一、数字
1.将字符串转换为数字
a = "123"
print(type(a))
b = int(a)
print(type(b))
2.进制转换
num = "0011"
v = int(num, base=2)
print(v)
3.bit_length()方法
age1 = 5 //5的二进制表示为101
age2 = 10 //10的二进制表示为1010
r1 = age1.bit_length() //该方法求出该数字至少可用几位二进制数表示
r2 = age2.bit_length()
print(r1,r2)
4.n次方表示方法
a = 2 ** 3
print(a)
二、字符串
1.字符串常用方法
test = "zhangyixing"
a = test.capitalize()
b = test.casefold()
c = test.center(20)
d = test.center(20,"*")
e1 = test.count('i')
e2 = test.count('i',5)
f = test.endswith("n")
g1 = test.find('i')
g2 = test.find('in',5,8)
g3 = test.find('in',5,9)
2.format()方法
test2 = "I am {name}, age {a}"
h1 = test2.format(name='zhangyixing', a=29)
test2_1 = "I am {0}, age {1}"
h2 = test2_1.format('zhangyixing', 29)
h3 = test2.format_map({"name": 'alex', "a": 29})
print(test2)
print(h1)
print(h2)
print(h3)
3.索引、切片
test = “zhangyixing”
v1 = test[1]
print(v1)
v2 = test[0:1]
v2 = test[0:-1]
print(v2)
三、列表
li = ['alex', 'seven', 'eric']
li = list(['alex', 'seven', 'eric'])
li = [1, 2, 3, "age", "alex", [1, ["zhai", 21], 120], True]
列表常用操作有:append/extend/insert方法、索引、切片、循环等。
print(li[4])
print(li[3:5])
for item in li:
print(item)
del li[0]
print(li)
v = "age" in li
print(v)
将列表中的元素拼接起来有很多种情况:a.元素中包含多种类型;b.元素全是字符串;c.元素全是数字。
s = ""
for index in li:
s = s + str(index)
print(s)
li = ["zhang", "yi", "xing", "1991"]
v = "".join(li)
print(v)
li = [11, 22, 33, 44]
li.append("zhai")
li.append(2000)
print(li)
注:列表中的元素是有序的且可以被修改。
四、元组
元组是对列表的二次加工,一级元素不可被修改,不能被增加或者删除。
ages1 = (11, 22, 33, 44, 55)
ages2 = tuple((11, 22, 33, 44, 55))
元组也可以索引取值和切片取值:
tu1 = (11, 22, 33, 44, True, (111, 222), )
print(tu1)
v1 = tu1[0]
v2 = tu1[0:2]
print(v1)
print(v2)
tu2 = (1, 2, 3, "age", "alex", [1, ("zhang", 21), 120], True)
v = tu2[5][1][0]
print(v)
元组的一级元素不可被修改:
tu2[3] = 10
print(tu2)
tu2[5][1] = 1007
print(tu2)
字符串、列表、元组之间可以相互转换:
s = "zifuchuan"
li = ["liebiao", 2000]
tu = ("yuanzu", 1998)
t1 = tuple(s)
print(t1)
t2 = list(s)
print(t2)
t3 = tuple(li)
print(t3)
t4 = str(li)
print(t4)
t5 = list(tu)
print(t5)
t6 = str(tu)
print(t6)
运行结果为: 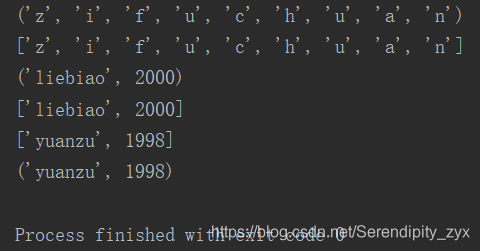
【练习题】
【例1】有两个列表l1 = [11,22,33]和l2 = [22,33,44],分别求a.获取内容相同的元素列表;b.获取 l1中有,l2中没有的元素列表;c.获取 l2中有,l1中没有的元素列表。
l1 = [11, 22, 33]
l2 = [22, 33, 44]
for item1 in l1:
if item1 in l2:
print(item1)
for item2 in l1:
if item2 not in l2:
print(item2)
for item3 in l2:
if item3 not in l1:
print(item3)
【例2】有1、2、3、4、5、6、7、8这8个数字,能组成多少互不相同且无重复数字的两位数。
li_01 = ["1", "2", "3", "4", "5", "6", "7", "8"]
count1 = 0
for index1 in li_01:
for index2 in li_01:
if index1 != index2:
count1 = count1 + 1
print(count1)
count2 = 0
for i in range(1, 9):
for v in range(1, 9):
if i != v:
count2 += 1
print(count2)
【例3】用while循环实现九九乘法表。
for i in range(1, 10):
for j in range(1, i + 1):
print("%d*%d=%d" % (j, i, j * i), end=" ")
print("")
i = 1
while i < 10:
j = 1
while j <= i:
print("%d*%d=%d" % (j, i, j * i), end=' ')
j += 1
print("")
i += 1
运行结果为: 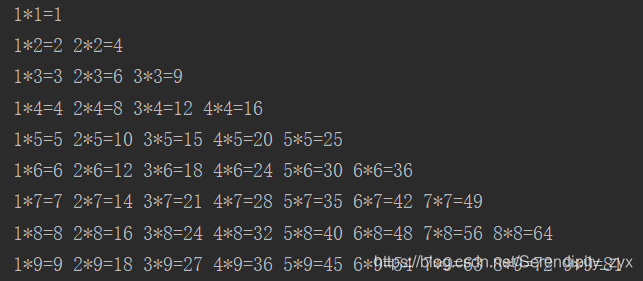
五、字典
person1 = {"name": "mr.wu", 'age': 18}
person2 = dict({"name": "mr.wu", 'age': 18})
字典元素是无序的。
info = {"k1": "zhang",
2: True,
"k3": [11, 22, 33, {
"kk1": "vv1",
"kk2": "vv2"
}],
"k4": (111, 222, 333),
True: "yu"
}
print(info)
v1 = info["k1"]
v2 = info[2]
v3 = info["k3"][3]["kk1"]
print(v1)
print(v2)
print(v3)
运行结果为: 字典是支持删除操作的。
del info["k4"]
print(info)
字典的for循环:
print("****第一种循环****")
for item in info:
print(item)
print("****第二种循环****")
for index in info.keys():
print(index, info[index])
print("****第三种循环****")
for k, v in info.items():
print(k, v)
运行结果为: 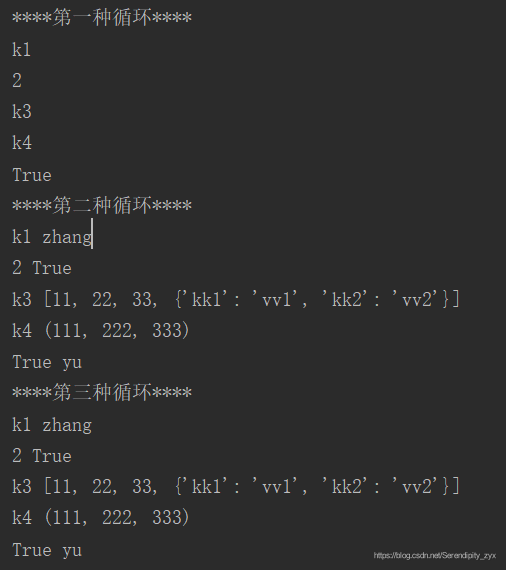 字典的常用方法: 1.fromkeys()
v1 = dict.fromkeys(["zhai", "yu", "xin"],1007)
print(v1)
2.get()
dic = {
"key01": "value01"
}
v2 = dic.get("key02", 2000)
print(v2)
3.setdefault()
v3 = dic.setdefault("key02", 'value02')
print(dic)
4.update()
dic.update(k1=123, k2=345)
print(dic)
5.keys()和items()
v4 = dic.keys()
print(v4)
v5 = dic.items()
print(v5)
6.in操作
v6 = "value01" in dic.values()
print(v6)
六、布尔值
【练习题】
【例1】元素分类:有如下值集合 [11,22,33,44,55,66,77,88,99,90],将所有大于等于 66 的值保存至字典的第一个key中,将小于 66 的值保存至第二个key的值中。即: {‘k1’: 大于等于66的所有值, ‘k2’: 小于66的所有值}。
li = [11, 22, 33, 44, 55, 66, 77, 88, 99, 90]
item1 = []
item2 = []
for index in li:
if index >= 66:
item1.append(index)
else:
item2.append(index)
dic = {
'k1': item1,
'k2': item2
}
print(dic)
【例2】查找:查找列表中元素,移除每个元素的空格,并查找以 a或A开头 并且以 c 结尾的所有元素。li = [“alec”, " aric", “Alex”, “Tony”, “rain”],tu = (“alec”, " aric", “Alex”, “Tony”, “rain”),dic = {‘k1’: “alex”, ‘k2’: ’ aric’, “k3”: “Alex”, “k4”: “Tony”}。
li = ["alec", " aric", "Alex", "Tony", "rain"]
tu = ("alec", " aric", "Alex", "Tony", "rain")
dic = {'k1': "alex", 'k2': ' Aric', "k3": "Alex", "k4": "Tony"}
for a in range(len(li)):
li[a] = li[a].replace(" ", "")
tu = list(tu)
for b in range(len(tu)):
tu[b] = tu[b].replace(" ", "")
tu = tuple(tu)
for c in dic:
dic[c] = dic[c].replace(" ", "")
for a1 in li:
if (a1.startswith("a") or a1.startswith("A")) and a1.endswith("c"):
print(a1)
for b1 in tu:
if (b1.startswith("a") or b1.startswith("A")) and b1.endswith("c"):
print(b1)
for c1 in dic:
c2 = dic[c1].strip()
if (c2.startswith("a") or c2.startswith("A")) and c2.endswith("c"):
print(c2)
【例3】打印输出商品列表,用户根据商品信息输入序号,页面显示用户选中的商品,li = [“手机”, “电脑”, ‘鼠标垫’, ‘游艇’]。
li = ["手机", "电脑", '鼠标垫', '游艇']
for key, i in enumerate(li, 0):
print(key, i)
user = int(input("请输入商品序号:"))
print(li[user])
运行结果为: 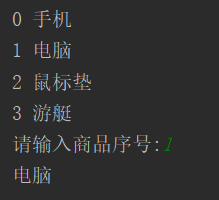 【例4】功能要求:①要求用户输入总资产,例如:2000;②显示商品列表:让用户根据序号选择商品,加入购物车;③购买:如果商品总额大于总资产,提示账户余额不足,否则,购买成功。
goods = [
{"name": "电脑", "price": 1999},
{"name": "鼠标", "price": 10},
{"name": "游艇", "price": 20},
{"name": "美女", "price": 998},
]
具体代码为:
goods = [
{"name": "电脑", "price": 1999},
{"name": "鼠标", "price": 10},
{"name": "游艇", "price": 20},
{"name": "美女", "price": 100}
]
count = 0
buymoney = 0
shopcar = []
def Shopping():
inputnum = input("--------------------\n请选择操作:\n1、查看资产\n2、查看商品\n3、查看购物车\n4、购买\n--------------------")
if inputnum == "1":
MyCount()
elif inputnum == "2":
Goods()
elif inputnum == "3":
ShopCar()
elif inputnum == "4":
pay()
else:
print("无此操作!")
Shopping()
def MyCount():
print("账户余额:" + str(count))
inputop = input("--------------------\n请选择操作:\n1、返回\n2、退出\n")
if inputop == "1":
Shopping()
if inputop == "2":
quit()
else:
print("无此操作!")
MyCount()
def Goods():
global buymoney
global shopcar
for i in range(len(goods)):
print(str(i + 1) + "、商品名称:" + goods[i]["name"] + " " + str(goods[i]["price"]) + "元")
inputop = input("(输入选择序号加入购物车)\n其他操作:\no、查看购物车\nq、退出\nr、返回\n--------------------")
if inputop == "1":
shopcar.append(goods[0])
print("已将【" + goods[int(inputop) - 1]["name"] + "】加入购物车\n--------------------")
buymoney = buymoney + goods[int(inputop) - 1]["price"]
elif inputop == "2":
shopcar.append(goods[1])
print("已将" + goods[int(inputop) - 1]["name"] + "加入购物车\n--------------------")
buymoney = buymoney + goods[int(inputop) - 1]["price"]
elif inputop == "3":
shopcar.append(goods[2])
print("已将" + goods[int(inputop) - 1]["name"] + "加入购物车\n--------------------")
buymoney = buymoney + goods[int(inputop) - 1]["price"]
elif inputop == "4":
shopcar.append(goods[3])
print("已将" + goods[int(inputop) - 1]["name"] + "加入购物车\n--------------------")
buymoney = buymoney + goods[int(inputop) - 1]["price"]
elif inputop == "o":
ShopCar()
elif inputop == "q":
exit()
elif inputop == "r":
Shopping()
else:
print("无此操作!")
Goods()
def ShopCar():
if len(shopcar) == 0:
print("购物车为空")
for item in shopcar:
print(item["name"] + " " + str(item["price"]))
def pay():
global count
global buymoney
if buymoney < count:
count = count - buymoney
print("您已经成功购买!")
print("账户余额为:" + str(count))
shopcar.clear()
else:
print("账户余额不足!")
count = int(input("请输入您的总资产:"))
Shopping()
七、集合
集合(set)是一个无序的不重复元素序列。可以使用大括号 { } 或者 set() 函数创建集合,注意:创建一个空集合必须用 set() 而不是 { },因为 { } 是用来创建一个空字典。
parame = {value01,value02,...}
s = set(['alex', 'alex', 'zhai'])
集合的内置方法: 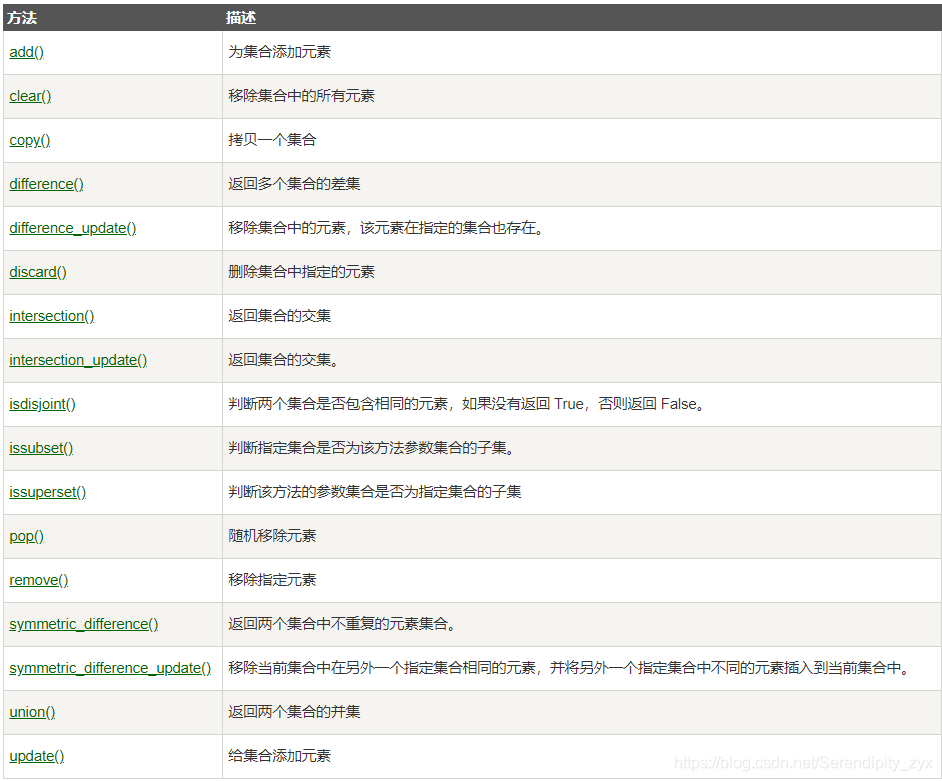 两个集合间的多种运算:
a = set('abracadabra')
b = set('alacazam')
print("集合a:", a)
print("集合b:", b)
print("集合a中包含而集合b中不包含的元素:")
print(a - b)
print("集合a或b中包含的所有元素:")
print(a | b)
print("集合a和b中都包含了的元素:")
print(a & b)
print("不同时包含于a和b的元素")
print(a ^ b)
运行结果为: 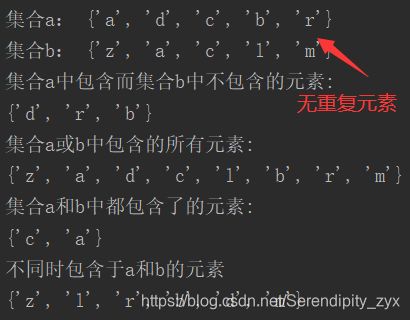 部分方法实例:
1.add()
s = {1, 2, 3, 4, 5, 6}
s.add('zhai')
2.clear()
s = {1, 2, 3, 4, 5, 6}
s.clear()
print(s)
3.copy()
s = {1, 2, 3, 4, 5, 6}
s1 = s.copy()
print(s1)
4.pop()
s = {1, 2, 3, 4, 5, 6, 'zhang', 'yi', 'xing'}
s.pop()
print(s)
5.remove()
s = {1, 2, 3, 4, 5, 6, 'zhang', 'yi', 'xing'}
s.remove('zhang')
print(s)
【补充知识】字符串格式化
1、百分号方式
①打印字符串和数字
str1 = 'I am %s ,my age is 21' % 'zhang'
print(str1)
str2 = 'I am %s,my hobby is %s,my age is %d' % ('zhang', 'reading', 21)
print(str2)
②打印float类
v1 = "percent %f" % 99.9999
print(v1)
v2 = "percent %.2f" % 99.987654
print(v2)
③打印百分比
v = "percent %.2f %%" % 99.9824
print(v)
④打印字典类型
tp = 'I am %(name)s, My age is %(age)d.' % {"name": "zhang", "age": 18}
print(tp)
2、Format方式
%[(name)][flags][width].[precision]typecode
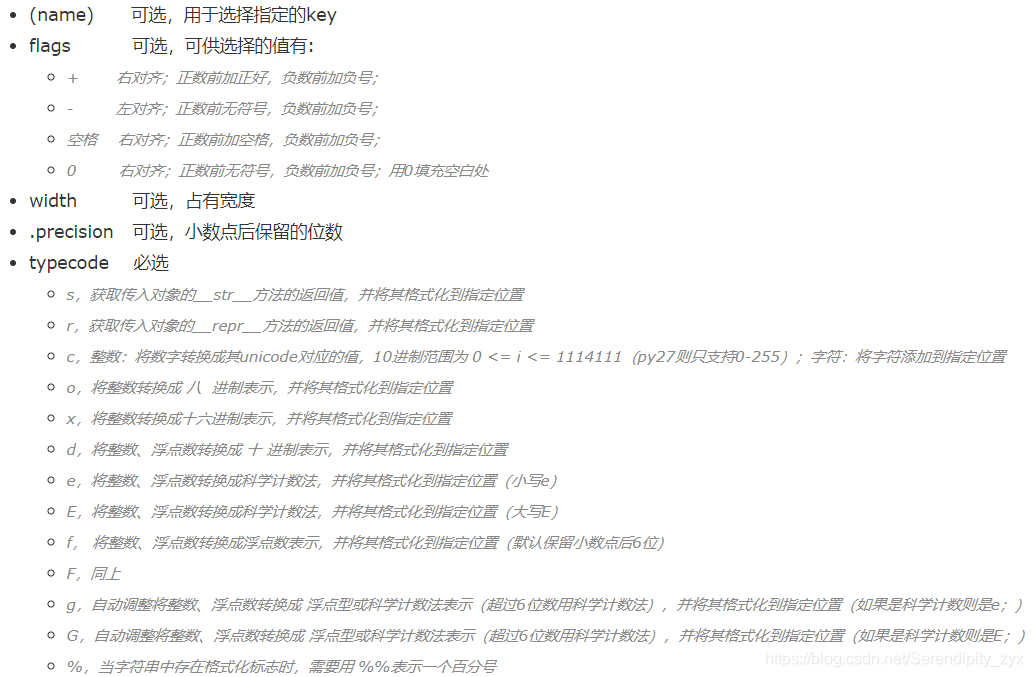
tpl = "i am {}, age {}, {}".format("seven", 18, 'alex')
tp2 = "i am {}, age {}, {}".format(*["seven", 18, 'alex'])
tp3 = "i am {0}, age {1}, really {0}".format("seven", 18)
tp4 = "i am {0}, age {1}, really {0}".format(*["seven", 18])
tp5 = "i am {name}, age {age}, really {name}".format(name="seven", age=18)
tp6 = "i am {name}, age {age}, really {name}".format(**{"name": "seven", "age": 18})
tp7 = "i am {0[0]}, age {0[1]}, really {0[2]}".format([1, 2, 3], [11, 22, 33])
tp8 = "i am {:s}, age {:d}, money {:f}".format("seven", 18, 88888.1)
tp9 = "i am {:s}, age {:d}".format(*["seven", 18])
tp_l0 = "i am {name:s}, age {age:d}".format(name="seven", age=18)
tp_l1 = "i am {name:s}, age {age:d}".format(**{"name": "seven", "age": 18})
tp_l2 = "numbers: {:b},{:o},{:d},{:x},{:X}, {:%}".format(15, 15, 15, 15, 15, 15.87623, 2)
tp_l3 = "numbers: {:b},{:o},{:d},{:x},{:X}, {:%}".format(15, 15, 15, 15, 15, 15.87623, 2)
tp_l4 = "numbers: {0:b},{0:o},{0:d},{0:x},{0:X}, {0:%}".format(15)
tp_l5 = "numbers: {num:b},{num:o},{num:d},{num:x},{num:X}, {num:%}".format(num=15)
运行结果为: 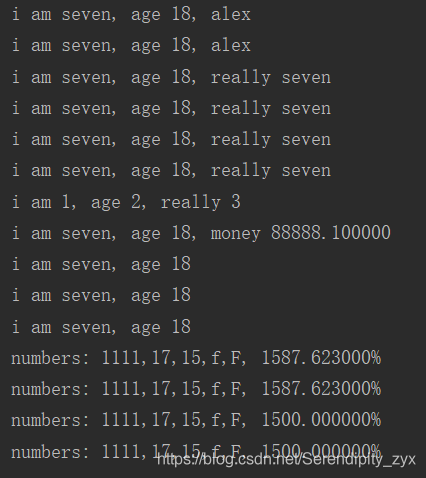
|