Python 将不同尺寸的图片和xml标签等比例缩放到指定大小
xml文件格式: 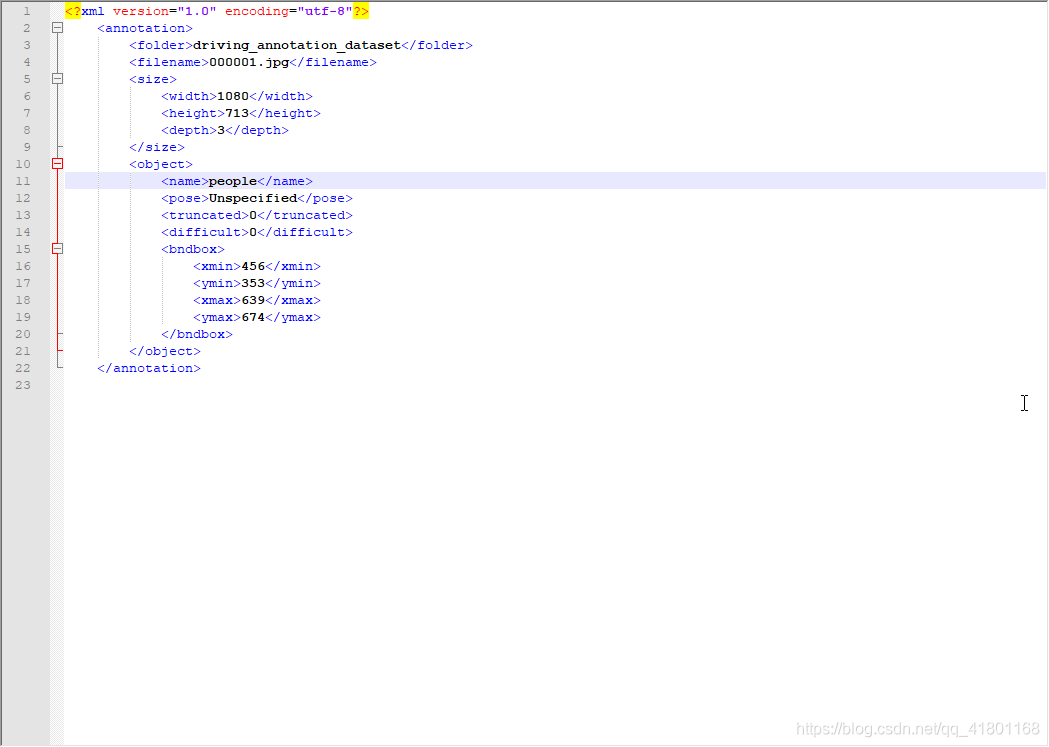
import os
import cv2
import numpy as np
import xml.etree.ElementTree as ET
path = r'C:\Users\1\Desktop\test\old'
newpath = r'C:\Users\1\Desktop\test\new'
c_w, c_h = 224, 224
def edit_xml(xml_file, ratio, i):
all_xml_file = os.path.join(path, xml_file)
tree = ET.parse(all_xml_file)
objs = tree.findall('object')
for obj in objs:
obj_bnd = obj.find('bndbox')
obj_bnd = obj.find('bndbox')
obj_xmin = obj_bnd.find('xmin')
obj_ymin = obj_bnd.find('ymin')
obj_xmax = obj_bnd.find('xmax')
obj_ymax = obj_bnd.find('ymax')
xmin = float(obj_xmin.text)
ymin = float(obj_ymin.text)
xmax = float(obj_xmax.text)
ymax = float(obj_ymax.text)
obj_xmin.text = str(round(xmin * ratio))
obj_ymin.text = str(round(ymin * ratio))
obj_xmax.text = str(round(xmax * ratio))
obj_ymax.text = str(round(ymax * ratio))
newfile = os.path.join(newpath, '%05d' % (0 + i) + '.xml')
tree.write(newfile, method='xml', encoding='utf-8')
if __name__ == '__main__':
files = os.listdir(path)
i = 0
for file in files:
img_zeros = np.zeros((c_w, c_h, 3), np.uint8)
if file.endswith('.jpg'):
imgName = os.path.join(path, file)
xml_file = file.replace('.jpg', '.xml')
img = cv2.imread(imgName)
h, w, _ = img.shape
if max(w, h) > c_w:
ratio = c_w / max(w, h)
imgcrop = cv2.resize(img, (round(w * ratio), round(h * ratio)))
img_zeros[0:round(h * ratio), 0:round(w * ratio)] = imgcrop
edit_xml(xml_file, ratio, i)
else:
img_zeros[0:h, 0:w] = img
edit_xml(xml_file, 1, i)
newName = os.path.join(newpath, '%05d' % (0 + i) + '.jpg')
i += 1
print(newName)
cv2.imwrite(newName, img_zeros)
参考链接:https://blog.csdn.net/qq_36563273/article/details/109580882
|