import numpy as np
arr = np.array(range(10))
print(arr)
type_ = arr.dtype
print(type_)
arr = np.array([1, 2, '1', 300, 1.14], dtype=np.int8)
print(arr.dtype, '\t', arr)
myc = np.dtype('<i4')
print(myc)
myc = np.dtype([('id', 'i4'), ('name', '<U11')])
print(myc)
arr = np.array([(10000, 'snake'), (20000, 'roje'), (30000, 'monty_python')],
dtype=myc)
print(arr['id'], arr[0])
count = 0
temp = arr['id']
for id in temp:
if id == 30000:
temp[count] = 1024
break
count += 1
print(arr)
'''
type_ = [
('col0', 'type0'),
('col1', 'type1'),
... ...
]
data = [
(data0, data1, ... ...),
(data0, data1, ... ...),
... ...
]
# 此处的[]代表的是可省略项,伪代码的一种表达方式
array = numpy.array(data, [type_ | dtype=type | None])
'''
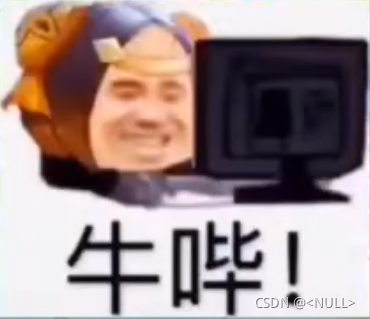
|