本文不会拆步骤对源码进行实现,只介绍vue原理及相关核心实现思想。在之前的四篇文章中已对vue进行响应实现。需要可阅读相关文章:
?? 理解Vue的设计思想
MVVM框架的三要素:数据响应式、模板引擎及其渲染。 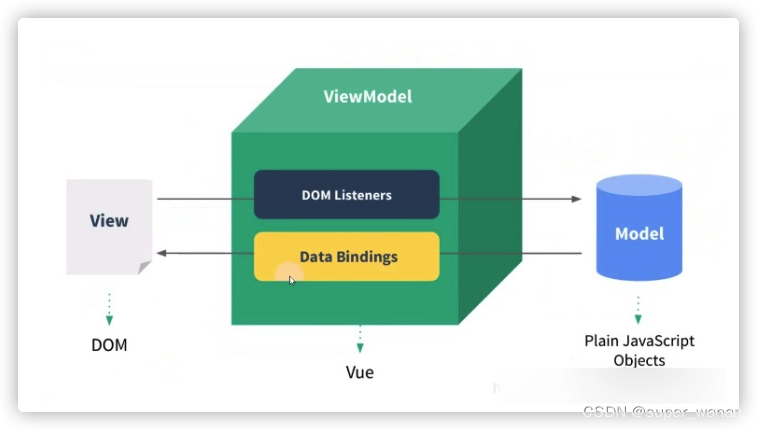
数据响应式:监听数据变化并在视图中更新
- Object.defineProperty()
- Proxy
模版引擎:提供描述视图的模版语法
- 插值:{{}}
- 指令:v-bind,v-on,v-model,v-for,v-if
渲染:如何将模板转换为html
🌺数据响应式原理
数据变更能够响应在视图中,就是数据响应式。vue2中利用 Object.defineProperty() 实现变更检测。
- 遍历需要响应化的对象
- 解决嵌套对象问题
- 解决赋的值是对象的情况
- 解决添加/删除了新属性无法检测的情况
- 解决数组数据的响应化
??Vue中的数据响应化
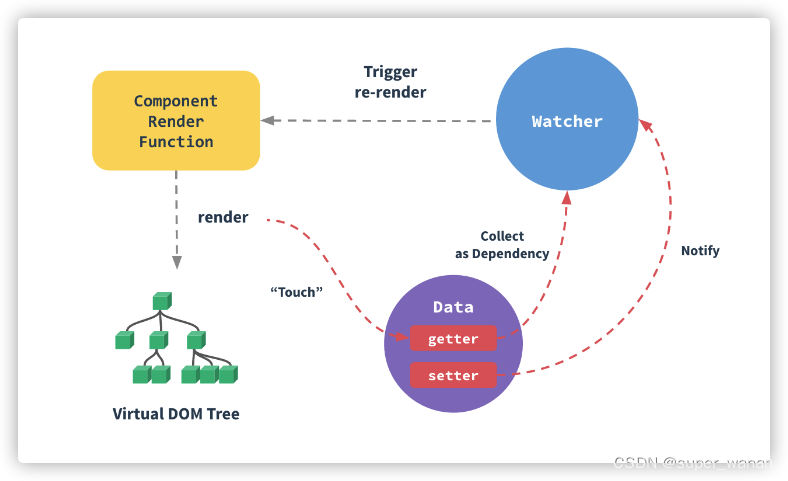
原理分析:
- newVue()首先执行初始化,对data执行响应化处理,这个过程发生在Observer中
- 同时对模板执行编译,找到其中动态绑定的数据,从data中获取并初始化视图,这个过程发生在 Compile中
- 同时定义一个更新函数和Watcher,将来对应数据变化时Watcher会调用更新函数
- 由于data的某个key在一个视图中可能出现多次,所以每个key都需要一个管家Dep来管理多个
Watcher - 将来data中数据一旦发生变化,会首先找到对应的Dep,通知所有Watcher执行更新函数
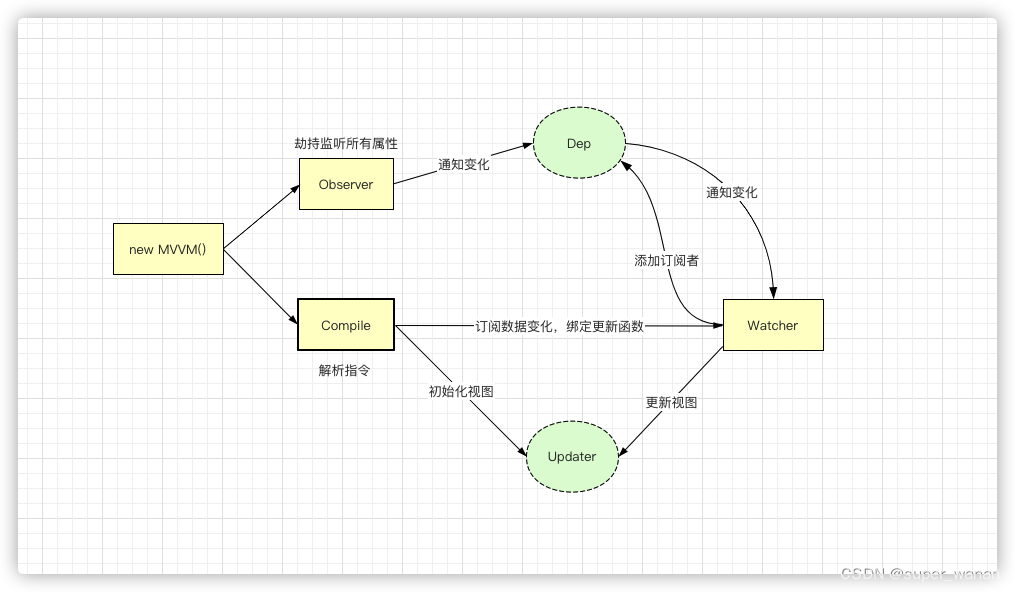
涉及类型介绍:
- Vue:框架构造函数
- Observer:执行数据响应化(分辨数据是对象还是数组)
- Compile:编译模板,初始化视图,收集依赖(更新函数、watcher创建)
- Watcher:执行更新函数(更新dom)
- Dep:管理多个Watcher,批量更新
🐝编译Compile
编译模板中vue模板特殊语法,初始化视图、更新视图 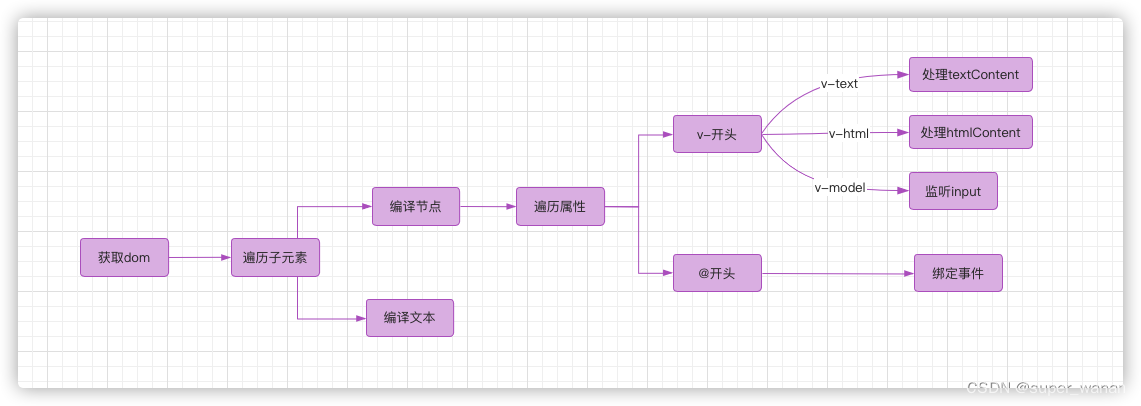
🦜依赖收集
视图中会用到data中某key,这称为依赖。同一个key可能出现多次,每次都需要收集出来用一个 Watcher来维护它们,此过程称为依赖收集。 多个Watcher需要一个Dep来管理,需要更新时由Dep统一通知。 看下面案例,理出思路:
new Vue({
template:
`<div>
<p>{{name1}}</p>
<p>{{name2}}</p>
<p>{{name1}}</p>
<div>`,
data: {
name1: 'name1',
name2: 'name2'
}
});
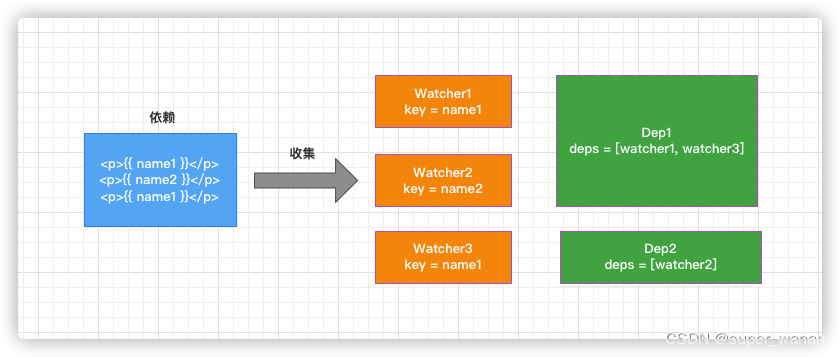
实现思路
- defineReactive时为每一个key创建一个Dep实例
- 初始化视图时读取某个key,例如name1,创建一个watcher1
- 由于触发name1的getter方法,便将watcher1添加到name1对应的Dep中
- 当name1更新,setter触发时,便可通过对应Dep通知其管理所有Watcher更新
👗完整代码
const oldArrayProperty = Array.prototype
const arrayProto = Object.create(oldArrayProperty)
const methodsToPatch = ['push', 'pop', 'unshift', 'shift', 'sort', 'splice', 'reverse']
methodsToPatch.forEach(methodName => {
Object.defineProperty(arrayProto, methodName, {
value () {
console.log('you change the array')
oldArrayProperty[methodName].apply(this, arguments)
}
})
})
function defineReactive (obj, key, val) {
observe(val)
const dep = new Dep()
Object.defineProperty(obj, key, {
get () {
Dep.target && dep.addDep(Dep.target)
return val
},
set (newVal) {
if (newVal !== val) {
console.log('set', key, val)
val = newVal
observe(newVal)
dep.notify()
}
}
})
}
function observe (obj) {
if (typeof obj !== 'object' || obj == null) {
return obj
}
new Observer(obj)
}
class Observer {
constructor (obj) {
if (Array.isArray(obj)) {
Object.setPrototypeOf(obj, arrayProto)
const keys = Object.keys(obj)
keys.forEach(key => observe(obj[key]))
} else {
this.walk(obj)
}
}
walk (obj) {
Object.keys(obj).forEach((key) => {
defineReactive(obj, key, obj[key])
})
}
}
function proxy (vm) {
Object.keys(vm.$data).forEach((key) => {
Object.defineProperty(vm, key, {
get () {
return vm.$data[key]
},
set (v) {
vm.$data[key] = v
}
})
})
}
class MVue {
constructor (options) {
this.$options = options
this.$data = options.data
this.$methods = options.methods
observe(this.$data)
proxy(this)
new Compile(options.el, this)
}
}
class Compile {
constructor (el, vm) {
this.$vm = vm
this.$el = document.querySelector(el)
if (this.$el) {
this.compile(this.$el)
}
}
compile (node) {
const childNodes = node.childNodes
Array.from(childNodes).forEach(n => {
if (this.isElment(n)) {
this.compileElement(n)
if (n.childNodes.length > 0) {
this.compile(n)
}
} else if (this.isInter(n)) {
this.compileText(n)
}
})
}
isElment (node) {
return node.nodeType === 1
}
isInter (n) {
return n.nodeType === 3 && /\{\{(.*)\}\}/.test(n.textContent)
}
isDir (attrName) {
return attrName.startsWith('m-')
}
isEvent (attrName) {
return attrName.startsWith('@')
}
compileElement (node) {
const attrs = node.attributes
Array.from(attrs).forEach(attr => {
const attrName = attr.name
const exp = attr.value
if (this.isDir(attrName)) {
const dir = attrName.substring(2)
this[dir] && this[dir](node, exp)
} else if (this.isEvent(attrName)) {
const event = attrName.substring(1)
node.addEventListener(event, this.$vm.$methods[exp].bind(this.$vm))
}
})
}
update (node, exp, dir) {
const fn = this[dir + 'Updater']
fn && fn(node, this.$vm[exp])
new Watcher(this.$vm, exp, val => {
fn && fn(node, val)
})
}
compileText (n) {
this.update(n, RegExp.$1.trim(), 'text')
}
text (node, exp) {
this.update(node, exp, 'text')
}
textUpdater (node, val) {
node.textContent = val
}
html (node, exp) {
this.update(node, exp, 'html')
}
htmlUpdater (node, val) {
node.innerHTML = val
}
model (node, exp) {
node.value = this.$vm[exp]
node.addEventListener('input', (e) => {
this.$vm[exp] = e.target.value
})
}
}
class Watcher {
constructor (vm, key, updateFn) {
this.vm = vm
this.key = key
this.updateFn = updateFn
Dep.target = this
this.vm[this.key]
Dep.target = null
}
update () {
this.updateFn.call(this.vm, this.vm[this.key])
}
}
class Dep {
constructor () {
this.deps = []
}
addDep (dep) {
this.deps.push(dep)
}
notify () {
this.deps.forEach(dep => dep.update())
}
}
测试代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div id="app">
<p>{{ counter }}</p>
<p m-text="测试"></p>
<p m-text="counter"></p>
<p m-html="desc"></p>
<p @click="test">点击我</p>
<input m-model="desc" />
</div>
</body>
<script src="./index.js"></script>
<script>
const app = new MVue({
el: '#app',
data: {
counter: 1,
desc: '<span style = "color: red">super wanan</span>',
name: 'wanan',
arr: [{
name: 1,
arr: [12]
}]
},
methods: {
test() {
this.counter++;
console.log('进入了', this.counter)
this.arr.push(2)
this.arr[0].arr.push(2)
console.log('进入了', this.arr)
}
},
})
</script>
</html>
|