一、表单验证
1.1 出现表单组件 el-form
这里我们参照官网可以去form组件中kopi,然后进行对应的值改变
<el-dialog :title="title" :visible.sync="editFormVisible" width="30%" @click="closeDialog">
<el-form label-width="120px" :model="editForm" :rules="rules" ref="editForm">
<el-form-item label="文章标题" prop="title">
<el-input size="small" v-model="editForm.title" auto-complete="off" placeholder="请输入文章标题"></el-input>
</el-form-item>
<el-form-item label="文章内容" prop="body">
<el-input size="small" v-model="editForm.body" auto-complete="off" placeholder="请输入内容"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button size="small" @click="closeDialog">取消</el-button>
<el-button size="small" type="primary" class="title" @click="submitForm('editForm')">保存</el-button>
</div>
</el-dialog>
1.2 通过点击 新增/编辑将表单对应窗口弹出
通过写方法让其对应的表单显示出来
handleEdit() {
//展示新增文章的表单窗体
this.editFormVisible = true;
}
1.3 给表单设置规则 rules
给表单增加校验规则
rules: {
title: [{
required: true,
message: '请输入文章标题',
trigger: 'blur'
},
{
min: 5,
max: 10,
message: '长度在 5 到 10 个字符',
trigger: 'blur'
}
],
body: [{
required: true,
message: '请输入文章内容',
trigger: 'blur'
}],
}
1.4 当表单提交时要校验规则
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
alert('submit!');
} else {
console.log('error submit!!');
return false;
}
});
最后总页面 Articles.vue
<template>
<div>
<el-form :inline="true" :model="formInline" class="user-search">
<el-form-item label="搜索:">
<el-input size="small" v-model="formInline.title" placeholder="请输入文章标题"></el-input>
</el-form-item>
<el-form-item>
<el-button size="small" type="primary" icon="el-icon-search" @click="search">搜索</el-button>
<el-button size="small" type="primary" icon="el-icon-plus" @click="handleEdit()">添加</el-button>
</el-form-item>
</el-form>
<el-table size="small" :data="listData" highlight-current-row style="width: 100%;">
<el-table-column align="center" type="selection" width="60">
</el-table-column>
<el-table-column sortable prop="id" label="文章id" width="300">
</el-table-column>
<el-table-column sortable prop="title" label="文章标题" width="300">
</el-table-column>
<el-table-column sortable prop="body" label="文章内容" width="300">
</el-table-column>
<el-table-column align="center" label="操作" min-width="300">
<template slot-scope="scope">
<el-button size="mini" @click="handleEdit(scope.$index, scope.row)">编辑</el-button>
<el-button size="mini" type="danger" @click="deleteUser(scope.$index, scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
<el-pagination style="margin-top: 20px;" @size-change="handleSizeChange" @current-change="handleCurrentChange"
:current-page="formInline.page" :page-sizes="[10, 20, 30, 50]" :page-size="100" layout="total, sizes, prev, pager, next, jumper"
:total="formInline.total">
</el-pagination>
<el-dialog :title="title" :visible.sync="editFormVisible" width="30%" @click="closeDialog">
<el-form label-width="120px" :model="editForm" :rules="rules" ref="editForm">
<el-form-item label="文章标题" prop="title">
<el-input size="small" v-model="editForm.title" auto-complete="off" placeholder="请输入文章标题"></el-input>
</el-form-item>
<el-form-item label="文章内容" prop="body">
<el-input size="small" v-model="editForm.body" auto-complete="off" placeholder="请输入内容"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button size="small" @click="closeDialog">取消</el-button>
<el-button size="small" type="primary" class="title" @click="submitForm('editForm')">保存</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
export default {
name: 'Articles',
data() {
return {
title: '',
editFormVisible: false,
editForm: {
title: '',
body: ''
},
rules: {
title: [{
required: true,
message: '请输入文章标题',
trigger: 'blur'
},
{
min: 5,
max: 10,
message: '长度在 5 到 10 个字符',
trigger: 'blur'
}
],
body: [{
required: true,
message: '请输入文章内容',
trigger: 'blur'
}],
},
listData: [],
formInline: {
page: 1,
total: 10,
title: ''
}
};
},
methods: {
handleEdit() {
this.editFormVisible = true;
},
deleteUser() {
},
handleSizeChange(rows) {
console.log("页面大小发送改变");
this.formInline.page = 1;
this.formInline.rows = rows;
this.search();
},
handleCurrentChange(page) {
console.log("当前页发送改变");
this.formInline.page = page;
this.search();
},
doSearch(param) {
let url = this.axios.urls.SYSTEM_ARTICLE_LIST;
this.axios.post(url, param)
.then(resp => {
console.log(resp);
this.listData = resp.data.result;
this.formInline = resp.data.pageBean;
})
.catch(function() {
});
},
search() {
this.doSearch(this.formInline);
},
closeDialog() {
},
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
alert('submit!');
} else {
console.log('error submit!!');
return false;
}
});
}
},
created() {
this.doSearch({});
}
}
</script>
<style>
</style>
效果 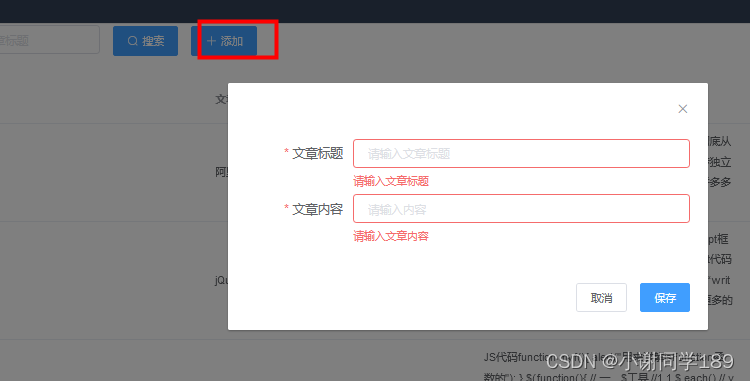 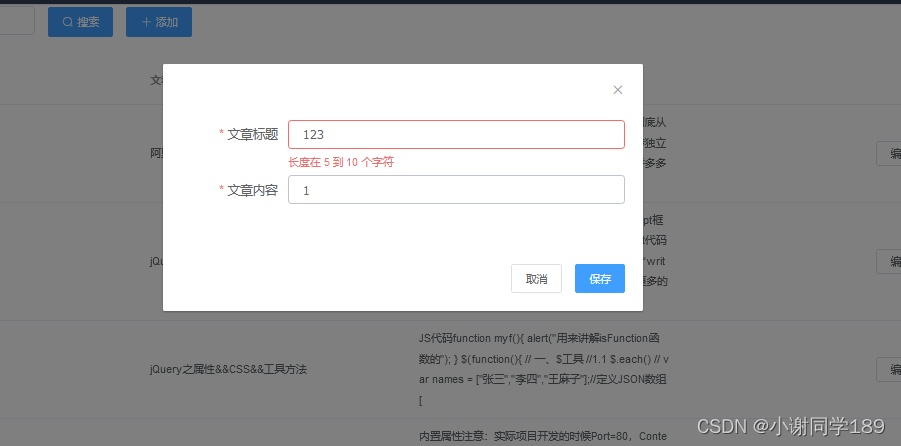 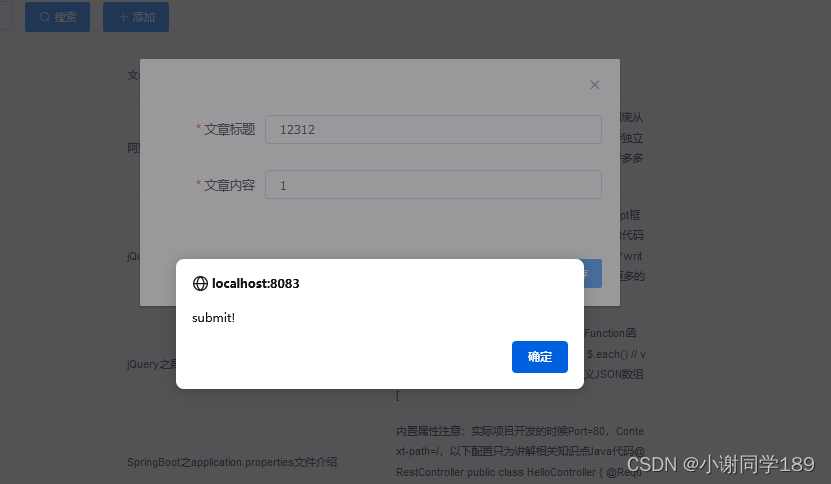
存在问题:有缓存,而且后期我们编辑和增加弹出的是同一个窗口,要进行判断(后面CRUD优化)
二、CRUD
2.1 保障编辑窗体正常弹出
因为我们编辑和新增用的是同一个方法,所有我们要再其进行判断弹框,因为我们有缓存问题,所有我们还要新增一个清除缓存的方法,在关闭窗体调用
Articles.vue
<template>
<div>
<el-form :inline="true" :model="formInline" class="user-search">
<el-form-item label="搜索:">
<el-input size="small" v-model="formInline.title" placeholder="请输入文章标题"></el-input>
</el-form-item>
<el-form-item>
<el-button size="small" type="primary" icon="el-icon-search" @click="search">搜索</el-button>
<el-button size="small" type="primary" icon="el-icon-plus" @click="handleEdit()">添加</el-button>
</el-form-item>
</el-form>
<el-table size="small" :data="listData" highlight-current-row style="width: 100%;">
<el-table-column align="center" type="selection" width="60">
</el-table-column>
<el-table-column sortable prop="id" label="文章id" width="300">
</el-table-column>
<el-table-column sortable prop="title" label="文章标题" width="300">
</el-table-column>
<el-table-column sortable prop="body" label="文章内容" width="300">
</el-table-column>
<el-table-column align="center" label="操作" min-width="300">
<template slot-scope="scope">
<el-button size="mini" @click="handleEdit(scope.$index, scope.row)">编辑</el-button>
<el-button size="mini" type="danger" @click="deleteUser(scope.$index, scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
<el-pagination style="margin-top: 20px;" @size-change="handleSizeChange" @current-change="handleCurrentChange"
:current-page="formInline.page" :page-sizes="[10, 20, 30, 50]" :page-size="100" layout="total, sizes, prev, pager, next, jumper"
:total="formInline.total">
</el-pagination>
<el-dialog :title="title" :visible.sync="editFormVisible" width="30%" @click="closeDialog">
<el-form label-width="120px" :model="editForm" :rules="rules" ref="editForm">
<el-form-item label="文章标题" prop="title">
<el-input size="small" v-model="editForm.title" auto-complete="off" placeholder="请输入文章标题"></el-input>
</el-form-item>
<el-form-item label="文章内容" prop="body">
<el-input size="small" v-model="editForm.body" auto-complete="off" placeholder="请输入内容"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button size="small" @click="closeDialog">取消</el-button>
<el-button size="small" type="primary" class="title" @click="submitForm('editForm')">保存</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
export default {
name: 'Articles',
data() {
return {
title: '',
editFormVisible: false,
editForm: {
title: '',
body: '',
id:''
},
rules: {
title: [{
required: true,
message: '请输入文章标题',
trigger: 'blur'
},
{
min: 5,
max: 10,
message: '长度在 5 到 10 个字符',
trigger: 'blur'
}
],
body: [{
required: true,
message: '请输入文章内容',
trigger: 'blur'
}],
},
listData: [],
formInline: {
page: 1,
total: 10,
title: ''
}
};
},
methods: {
handleEdit(index,row) {
this.editFormVisible = true;
if(row){
this.title = '编辑窗体';
this.editForm.id = row.id;
this.editForm.title = row.title;
this.editForm.body = row.body;
}
else{
this.title = '新增窗体';
}
},
deleteUser() {
},
handleSizeChange(rows) {
console.log("页面大小发送改变");
this.formInline.page = 1;
this.formInline.rows = rows;
this.search();
},
handleCurrentChange(page) {
console.log("当前页发送改变");
this.formInline.page = page;
this.search();
},
doSearch(param) {
let url = this.axios.urls.SYSTEM_ARTICLE_LIST;
this.axios.post(url, param)
.then(resp => {
console.log(resp);
this.listData = resp.data.result;
this.formInline = resp.data.pageBean;
})
.catch(function() {
});
},
search() {
this.doSearch(this.formInline);
},
closeDialog() {
this.clearData();
},
clearData(){
this.editForm.title = '';
this.editForm.body = '';
this.editForm.id = '';
this.title = '';
this.editFormVisible = false;
},
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
let url = this.axios.urls.SYSTEM_ARTICLE_ADD;
} else {
console.log('error submit!!');
return false;
}
});
}
},
created() {
this.doSearch({});
}
}
</script>
<style>
</style>
效果 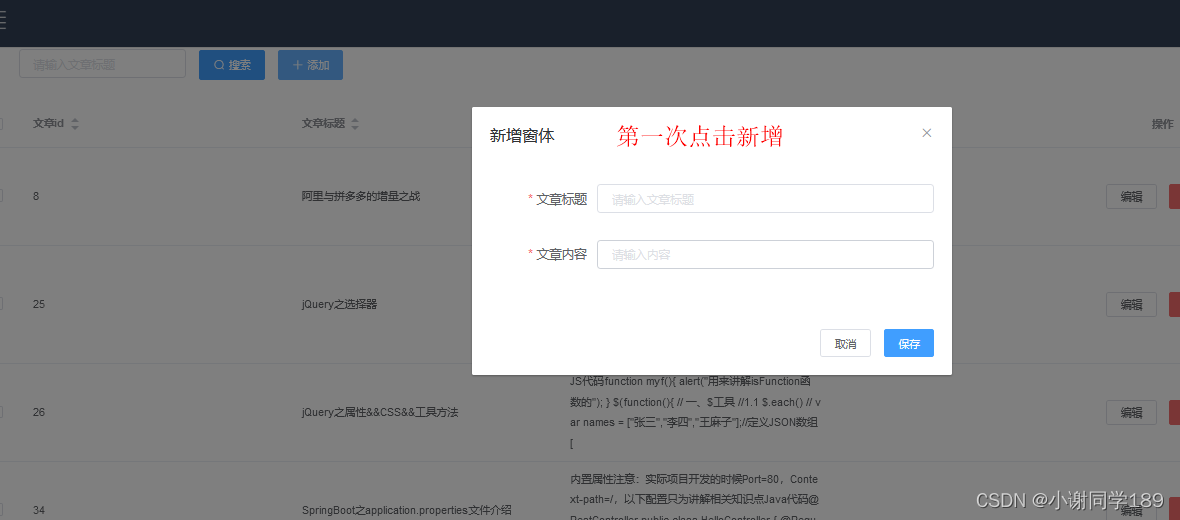 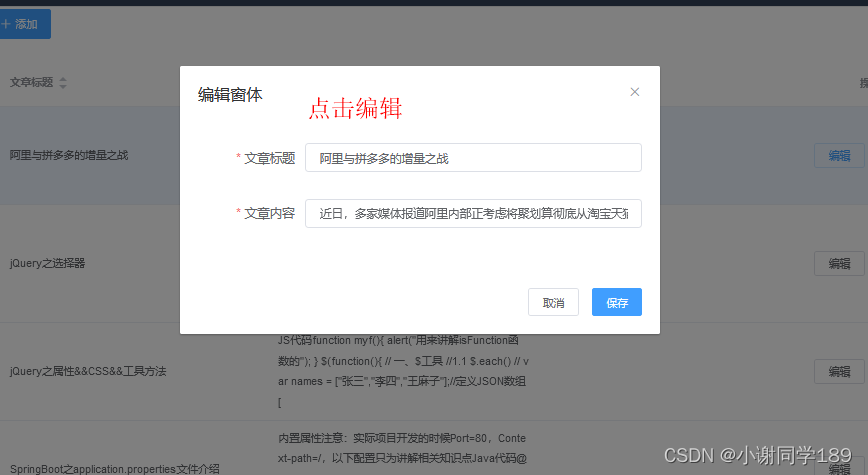 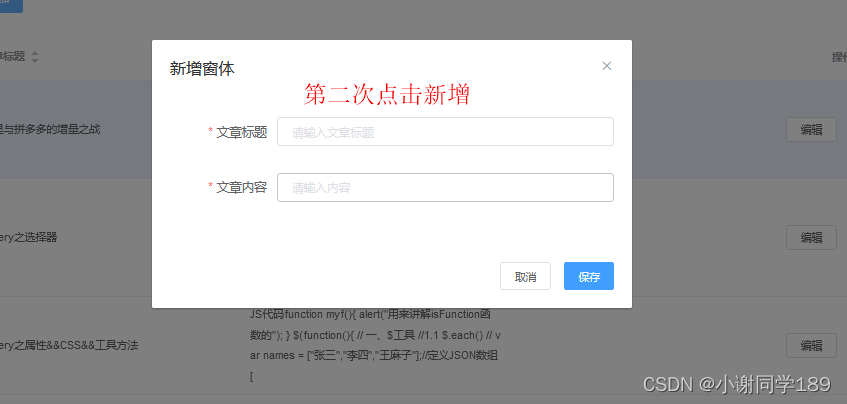
2.2 增加修改
这里因为我们用的是同一个方法,所有我们通过判断id来看是删除还是修改,在通过axios调用接口来完成
<template>
<div>
<el-form :inline="true" :model="formInline" class="user-search">
<el-form-item label="搜索:">
<el-input size="small" v-model="formInline.title" placeholder="请输入文章标题"></el-input>
</el-form-item>
<el-form-item>
<el-button size="small" type="primary" icon="el-icon-search" @click="search">搜索</el-button>
<el-button size="small" type="primary" icon="el-icon-plus" @click="handleEdit()">添加</el-button>
</el-form-item>
</el-form>
<el-table size="small" :data="listData" highlight-current-row style="width: 100%;">
<el-table-column align="center" type="selection" width="60">
</el-table-column>
<el-table-column sortable prop="id" label="文章id" width="300">
</el-table-column>
<el-table-column sortable prop="title" label="文章标题" width="300">
</el-table-column>
<el-table-column sortable prop="body" label="文章内容" width="300">
</el-table-column>
<el-table-column align="center" label="操作" min-width="300">
<template slot-scope="scope">
<el-button size="mini" @click="handleEdit(scope.$index, scope.row)">编辑</el-button>
<el-button size="mini" type="danger" @click="deleteUser(scope.$index, scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
<el-pagination style="margin-top: 20px;" @size-change="handleSizeChange" @current-change="handleCurrentChange"
:current-page="formInline.page" :page-sizes="[10, 20, 30, 50]" :page-size="100" layout="total, sizes, prev, pager, next, jumper"
:total="formInline.total">
</el-pagination>
<el-dialog :title="title" :visible.sync="editFormVisible" width="30%" @click="closeDialog">
<el-form label-width="120px" :model="editForm" :rules="rules" ref="editForm">
<el-form-item label="文章标题" prop="title">
<el-input size="small" v-model="editForm.title" auto-complete="off" placeholder="请输入文章标题"></el-input>
</el-form-item>
<el-form-item label="文章内容" prop="body">
<el-input size="small" v-model="editForm.body" auto-complete="off" placeholder="请输入内容"></el-input>
</el-form-item>
</el-form>
<div slot="footer" class="dialog-footer">
<el-button size="small" @click="closeDialog">取消</el-button>
<el-button size="small" type="primary" class="title" @click="submitForm('editForm')">保存</el-button>
</div>
</el-dialog>
</div>
</template>
<script>
export default {
name: 'Articles',
data() {
return {
title: '',
editFormVisible: false,
editForm: {
title: '',
body: '',
id: ''
},
rules: {
title: [{
required: true,
message: '请输入文章标题',
trigger: 'blur'
},
{
min: 5,
max: 10,
message: '长度在 5 到 10 个字符',
trigger: 'blur'
}
],
body: [{
required: true,
message: '请输入文章内容',
trigger: 'blur'
}],
},
listData: [],
formInline: {
page: 1,
total: 10,
title: ''
}
};
},
methods: {
handleEdit(index, row) {
this.editFormVisible = true;
if (row) {
this.title = '编辑窗体';
this.editForm.id = row.id;
this.editForm.title = row.title;
this.editForm.body = row.body;
} else {
this.title = '新增窗体';
}
},
deleteUser() {
},
handleSizeChange(rows) {
console.log("页面大小发送改变");
this.formInline.page = 1;
this.formInline.rows = rows;
this.search();
},
handleCurrentChange(page) {
console.log("当前页发送改变");
this.formInline.page = page;
this.search();
},
doSearch(param) {
let url = this.axios.urls.SYSTEM_ARTICLE_LIST;
this.axios.post(url, param)
.then(resp => {
console.log(resp);
this.listData = resp.data.result;
this.formInline = resp.data.pageBean;
})
.catch(function() {
});
},
search() {
this.doSearch(this.formInline);
},
closeDialog() {
this.clearData();
},
clearData() {
this.editForm.title = '';
this.editForm.body = '';
this.editForm.id = '';
this.title = '';
this.editFormVisible = false;
},
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
let url;
if (this.editForm.id == 0) {
url = this.axios.urls.SYSTEM_ARTICLE_ADD;
} else {
url = this.axios.urls.SYSTEM_ARTICLE_EDIT;
}
this.axios.post(url, this.editForm).then(t => {
this.$message({
message: t.data.msg,
type: 'success'
});
this.clearData();
this.search();
}).catch(e => {
})
} else {
console.log('error submit!!');
return false;
}
});
}
},
created() {
this.doSearch({});
}
}
</script>
<style>
</style>
效果 点击新增 点击编辑 
2.3 删除
注意删除只要传入相对应的id即可
删除方法
在deleteUser(index, row) {
this.$confirm('此操作将永久删除该文件, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
let url = this.axios.urls.SYSTEM_ARTICLE_DEL;
this.axios.post(url, {id:row.id}).then(t => {
this.$message({
type: 'success',
message: '删除成功!'
});
this.clearData(); //关闭清空
this.search(); //查询
}).catch(e => {
})
}).catch(() => {
this.$message({
type: 'info',
message: '已取消删除'
});
});
},
点击删除 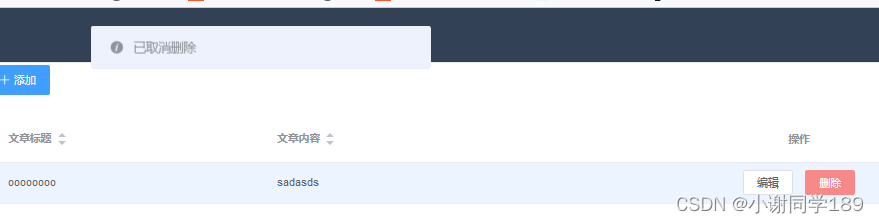 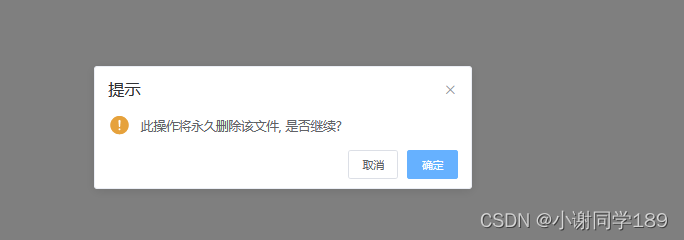

|