Python学习Day02
改进猜数字的小游戏
三个改进方向
- 用户猜错时,程序应该给出提示
- 应该提供多次的机会给用户
- 每次运行程序时,答案应该时随机的
伪随机数(random)
Python 3.8.11 文档
random–生成伪随机数
python模块的使用方法:import + 模块名
>>> import random
>>> random.randint(1,10)
4
>>> random.randint(1,10)
5
>>> random.randint(1,10)
5
>>> random.randint(1,10)
10
>>> random.randint(100,1000)
random生成的随机数可以被重现
原理:要实现对伪随机数的攻击,就要拿到它的种子
random.getstate()
获取随机数种子加工之后随机数生成器的内部状态 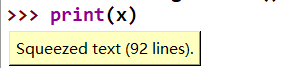
>>> import random
>>> x = random.getstate()
>>> print(x)
random.setstate()
重新设置随机数生成器的内部状态
>>> import random
>>> x = random.getstate()
>>> print(x)
>>> random.randint(1,10)
10
>>>
>>> random.randint(1,10)
6
>>> random.randint(1,10)
5
>>> random.setstate(x)
>>> random.randint(1,10)
10
>>> random.randint(1,10)
6
>>> random.randint(1,10)
5
数字类型
整数
python的整数长度不受限制
浮点数
python的浮点数并不是百分百精确的
>>> 0.1 + 0.2
0.30000000000000004
>>> 0.3 == 0.1 + 0.2
False
>>> 0.3 < 0.1 + 0.2
True
精确浮点数—decimal模块
decimal—十进制定点和浮点运算
>>> import decimal
>>> a = decimal.Decimal('0.1')
>>> b = decimal.Decimal('0.2')
>>> print(a + b)
0.3
>>> c = decimal.Decimal('0.3')
>>> a + b == c
True
复数
>>> 1 + 2j
(1+2j)
>>> x = 1 + 2j
>>> x.real
1.0
>>> x.imag
2.0
>>>
数据运算
操作 | 操作结果 |
---|
x + y | x加y的结果 | x - y | x减y的结果 | x * y | x乘以y的结果 | x / y | x除以y的结果 | x // y | x除以y的结果(地板除) | x % y | x除以y的余数 | - x | x的相反数 | + x | x本身 | abs(x) | x的绝对值 | int(x) | 将x转换成整数 | float(x) | 将x转换成浮点数 | complex(re,im) | 返回一个复数,re是实部,im是虚部 | c.conjugate() | 返回c的共轭复数 | divmod(x , y) | 返回(x//y,x%y) | pow(x , y) | 计算x的y次方 | x ** y | 计算x的y次方 |
地板除(//):取比目标结果小的最大整数(向下取整)
>>> 3 / 2
1.5
>>> 3 // 2
1
>>> -3 // 2
-2
>>> 2**3
8
>>> pow(2,3)
8
>>> pow(2,3,5)
3
>>>
布尔值类型
定义为False的对象
- None和False
- 值为0的数字类型:0,0.0,0j,Decimal(0),Fraction(0,1)
- 空的序列和集合:’’,(),[],{},set(),range(0)
逻辑运算符
运算符 | 含义 |
---|
and | 左边和右边同时为True,结果为True | or | 左边和右边其中一个为True,结果为True | not | 如果操作数为True,结果为False;如果操作数为True,结果为False |
>>> 3 < 4 and 4 < 5
True
>>> 3 < 4 and 4 > 5
False
>>> 3 < 4 or 4 > 5
True
>>> 3 < 4 not 4 < 5
>>> not True
False
>>> not False
True
>>> not 250
False
>>> not 3 < 4
False
and中含0,返回0;均为非0时,返回后一个值。
or中,至少有一个非0时,返回第一个非0值
>>> 3 and 4
4
>>> 3 or 4
3
>>> "Study" and 4
4
>>> "Study" or 4
'Study'
|