版本
flask版本:1.1.4
jinjia2版本:2.11.3
flask-script版本:2.0.6
flask-blueprint版本:1.3.0
flask-bootstrap版本:3.3.7.1
文档
flask英文文档:https://flask.palletsprojects.com/en/1.1.x/ flask中文文档:https://dormousehole.readthedocs.io/en/1.1.2/ jinja2英文文档:https://jinja.palletsprojects.com/en/3.0.x/ flask-bootstrap中文文档:https://flask-bootstrap-zh.readthedocs.io/zh/latest/ bootstrap3组件文档:https://v3.bootcss.com/components/
flask-script
a) 安装flask-script
pip install flask-script
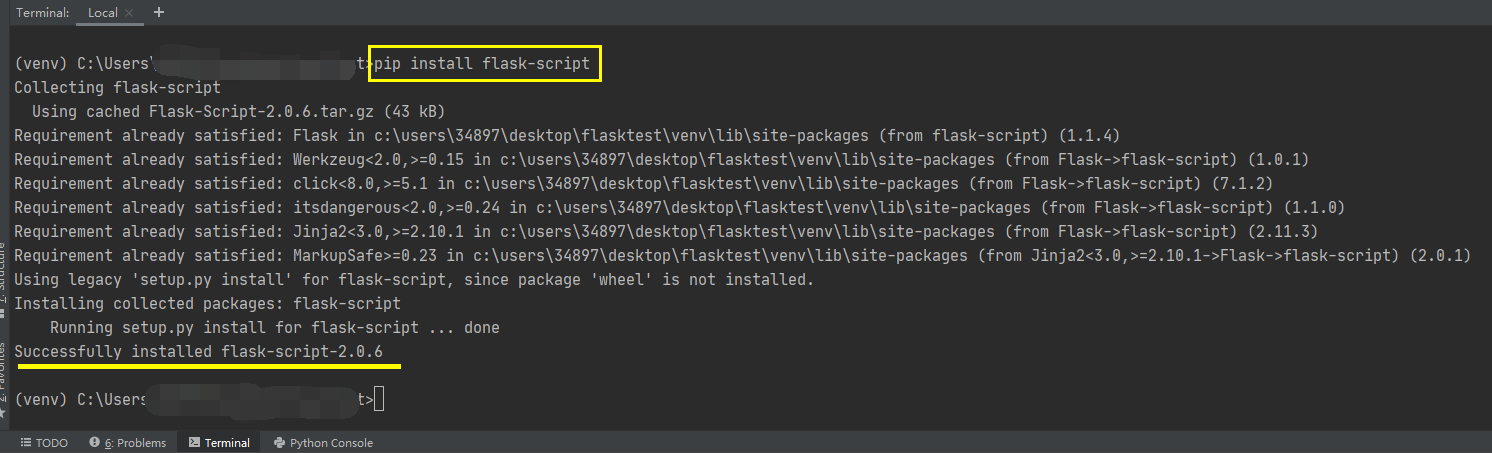
b) 修改app.py
from flask import Flask
from flask_script import Manager
app = Flask(__name__)
manager = Manager(app=app)
@app.route('/')
def hello_world():
return 'Hello World!'
if __name__ == '__main__':
manager.run()
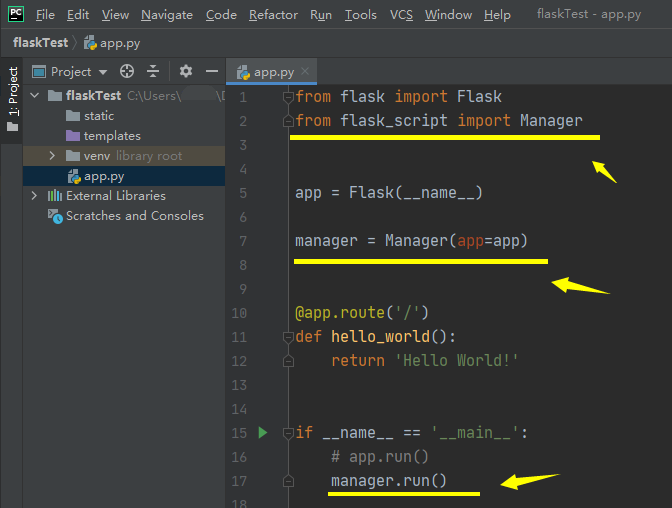
c) 运行
python app.py runserver -r -d
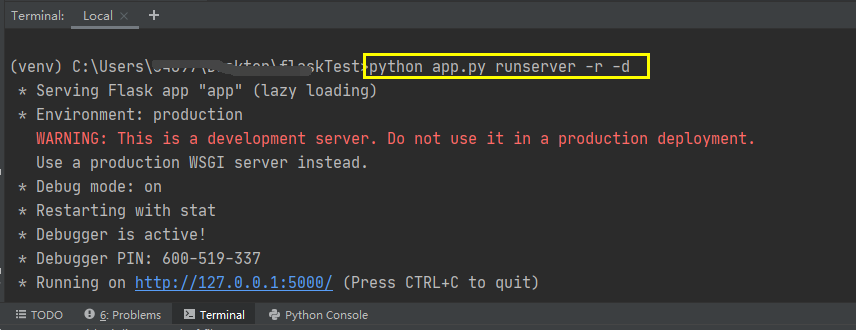
d) 浏览器访问
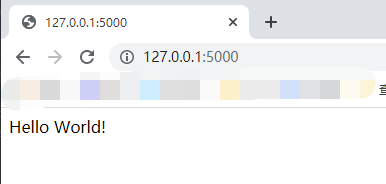
蓝图 flask-blueprint
a) 安装flask-blueprint
pip install flask-blueprint
b) 创建route.py
from flask import Blueprint
blue = Blueprint('blue', __name__)
@blue.route('/')
def hello_world():
return 'Hello World!'
@blue.route('/index/')
def index():
return 'Hello Index!'
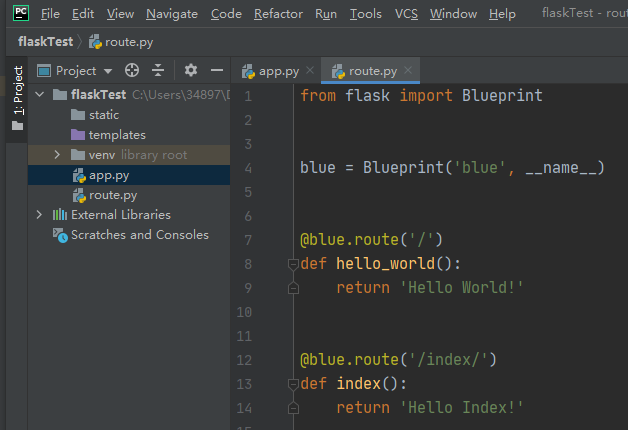
c) 修改app.py
from flask import Flask
from flask_script import Manager
from route import blue
app = Flask(__name__)
app.register_blueprint(blueprint=blue)
manager = Manager(app=app)
if __name__ == '__main__':
manager.run()
d) 访问
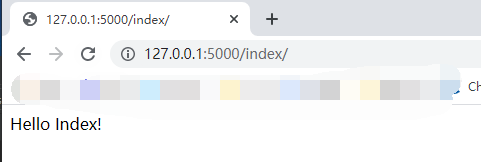
render_template
a1) 创建home.html
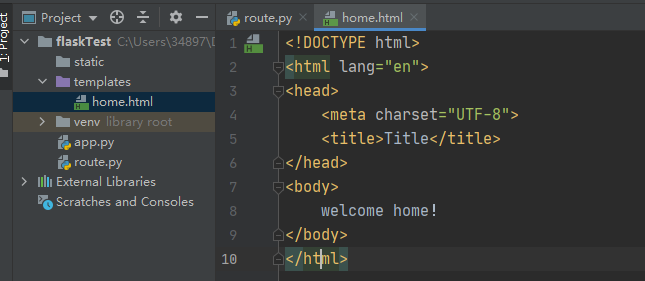
a2) 修改route.py
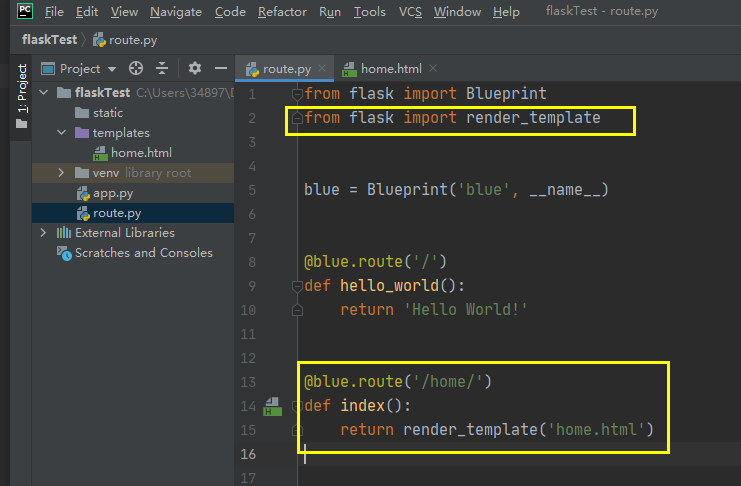
a3) 访问
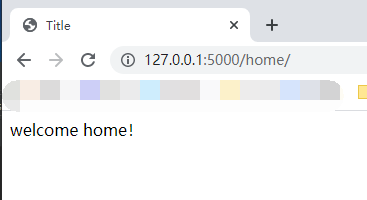
b1) 修改route.py,传递数据,如字符串等
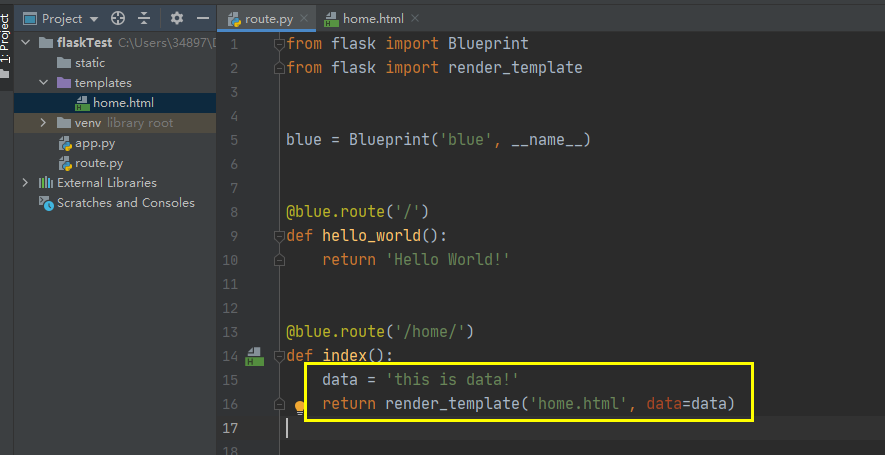
b2) home.html 中接收数据
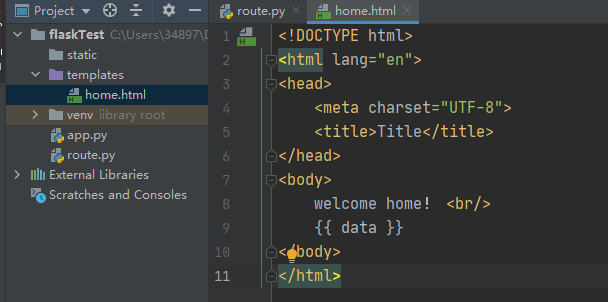
b3) 访问
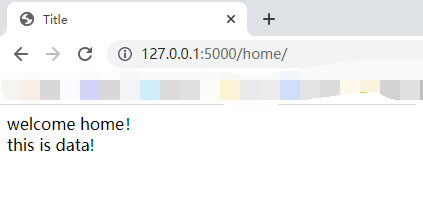
url_for、redirect
a) 修改route.py
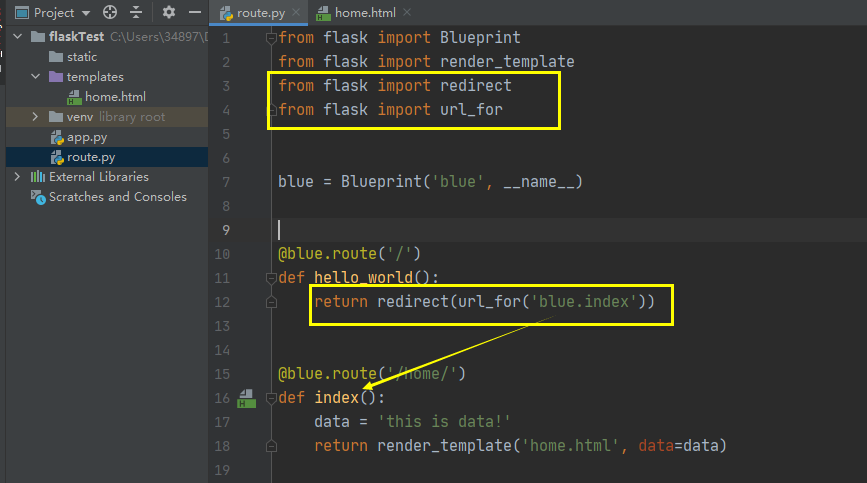
b) 访问
http://127.0.0.1:5000
跳转至:
http://127.0.0.1:5000/home/
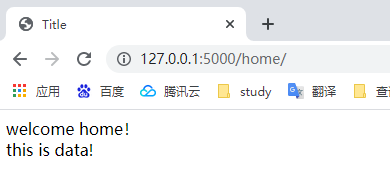
<a>标签跳转
从home跳转到 hello_world 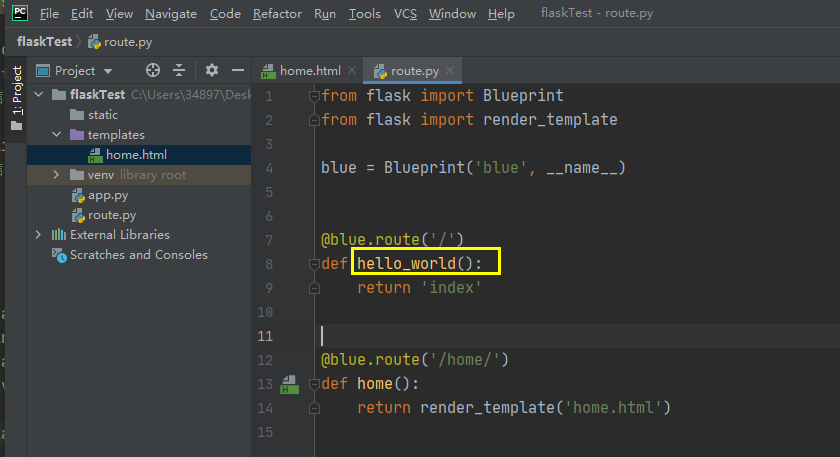 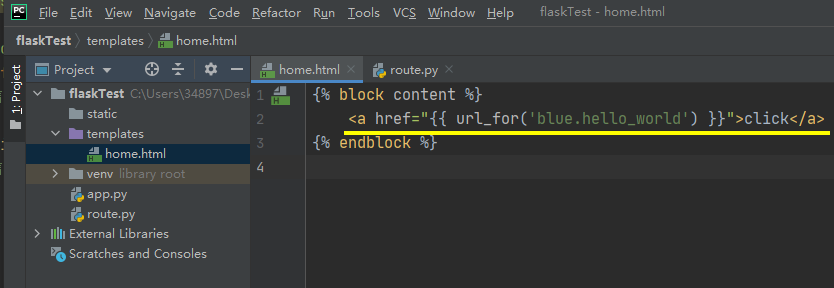
request
a) 添加/获取/删除Cookie
from flask import Blueprint
from flask import render_template
from flask import Response, request, redirect, url_for
blue = Blueprint('blue', __name__)
@blue.route('/')
def hello_world():
return 'index'
@blue.route('/setCookie/')
def setCookie():
rsp = Response('set cookie, 21')
rsp.set_cookie('myCookie', '21')
return rsp
@blue.route('/getCookie/')
def getCookie():
myCookie = request.cookies.get('myCookie')
return 'cookie is {}'.format(myCookie)
@blue.route('/deleteCookie/')
def deleteCookie():
rsp = redirect(url_for('blue.hello_world'))
rsp.delete_cookie('myCookie')
return rsp
b) 添加/获取/删除Session
app.py 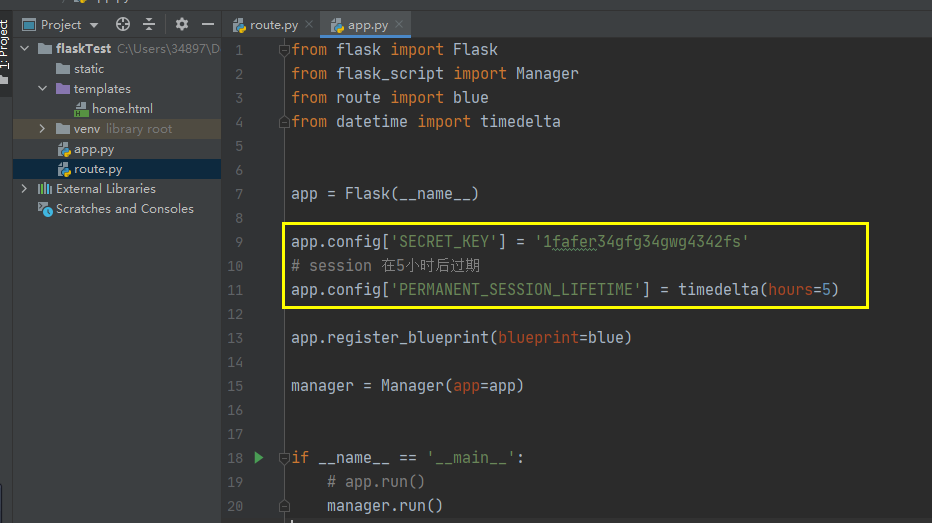 route.py 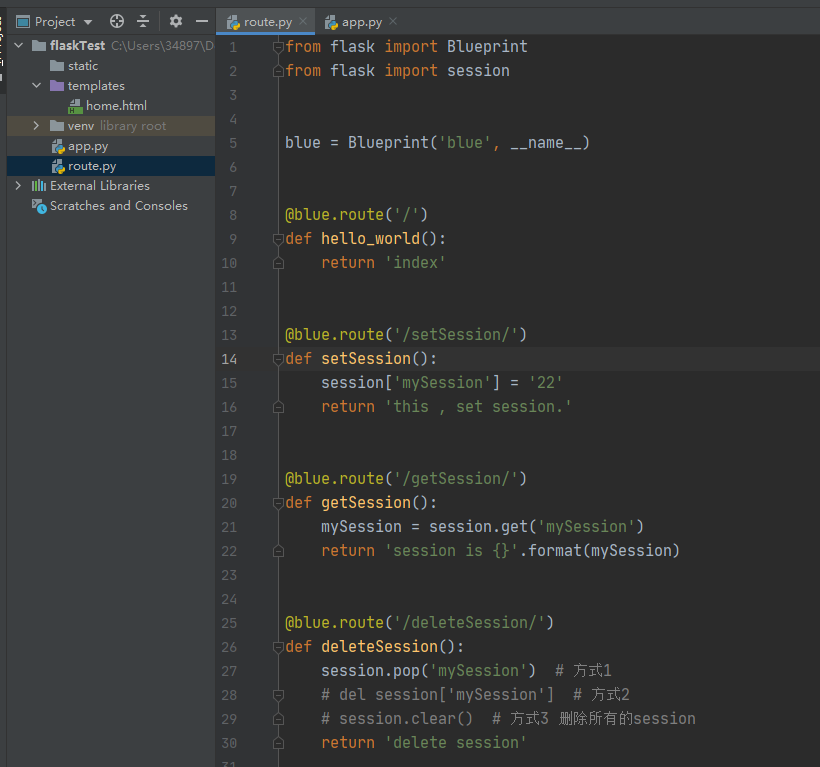
c) method (GET POST…) \ form表单提交
login.html 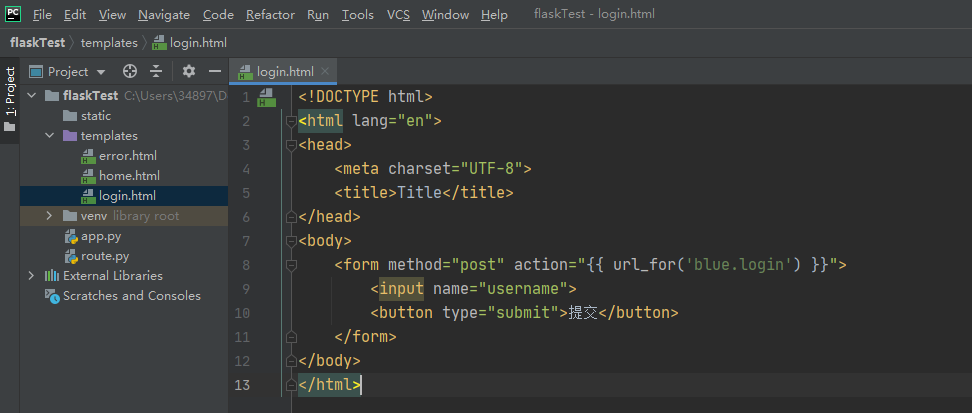 route.py 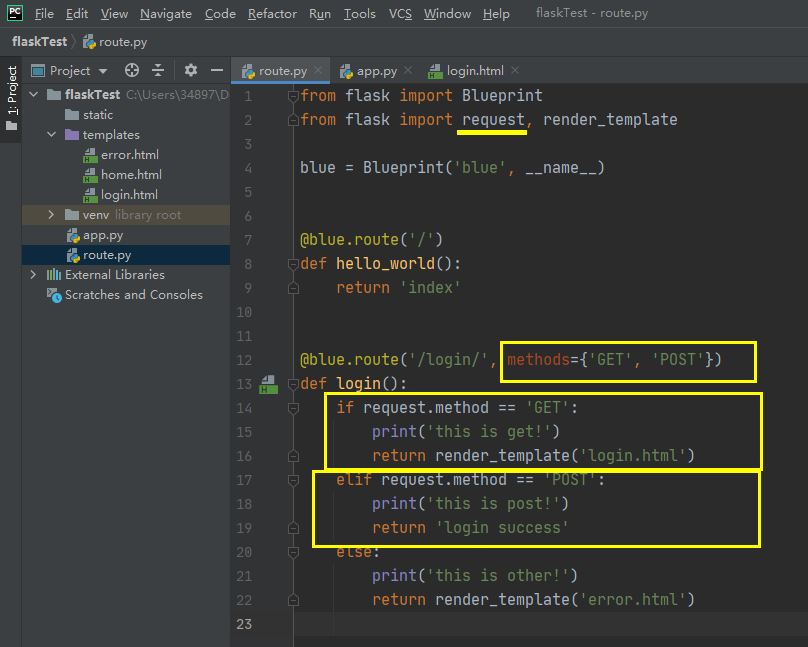
jinja2模板
{% %} 控制语句,如
{% if data == 'name' %}
{{ data }}
{% else %}
{{ data }}
{% endif %}
{{ }} 变量,如 {{ data }}
{
a) 传递字符串
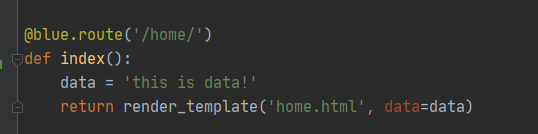 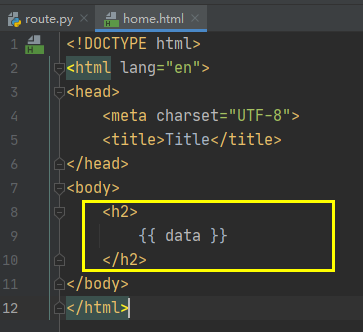
b) 传递列表 - for 循环
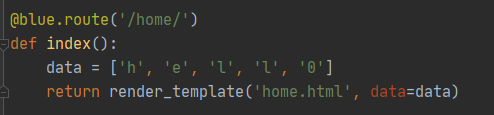
for 遍历
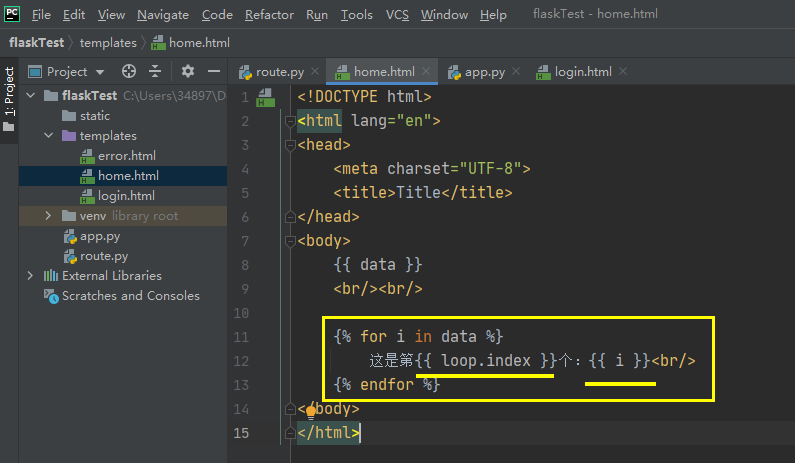
根据索引取值
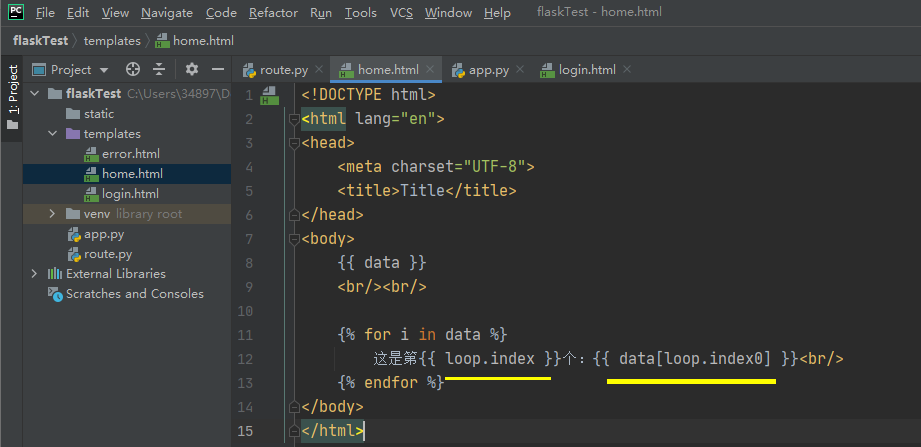
c) if 判断
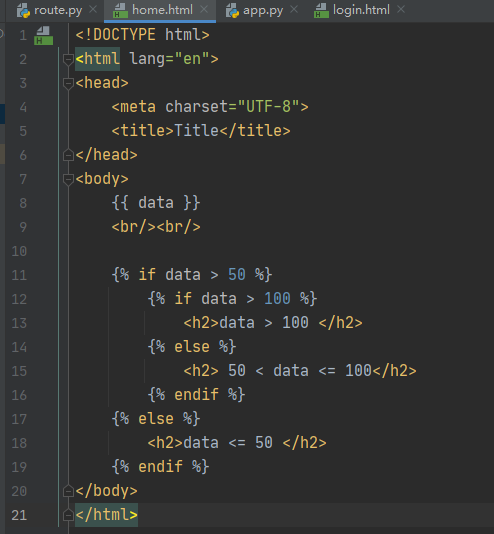
d) set 设置变量
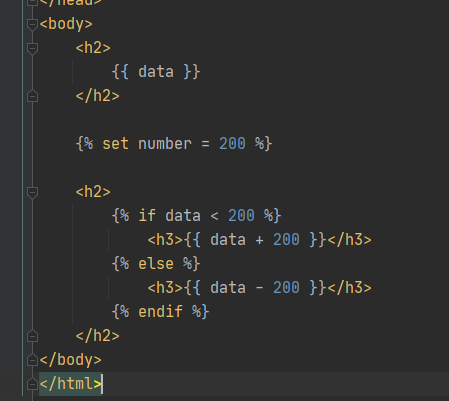
e) extends html引入html
base.html 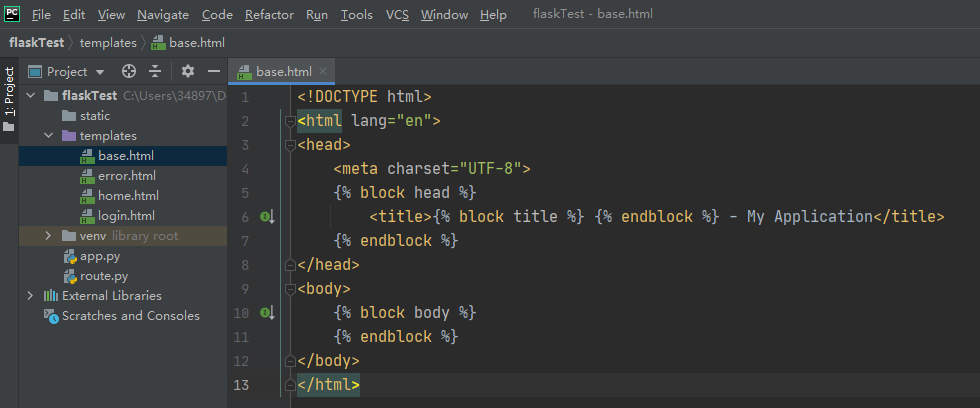 home.html 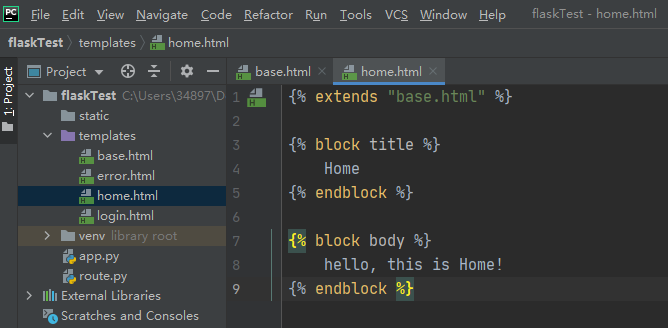
f) 宏 macro
定义一个宏 macro 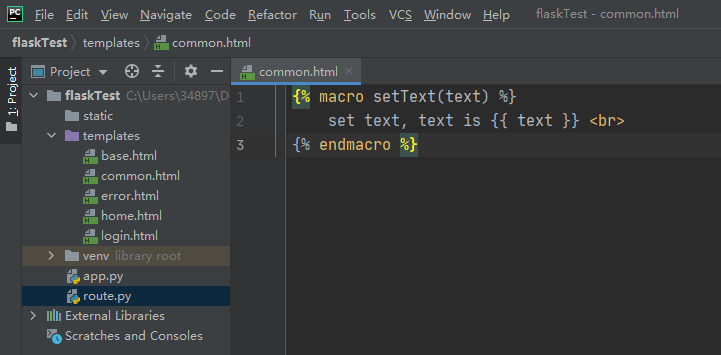 引入宏 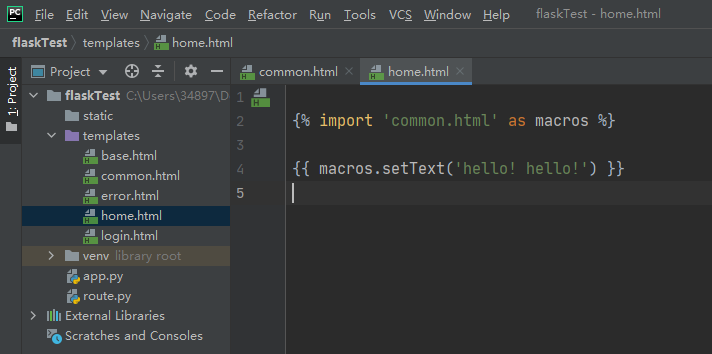
bootstrap
a) 安装flask-bootstrap
pip install flask-bootstrap
b) 引入
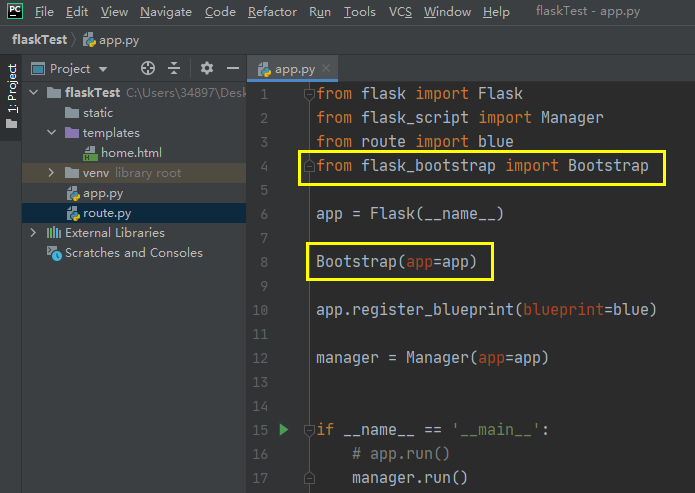
c) 使用bootstrap
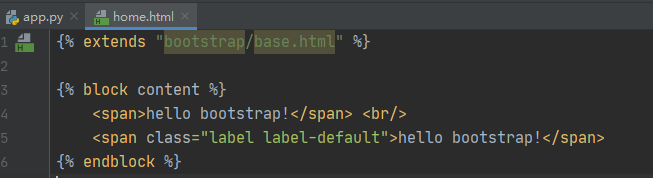
自定义css
创建home.css
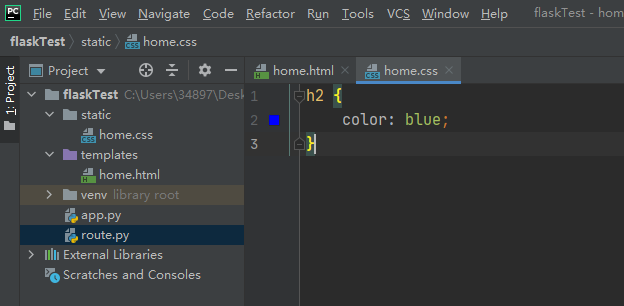
home.html引用css
{% extends "bootstrap/base.html" %}
{% block styles %}
{{ super() }}
<link rel="stylesheet" href="{{ url_for('static', filename='home.css') }}">
{% endblock %}
{% block content %}
<h2>hello</h2>
<h3>flask</h3>
{% endblock %}
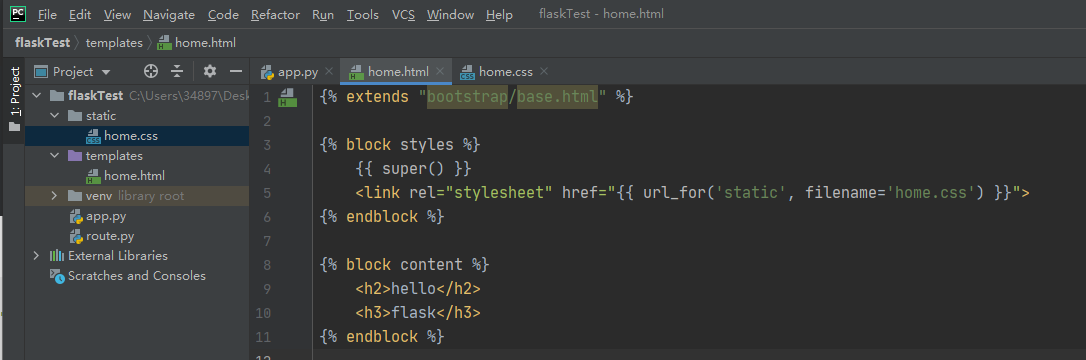
自定义JS
创建js
$("#test_id").click(function(){
alert('test!test!')
});
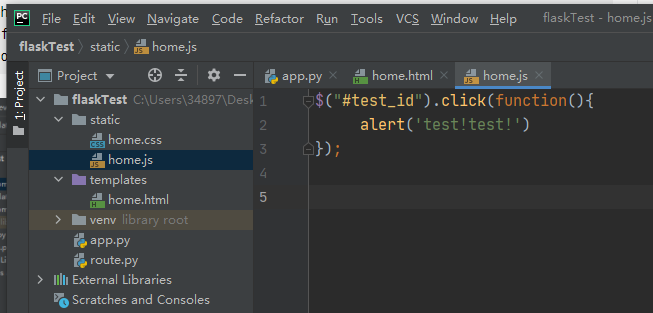
引入js
{% extends "bootstrap/base.html" %}
{% block scripts %}
{{super()}}
<script src="{{url_for('static', filename='home.js')}}"></script>
{% endblock %}
{% block content %}
<button id="test_id">click</button>
{% endblock %}
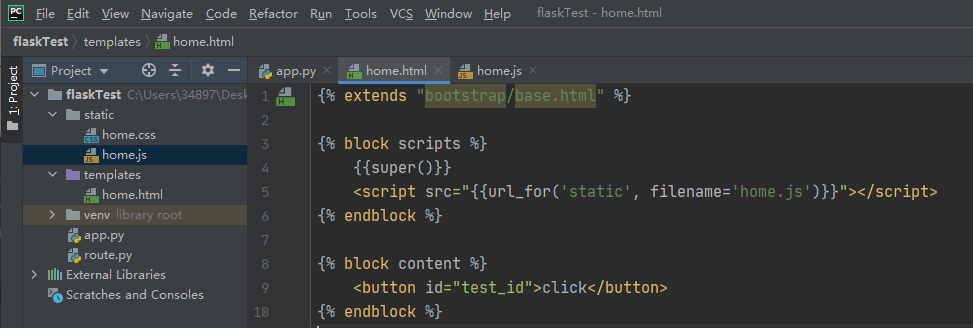
|