Python爬虫
爬虫基础知识
环境搭配
import urllib.request
import urllib.parse
获取一个get请求
获取一个get请求
response = urllib.request.urlopen("http://www.baidu.com")
print(response.read().decode('utf-8'))
获得一个post请求
data=bytes(urllib.parse.urlencode({"hello":"world"}),encoding="utf-8")
response = urllib.request.urlopen("http://www.httpbin.org/post",data=data)
print(response.read().decode("utf-8"))
超时处理
try:
response = urllib.request.urlopen("http://www.httpbin.org/get",timeout=0.01)
print(response.read().decode("utf-8"))
except urllib.error.URLError as e:
print(e)
进行伪装(伪装成浏览器爬取网页)
如果不进行伪装的话,服务器会判断是爬虫爬取的信息,进而阻止爬取,如下

所以我们要进行伪装:
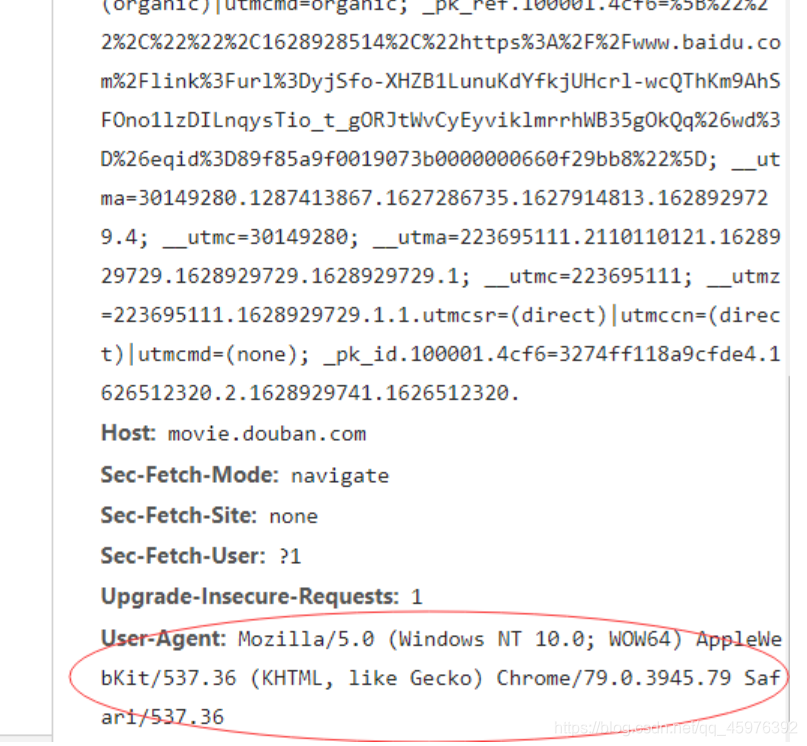
headers={
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/79.0.3945.79 Safari/537.36"
}
url = "https://movie.douban.com/top250"
req = urllib.request.Request(url=url,headers=headers)
response=urllib.request.urlopen(req)
print(response.read().decode("utf-8"))
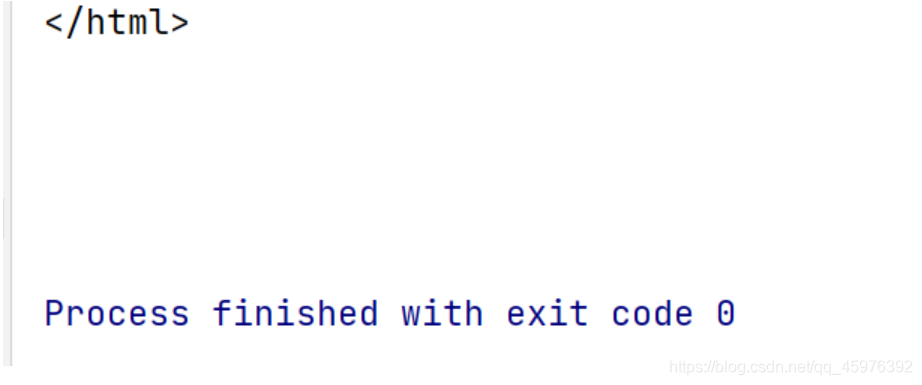
Beautifulsoup的使用
准备工作
from bs4 import BeautifulSoup
file=open("./baidu.html","rb")
html=file.read()
bs=BeautifulSoup(html,"html.parser")
Tag标签
拿到他所找到的第一个内容
print(bs.title)
NavigableString
标签里的内容(字符串)
print(bs.titile.string)
print(type(bs.title.string))
BeautifulSoup
表示整个文档
print(type(bs))
Comment
输出注释,是一个特殊的NavigableString ,输出的内容不包含注释符号
print(bs.a.string)
print(type(bs.a.string))
文档的遍历
print(bs.head.contents[1])
文档的搜索
字符串过滤
会查找与字符串完全匹配的内容
t_list = bs.findAll("a")
print(t_list)
正则表达式搜索
使用search() 方法来匹配内容
t_list = bs.findAll(re.compile("a"))
t_list = bs.find_all(text=re.compile("\d"))
方法:传入一个函数(方法),根据函数的要求搜索(了解)
def nexit(tag):
return tag.has_attr("name")
t_list=bs.find_all(nexit)
for i in t_list:
print(i)
kwargs 参数
查找属性等于特定值的tab
t_list=bs.find_all(id="top_div")
for i in t_list:
print(i)
t_list=bs.find_all(class_=True)
for i in t_list:
print(i)
text参数
查找text文本为特定值的tab
t_list=bs.find_all(text=["hao123","地图","贴吧"])
for i in t_list:
print(i)
limit参数
限制获取的个数(不含limit默认获取第一个)
t_list = bs.find_all("a",limit=3)
for i in t_list:
print(i)
css选择器
t_list=bs.select('title')
t_list=bs.select('#id')
t_list=bs.select('.class')
t_list=bs.select("li[class='bri']")
t_list=bs.select(".manv ~ .bri")
for item in t_list:
print(item.get_text())
正则表达式
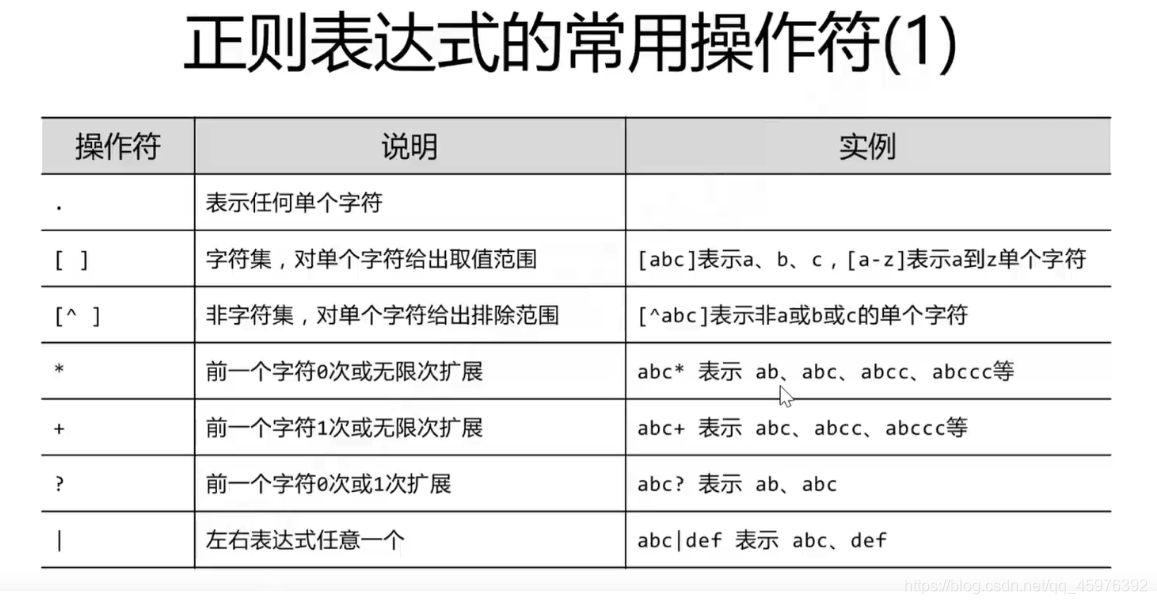
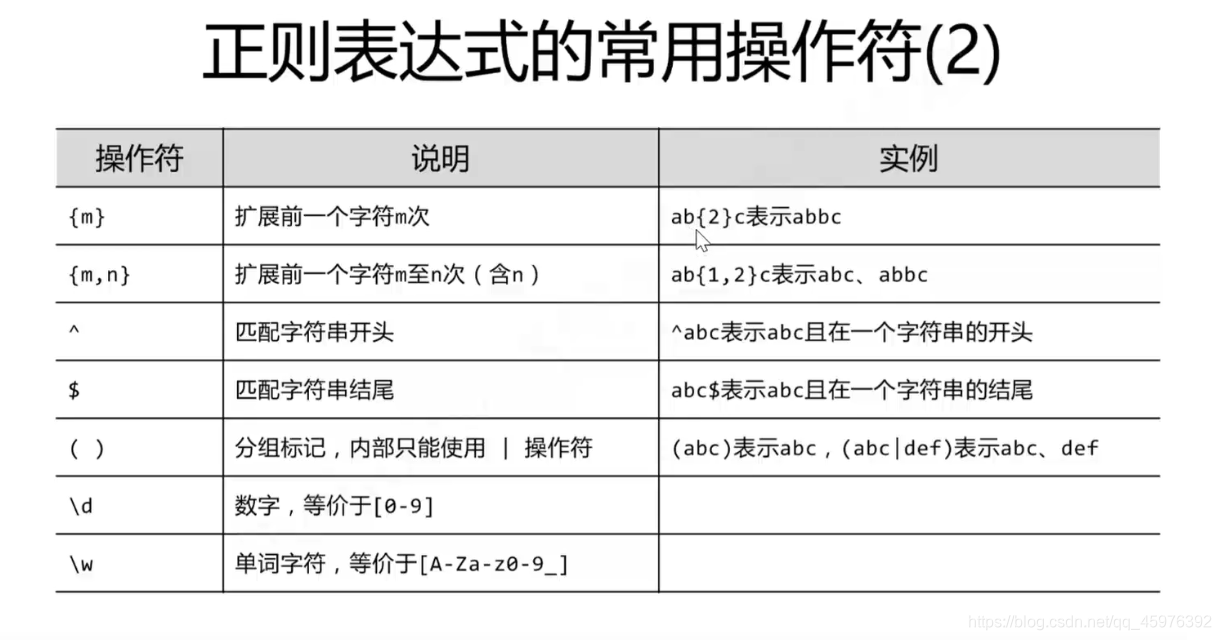
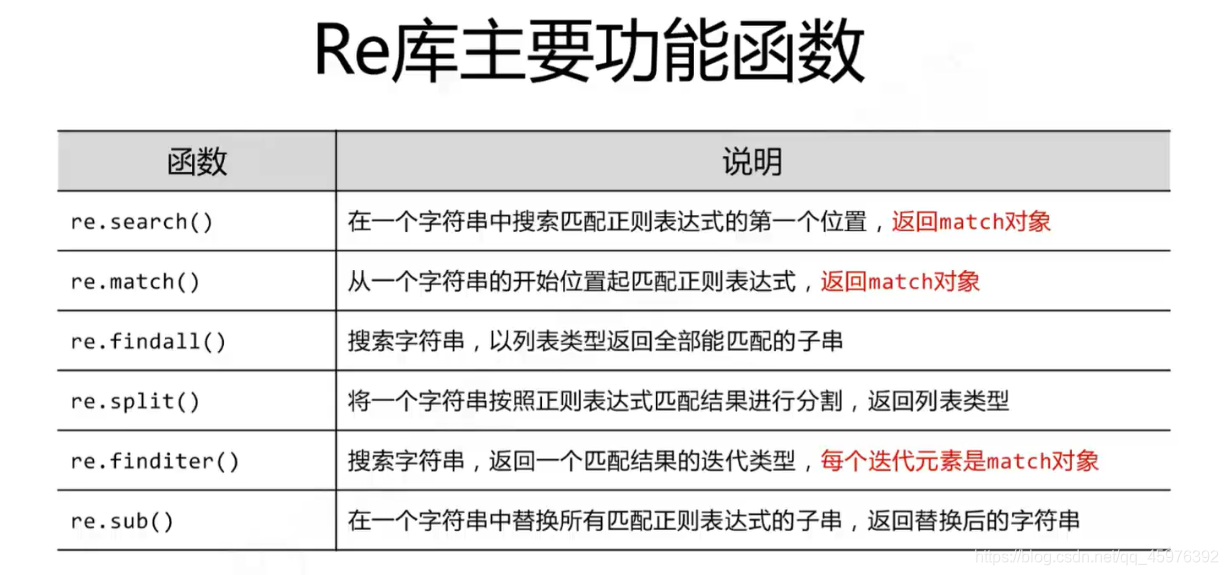
简单使用
import re
pat=re.compile("AA")
m=pat.search("CBAA")
print(m)
m=re.search("asd","Aasd")
m=re.findall("a","Aamawidjaiiosand")
m=re.findall("[A-Z]+","AamawidjaiiAWDAWDosand")
m=re.sub("a","A","ancioancoacasafacsa")
print(m)
|