目录
自定义事件(绑定)
自定义事件(解绑)
组件自定义事件——总结
自定义事件(绑定)
App.vue
<template>
<div class="app">
<h1>{{ msg }}</h1>
<!-- 通过父组件给子组件传递函数类型的props实现,子给父传递数据 -->
<School :getSchoolName="getSchoolName" />
<!-- v-on在组件student上,所以说给组件的实例对象vc绑定了事件atguigu,如果有人触发了事件,则demo函数会被调用 -->
<!-- 通过父组件给子组件绑定一个自定义事件实现,子给父传递数据(第一种写法,使用@或v-on) -->
<!-- <Student v-on:atguigu="getStudentName" /> -->
<!-- 也能只触发一次 -->
<Student @atguigu.once="getStudentName" />
<!-- 通过父组件给子组件绑定一个自定义事件实现,子给父传递数据 (第二种写法,使用ref)-->
<!-- 优点:更灵活一点,可以用定时器,可以设置只能触发一次 -->
<!-- <Student ref="student"/> -->
</div>
</template>
<script>
// 引入school组件
import Student from "./componments/Student.vue";
import School from "./componments/School.vue";
export default {
name: "App",
components: { Student, School },
data() {
return {
msg: "你好啊!",
};
},
methods: {
getSchoolName(name) {
console.log("App收到了学校名:", name);
},
// 除了name,其他东西都用params接收
getStudentName(name,...params) {
console.log("App收到了学生名:", name,params);
},
},
mounted(){
// 定时器
// setTimeout(()=>{
// this.$refs.student.$on('atguigu',this.getStudentName)
// 绑定自定义事件
// },3000)
// 绑定自定义事件
// 只能触发一次
// this.$refs.student.$once('atguigu',this.getStudentName)
}
};
</script>
<style scoped>
.app {
background-color: gray;
padding: 5px;
}
</style>
Student.vue
<template>
<div class="student">
<h2>学生姓名:{{ name }}</h2>
<h2>学生性别:{{ sex }}</h2>
<button @click="sendStudentName">把学生名给App</button>
</div>
</template>
<script>
export default {
name: "Student",
data() {
return {
name: "张三",
sex: "男",
};
},
methods:{
sendStudentName(){
// 触发Student组件实例对象身上的atguigu事件
// this.$emit('atguigu',this.name)
// 如果有很多东西需要传,方法1,直接写
// this.$emit('atguigu',this.name,666,888,999)
// 方法2,包装成对象,ES6语法
this.$emit('atguigu',this.name,666,888,999)
}
}
};
</script>
<style lang="less" scoped>
.student {
background-color: pink;
padding: 5px;
margin-top: 30px;
}
</style>
自定义事件(解绑)
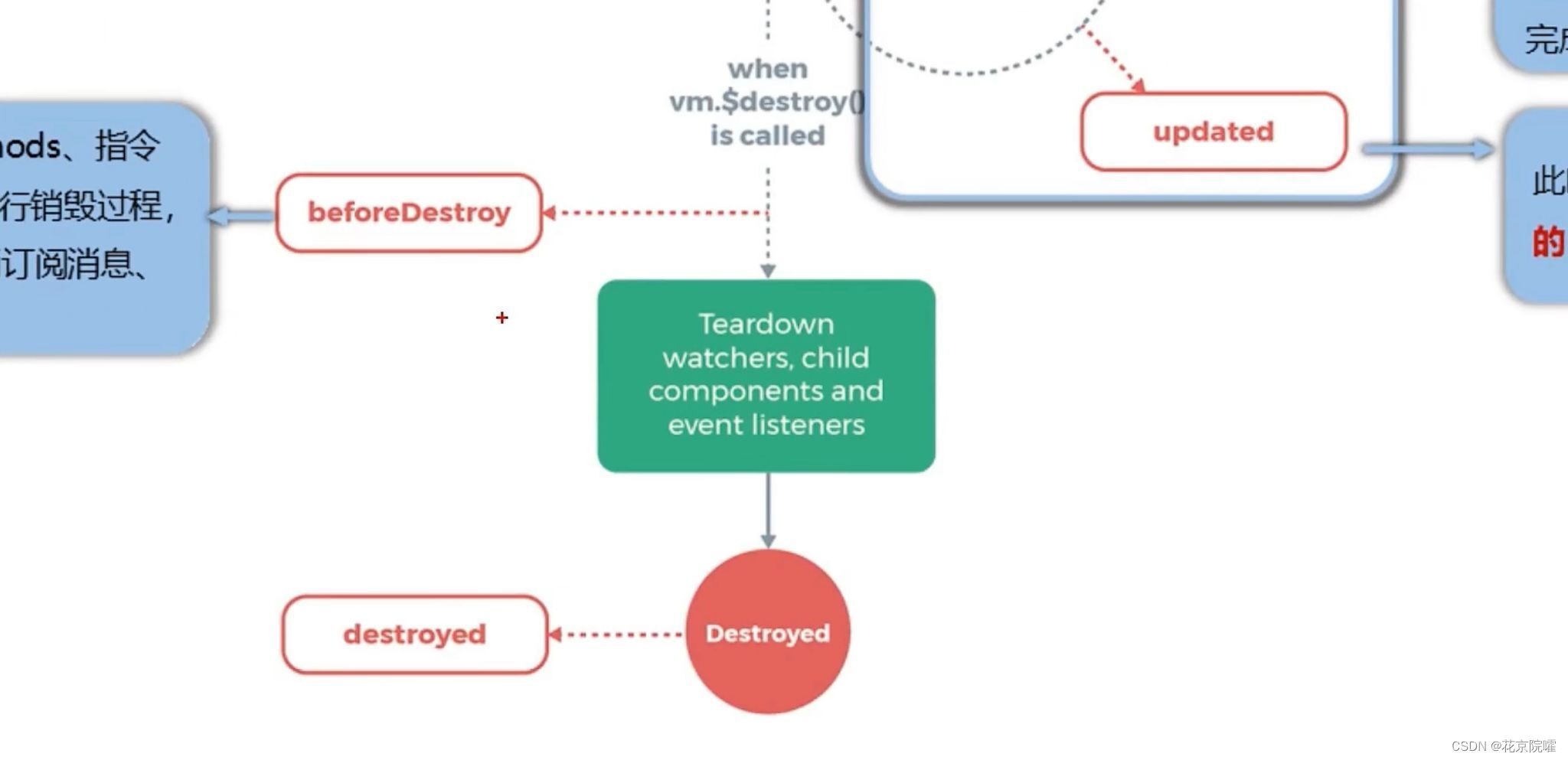
?图中意思是当vm被this.destory()时,即被销毁,内部的所有自定义事件,子组件,监视,监听全部被销毁
?Student.vue
<template>
<div class="student">
<h2>学生姓名:{{ name }}</h2>
<h2>学生性别:{{ sex }}</h2>
<h2>当前求和为:{{number}}</h2>
<button @click="add">点我number++</button>
<button @click="sendStudentName">把学生名给App</button>
<button @click="unbind">解绑atguigu事件</button>
<button @click="death">销毁当前Student组件的实例(vc)</button>
</div>
</template>
<script>
export default {
name: "Student",
data() {
return {
name: "张三",
sex: "男",
number:0
};
},
methods:{
add(){
console.log('add回调被调用了');
this.number++
},
sendStudentName(){
// 触发Student组件实例对象身上的atguigu事件
// this.$emit('atguigu',this.name)
// 如果有很多东西需要传,方法1,直接写
// this.$emit('atguigu',this.name,666,888,999)
// 方法2,包装成对象,ES6语法
this.$emit('atguigu',this.name,666,888,999)
this.$emit('demo')
},
unbind(){
// 只能解绑一个事件
// this.$off('atguigu')
// 解绑多个自定义事件
// this.$off(['atguigu','demo'])
// 解绑所有的自定义事件
this.$off()
},
death(){
// 销毁了当前Student组件的实例
// 销毁后所有Student实例的自定义事件全都不奏效
this.$destroy()
}
}
};
</script>
<style lang="less" scoped>
.student {
background-color: pink;
padding: 5px;
margin-top: 30px;
}
</style>
main.js
// 引入Vue
import Vue from 'vue'
// 引入App
import App from './App.vue'
// 关闭Vue的生产提示
Vue.config.productionTip=false
// 创建vm
new Vue({
el:'#app',
render:h=>h(App),
mounted() {
setTimeout(()=>{
this.$destroy()
},3000)
},
})
以上代码使用了定时器,3秒过后,app被销毁
组件自定义事件——总结
插值语法的来源:
- data
- props
- computed
?1.一种组件间通信的方式,适用于:子组件===>父组件
子组件会触发父组件的事件(回调函数),所以自定义事件是子组件传给父组件。回调函数是留在父组件
2.使用场景:A是父组件,B是子组件,B想给A传数据,那么就要在A中给B绑定自定义事件(事件的回调在A中)
3.绑定自定义事件:
- 第一种方式,在父组件中:<Demo @athuihu="test"/> 或 <Demo v-on:atguigu="test">
- 第二种方式,在父组件中:
<Demo ref="demo"/>
......
mounted(){
this.$refs.xxx.$on('atguigu',this.test)
}
- 若想让自定义事件只能触发一次,可以使用once修饰符,或$once方法
4.触发自定义事件:this.$emit('atguigu',数据)
5.解绑自定义事件:this.$off('atguigu')
6.组件也可以绑定原生DOM事件,需要使用native修饰符
7.注意:通过this.$refs.xxx.$on('atguigu',回调)绑定自定义事件,回调要么配置在methods中,要么箭头函数,否则this指向会出问题
<!-- 对于组件除了可以设置自定义事件,还可以使用原生dom事件 -->
<Student ref="student" @click.native="show"/>
// 第二种方法
this.$refs.student.$on('atguigu',(name,...params)=>{
console.log('App收到了学生名:',name,params);
console.log(this);
this.studentName=name
})
|