16–Django笔记–评论功能设计和用户登录
一、创建评论模型
评论模型中需要应用到的部分包括:
首先使用命令创建评论应用:
python3 manage.py startapp comment
创建模型:
from django.db import models
from django.contrib.contenttypes.fields import GenericForeignKey
from django.contrib.contenttypes.models import ContentType
from django.contrib.auth.models import User
class Comment(models.Model):
content_type = models.ForeignKey(ContentType, on_delete=models.DO_NOTHING)
object_id = models.PositiveIntegerField()
content_object = GenericForeignKey('content_type', 'object_id')
text = models.TextField()
comment_time = models.DateTimeField(auto_now_add = True)
user = models.ForeignKey(User, on_delete = models.DO_NOTHING)
注册应用:
from django.contrib import admin
from .models import Comment
@admin.register(Comment)
class CommentAdmin(admin.ModelAdmin):
list_display = ('content_object', 'text', 'comment_time', 'user')
setting.py 中注册应用:
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'blog',
'ckeditor',
'ckeditor_uploader',
'read_statistics',
'comment',
]
最后,迁移数据库
二、设计判读用户登录部分
request中有一个属性是user
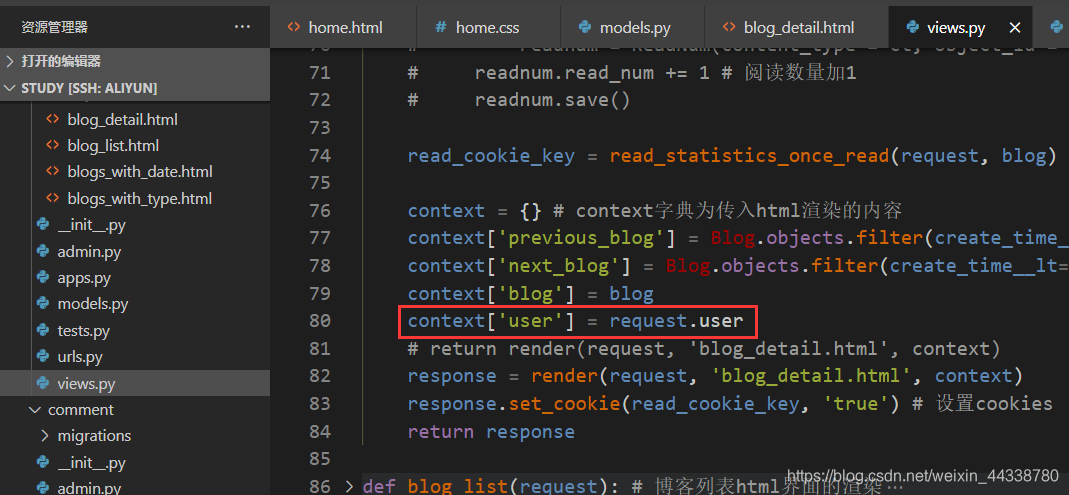
用户登录文档
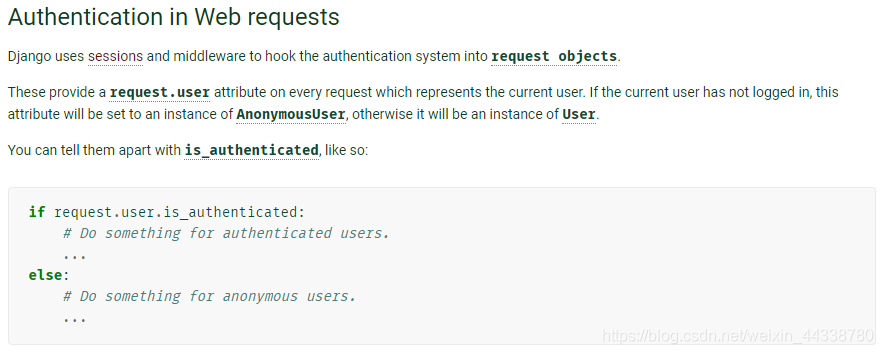
在前端页面中添加登录判断:
<div class="row">
<div class="col-xs-10 col-xs-offset-1">
<div style="margin-top: 2em; border: 1px dashed; padding: 2em;">
提交评论区
{% if user.is_authenticated %}
用户已登录
{% else %}
用户未登录
<form action="{% url 'login' %}" method="POST">
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="登录">
</form>
{% endif %}
</div>
<div style="margin-top: 2em; border: 1px dashed; padding: 2em;">评论列表区</div>
</div>
</div>
添加登录的url和方法:
urls.py
urlpatterns = [
path('', views.home, name = 'home'),
path('admin/', admin.site.urls),
path('ckeditor', include('ckeditor_uploader.urls')),
path('blog/', include('blog.urls')),
path('login/', views.login, name = 'login'),
]
views.py
from django.shortcuts import render, redirect
from read_statistics.utils import get_seven_days_read_data, get_today_hot_data, get_yesterday_hot_data
from django.contrib.contenttypes.models import ContentType
from blog.models import Blog
import datetime
from django.core.cache import cache
from django.utils import timezone
from django.db.models import Sum
from django.contrib import auth
def get_7_days_blogs():
today = timezone.now().date()
seven_date = today - datetime.timedelta(days = 7)
blogs = Blog.objects.filter(read_details__date__lt = today, read_details__date__gte = seven_date)\
.values('id', 'title')\
.annotate(read_num_sum = Sum('read_details__read_num'))\
.order_by('-read_num_sum')
return blogs
def home(request):
blog_content_type = ContentType.objects.get_for_model(Blog)
dates, read_nums = get_seven_days_read_data(blog_content_type)
hot_blogs_for_7_days = cache.get('hot_blogs_for_7_days')
if hot_blogs_for_7_days is None:
hot_blogs_for_7_days = get_7_days_blogs()
cache.set('hot_blogs_for_7_days', hot_blogs_for_7_days, 3600)
today_hot_data = get_today_hot_data(blog_content_type)
yesterday_hot_data = get_yesterday_hot_data(blog_content_type)
hot_blogs_for_7_days = get_7_days_blogs()
context = {}
context['dates'] = dates
context['read_nums'] = read_nums
context['today_hot_data'] = today_hot_data
context['yesterday_hot_data'] = yesterday_hot_data
context['hot_blogs_for_7_days'] = hot_blogs_for_7_days
return render(request, 'home.html', context)
def login(request):
username = request.POST.get('username', '')
password = request.POST.get('password', '')
user = auth.authenticate(request, username = username, password = password)
if user is not None:
auth.login(request, user)
return redirect('/')
else:
return render(request, 'error.html', {'message': '用户名或密码不正确'})
错误页面 error.html :
{% extends 'base.html'%}
{% load static %}
{% block title %}
我的网站|错误
{% endblock %}
{% block nav_home_active %}
active
{% endblock %}
{% block nav_blog_active %}
{% endblock %}
{% block content %}
{{ message }}
{% endblock %}
此时运行代码,提交表单时会出现报错:
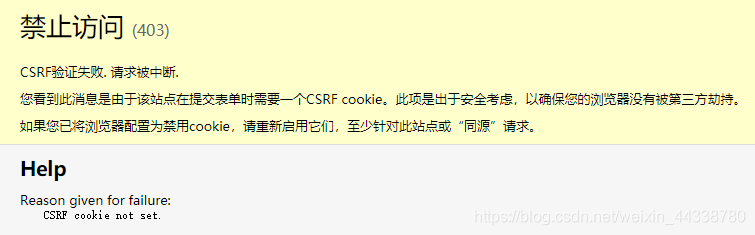
这是django自带的防止跨站攻击的验证
只需要在表单提交部分添加 {% csrf_token %} 即可:
<form action="{% url 'login' %}" method="POST">
{% csrf_token %}
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="登录">
</form>
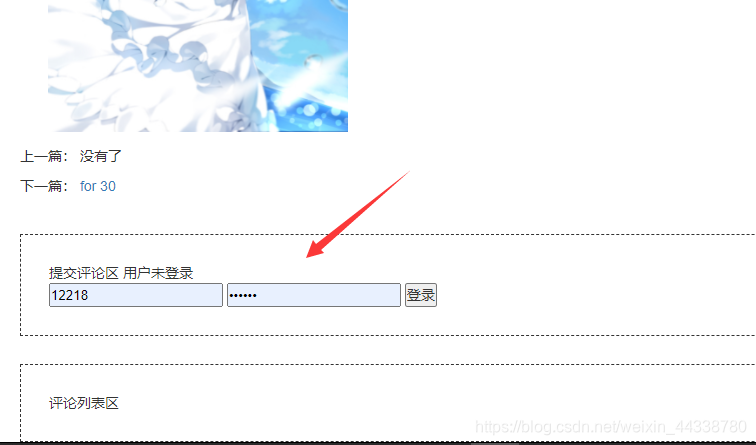
|