系统环境
- JetPack4.4
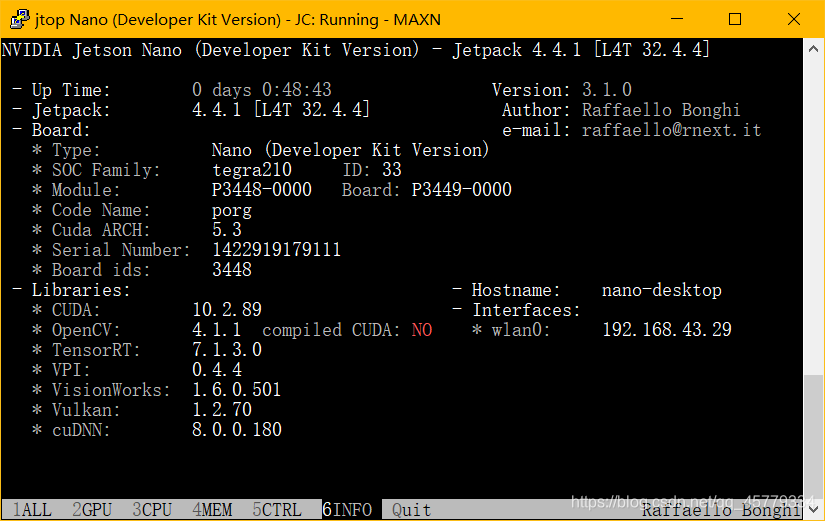
如果需要此镜像的同学可以在Jetson 下载中心下载即可。
一、安装PaddlePaddle
有两种方式,因为nano官方有已经编译好的python3.6的whl,所以我们直接下载就好,不用编译。
1.直接下载或编译预测库
(1)直接下载官方编译好的Jetson nano预测库
下载地址
下载
选择python3.6版本的下载即可(注意不要下载后三个版本,因为nano的GPU型号与之不匹配,安装会报错。) 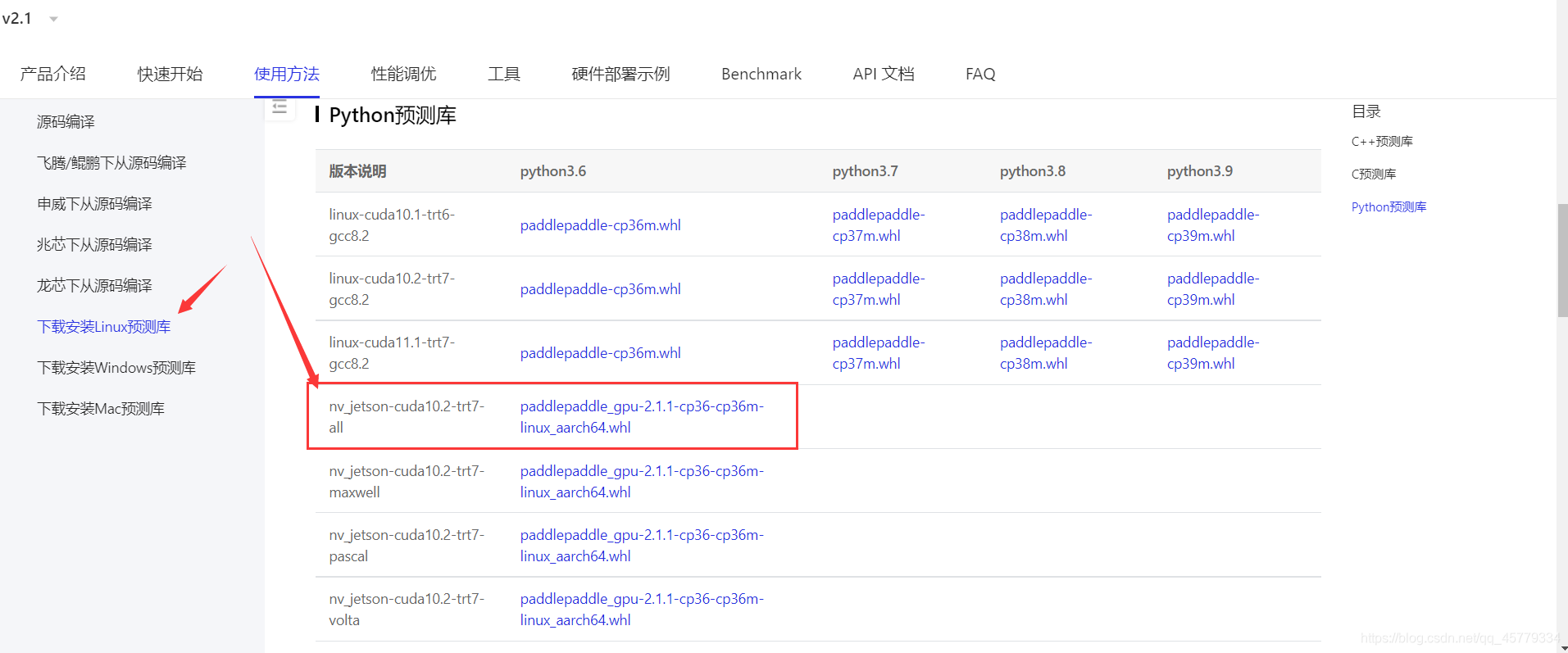
(2)编译官方预测库
在Jetson nano上编译paddlepaddle(带TensorRT)并跑通Paddle-Inference-Demo
2.安装whl
(1)安装依赖项
这里必须安装1.18.3版本的numpy,否则后边会报错。
pip3 install numpy==1.18.3
将下载好的whl文件传送到nano上,然后安装whl:
pip3 install paddlepaddle_gpu-2.1.1-cp36-cp36m-linux_aarch64.whl
(2)测试
打开python3:
import paddle
paddle.fluid.install_check.run_check()
报warning忽略即可,不影响使用。如果遇到报错,请继续往下看。 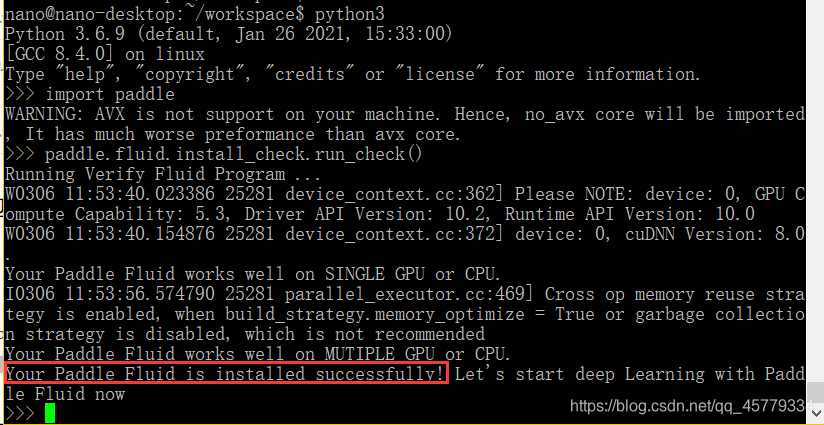
(3)报错解决
提取出报错中的关键信息:
弃用警告:imp模块弃用,取而代之的是importlib;有关其他用途,请参阅模块文档 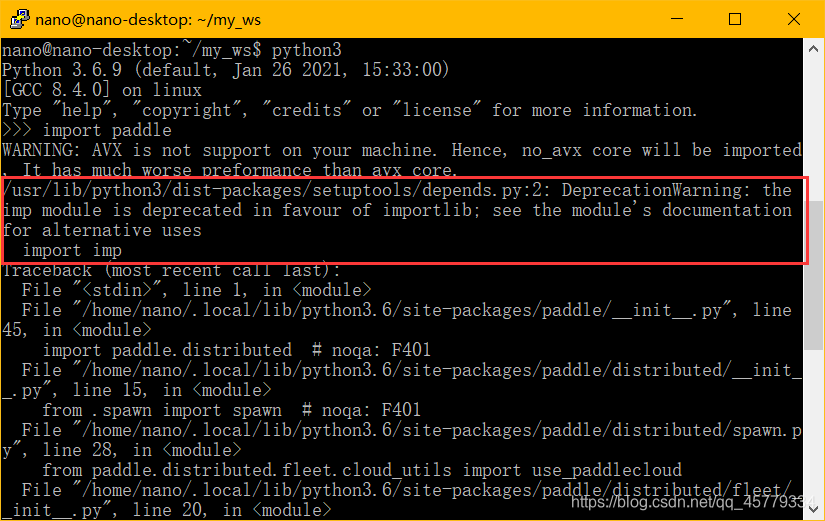
解决方法
- 打开
/usr/lib/python3/dist-packages/setuptools/depends.py ,将其中的imp 都修改为importlib ;保存时会报权限错误,所以需要给该文件修改权限,我这里直接使用命令:sudo chmod 777 /usr/lib/python3/dist-packages/setuptools/depends.py - 再次运行
import paddle ,会发现还会有类似报错,依然进行如上更换操作。 - 遇到报错如下:
 重装一下six模块就可以:
pip3 uninstall six
pip3 install six
二、测试Paddle Inference
1.环境准备
拉取Paddle-Inference-Demo:
git clone https://github.com/PaddlePaddle/Paddle-Inference-Demo.git
拉取比较慢的话可以在gitee上建个仓库下载,我建的仓库:https://gitee.com/irvingao/Paddle-Inference-Demo.git 。
2.测试跑通GPU预测模型
给可执行权限:
cd Paddle-Inference-Demo/python
chmod +x run_demo.sh
需要注意的是,需要将所有子文件夹中的run.sh 最后的python 修改为python3 : 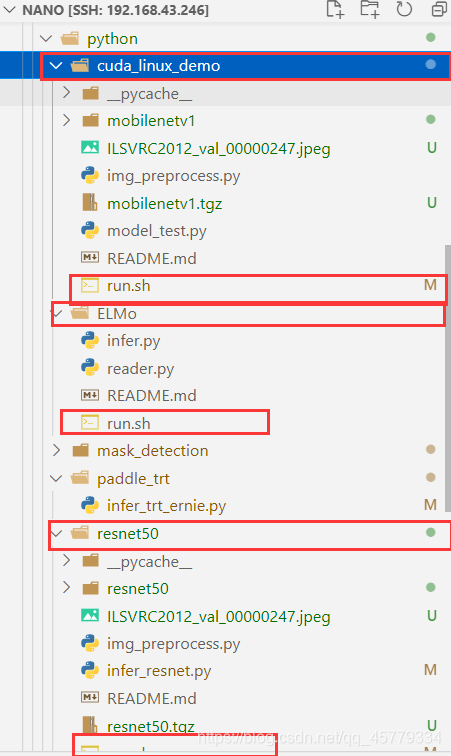 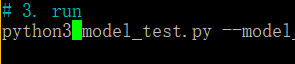
./run_demo.sh
也可以选择运行单个模型的run.sh 。如果过程中有报错,请继续往下看。
3.报错解决
(1)运行demo过程中卡住
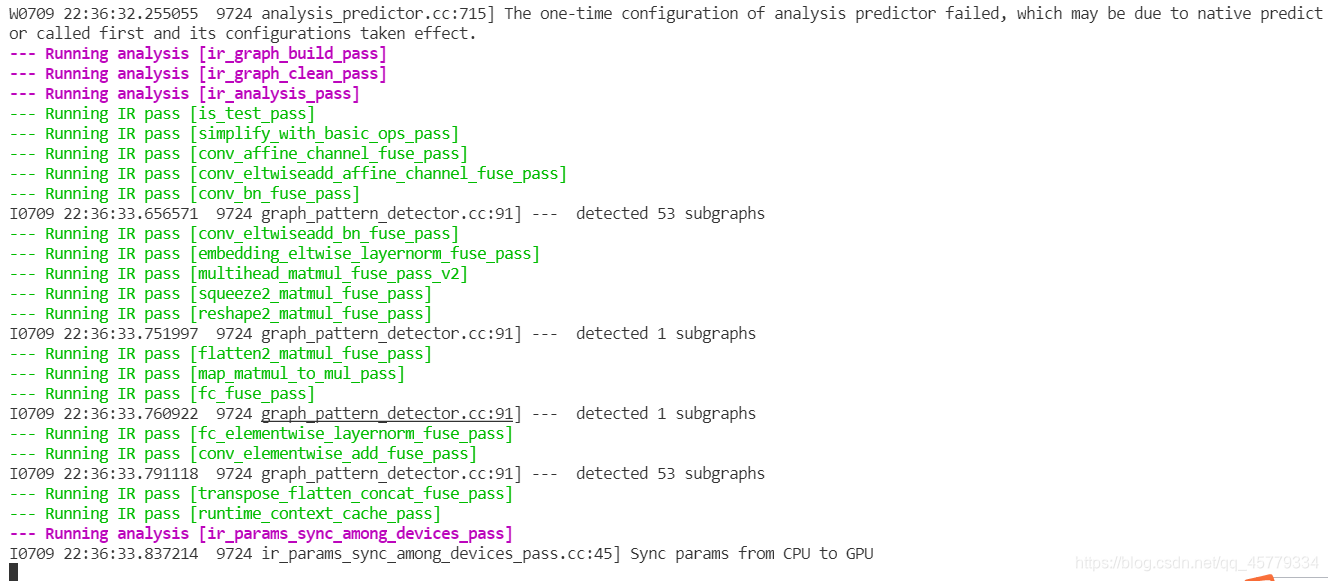 解决方法: 扩大运行内存 (亲测:建议至少给8G,反正6G不行)
sudo fallocate -l 8G /var/swapfile8G
sudo chmod 600 /var/swapfile8G
sudo mkswap /var/swapfile8G
sudo swapon /var/swapfile8G
sudo bash -c 'echo "/var/swapfile8G swap swap defaults 0 0" >> /etc/fstab'
扩大虚拟内存后,运行正常: 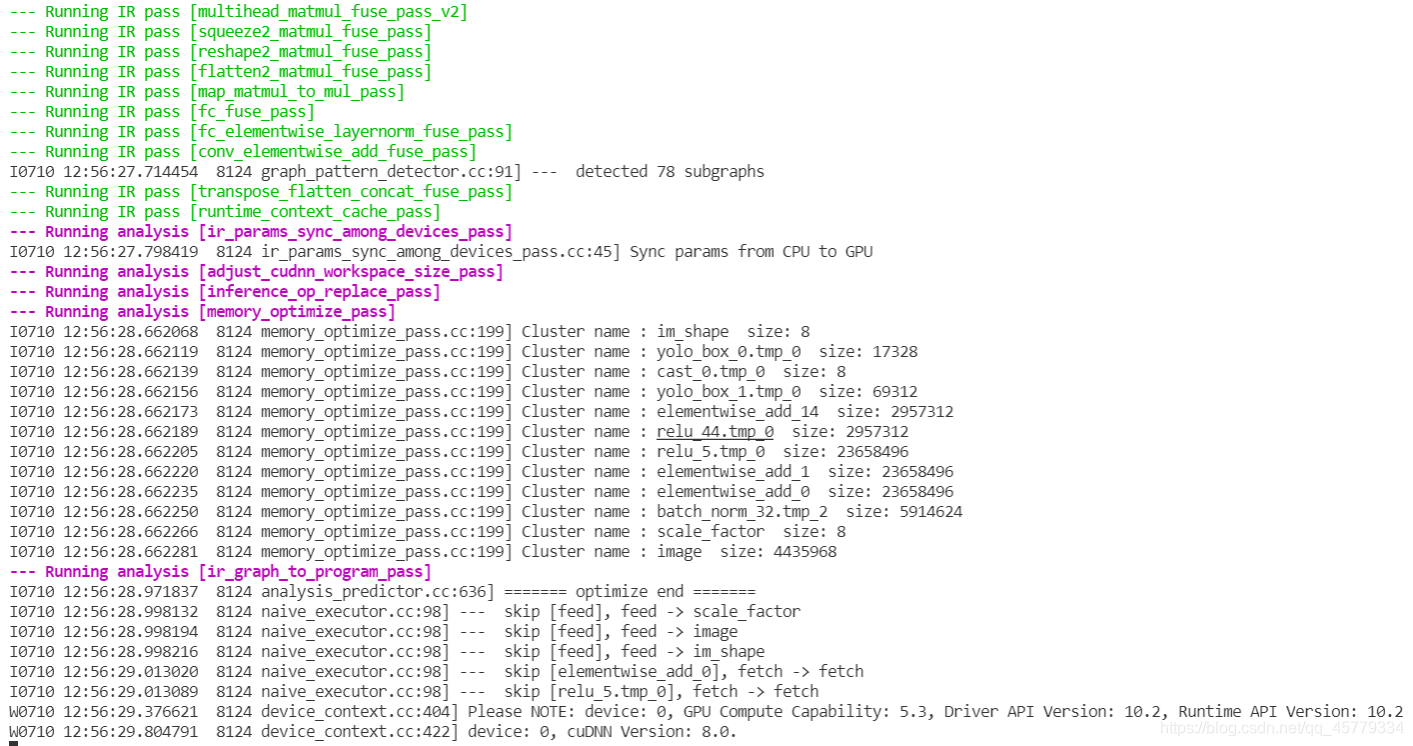
(2)core dumped
原因是因为JetPack4.4自带的numpy版本不对,numpy版本应为1.18.3。
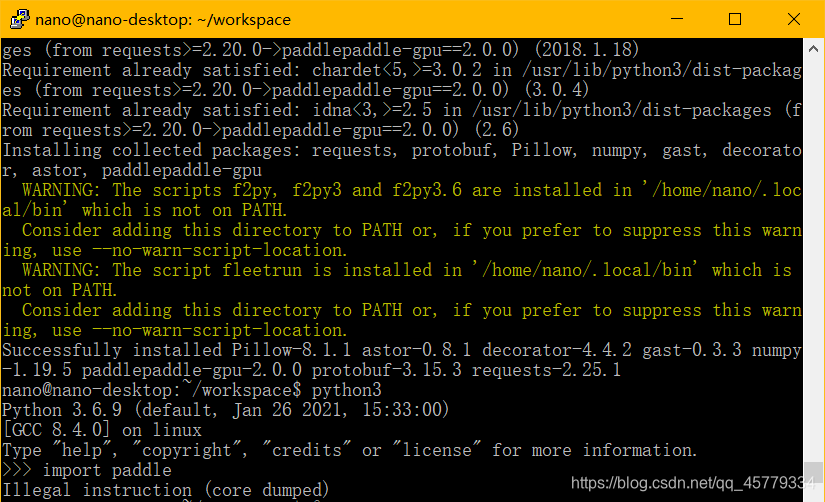 解决方法:
pip3 uninstall numpy
python3 -m pip install numpy==1.18.3 -i https://mirror.baidu.com/pypi/simple
三、部署自己的目标检测 / 图像分类模型
1.图像分类
import cv2
import numpy as np
from paddle.inference import Config
from paddle.inference import create_predictor
def resize_short(img, target_size):
""" resize_short """
percent = float(target_size) / min(img.shape[0], img.shape[1])
resized_width = int(round(img.shape[1] * percent))
resized_height = int(round(img.shape[0] * percent))
resized = cv2.resize(img, (resized_width, resized_height))
return resized
def crop_image(img, target_size, center):
""" crop_image """
height, width = img.shape[:2]
size = target_size
if center == True:
w_start = (width - size) / 2
h_start = (height - size) / 2
else:
w_start = np.random.randint(0, width - size + 1)
h_start = np.random.randint(0, height - size + 1)
w_end = w_start + size
h_end = h_start + size
img = img[int(h_start):int(h_end), int(w_start):int(w_end), :]
return img
def preprocess(img):
mean = [0.485, 0.456, 0.406]
std = [0.229, 0.224, 0.225]
img = resize_short(img, 224)
img = crop_image(img, 224, True)
img = img[:, :, ::-1].astype('float32').transpose((2, 0, 1)) / 255
img_mean = np.array(mean).reshape((3, 1, 1))
img_std = np.array(std).reshape((3, 1, 1))
img -= img_mean
img /= img_std
return img[np.newaxis, :]
def predict_config(model_file, params_file):
config = Config()
config.set_prog_file(model_file)
config.set_params_file(params_file)
config.enable_use_gpu(500, 0)
config.switch_ir_optim()
config.enable_memory_optim()
predictor = create_predictor(config)
return predictor
def predict(image, predictor):
img = preprocess(image)
input_names = predictor.get_input_names()
input_tensor = predictor.get_input_handle(input_names[0])
input_tensor.reshape(img.shape)
input_tensor.copy_from_cpu(img.copy())
predictor.run()
output_names = predictor.get_output_names()
output_tensor = predictor.get_output_handle(output_names[0])
output_data = output_tensor.copy_to_cpu()
print("output_names", output_names)
print("output_tensor", output_tensor)
print("output_data", output_data)
return output_data
def post_res(label_dict, res):
res = res.tolist()
target_index = res.index(max(res))
print("结果是:" + " " + label_dict[target_index])
if __name__ == '__main__':
label_dict = {0:"metal", 1:"paper", 2:"plastic", 3:"glass"}
model_file = "PaddleInfence\model\ResNet50_trashClas_x86_model\__model__"
params_file = "PaddleInfence\model\ResNet50_trashClas_x86_model\__params__"
image = cv2.imread("PaddleInfence\python_demo\metal1.jpg")
predictor = predict_config(model_file, params_file)
res = predict(image, predictor)
post_res(label_dict, res)
cv2.imshow("image", image)
cv2.waitKey()
2.目标检测模型
参考文章:
参考文献:
|