1、 CylinderExample
Description This example creates a minimal visualization program, demonstrating VTK’s basic rendering and pipeline creation.
CylinderExample.py
import vtk
def main():
colors = vtk.vtkNamedColors()
bkg = map(lambda x: x / 255.0, [26, 51, 102, 255])
colors.SetColor("BkgColor", *bkg)
cylinder = vtk.vtkCylinderSource()
cylinder.SetResolution(8)
cylinderMapper = vtk.vtkPolyDataMapper()
cylinderMapper.SetInputConnection(cylinder.GetOutputPort())
cylinderActor = vtk.vtkActor()
cylinderActor.SetMapper(cylinderMapper)
cylinderActor.GetProperty().SetColor(colors.GetColor3d("Tomato"))
cylinderActor.RotateX(30.0)
cylinderActor.RotateY(-45.0)
ren = vtk.vtkRenderer()
renWin = vtk.vtkRenderWindow()
renWin.AddRenderer(ren)
iren = vtk.vtkRenderWindowInteractor()
iren.SetRenderWindow(renWin)
ren.AddActor(cylinderActor)
ren.SetBackground(colors.GetColor3d("BkgColor"))
renWin.SetSize(300, 300)
renWin.SetWindowName('CylinderExample')
iren.Initialize()
ren.ResetCamera()
ren.GetActiveCamera().Zoom(1.5)
renWin.Render()
iren.Start()
if __name__ == '__main__':
main()
运行效果: 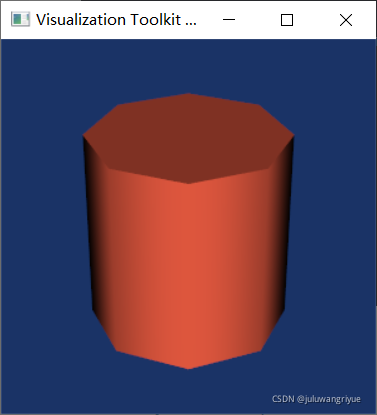
2、DistanceBetweenPoints
Description This example finds the squared distance and the Euclidean distance between two 3D points.
DistanceBetweenPoints.py
import math
import vtk
def main():
p0 = (0, 0, 0)
p1 = (1, 1, 1)
distSquared = vtk.vtkMath.Distance2BetweenPoints(p0, p1)
dist = math.sqrt(distSquared)
print('p0 = ', p0)
print('p1 = ', p1)
print('distance squared = ', distSquared)
print('distance = ', dist)
if __name__ == '__main__':
main()
运行结果:
D:\anaconda3\python.exe D:/documents/python/vtk/DistanceBetweenPoints.py
p0 = (0, 0, 0)
p1 = (1, 1, 1)
distance squared = 3.0
distance = 1.7320508075688772
Process finished with exit code 0
3、Input and Output
Graph Formats 3D File Formats Standard Formats
3.1 ReadExodusData
Description The example uses vtkExodusIIReader to read an ExodusII file. The nodal variable to read is the second argument. The nodal variable is displayed with a color map.
import vtk
def get_program_parameters():
import argparse
description = 'Read and display ExodusII data.'
epilogue = '''
'''
parser = argparse.ArgumentParser(description=description, epilog=epilogue)
parser.add_argument('filename', help='A required filename e.g mug.e.')
parser.add_argument('nodal_var', help='The nodal variable e,g, convected.')
args = parser.parse_args()
return args.filename, args.nodal_var
def main():
colors = vtk.vtkNamedColors()
filename, nodal_var = get_program_parameters()
reader = vtk.vtkExodusIIReader()
reader.SetFileName(filename)
reader.UpdateInformation()
reader.SetTimeStep(10)
reader.SetAllArrayStatus(vtk.vtkExodusIIReader.NODAL, 1)
reader.Update()
geometry = vtk.vtkCompositeDataGeometryFilter()
geometry.SetInputConnection(0, reader.GetOutputPort(0))
geometry.Update()
mapper = vtk.vtkPolyDataMapper()
mapper.SetInputConnection(geometry.GetOutputPort())
mapper.SelectColorArray(nodal_var)
mapper.SetScalarModeToUsePointFieldData()
mapper.InterpolateScalarsBeforeMappingOn()
actor = vtk.vtkActor()
actor.SetMapper(mapper)
renderer = vtk.vtkRenderer()
renderer.AddViewProp(actor)
renderer.SetBackground(colors.GetColor3d('DimGray'))
renderer.GetActiveCamera().SetPosition(9.0, 9.0, 7.0)
renderer.GetActiveCamera().SetFocalPoint(0, 0, 0)
renderer.GetActiveCamera().SetViewUp(0.2, -0.7, 0.7)
renderer.GetActiveCamera().SetDistance(14.5)
window = vtk.vtkRenderWindow()
window.AddRenderer(renderer)
window.SetSize(600, 600)
window.SetWindowName('ReadExodusData')
interactor = vtk.vtkRenderWindowInteractor()
interactor.SetRenderWindow(window)
interactor.Initialize()
window.Render()
interactor.Start()
if __name__ == '__main__':
main()
怎么执行还不懂,先记下来。
参考:Python Examples
|