定义logit类
from functools import wraps
import smtplib
from email.mime.text import MIMEText
from email.header import Header
import time
class logit(object):
def __init__(self, logfile='out.log'):
self.logfile = logfile
def __call__(self, func):
@wraps(func)
def wrapped_function(*args, **kwargs):
log_string = func.__name__ + " was called"
print(log_string)
with open(self.logfile, 'a') as opened_file:
opened_file.write(log_string + '\n')
self.notify()
return func(*args, **kwargs)
return wrapped_function
def notify(self):
pass
@logit()
def myfunc1():
pass
myfunc1()
创建logit的子类email_logit
class email_logit(logit):
'''
一个logit的实现版本,可以在函数调用时发送email给管理员
'''
def __init__(self, email='xxxxxxx', *args, **kwargs):
self.email = email
super(email_logit, self).__init__(*args, **kwargs)
self.mail_host="smtp.qq.com"
self.mail_pass="xxxxxxx"
self.sender = 'xxxxxxx'
self.receivers = [email]
def notify(self):
receivers = self.receivers
content = "日志创建"+time.strftime("%a %b %d %H:%M:%S %Y", time.localtime())
message = MIMEText('content', 'plain', 'utf-8')
message['From'] = Header("测试", 'utf-8')
message['To'] = Header("测试", 'utf-8')
subject = "日志创建"+ time.strftime("%a %b %d %H:%M:%S %Y", time.localtime())
message['Subject'] = Header(subject, 'utf-8')
try:
smtpObj = smtplib.SMTP_SSL(self.mail_host, 465)
smtpObj.login(self.sender,self.mail_pass)
smtpObj.sendmail(self.sender, self.receivers, message.as_string())
smtpObj.quit()
print('邮件发送成功')
except smtplib.SMTPException as e:
print('邮件发送失败')
@email_logit()
def myfunc2():
pass
myfunc2()
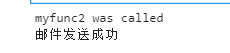 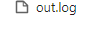 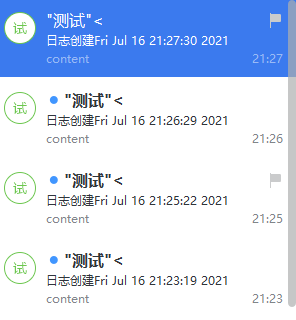
|