uiautomator
uiautomator 是python的安卓自动化测试模块,需要在android4.1+(API 16~30)的版本上工作,只需要通过adb连接android设备,不需要在android设备上安装别的东西。
安装uiautomator
pip install uiautomator2
为了更好的写自动化脚本,需要在安卓设备中定位图标位置,在此需要安装weditor包
pip install weditor
此外为了模拟安卓设备,需要安装个模拟器,现在安装“雷电模拟器”,可以自行在网上下载。 现在需要使用adb工具找到模拟器设备, ADB前置条件: 1.安装Android SDK,并将Android_HOME environment设置为正确路径。 2.在设备上启用ADB设置,并使用usb将android设备与PC连接。 3.允许在设备设置上从未知源安装应用程序。 在cmd中输入:
adb devices
即可得到设备的信息,下图就是说明设备已经连接上了。 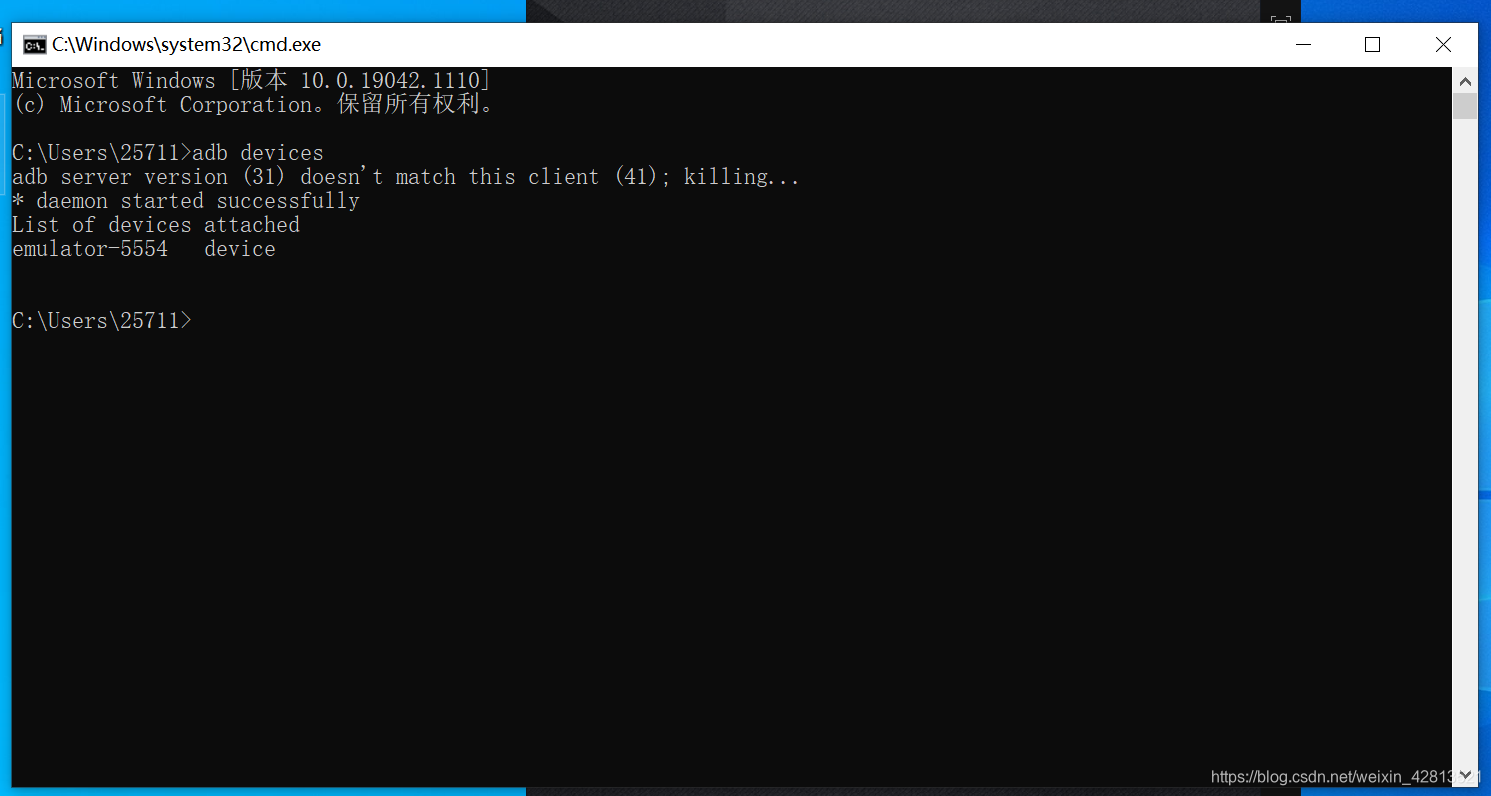 有了上面的工具就可以进行安卓设备的测试了!
连接方法: 1.通过WIFI连接,这需要你的设备在同一个网络下: 假设设备的ip为11.1.1.1
import uiautomator2 as u2
d = u2.connect('http://11.1.1.1')
2.USB,使用设备的序列号进行连接,这样在连接多个设备的时候就可以有选择的测试某个设备:
from uiautomator import Device
d = Device('emulator-5554')
Note:在下面的例子里,我们使用d代表安卓设备
基本API的使用
本部分通过一些简单的例子展示了设备的一些操作。 检索设备信息
import uiautomator2 as u2
d=u2.connect(r'emulator-5554')
print(d.info)
下面是运行的结果 设备的关键事件操作 安卓设备的开关
d.screen.on()
d.screen.off()
d.unlock()
检查设备是开还是关
d.info.get('screenOn')
按键操作
d.press("home")
d.press("back")
d.press(0x07, 0x02)
此外还支持以下按键操作: home back left right up down center menu search enter delete ( or del) recent (recent apps) volume_up volume_down volume_mute camera power
设备的手势交互
点击屏幕
d.click(x, y)
长时间点击屏幕
d.long_click(x, y)
d.long_click(x, y, 0.5)
滑动屏幕
d.swipe(sx, sy, ex, ey)
d.swipe(sx, sy, ex, ey, steps=10)
d.swipe(sx, sy, ex, ey, 0.5)
拖拽屏幕
d.drag(sx, sy, ex, ey)
d.drag(sx, sy, ex, ey, steps=10)
d.drag(sx, sy, ex, ey, 0.5)
屏幕操作
检索方向
orientation=d.orientation
设置方向
d.set_orientation("n")
d.set_orientation("l")
d.set_orientation("r")
冻结/解冻旋转
d.freeze_rotation()
d.freeze_rotation(False)
截图
d.screenshot("C:/Users/25711/Desktop/kg/demo.png")
image=d.screenshot()
image.save("C:/Users/25711/Desktop/kg/demo.png")
import cv2
image=d.screenshot(format='opencv')
cv2.imwrite("C:/Users/25711/Desktop/kg/demo.png",image)
存储窗口层次结构
xml=d.dump_hierarchy()
打开通知或快速设置
d.open_notification()
d.open_quick_setting()
推送和提取文件
将文件推送到设备
d.push("foot.txt","/data/")
d.push("foo.txt"."/data/data.txt")
with open("foo.txt","rb") as f:
d.push(f,"/data/")
d.push("foo.sh","/data/local/tmp/",mode=0o755)
从设备中提取文件
d.pull("/data/tmp.txt","tmp.txt")
d.pull("/data/some_not_exist.txt","tmp.txt")
APP管理
APP的安装 目前仅支持从url安装
d.app_install(url)
APP启动
d.app_start("包名")
APP停止
d.app_stop("包名")
d.app_clear("包名")
停止所有APP的运行
d.app_stop_all()
d.app_stop_all(excludes=["包名"])
选择器Selecor
选择器用于识别当前窗口中的特定对象
d(text='Clock', className='android.widget.TextView')
选择器支持以下的参数 ? text, textContains, textMatches, textStartsWith ? className, classNameMatches ? description, descriptionContains, descriptionMatches, descriptionStartsWith ? checkable, checked, clickable, longClickable ? scrollable, enabled,focusable, focused, selected ? packageName, packageNameMatches ? resourceId, resourceIdMatches ? index, instance
获取儿孙和同级对象
儿孙级
d(className="android.widget.ListView").child(text="Bluetooth")
同级
d(text="Google").sibling(className="android.widget.ImageView")
通过text或description或instance,获取儿孙级的对象
d(className="android.widget.ListView", resourceId="android:id/list").child_by_text("Bluetooth", className="android.widget.LinearLayout")
d(className="android.widget.ListView", resourceId="android:id/list")
.child_by_text(
"Bluetooth",
allow_scroll_search=True,
className="android.widget.LinearLayout"
)
相对位置
我们可以使用相对位置的方法获取视图中的对象:left,right,top,bottom d(A).left(B) 表示选择A左侧的B d(A).right(B) 表选择A 右侧的B d(A).up(B), 表示选择A上方的B. d(A).down(B), 表示选择在A下面的B. 因此对于“WIFI”开关,我们可以编写以下的代码:
d(text="Wi Fi").right(className="android.widget.Switch").click()
多个实例
有时候屏幕上包含多个相同特点的多个对象,那么我们将在选择器中使用“instance”属性
d(text="Add new",instance=0)
uiautomator也提供了类似列表的方法来处理类似的元素对象
d(text="Add new").count
len(d(text="Add new"))
d(text="Add new")[0]
d(text="Add new")[1]
...
for data in d(text="Add new"):
data.info
在使用迭代器的时候,必须保证屏幕不变,否则会出现ui not found error
获取ui对象的状态和信息
检查制定ui对象是否存在
d(text="Setting").exists
d.exists(text="Settings")
检索制定ui对象的信息
d(text="Settings").info
设置/清除可编辑字段的text
d(text="Settings").clear_text()
d(text="Settings").set_text("my text..")
对指定ui执行click
单击指定ui object
d(text="Settings").click()
d(text="Settings").click(timeout=10)
d(text="Settings").tap()
长按指定ui对象
d(text="Setting").long_click()
指定ui object手势动作
将对象拖动到定点或另一个对象
d(text="Setting").drag_to(x,y,duration=0.5)
d(text="Settings").drag_to(text="Clock",duration=0.25)
两点手势
d(text="Settings").gesture((sx1, sy1), (sx2, sy2), (ex1, ey1), (ex2, ey2))
制定ui object 两点手势
支持两种手势 in 从边缘到中心 out 从中心到边缘
d(text="Settings").pinch_in(percent=100,steps=10)
d(text="Settings").pinch_out()
等待指定的ui对象出现或消失
d(text="Settings").wait(timeout=3.0)
d(text="Settings").wait_gone(timeout=1.0)
对(可滚动)ui对象执行fling
Fling(飞滑)一般用于水平或垂直翻页,可能的属性:horiz 或vert forward 或backward 或toBegining 或toEnd
d(scrollable=True).fling()
d(scrollable=True).fling.horiz.forward()
d(scrollable=True).fling.vert.backward()
d(scrollable=True).fling.h或iz.toBeginning(max_swipes=1000)
d(scrollable=True).fling.toEnd()
对(可滚动)ui对象执行scroll
可能的属性: horiz 或 vert forward 或 backward 或 toBeginning 或 toEnd, 或 to
d(scrollable=True).scroll(steps=10)
d(scrollable=True).scroll.h或iz.forward(steps=100)
d(scrollable=True).scroll.vert.backward()
d(scrollable=True).scroll.h或iz.toBeginning(steps=100, max_swipes=1000)
d(scrollable=True).scroll.toEnd()
d(scrollable=True).scroll.to(text="Security")
|