1.使用xlwt新建一个excel文件并向里面写入一些内容
import xlwt
def write_excel():
writeBook = xlwt.Workbook(encoding="utf-8")
writeSheet = writeBook.add_sheet("内容")
writeSheet.write(0, 0, 1)
writeSheet.write(0, 1, "数值")
writeBook.save(r"F:\新建excel.xlsx")
write_excel()
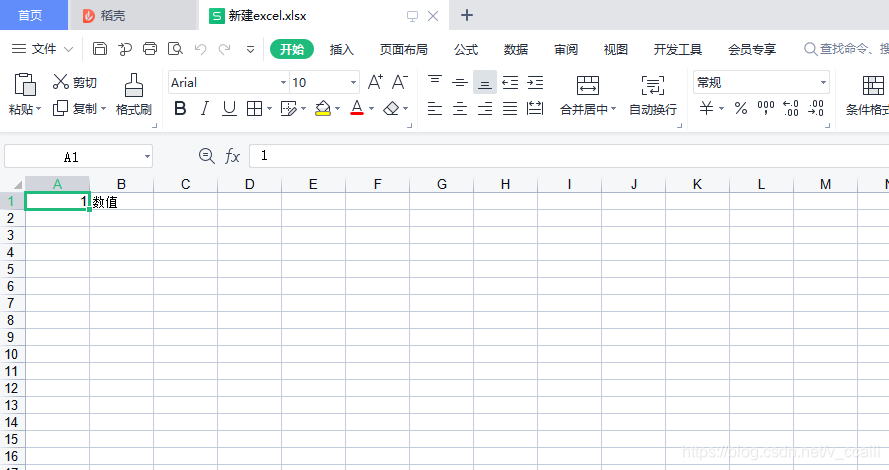
2.使用xlrd读取excel文件中的内容
import xlrd
def read_excel():
filePath = r"F:\数据.xlsx"
workBook = xlrd.open_workbook(filePath)
workSheet = workBook.sheet_by_name("简单数据")
rowCount = workSheet.nrows
colCount = workSheet.ncols
print("excel文件中一共:"+str(rowCount)+"行数据")
print("excel文件中一共:"+str(colCount)+"列数据")
data = workSheet.cell_value(1,1)
print("excel文件中第2行第2列的值是:"+str(data))
rowValue = workSheet.row_values(0)
print("excel文件中第1行的内容是:"+"\n"+str(rowValue))
colValue = workSheet.col_values(1)
print("excel文件中第2列的内容是:"+"\n"+str(colValue))
read_excel()
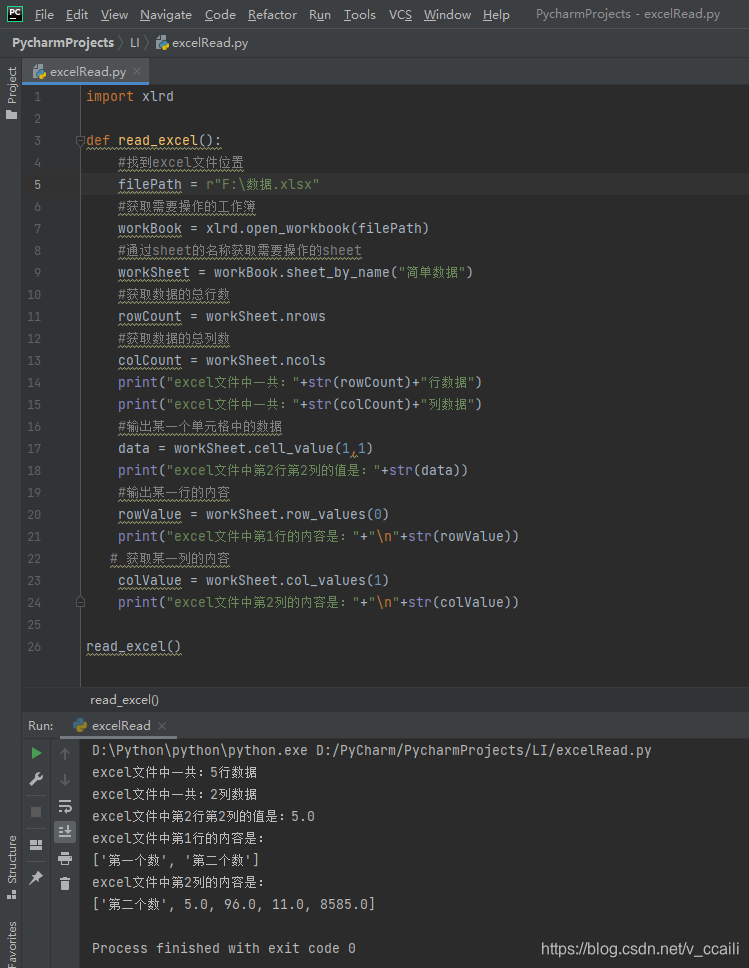 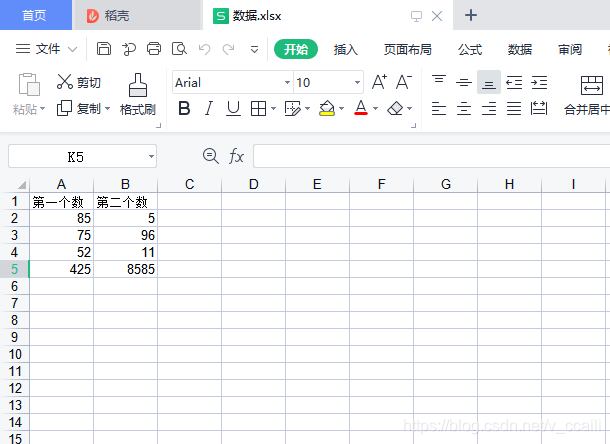
3.使用xlutils向一个已有数据的excel文件中添加内容
import xlrd
from xlutils.copy import copy
def xlutils_excel():
file_path = r"F:\数据.xlsx"
workBook = xlrd.open_workbook(file_path)
workSheet = workBook.sheet_by_index(0)
rowCount = workSheet.nrows
colCount = workSheet.ncols
new_workBook = copy(workBook)
new_workSheet = new_workBook.get_sheet(0)
new_workSheet.write(rowCount,0,"新添加的内容1")
new_workSheet.write(0,colCount,"新添加的内容2")
new_workBook.save(file_path)
xlutils_excel()
|