挂载与卸载
挂载过程
四个方法:
- constructor:构造器
- componentWillMount:渲染前
- render:渲染
- componentDidMount:渲染后
import {Component} from "react";
class App extends Component {
constructor(props) {
super(props);
console.log("constructor")
}
render() {
console.log("render")
return (
<div>
</div>
)
}
componentWillMount() {
console.log('componentWillMount')
}
componentDidMount() {
console.log('componentDidMount')
}
}
export default App;
效果: 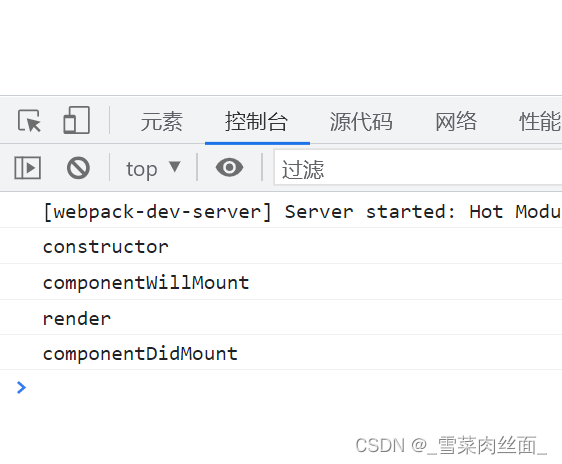
案例:计时器
渲染后开启定时器。
import {Component} from "react";
class App extends Component {
state = {
count: 0
}
render() {
return (
<div>
<h1>{this.state.count}</h1>
</div>
)
}
componentDidMount() {
setInterval(() => {
this.setState({
count: this.state.count + 1
})
}, 1000)
}
}
export default App;
效果:
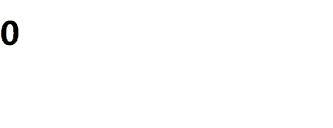
卸载之前
很简单,componentWillUnmount。
import {Component} from "react";
import * as ReactDOM from "react-dom";
class App extends Component {
render() {
return (
<div>
<button onClick={() => {
ReactDOM.unmountComponentAtNode(document.getElementById('app'))
}}>卸载
</button>
</div>
)
}
componentWillUnmount() {
console.log('componentWillUnmount')
}
}
export default App;
效果: 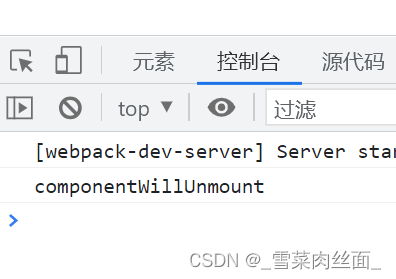
更新
触发更新的情况
setState方法,可以触发更新。这个很简单。
父组件更新时,props发生变化,可以触发更新。 forceUpdate方法,强制更新。
import {Component} from "react";
class A extends Component {
render() {
console.log("render")
return (
<div>{this.props.count || 0}</div>
)
}
}
class App extends Component {
state = {
count: 0
}
render() {
return (
<div>
<A count={this.state.count}/>
<button onClick={() => {
this.setState({
count: this.state.count + 1
})
}}>+
</button>
<button onClick={() => {
this.forceUpdate()
}}>强制更新
</button>
</div>
)
}
}
export default App;
props变化导致更新:
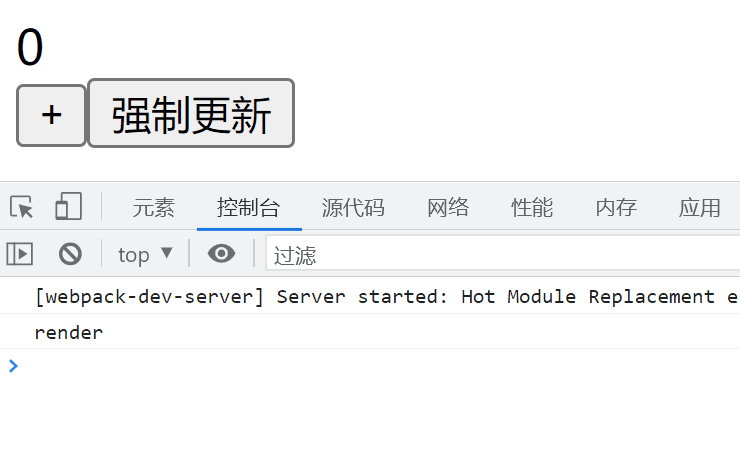 强制更新:
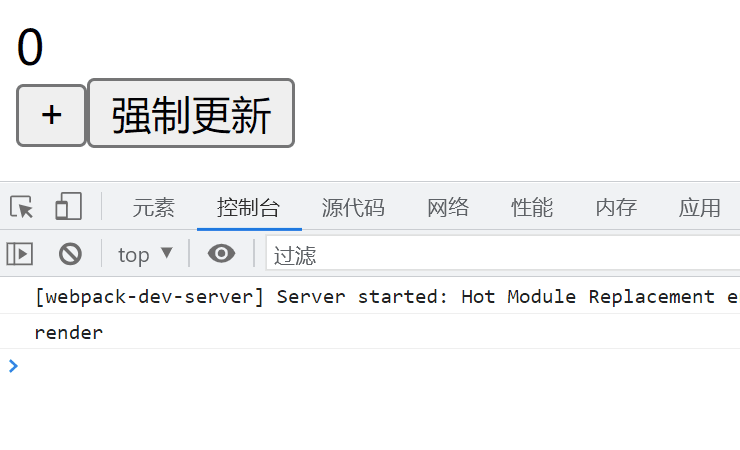
更新前后
很简单:
- componentWillUpdate
- componentDidUpdate
import {Component} from "react";
class App extends Component {
state = {
count: 0
}
render() {
return (
<div>
<h1>{this.state.count}</h1>
<button onClick={() => {
this.setState({
count: this.state.count + 1
})
}}>+
</button>
</div>
)
}
componentWillUpdate(nextProps, nextState, nextContext) {
console.log('componentWillUpdate')
console.log(nextProps)
console.log(nextState)
console.log(nextContext)
}
componentDidUpdate(prevProps, prevState, snapshot) {
console.log('componentDidUpdate')
console.log(prevProps)
console.log(prevState)
console.log(snapshot)
}
}
export default App;
效果:
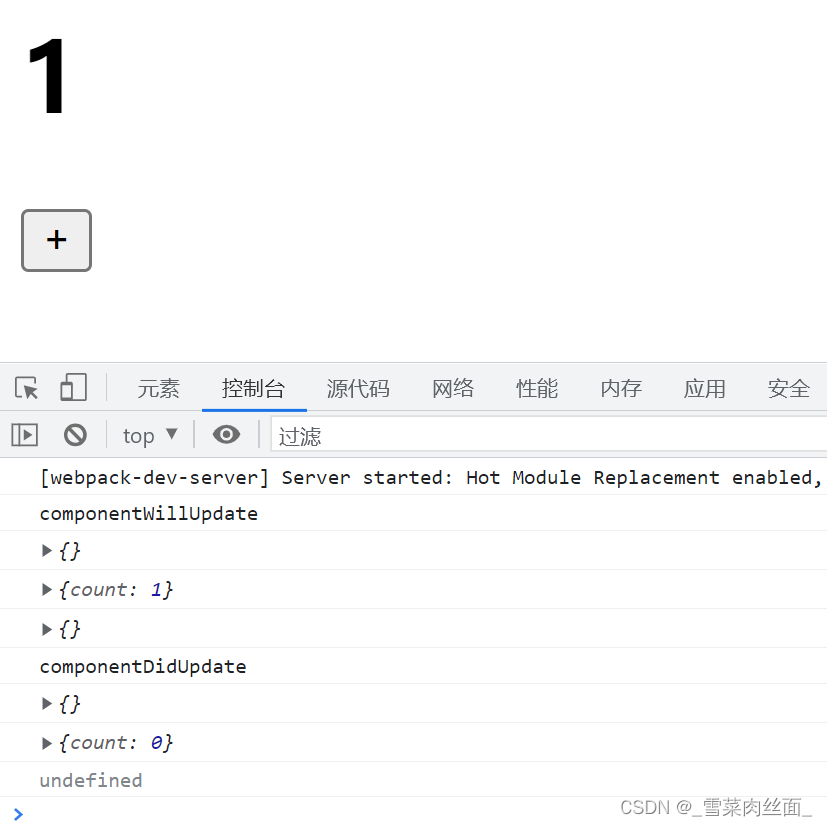
拦截更新
强制更新之外的两种方式,可以由shouldComponentUpdate方法进行拦截判断。 返回假则不更新,返回真则更新。
举例:只有变成偶数的时候才更新。
import {Component} from "react";
class App extends Component {
state = {
count: 0
}
render() {
return (
<div>
<h1>{this.state.count}</h1>
<button onClick={() => {
this.setState({
count: this.state.count + 1
})
}}>+
</button>
</div>
)
}
shouldComponentUpdate(nextProps, nextState, nextContext) {
return nextState.count % 2 === 0;
}
}
export default App;
效果:
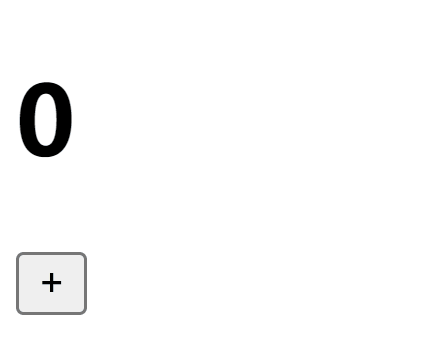
props变化前
父组件中props发生变化导致的更新,会调用一次componentWillReceiveProps方法。
import {Component} from "react";
class A extends Component {
render() {
return (
<div>{this.props.count || 0}</div>
)
}
componentWillReceiveProps(nextProps, nextContext) {
console.log('componentWillReceiveProps')
console.log(nextProps)
console.log(nextContext)
}
}
class App extends Component {
state = {
count: 0
}
render() {
return (
<div>
<A count={this.state.count}/>
<button onClick={() => {
this.setState({
count: this.state.count + 1
})
}}>+
</button>
<button onClick={() => {
this.forceUpdate()
}}>强制更新
</button>
</div>
)
}
}
export default App;
效果:
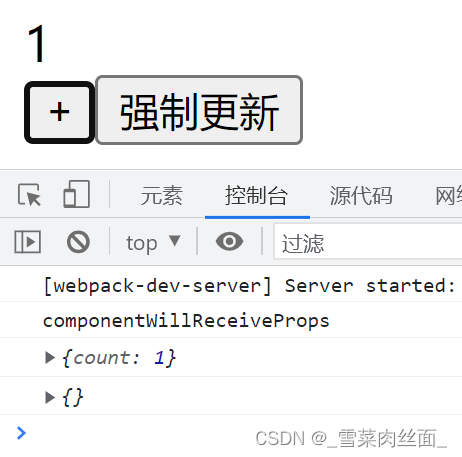
生命周期
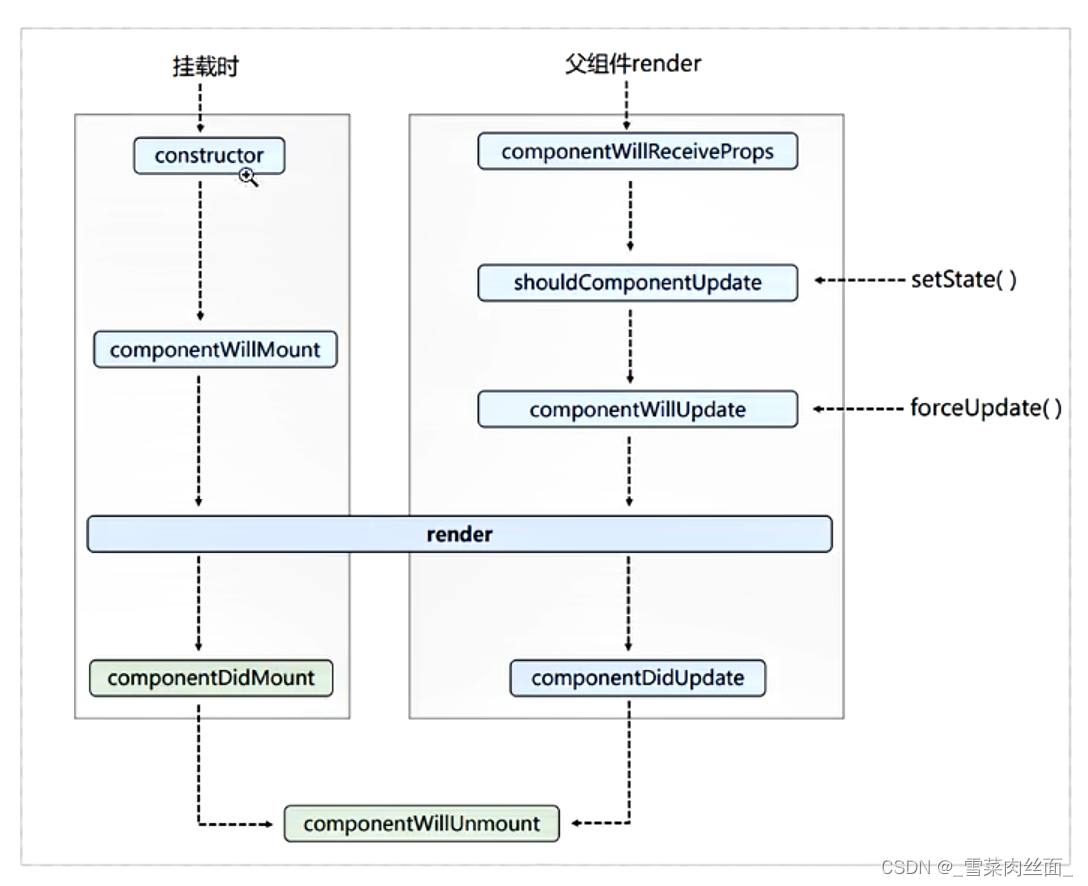
番外:两个新的生命周期方法
官方文档地址:
getDerivedStateFromProps() getSnapshotBeforeUpdate()
|