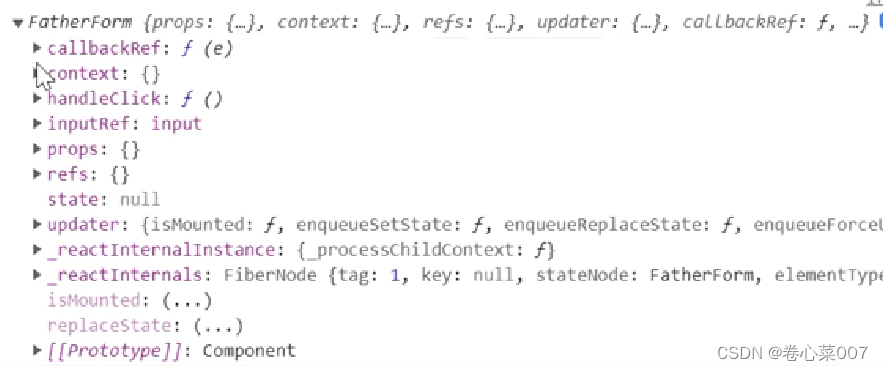
? ? ? ? ? ? ? ? ?//需求:获取子组件中某个DOM
? ? ? ? ? ? ? ? this.inputRef.focus()
????????}
? ? ? ? render(){
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <ChildInput? callbackRef = {this.callbackRef} />
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <button onClick={this.handleClick}>commit</button>
? ? ? ? ? ? ? ? ? ? ? ? </div>
????????????????)
????????}
}
2.函数组件形式
?注意:函数组件没有this,没有实例的概念,传值依靠props
function ChildInput(props){
? ? ? ? render(){
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <h2>child input</h2>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <inpur type="text" ref={props.callbackRef}/>
????????????????????????</div>
????????????????)
????????}
}
class FatherForm extends React.Componet{
? ? ? ? callbackRef=(e)=>{
? ? ? ? ? ? ? ? this.inputRef = e
????????}
? ? ? ? handleClick=()=>{
? ? ? ? ? ? ? ? this.inputRef.focus()
????????}
? ? ? ? render(){
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <ChildInput? callbackRef = {this.callbackRef} />
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <button onClick={this.handleClick}>commit</button>
? ? ? ? ? ? ? ? ? ? ? ? </div>
????????????????)
????????}
}
调用React.forwardRef()引用转发:引用地址的转发,自动传递ref的一种技术。
?function ChildInput(props){
? ? ? ? render(){
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <h2>child input</h2>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <inpur type="text" ref={props.callbackRef}/>
????????????????????????</div>
????????????????)
????????}
}
?function ChildInput2(props,ref){ //子组件添加ref参数接
? ? ? ? render(){
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <h2>child input</h2>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <inpur type="text" ref={ref}/> // 地址=>{current:null}=>{current:input}
????????????????????????</div>
????????????????)
????????}
}
const RefChildInput2 = React.forwardRef(ChildInput2)
class FatherForm extends React.Componet{
? ? ? ? constructor(){
? ? ? ? ? ? ? ? super()
? ? ? ? ? ? ? ? this.inputRef1 = React.createRef()
????????}
? ? ? ? callbackRef=(e)=>{
? ? ? ? ? ? ? ? this.inputRef2 = e
????????}
? ? ? ? handleClick=()=>{
? ? ? ? ? ? ? ? //点击input2自动获取焦点
? ? ? ? ? ? ? ? this.inputRef2.focus()
? ? ? ? ? ? ? ? //点击input1自动获取焦点
? ? ? ? ? ? ? ? console.log(thiis)
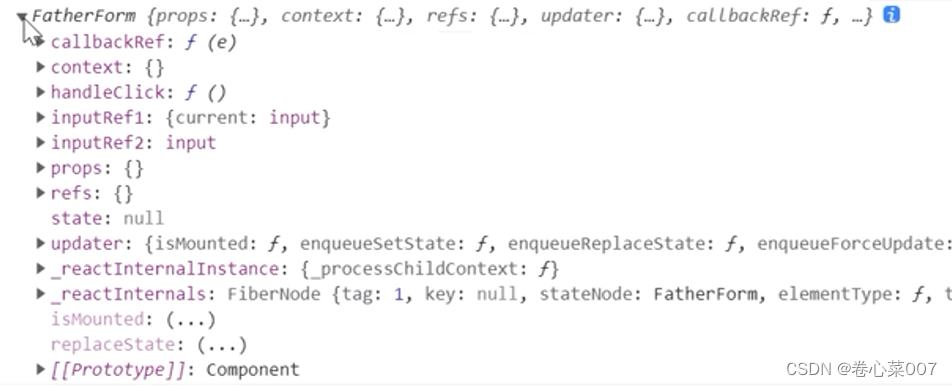
?
? ? ? ? ? ? ? ? this.inputRef1.current.focus()
????????}
? ? ? ? render(){
? ? ? ? ? ? ? ? return(
? ? ? ? ? ? ? ? ? ? ? ? <div>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <ChildInput? callbackRef = {this.callbackRef} />
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <RefChildInput2 ref={this.inputRef1}/>
? ? ? ? ? ? ? ? ? ? ? ? ? ? ? ? <button onClick={this.handleClick}>commit</button>
? ? ? ? ? ? ? ? ? ? ? ? </div>
????????????????)
????????}
}
|