vue实战-vuex模块化开发
1.vuex是什么?
vuex是官方提供的插件,状态管理库,集中式管理项目中组件共用的数据。
2.五大属性说明
state 对象类型,类似于实例的 data属性,存放数据 getters 对象类型,类似于实例的计算属性 computed mutations 对象类型,类似于实例的 methods,但是不能处理异步方法 actions 对象类型,类似于实例的 methods,可以处理异步方法 modules 对象类型,当state内容比较多时,通过该属性分割成小模块,每个模块都拥有自己的 state、mutation、action、getter
3.vuex基本使用
例:动态展示三级联动数据
1)创建好vuex仓库
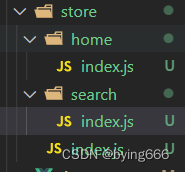 TypeNav在home组件模块下,于是在home的小仓库中动态获取与展示数据. home的index.js
import { reqCategoryList } from "@/api"
const state={
categoryList:[]
}
const mutations={
CATEGORYLIST(state,categoryList){
state.categoryList = categoryList
}
}
const actions={
async categoryList({commit}){
let result = await reqCategoryList()
if(result.code == 200){
commit('CATEGORYLIST', result.data)
}
}
}
const getters={}
export default {
state,
mutations,
actions,
getters
}
在home小仓库中将接口的数据获取处理好,放进state中便于组件获取。
总仓库
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
import home from './home'
import search from './search'
export default new Vuex.Store({
modules:{
home,
search
}
})
2)在TypeNav组件中设置挂载跳转到仓库获取数据
mounted(){
this.$store.dispatch('categoryList')
},
再进入已经创建好的home小仓库处理,得到数据放入仓库中。 再获取仓库中的数据展示在页面中 利用vuex中的mapState方法
import { mapState } from 'vuex';
computed:{
...mapState({
categoryList:state => state.home.categoryList
})
}
3)在TypeNav的html页面中使用数据动态展示
<template>
<div class="type-nav">
<div class="container">
<h2 class="all">全部商品分类</h2>
<nav class="nav">
<a href="###">服装城</a>
<a href="###">美妆馆</a>
<a href="###">尚品汇超市</a>
<a href="###">全球购</a>
<a href="###">闪购</a>
<a href="###">团购</a>
<a href="###">有趣</a>
<a href="###">秒杀</a>
</nav>
<div class="sort">
<div class="all-sort-list2">
<div class="item" v-for="c1 in categoryList" :key="c1.categoryId">
<h3>
<a href="">{{c1.categoryName}}</a>
</h3>
<div class="item-list clearfix">
<div class="subitem">
<dl class="fore" v-for="c2 in c1.categoryChild" :key="c2.categoryId">
<dt>
<a href="">{{c2.categoryName}}</a>
</dt>
<dd>
<em v-for="c3 in c2.categoryChild" :key="c3.categoryId">
<a href="">{{c3.categoryName}}</a>
</em>
</dd>
</dl>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
</template>
仓库中的数据 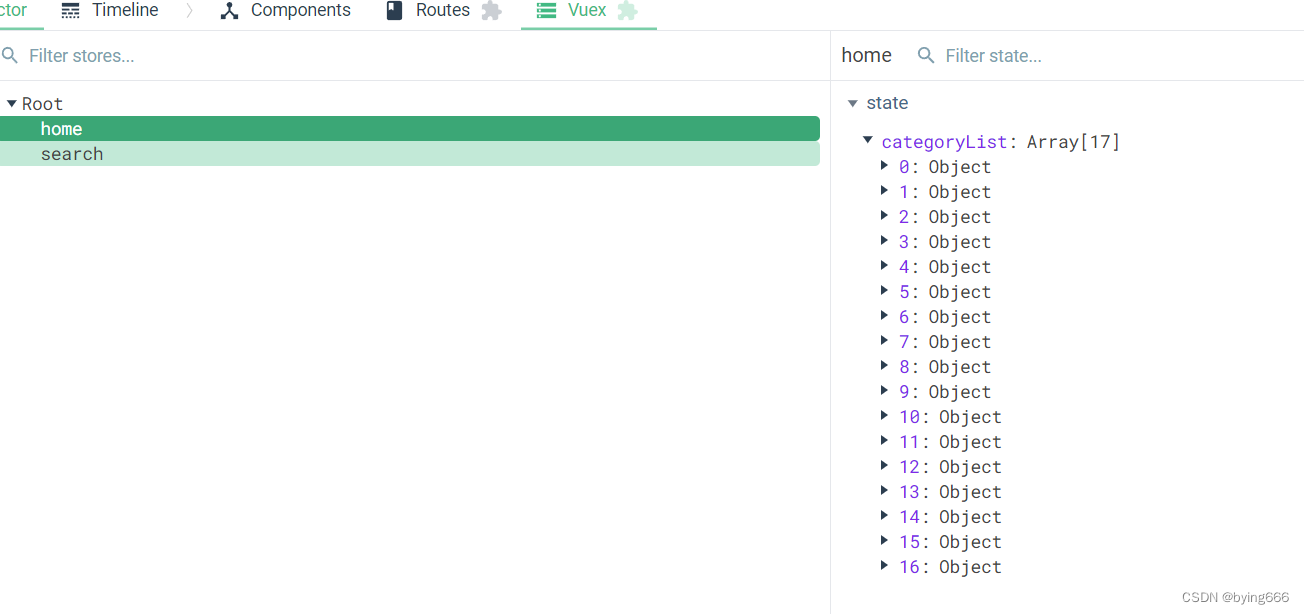
|