前端研习录(41)——ES6 Promise对象讲解及示例分析
版权声明
- 本文原创作者:清风不渡
- 博客地址:https://blog.csdn.net/WXKKang
??重拾前端记忆,记录学习笔记,现在进入ES6Promise对象部分
一、Promise对象
1、定义
??Promise是异步编程的一个解决方案,比起传统的解决方案(回调函数和事件)要更加合理和强大
优点: ?? Promise对象可以将异步操作以同步操作的流程表达出来,避免了层层嵌套的回调函数,另外也提供了同一的接口,使得控制异步操作更加容易
2、语法
??ES6规定,Promise对象是一个构造函数,基本语法如下:
const promise = new Promise(function(resolve,reject){
if () {
resolve(value);
}else{
reject(error)
}
});
??Promise构造函数接受一个函数作为参数,该函数有两个参数(resolve,reject)。它们是两个函数,有JavaScript引擎提供,不用自己部署 ??Promise实例生成后,可以用then方法分别指定resolved状态和rejected状态的回调函数
promise.then(function(value){
},function(error){
})
3、示例
??通过promise 对象实现图片加载,示例代码如下:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>清风不渡</title>
</head>
<body>
<div id="box"></div>
<script>
var box = document.getElementById("box");
function loadImage(url){
const promise = new Promise(function(resolve,reject){
const image = new Image();
image.src = url;
image.onload = function(){
resolve(image);
}
image.onerror = function(){
reject(new Error('Could not load Image at '+url));
}
});
return promise;
}
const promise = loadImage("./img/1.webp");
promise.then(function(value){
box.appendChild(value)
},function(error){
box.innerHTML = "图片加载失败";
console.log(error);
})
</script>
</body>
</html>
??结果示例如下:
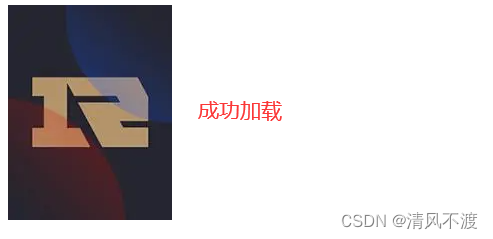 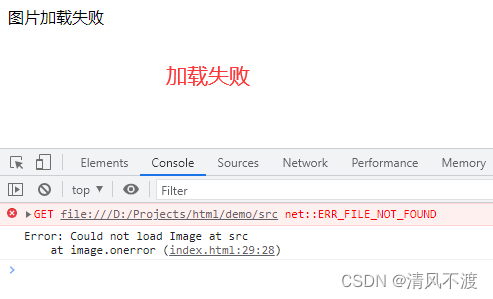
二、Promise对象_Ajax实操
??通过Promise对象实现网络请求
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>清风不渡</title>
</head>
<body>
<script>
const getJSON = function(url){
const promise = new Promise(function(resolve,reject){
const handler = function(){
if(this.readyState !== 4){
return;
}
if (this.status === 200){
resolve(this.response)
}else{
reject(new Error(thsi.statusText))
}
}
const client = new XMLHttpRequest();
client.open("GET",url);
client.onreadystatechange = handler;
client.responseType = "json";
client.setRequestHeader("Accept","application/json");
client.send();
})
return promise;
}
getJSON("请求地址").then(function(data){
console.log(data);
},function(error){
console.log(error);
})
</script>
</body>
</html>
|