python学习——Day1
print()函数
print() 函数是 Python 中的信息输出函数,通过 print() 这一指令,将 () 内的内容打印出来。
print(50+50)
100
print('50+50')
50+50
转义字符
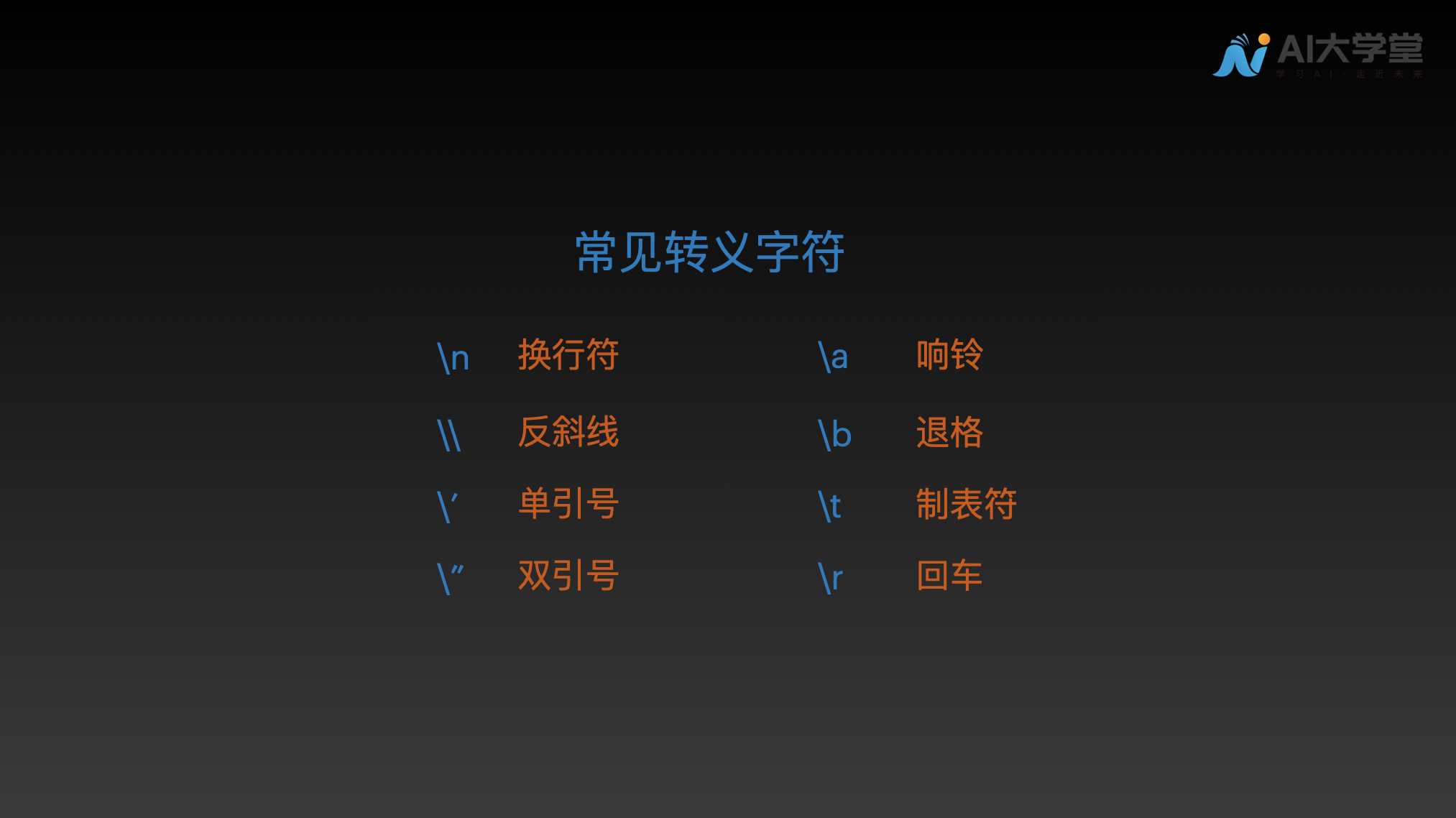
变量
首次赋值时才会创建变量 单变量赋值
name = '女娲'
print(name)
女娲
多变量赋值
name = '女娲'
name = '女娲后人'
print(name)
女娲后人
同一个变量名称,我们对其进行多次赋值的时候,变量会取最后一次的赋值内容。
x = y = z = '女娲'
print(x)
print(y)
print(z)
女娲
女娲
女娲
x,y,z = '女娲',42 16
print(x)
print(y)
print(z)
女娲
42
16
变量命名规则
- 变量名只能包含数字、字母和下划线
- 变量名必须以字母和下划线开头
- 变量名称区分大小写
- 如果一个变量名包含多个单词,用下划线进行的分隔
python中还有关键字,这些关键字不能用作变量名、函数名或任何其他标识符 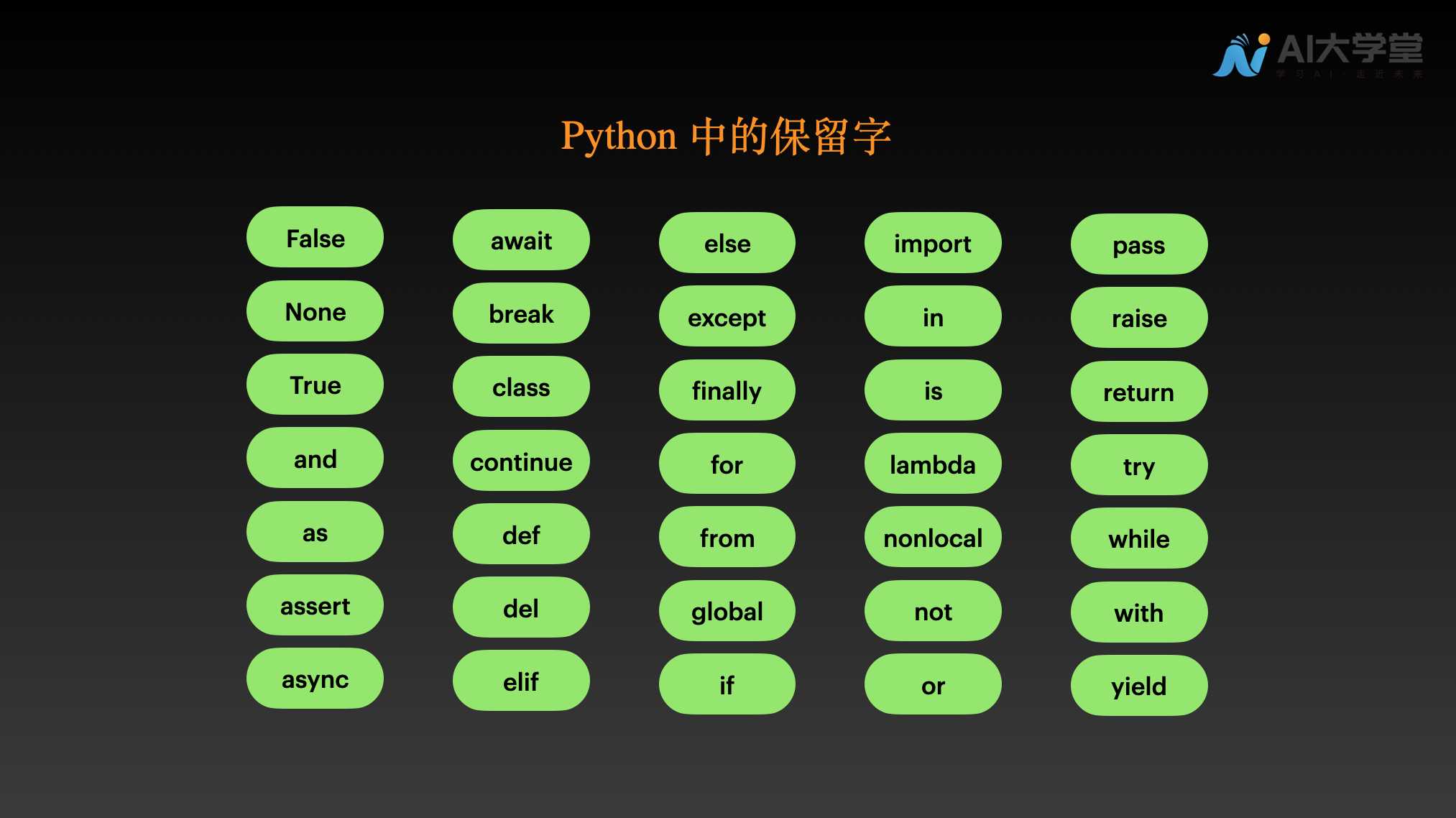
数据类型
数字类型
整型 int 浮点型 float
pi = 3.1415926
negative_pi = -3.1415926
print(pi)
print(type(negative_pi))
3.1415926
<class 'float'>
运算符 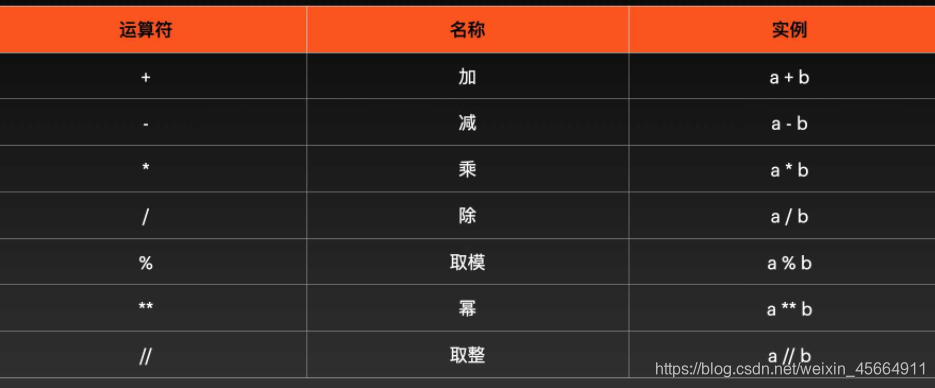 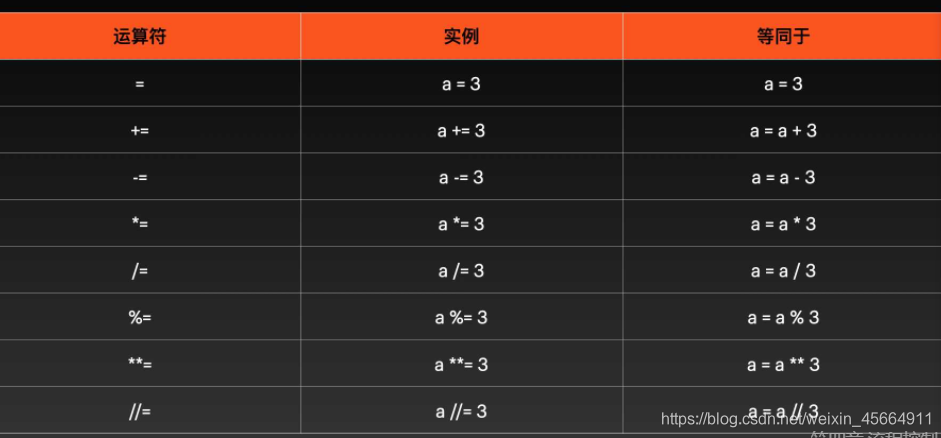 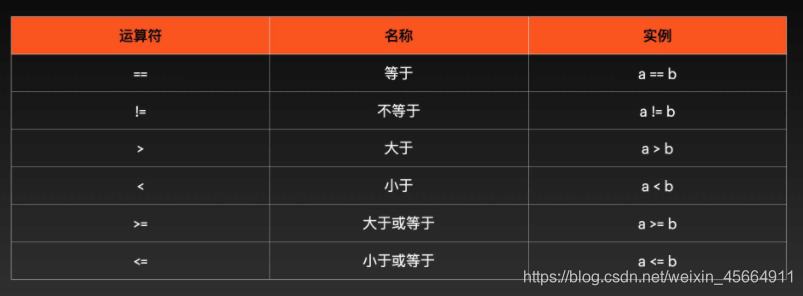 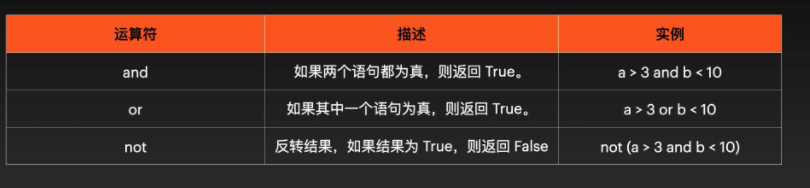
布尔型
用true和false表示布尔值 布尔值可以用and,or,not运算
字符串类型
以单引号或者双引号括起来的任何文本都是字符串
如果字符串横跨多行,可以使用三个单引号或三个双引号将字符串括起来。
整型和浮点数可以赋值给变量,字符串也可以
索引
索引分为从前往后索引和从后往前索引 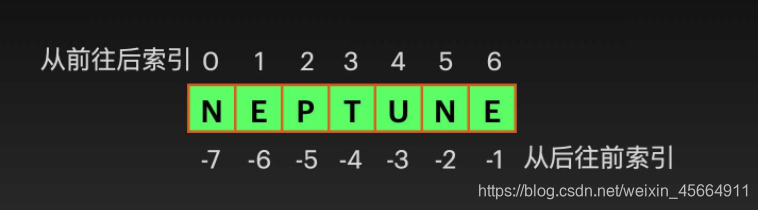
key = 'NEPTUNE'
print(key[0])
print(key[-1])
print(key[6])
print(key[-7])
N
E
N
N
切片
索引只能提取单个字符,我们利用切片提取变量的多个字符
切片语法是:变量[头下标:尾下标] 当只指定头下标时,获取的是从头下标到字符串结尾的所有字符。 当只指定尾下标时,获取的是字符串的开头到尾下标的字符串。 头下标和尾下标也可以使用负值。
例如
star = "NEPTUNE"
print(star[0:3])
print(star[:])
print(star[-7:-5])
print(star[1:])
print(star[:4])
字符串长度
利用len()获取字符串长度
star = "NEPTUNE"
print(len(star))
7
字符串方法
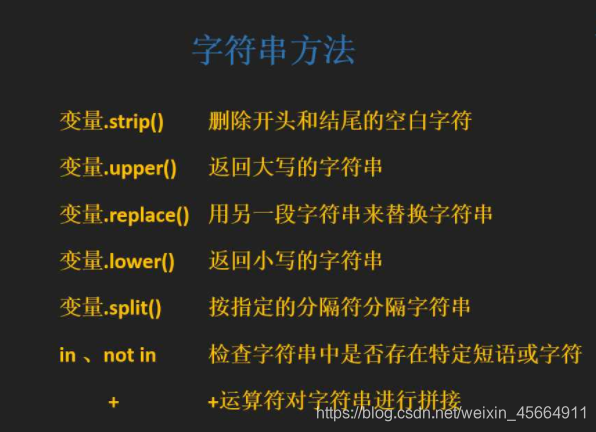 例如 .strip()
star = " NEPTUNE "
print(star)
print(len(star))
print(star.strip())
print(len(star.strip()))
NEPTUNE
9
NEPTUNE
7
.replace()
say_hi = "Hello World!"
print(say_hi.replace("World", "Kitty"))
Hello Kitty!
.split()
say_hi = "Mercury,Venus,Earth,Mars,Jupiter,Saturn,Uranus,Neptune,Pluto"
print(say_hi.split(","))
['Mercury', 'Venus', 'Earth', 'Mars', 'Jupiter', 'Saturn', 'Uranus', 'Neptune', 'Pluto']
使用 in 或 not in 来检查字符串中是否存在特定短语或字符
book_name = "Men Are from Mars, Women Are from Venus"
is_exist = "Mars" in book_name
print(is_exist)
True
使用 + 运算符对字符串进行拼接
first_part = "Men Are from Mars"
second_part = "Women Are from Venus"
print(first_part + ', ' + second_part)
Men Are from Mars, Women Are from Venus
在字符串中引用变量的值
在字符串前面加小写字母 ‘f’ 在字符串中,将需要引用的变量,用花括号包起来 {name}
name = 'Earth'
age = 4.543E9
print(f"My name is {name}, {age} years old")
My name is Earth, 4543000000.0 years old
转义
对于双引号中含有双引号或者单引号中有单引号进行转义,以免出现编译错误
print('You\'re uinique, nothing can replace you.')
You're uinique, nothing can replace you.
print('You're uinique, nothing can replace you.')
报错
获取数据类型和数据类型转换
利用type()获取变量类型
weight1= 50
weight2 = '50'
weight3= 50.00
print(type(weight1))
print(type(weight2))
print(type(weight3))
<class 'int'>
<class 'str'>
<class 'float'>
利用int(),float(),str()进行类型转换
weight1= 50
weight3= 50.00
weight4= int(weight3)
weight5=float(weight1)
print(type(weight1))
print(type(weight3))
print(type(weight4))
print(type(weight5))
<class 'int'>
<class 'float'>
<class 'int'>
<class 'float'>
运用str() 和字符串的+运算符打印内容
num1 = 3
num2 = 50
num3 = 150
print('神农每天品尝 '+str(num1)+' 种草药,品尝了 '+str(num2)+' 天,一共品尝了 '+str(num3) + ' 种草药')
神农每天品尝 3 种草药,品尝了 50 天,一共品尝了 150 种草药
|