书上代码和练习代码均有,不多说了,上代码:
python真的是越到后来越难以理解,这个时候的确需要有足够的意志力支撑自己把代码敲下来
第八章? ? ? ? 函数
'''def greet_user():
print("Hello!")
greet_user()'''
'''def greet_user(username):
print(f"Hello,{username.title()}!")
greet_user('jesse')'''
'''def greet_user(username):
print(f"Hello,{username.title()}!")
greet_user('sarah')
greet_user('jeese')'''
'''def display_message():
print("We are learning function now.")
display_message()'''
'''def capitalize(text):#每个字符首字母大写
return text.title()
str1="alice in wonderland"
def favorite_book(title):
print(f"\nOne of my favorite books is {title}.")
favorite_book(capitalize(str1))'''
#最简单的方式是str1.title()
'''def describe_pet(animal_type,pet_name):
print(f"\nI have a {animal_type}.")
print(f"My {animal_type}'s name is {pet_name.title()}.")
describe_pet('hamster','harry')
describe_pet('dog','willie')'''
'''def describe_pet(animal_type,pet_name):
print(f"\nI have a {animal_type}.")
print(f"My {animal_type}'s name is {pet_name.title()}.")
describe_pet(animal_type='hamster',pet_name='harry')
describe_pet(animal_type='dog',pet_name='willie')'''
'''def describe_pet(pet_name,animal_type='dog'):
print(f"\nI have a {animal_type}.")
print(f"My {animal_type}'s name is {pet_name.title()}.")
describe_pet('harry',animal_type='hamster')
describe_pet(animal_type='hamster',pet_name='harry')'''
'''def make_shirt(shirt_type,shirt_name='I love python'):
print(f"\nI have a {shirt_type} shirt,there has '{shirt_name}' on it.")
make_shirt('big')
make_shirt('small','I love C')
make_shirt(shirt_name='I love C++',shirt_type='medium')'''
'''def describe_city(city_name,city_country='China'):#要有单引号
print(f"{city_name} is in {city_country}.")
describe_city('Beijing')
describe_city('Shanghai')
describe_city('Reykjavik','Iceland')'''
'''def get_formatted_name(first_name,last_name):
full_name=f"{first_name} {last_name}"
return full_name.title()
musician=get_formatted_name('jimi','hendrix')
print(musician)'''
'''def get_formatted_name(first_name,last_name,middle_name=''):
if middle_name:
full_name=f"{first_name} {middle_name} {last_name}"
else:
full_name=f"{first_name} {last_name}"
return full_name.title()
musician=get_formatted_name('jimi','hendrix')
print(musician)
musician=get_formatted_name('john','hooker','lee')
print(musician)'''
'''def build_person(first_name,last_name):
person={'first':first_name,'last':last_name}
return person
musician=build_person('jimi','hendrix')
print(musician)'''
'''def build_person(first_name,last_name,age='',gender=''):
person={'first':first_name,'last':last_name}
if age:
person['age']=age
if gender:
person['gender']=gender
return person
musician=build_person('jimi','hendrix',age=27,gender='male')
print(musician)'''
'''def get_formatted_name(first_name,last_name):
full_name=f"{first_name} {last_name}"
return full_name.title()#放在末尾已经是另一个循环了
while True:
print("\nPlease tell me your name:")
print("(Enter 'q' at any time to quit)")
f_name=input("First name: ")
if f_name=='q':
break
l_name=input("last name: ")
if l_name=='q':
break
formatted_name=get_formatted_name(f_name,l_name)
print(f"Hello,{formatted_name}!")'''
'''def city_country(city_name,country):
shuchu=f"{city_name},{country}"
return shuchu.title()
city_xiangguan=city_country('santiago','chile')
print(city_xiangguan)'''
'''def city_country(city_name,country):
print(f"{city_name.title()},{country.title()}")
city_country('santiago','chile')
city_country('Beijing','china')
city_country('shanghai','china')'''
'''def make_album(singer_name,album_name,sing_number=None):
shuchu={'singer_name':singer_name,'album_name':album_name}
if sing_number:
shuchu['sing_number']=sing_number
return shuchu
while True:
print("Please input some messages:\n")
print("enter 'q' at any time to quit,enter 's' to skip the question of sing number")
s_name=input("singer name:")
if s_name=='q':
break
a_name=input("album name:")
if a_name=='q':
break
s_number=input("sing_number:")
if s_number=='q':
break
if s_number=='s':
album=make_album(s_name,a_name)
print(album)
continue
album = make_album(s_name, a_name,s_number)
print(album)'''
'''def greet_users(names):
for name in names:
msg=f"Hello, {name.title()}!"
print(msg)
usernames=['hannah','ty','margot']
greet_users(usernames)'''
#names为形参,usernames[]这个列表为实参(将列表传递给函数)
#在函数中修改列表
'''unprinted_designs=['phone case','robot pendant','dodecahedron']
completed_models=[]
while unprinted_designs:
current_design=unprinted_designs.pop()
print(f"Printing model:{current_design}")
completed_models.append(current_design)
print("\nThe following models have been printed:")
for completed_model in completed_models:
print(completed_model)'''
#维护更简单,效率更高的一段代码
#一个函数完成一项功能
'''def print_models(unprinted_designs,completed_models):
while unprinted_designs:
current_design = unprinted_designs.pop()
print(f"Printing model:{current_design}")
completed_models.append(current_design)
def show_completed_models(completed_models):
print("\nThe following models have been printed:")
for completed_model in completed_models:
print(completed_model)
unprinted_designs=['phone case','robot pendant','dodecahedron']
completed_models=[]
print_models(unprinted_designs,completed_models)
show_completed_models(completed_models)'''
#function_name(list_name[:])#把列表副本传递给函数,不清空未打印的设计列表
'''def print_models(unprinted_designs,completed_models):
while unprinted_designs:
current_design = unprinted_designs.pop()
print(f"Printing model:{current_design}")
completed_models.append(current_design)
def show_completed_models(completed_models):
print("\nThe following models have been printed:")
for completed_model in completed_models:
print(completed_model)
unprinted_designs=['phone case','robot pendant','dodecahedron']
completed_models=[]
print_models(unprinted_designs[:],completed_models)
show_completed_models(completed_models)'''
'''unprinted_messages=['phone case','robot pendant','dodecahedron']
printed_messages=[]
while unprinted_messages:
current_message=unprinted_messages.pop()
print(f"Printing model:{current_message}")
printed_messages.append(current_message)#变量值才是中间值
def show_message(printed_messages):
print("\nThe following models have been printed:")
for printed_message in printed_messages:
print(printed_message)
show_message(printed_messages)#要调用函数,函数才会被打印出来'''
'''def send_message(unprinted_messages,printed_messages):
while unprinted_messages:
current_message = unprinted_messages.pop()
print(f"Printing model:{current_message}")
printed_messages.append(current_message) # 变量值才是中间值
def show_message(printed_messages):
print("\nThe following models have been printed:")
for printed_message in printed_messages:
print(printed_message)
unprinted_messages=['phone case','robot pendant','dodecahedron']
printed_messages=[]
send_message(unprinted_messages[:],printed_messages)
show_message(printed_messages)
print(unprinted_messages)'''
#不留副本,但可以提高效率
'''def send_message(unprinted_messages,printed_messages):
while unprinted_messages:
current_message = unprinted_messages.pop()
print(f"Printing model:{current_message}")
printed_messages.append(current_message) # 变量值才是中间值
def show_message(printed_messages):
print("\nThe following models have been printed:")
for printed_message in printed_messages:
print(printed_message)
unprinted_messages=['phone case','robot pendant','dodecahedron']
printed_messages=[]
send_message(unprinted_messages,printed_messages)
show_message(printed_messages)
print(unprinted_messages)'''
'''def make_pizza(*toppings):
print(toppings)
make_pizza('pepperoni')
make_pizza('mushroom','green peppers','extra cheese')'''
'''def make_pizza(*toppings):
print("\nMaking a pizza with the following toppings:")
for topping in toppings:
print(f"-{topping}")
make_pizza('pepperoni')
make_pizza('mushroom','green peppers','extra cheese')'''
'''def make_pizza(size,*toppings):
print(f"\nMaking a {size}-inch pizza with the following toppings:")
for topping in toppings:
print(f"-{topping}")
make_pizza(16,'pepperoni')
make_pizza(12,'mushroom','green peppers','extra cheese')'''
'''def build_profile(first,last,**user_info):
user_info['first_name']=first
user_info['last_name']=last
return user_info
user_profile=build_profile('albert','einstein',
location='princeton',
field='physics')
print(user_profile)'''
'''def make_sandwiches(*toppings):
print("\nMaking a sandwich with the following toppings:")
for topping in toppings:
print(f"-{topping}")
make_sandwiches('pepperoni')
make_sandwiches('mushroom','green peppers','extra cheese')'''
'''def build_profile(first,last,**user_info):
user_info['first_name']=first.title()
user_info['last_name']=last.title()
return user_info
user_profile=build_profile('hou','yixin',
location='princeton',
field='physics',
hobby='reading'
)
print(user_profile)'''
'''def make_car(manufacturer,model,**user_info):
user_info['manufacturer']=manufacturer
user_info['model'] = model#信息,情报
return user_info
car_profile=make_car('subaru','outback',#侧面
color='blue',
tow_package=True)#拖包
print(car_profile)'''
#8.6开始
def make_pizza(size,*toppings):
print(f"\nMaking a {size}-inch pizza with the following toppings:")
for topping in toppings:
print(f"-{topping}")
'''import pizza
pizza.make_pizza(16,'pepperoni')
pizza.make_pizza(12,'mushroom','green peppers','extra cheese')'''
#module_name.function_name()使用其中的任意一个函数
#from module_name import function_name
'''from pizza import make_pizza
make_pizza(16,'pepperoni')
make_pizza(12,'mushroom','green peppers','extra cheese')'''
'''from pizza import make_pizza as mp
mp(16,'pepperoni')
mp(12,'mushroom','green peppers','extra cheese')'''
'''import pizza as p
p.make_pizza(16,'pepperoni')
p.make_pizza(12,'mushroom','green peppers','extra cheese')'''
'''from pizza import *
make_pizza(16,'pepperoni')
make_pizza(12,'mushroom','green peppers','extra cheese')'''
#写出多种形参的方式
'''def function_name(
qw_0,qw_1,qw_2
qw_0,qw_1,qw_2):
function body...'''
?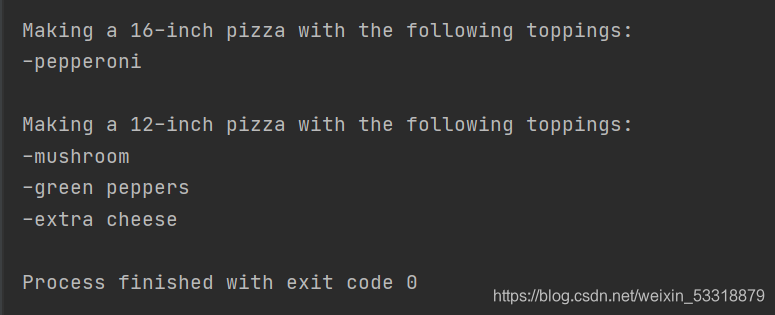
?
第九章? ? ? ? 类
#把类储存在模块中
class Dog:
def __init__(self,name,age):
self.name=name
self.age=age
def sit(self):
print(f"{self.name} is now sitting.")
def roll_over(self):
print(f"{self.name} rolled over!")
my_dog = Dog('Willie', 6)
your_dog=Dog('Lucy',3)
print(f"My dog's name is {my_dog.name}.")
print(f"My dog is {my_dog.age} years old.")
my_dog.sit()
my_dog.roll_over()
print(f"\nYour dog's name is {your_dog.name}.")
print(f"Your dog is {your_dog.age} years old.")
your_dog.sit()
your_dog.roll_over()
|