Python study notes Day 7 ‘function’
Today’s topic is about the function. This is an extremely important part in the world of computer programming. We can define a function by naming it and describe it in detail within the structure defined.
def function(): The contents of the function function()#The function is called
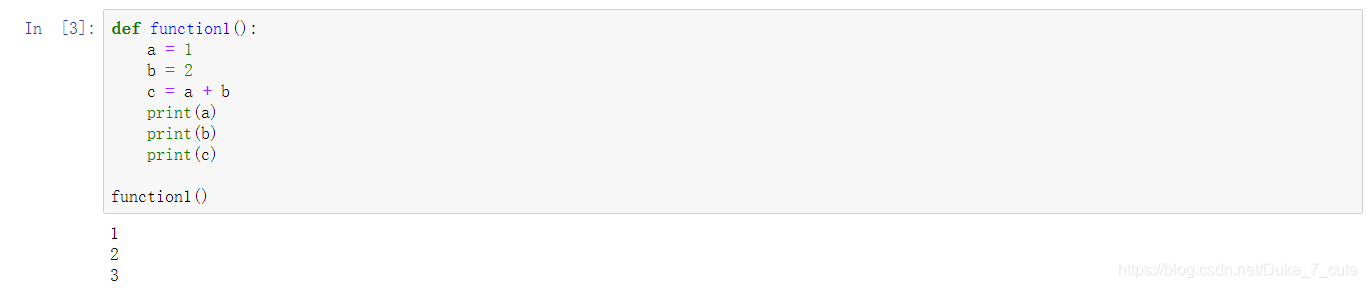
def function1():
a = 1
b = 2
c = a + b
print(a)
print(b)
print(c)
function1()
Or, we can define functions with parameters. Parameters are local variables that have the property of acting only inside the function. 
def function2(a,b):
c = a + b
print(a)
print(b)
print(c)
function2(3,4)
In the picture, ‘a’ and ‘b’ are parameters and when we call a function, we assign values to both parameters. Or, we can define and assign two global variables, and then assign the two global variables to the two parameters when the function is called. 
m = 5
n = 6
function2(m,n)
function2(n,m)
Or, we can also define a function by assigning default values to each parameter. 
def function3(a=7,b=8):
c = a + b
print(a)
print(b)
print(c)
function3()
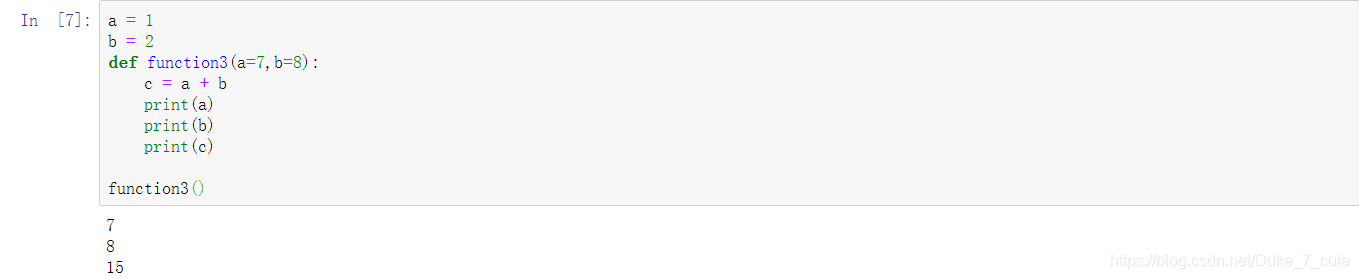
a = 1
b = 2
def function3(a=7,b=8):
c = a + b
print(a)
print(b)
print(c)
function3()
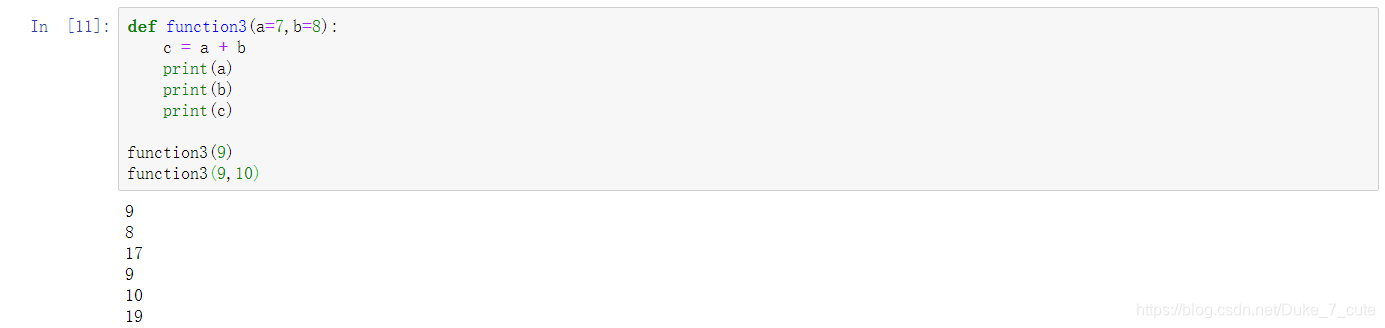
def function3(a=7,b=8):
c = a + b
print(a)
print(b)
print(c)
function3(9)
function3(9,10)
When we want to use global variables in a function: 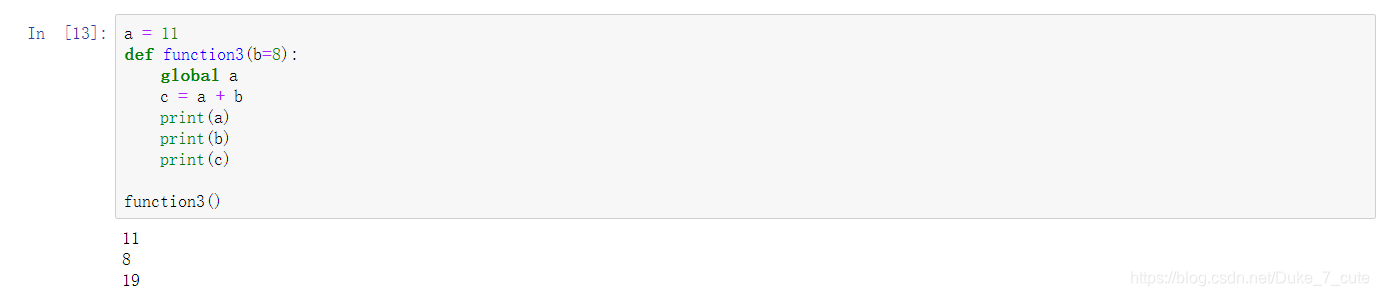
a = 11
def function3(b=8):
global a
c = a + b
print(a)
print(b)
print(c)
function3()
Sets the return value of the functionL 
def add(a,b):
c = a + b
return c
n = add(1,2)
print(n)
|