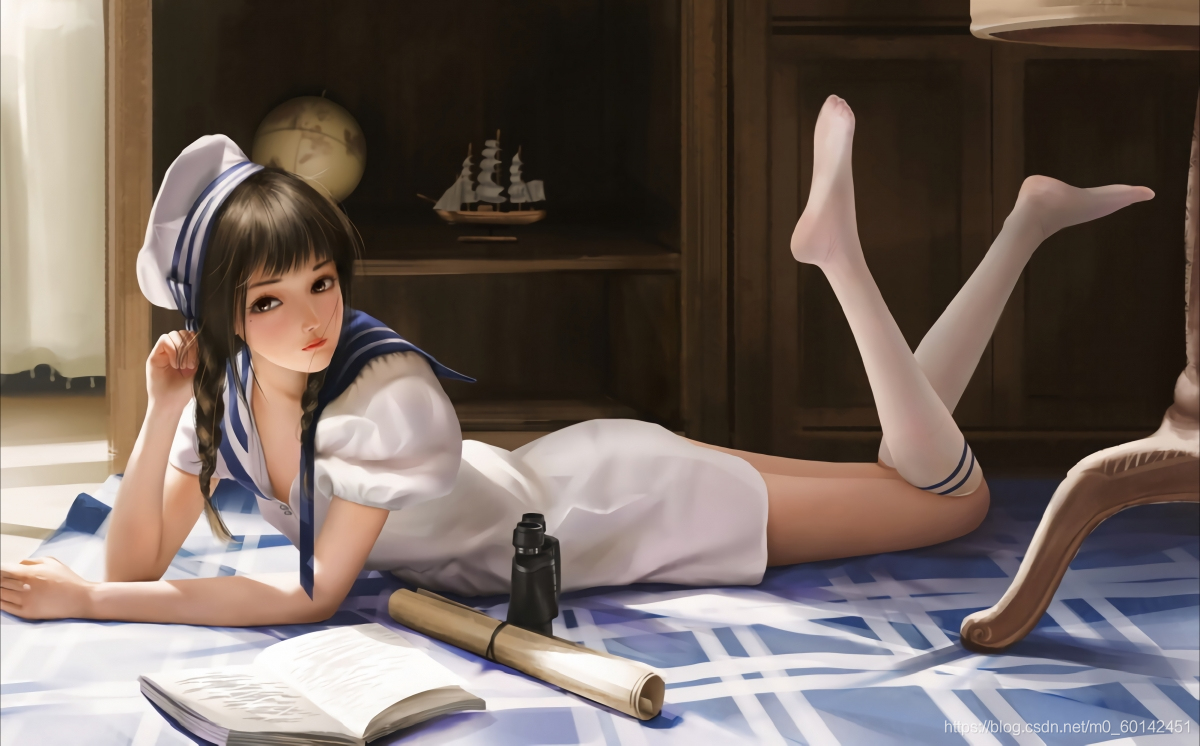
目录
1、交换变量
2、if 语句在行内
3、使用字典来存储选择操作
4、一行代码计算任何数的阶乘
5、连接
6、计算技巧
7、找到列表中出现最频繁的数
8、重置递归限制
9、原地交换两个数字
10、链状比较操作符
11、使用slots来减少内存开支
12、使用lambda来模仿输出方法
13、从两个相关的序列构建一个字典
14、一行代码搜索字符串的多个前后缀
15、在Python中实现一个真正的switch-case语句
16、多行字符串
17、两个列表同时迭代
18、存储列表元素到新的变量中
19、四种翻转字符串/列表的方式
20、数值比较
21、玩转枚举
22、在python中使用枚举量
23、从方法中返回多个值
24、打印引入模块的文件路径
25、带索引的列表迭代
26、列表推导
27、用下面的代替
28、字典推导
29、初始化列表的值
30、将列表转换成字符串
31、从字典中获取元素
32、获取子列表
33、60个字符解决FizzBuzz
34、集合
35、迭代工具
36、交互环境下的"_"操作符
37、字典/集合推导式
38、调试脚本
39、开启文件分享
40、简化if语句
41、组合多个字符串
42、 不使用循环构造一个列表
43、原地交换两个数字
44、链状比较操作符
45、使用三元操作符来实现条件赋值
46、多行字符串
47、存储列表元素到新的变量
48、打印引入模块的绝对路径
49、交互环境下的“_”操作符
50、字典/集合推导
51、调试脚本
52、开启文件分享
53、简化if语句
54、组合多个字符串
55、用枚举在循环中找到索引
56、定义枚举量
57、从方法中返回多个值
58、使用*运算符unpack函数参数
59、用字典来存储表达式
60、计算任何数的阶乘
61、找到列表中出现次数最多的数
62、重置递归限制
63、检查一个对象的内存使用
64、使用slots减少内存开支
65、用lambda 来模仿输出方法
66、从两个相关序列构建一个字典
67、搜索字符串的多个前后缀
68、不使用循环构造一个列表
69、实现switch-case语句
1、交换变量
有时候,当我们要交换两个变量的值时,一种常规的方法是创建一个临时变量,然后用它来进行交换。例:
# 输入
a = 5
b = 10
#创建临时变量
temp = a
a = b
b = temp
print(a)
print(b)
2、if 语句在行内
print "Hello" if True else "World"
>>> Hello
3、使用字典来存储选择操作
我们能构造一个字典来存储表达式:
In [70]: stdacl = {
...: 'sum':lambda x,y : x + y,
...: 'subtract':lambda x,y : x - y
...: }
In [73]: stdacl['sum'](9,3)
Out[73]: 12
In [74]: stdacl['subtract'](9,3)
Out[74]: 6
4、一行代码计算任何数的阶乘
python3环境:
In [75]: import functools
In [76]: result = ( lambda k : functools.reduce(int.__mul__,range(1,k+1),1))(3)
In [77]: result
Out[77]: 6
5、连接
下面的最后一种方式在绑定两个不同类型的对象时显得很酷。
nfc = ["Packers", "49ers"]
afc = ["Ravens", "Patriots"]
print nfc + afc
>>> [''Packers'', ''49ers'', ''Ravens'', ''Patriots'']
print str(1) + " world"
>>> 1 world
print `1` + " world"
>>> 1 world
print 1, "world"
>>> 1 world
print nfc, 1
>>> [''Packers'', ''49ers''] 1
6、计算技巧
#向下取整
print 5.0//2
>>> 2
# 2的5次方
print 2**5
>> 32
注意浮点数的除法
print .3/.1
>>> 2.9999999999999996
print .3//.1
>>> 2.0
7、找到列表中出现最频繁的数
In [82]: test = [1,2,3,4,2,2,3,1,4,4,4]
In [83]: print(max(set(test),key=test.count))
4
8、重置递归限制
Python 限制递归次数到 1000,我们可以重置这个值:
import sys
x=1001
print(sys.getrecursionlimit())
sys.setrecursionlimit(x)
print(sys.getrecursionlimit())
#1-> 1000
#2-> 100
9、原地交换两个数字
Python 提供了一个直观的在一行代码中赋值与交换(变量值)的方法,请参见下面的示例:
In [1]: x,y = 10 ,20
In [2]: print(x,y)
10 20
In [3]: x, y = y, x
In [4]: print(x,y)
20 10
10、链状比较操作符
比较操作符的聚合是另一个有时很方便的技巧:
In [5]: n = 10
In [6]: result = 1 < n < 20
In [7]: result
Out[7]: True
In [8]: result = 1 > n <= 9
In [9]: result
Out[9]: False
11、使用slots来减少内存开支
你是否注意到你的 Python 应用占用许多资源特别是内存?有一个技巧是使用 slots 类变量来在一定程度上减少内存开支。
```python
import sys
class FileSystem(object):
def __init__(self, files, folders, devices):
self.files = files
self.folders = folders
self.devices = devices
print(sys.getsizeof( FileSystem ))
class FileSystem1(object):
__slots__ = ['files', 'folders', 'devices']
def __init__(self, files, folders, devices):
self.files = files
self.folders = folders
self.devices = devices
print(sys.getsizeof( FileSystem1 ))
#In Python 3.5
#1-> 1016
#2-> 888
12、使用lambda来模仿输出方法
In [89]: import sys
In [90]: lprint = lambda *args: sys.stdout.write("".join(map(str,args)))
In [91]: lprint("python","tips",1000,1001)
Out[91]: pythontips1000100118
13、从两个相关的序列构建一个字典
In [92]: t1 = (1,2,3)
In [93]: t2 =(10,20,30)
In [94]: dict(zip(t1,t2))
Out[94]: {1: 10, 2: 20, 3: 30}
14、一行代码搜索字符串的多个前后缀
In [95]: print("http://www.google.com".startswith(("http://", "https://")))
True
In [96]: print("http://www.google.co.uk".endswith((".com", ".co.uk")))
True
15、在Python中实现一个真正的switch-case语句
下面的代码使用一个字典来模拟构造一个switch-case。
In [104]: def xswitch(x):
...: return xswitch._system_dict.get(x, None)
...:
In [105]: xswitch._system_dict = {'files': 10, 'folders': 5, 'devices': 2}
In [106]: print(xswitch('default'))
None
In [107]: print(xswitch('devices'))
2
16、多行字符串
基本的方式是使用源于 C 语言的反斜杠:
In [20]: multistr = " select * from multi_row \
...: where row_id < 5"
In [21]: multistr
Out[21]: ' select * from multi_row where row_id < 5'
另一个技巧是使用三引号
In [23]: multistr ="""select * from multi_row
...: where row_id < 5"""
In [24]: multistr
Out[24]: 'select * from multi_row \nwhere row_id < 5'
上面方法共有的问题是缺少合适的缩进,如果我们尝试缩进会在字符串中插入空格。所以最后的解决方案是将字符串分为多行并且将整个字符串包含在括号中:
In [25]: multistr = ("select * from multi_row "
...: "where row_id < 5 "
...: "order by age")
In [26]: multistr
Out[26]: 'select * from multi_row where row_id < 5 order by age'
17、两个列表同时迭代
nfc = ["Packers", "49ers"]
afc = ["Ravens", "Patriots"]
for teama, teamb in zip(nfc, afc):
print teama + " vs. " + teamb
>>> Packers vs. Ravens
>>> 49ers vs. Patriots
18、存储列表元素到新的变量中
我们可以使用列表来初始化多个变量,在解析列表时,变量的数目不应该超过列表中的元素个数:【译者注:元素个数与列表长度应该严格相同,不然会报错】
In [27]: testlist = [1,2,3]
In [28]: x,y,z = testlist
In [29]: print(x,y,z)
1 2 3
19、四种翻转字符串/列表的方式
翻转列表本身
In [49]: testList = [1, 3, 5]
In [50]: testList.reverse()
In [51]: testList
Out[51]: [5, 3, 1]
在一个循环中翻转并迭代输出
In [52]: for element in reversed([1,3,5]):
...: print(element)
...:
5
3
1
一行代码翻转字符串
In [53]: "Test Python"[::-1]
Out[53]: 'nohtyP tseT'
??
使用切片翻转列表
[1, 3, 5][::-1]
20、数值比较
x = 2
if 3 > x > 1:
print x
>>> 2
if 1 < x > 0:
print x
>>> 2
21、玩转枚举
使用枚举可以在循环中方便地找到(当前的)索引:
In [54]: testList= [10,20,30]
In [55]: for i,value in enumerate(testList):
...: print(i,':',value)
...:
0 : 10
1 : 20
2 : 30
22、在python中使用枚举量
我们可以使用下面的方式来定义枚举量:
In [56]: class Shapes:
...: Circle,Square,Triangle,Quadrangle = range(4)
...:
In [57]: Shapes.Circle
Out[57]: 0
In [58]: Shapes.Square
Out[58]: 1
In [59]: Shapes.Triangle
Out[59]: 2
In [60]: Shapes.Quadrangle
Out[60]: 3
23、从方法中返回多个值
并没有太多编程语言支持这个特性,然而 Python 中的方法确实(可以)返回多个值,请参见下面的例子来看看这是如何工作的:
In [61]: def x():
...: return 1,2,3,4
...:
In [62]: a,b,c,d = x()
In [63]: print(a,b,c,d)
1 2 3 4
24、打印引入模块的文件路径
如果你想知道引用到代码中模块的绝对路径,可以使用下面的技巧:
In [30]: import threading
In [31]: import socket
In [32]: print(threading)
<module 'threading' from '/usr/local/lib/python3.5/threading.py'>
In [33]: print(socket)
<module 'socket' from '/usr/local/lib/python3.5/socket.py'>
???
25、带索引的列表迭代
teams = ["Packers", "49ers", "Ravens", "Patriots"]
for index, team in enumerate(teams):
print index, team
>>> 0 Packers
>>> 1 49ers
>>> 2 Ravens
>>> 3 Patriots
26、列表推导
已知一个列表,刷选出偶数列表方法:
numbers = [1,2,3,4,5,6]
even = []
for number in numbers:
if number%2 == 0:
even.append(number)
27、用下面的代替
numbers = [1,2,3,4,5,6]
even = [number for number in numbers if number%2 == 0]
28、字典推导
teams = ["Packers", "49ers", "Ravens", "Patriots"]
print {key: value for value, key in enumerate(teams)}
>>> {''49ers'': 1, ''Ravens'': 2, ''Patriots'': 3, ''Packers'': 0}
29、初始化列表的值
items = [0]*3
print items
>>> [0,0,0]
30、将列表转换成字符串
teams = ["Packers", "49ers", "Ravens", "Patriots"]
print ", ".join(teams)
>>> ''Packers, 49ers, Ravens, Patriots''
31、从字典中获取元素
不要用下列的方式
data = {''user'': 1, ''name'': ''Max'', ''three'': 4}
try:
is_admin = data[''admin'']
except KeyError:
is_admin = False
替换为
data = {''user'': 1, ''name'': ''Max'', ''three'': 4}
is_admin = data.get(''admin'', False)
32、获取子列表
x = [1,2,3,4,5,6]
#前3个
print x[:3]
>>> [1,2,3]
#中间4个
print x[1:5]
>>> [2,3,4,5]
#最后3个
print x[-3:]
>>> [4,5,6]
#奇数项
print x[::2]
>>> [1,3,5]
#偶数项
print x[1::2]
>>> [2,4,6]
33、60个字符解决FizzBuzz
前段时间Jeff Atwood 推广了一个简单的编程练习叫FizzBuzz,问题引用如下:
写一个程序,打印数字1到100,3的倍数打印“Fizz”来替换这个数,5的倍数打印“Buzz”,对于既是3的倍数又是5的倍数的数字打印“FizzBuzz”。
这里有一个简短的方法解决这个问题:
for x in range(101):print"fizz"[x%34::]+"buzz"[x%54::]or x
34、集合
用到Counter库
from collections import Counter
print Counter("hello")
>>> Counter({''l'': 2, ''h'': 1, ''e'': 1, ''o'': 1})
35、迭代工具
和collections库一样,还有一个库叫itertools
from itertools import combinations
teams = ["Packers", "49ers", "Ravens", "Patriots"]
for game in combinations(teams, 2):
print game
>>> (''Packers'', ''49ers'')
>>> (''Packers'', ''Ravens'')
>>> (''Packers'', ''Patriots'')
>>> (''49ers'', ''Ravens'')
>>> (''49ers'', ''Patriots'')
>>> (''Ravens'', ''Patriots'')
在python中,True和False是全局变量,因此:
False = True
if False:
print "Hello"
else:
print "World"
>>> Hello
36、交互环境下的"_"操作符
这是一个我们大多数人不知道的有用特性,在 Python 控制台,不论何时我们测试一个表达式或者调用一个方法,结果都会分配给一个临时变量: _(一个下划线)。
In [34]: 2 + 3
Out[34]: 5
In [35]: _
Out[35]: 5
In [36]: print(_)
5
“_” 是上一个执行的表达式的输出。
37、字典/集合推导式
与我们使用的列表推导相似,我们也可以使用字典/集合推导,它们使用起来简单且有效,下面是一个例子:
In [37]: testDict = {i : i*i for i in range(5)}
In [38]: testSet = { i*2 for i in range(5)}
In [39]: testDict
Out[39]: {0: 0, 1: 1, 2: 4, 3: 9, 4: 16}
In [40]: testSet
Out[40]: {0, 2, 4, 6, 8}
? 注:两个语句中只有一个 <:> 的不同,另,在 Python3 中运行上述代码时,将 改为 。
38、调试脚本
我们可以在 模块的帮助下在 Python 脚本中设置断点,下面是一个例子:
import pdb
pdb.set_trace()
???
我们可以在脚本中任何位置指定 <pdb.set_trace()> 并且在那里设置一个断点,相当简便。
39、开启文件分享
Python 允许运行一个 HTTP 服务器来从根路径共享文件,下面是开启服务器的命令:(python3环境)
python3 -m http.server
上面的命令会在默认端口也就是 8000 开启一个服务器,你可以将一个自定义的端口号以最后一个参数的方式传递到上面的命令中。
Paste_Image.png
40、简化if语句
我们可以使用下面的方式来验证多个值:
if m in [1,3,5,7]:
而不是
if m==1 or m==3 or m==5 or m==7:
或者,对于 in 操作符我们也可以使用 ‘{1,3,5,7}’ 而不是 ‘[1,3,5,7]’,因为 set 中取元素是 O(1) 操作。 27、运行时检测Python版本
当正在运行的 Python 低于支持的版本时,有时我们也许不想运行我们的程序。为达到这个目标,你可以使用下面的代码片段,它也以可读的方式输出当前 Python 版本:
import sys
#Detect the Python version currently in use.
if not hasattr(sys, "hexversion") or sys.hexversion != 50660080:
print("Sorry, you aren't running on Python 3.5n")
print("Please upgrade to 3.5.n")
sys.exit(1)
#Print Python version in a readable format.
print("Current Python version: ", sys.version)
或者你可以使用 sys.version_info >= (3, 5) 来替换上面代码中的 sys.hexversion != 50660080,这是一个读者的建议。
python3运行结果:
Python 3.5.1 (default, Dec 2015, 13:05:11)
[GCC 4.8.2] on linux
Current Python version: 3.5.2 (default, Aug 22 2016, 21:11:05)
[GCC 5.3.0]
41、组合多个字符串
如果你想拼接列表中的所有记号,比如下面的例子:
In [44]: test = ['I', 'Like', 'Python', 'automation']
In [45]: ''.join(test)
Out[45]: 'ILikePythonautomation'
???
42、 不使用循环构造一个列表
In [101]: test = [[-1, -2], [30, 40], [25, 35]]
In [102]: import itertools
In [103]: print(list(itertools.chain.from_iterable(test)))
[-1, -2, 30, 40, 25, 35]
43、原地交换两个数字
x, y =10, 20
print(x, y)
y, x = x, y
print(x, y)
10 20
20 10
44、链状比较操作符
n = 10
print(1 < n < 20)
print(1 > n <= 9)
True
False
45、使用三元操作符来实现条件赋值
[表达式为真的返回值] if [表达式] else [表达式为假的返回值]
y = 20
x = 9 if (y == 10) else 8
print(x)
找abc中最小的数
def small(a, b, c):
return a if a<b and a<c else (b if b<a and b<c else c)
print(small(1, 0, 1))
print(small(1, 2, 2))
print(small(2, 2, 3))
print(small(5, 4, 3))
# 列表推导
x = [m**2 if m>10 else m**4 for m in range(50)]
print(x)
[0, 1, 16, 81, 256, 625, 1296, 2401, 4096, 6561, 10000, 121, 144, 169, 196, 225, 256, 289, 324, 361, 400, 441, 484, 529, 576, 625, 676, 729, 784, 841, 900, 961, 1024, 1089, 1156, 1225, 1296, 1369, 1444, 1521, 1600, 1681, 1764, 1849, 1936, 2025, 2116, 2209, 2304, 2401]
46、多行字符串
multistr = "select * from multi_row \
where row_id < 5"
print(multistr)
select * from multi_row where row_id < 5
multistr = """select * from multi_row
where row_id < 5"""
print(multistr)
select * from multi_row
where row_id < 5
multistr = ("select * from multi_row"
"where row_id < 5"
"order by age")
print(multistr)
select * from multi_rowwhere row_id < 5order by age
47、存储列表元素到新的变量
testList = [1, 2, 3]
x, y, z = testList # 变量个数应该和列表长度严格一致
print(x, y, z)
1 2 3
48、打印引入模块的绝对路径
import threading
import socket
print(threading)
print(socket)
<module 'threading' from 'd:\\python351\\lib\\threading.py'>
<module 'socket' from 'd:\\python351\\lib\\socket.py'>
49、交互环境下的“_”操作符
在python控制台,不论我们测试一个表达式还是调用一个方法,结果都会分配给一个临时变量“_”
50、字典/集合推导
testDic = {i: i * i for i in range(10)}
testSet = {i * 2 for i in range(10)}
print(testDic)
print(testSet)
{0: 0, 1: 1, 2: 4, 3: 9, 4: 16, 5: 25, 6: 36, 7: 49, 8: 64, 9: 81}
{0, 2, 4, 6, 8, 10, 12, 14, 16, 18}
51、调试脚本
用pdb模块设置断点
import pdb
pdb.ste_trace()
52、开启文件分享
python允许开启一个HTTP服务器从根目录共享文件
python -m http.server
53、简化if语句
# use following way to verify multi values
if m in [1, 2, 3, 4]:
# do not use following way
if m==1 or m==2 or m==3 or m==4:
54、组合多个字符串
test = ["I", "Like", "Python"]
print(test)
print("".join(test))
['I', 'Like', 'Python']
ILikePython
55、用枚举在循环中找到索引
test = [10, 20, 30]
for i, value in enumerate(test):
print(i, ':', value)
0 : 10
1 : 20
2 : 30
56、定义枚举量
class shapes:
circle, square, triangle, quadrangle = range(4)
print(shapes.circle)
print(shapes.square)
print(shapes.triangle)
print(shapes.quadrangle)
57、从方法中返回多个值
def x():
return 1, 2, 3, 4
a, b, c, d = x()
print(a, b, c, d)
1 2 3 4
58、使用*运算符unpack函数参数
def test(x, y, z):
print(x, y, z)
testDic = {'x':1, 'y':2, 'z':3}
testList = [10, 20, 30]
test(*testDic)
test(**testDic)
test(*testList)
z x y
1 2 3
10 20 30
59、用字典来存储表达式
stdcalc = {
"sum": lambda x, y: x + y,
"subtract": lambda x, y: x - y
}
print(stdcalc["sum"](9, 3))
print(stdcalc["subtract"](9, 3))
12
6
60、计算任何数的阶乘
import functools
result = (lambda k: functools.reduce(int.__mul__, range(1, k+1), 1))(3)
print(result)
61、找到列表中出现次数最多的数
test = [1, 2, 3, 4, 2, 2, 3, 1, 4, 4, 4, 4]
print(max(set(test), key=test.count))
4
62、重置递归限制
python限制递归次数到1000,可以用下面方法重置
import sys
x = 1200
print(sys.getrecursionlimit())
sys.setrecursionlimit(x)
print(sys.getrecursionlimit())
63、检查一个对象的内存使用
import sys
x = 1
print(sys.getsizeof(x)) # python3.5中一个32比特的整数占用28字节
64、使用slots减少内存开支
import sys
# 原始类
class FileSystem(object):
def __init__(self, files, folders, devices):
self.files = files
self.folder = folders
self.devices = devices
print(sys.getsizeof(FileSystem))
# 减少内存后
class FileSystem(object):
__slots__ = ['files', 'folders', 'devices']
def __init__(self, files, folders, devices):
self.files = files
self.folder = folders
self.devices = devices
print(sys.getsizeof(FileSystem))
65、用lambda 来模仿输出方法
import sys
lprint = lambda *args: sys.stdout.write(" ".join(map(str, args)))
lprint("python", "tips", 1000, 1001)
66、从两个相关序列构建一个字典
t1 = (1, 2, 3)
t2 = (10, 20, 30)
print(dict(zip(t1, t2)))
{1: 10, 2: 20, 3: 30}
67、搜索字符串的多个前后缀
print("http://localhost:8888/notebooks/Untitled6.ipynb".startswith(("http://", "https://")))
print("http://localhost:8888/notebooks/Untitled6.ipynb".endswith((".ipynb", ".py")))
68、不使用循环构造一个列表
import itertools
import numpy as np
test = [[-1, -2], [30, 40], [25, 35]]
print(list(itertools.chain.from_iterable(test)))
[-1, -2, 30, 40, 25, 35]
69、实现switch-case语句
def xswitch(x):
return xswitch._system_dict.get(x, None)
xswitch._system_dict = {"files":10, "folders":5, "devices":2}
print(xswitch("default"))
print(xswitch("devices"))
①3000多本Python电子书有 ?②Python开发环境安装教程有 ?③Python400集自学视频有 ?④软件开发常用词汇有 ?⑤Python学习路线图有 ?⑥项目源码案例分享有 如果你用得到的话可以直接拿走,关注+私我留言
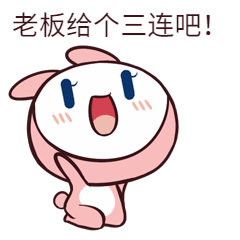
|