mongoDB增删改查
"""
@File : demo_1.py
@Time : 2021-09-06 15:12
@Author : XD
@Email : gudianpai@qq.com
@Software: PyCharm
"""
from pymongo import MongoClient
try:
client = MongoClient(host = "localhost", port = 27017)
client.admin.authenticate("myUserAdmin","abc123")
client.school.teacher.insert_one({"name":"李璐"})
client.school.teacher.insert_many([{"name":"李璐"},{"name":"陈刚"}])
teacher = client.school.teacher.find({})
for one in teacher:
print(one["_id"], one["name"])
print("-"*50)
teacher = client.school.teacher.find_one({"name":"李璐"})
print(teacher["_id"], teacher["name"])
except Exception as e:
print(e)
6135c961a775025ae4e741a8 李璐
6135c998a7750221283b703b 李璐
6135c998a7750221283b703c 李璐
6135c998a7750221283b703d 陈刚
6135c9efa775020e4898565e 李璐
6135c9efa775020e4898565f 李璐
6135c9efa775020e48985660 陈刚
6135ca00a7750237bc55221b 李璐
6135ca00a7750237bc55221c 李璐
6135ca00a7750237bc55221d 陈刚
6135ca43a7750235381ac77e 李璐
6135ca43a7750235381ac77f 李璐
6135ca43a7750235381ac780 陈刚
6135ca52a775023014df0554 李璐
6135ca52a775023014df0555 李璐
6135ca52a775023014df0556 陈刚
6135cd51a775024f806fff40 李璐
6135cd51a775024f806fff41 李璐
6135cd51a775024f806fff42 陈刚
6135cd70a7750220447fdfcb 李璐
6135cd70a7750220447fdfcc 李璐
6135cd70a7750220447fdfcd 陈刚
6135cd7fa775024dc80000d8 李璐
6135cd7fa775024dc80000d9 李璐
6135cd7fa775024dc80000da 陈刚
613612c5a775022f8cf757d1 李璐
613612c5a775022f8cf757d2 李璐
613612c5a775022f8cf757d3 陈刚
--------------------------------------------------
6135c961a775025ae4e741a8 李璐
from pymongo import MongoClient
try:
client = MongoClient(host="localhost", port=27017)
client.admin.authenticate("myUserAdmin", "abc123")
client.school.teacher.update_many({},{"$set":{"role":["班主任"]}})
client.school.teacher.update_one({"name":"李璐"},{"$set":{"sex":"女"}})
client.school.teacher.update_one({"name":"李璐"}, {"$push": {"role": "年级主任"}})
except Exception as e:
print(e)
数据删除
from mongo_db import client
try:
client.school.teacher.delete_one({"name":"李璐"})
client.school.teacher.delete_many({})
except Exception as e:
print(e)
分页,排序。
from mongo_db import client
student = client.school.teacher.find({}).skip(0).limit(10)
for one in student:
print(one["_id"], one["name"])
names = client.school.teacher.distinct("name")
for one in names:
print(one)
teachers = client.school.teacher.find({}).sort([("name", -1)])
for one in teachers:
print(one["_id"], one["name"])
pymongoDB模块向MongoDB中保存文件
"""
@File : demo_5.py
@Time : 2021-09-07 14:50
@Author : XD
@Email : gudianpai@qq.com
@Software: PyCharm
"""
from mongo_db import client
from gridfs import GridFS
db = client.school
gfs = GridFS(db, collection = "book")
file = open("./Attention-based_Saliency_Hashing_for_Ophthalmic_Im.pdf", "rb")
args = {"type":"PDF", "keyword":"hash"}
gfs.put(file, filename = "Attention-based_Saliency_Hashing_for_Ophthalmic_Im.pdf", **args)
file.close()
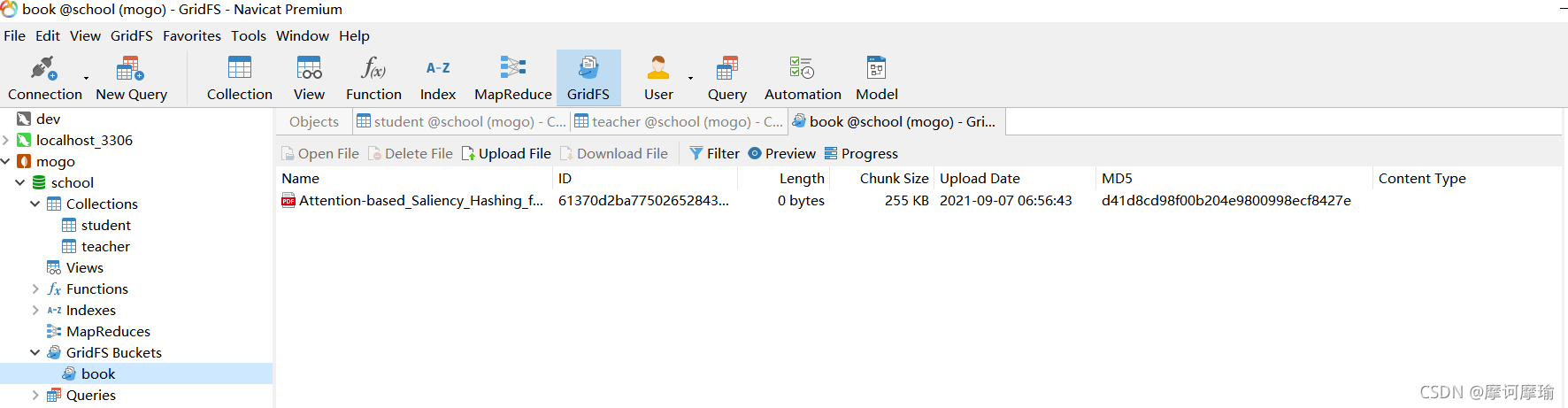 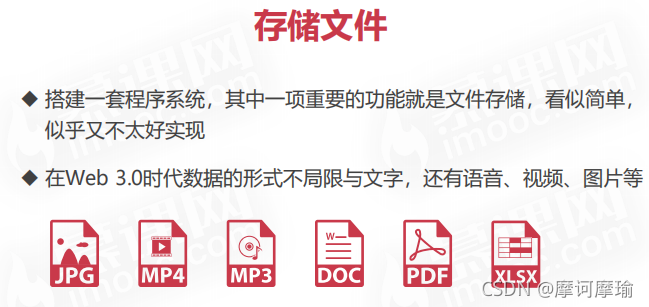 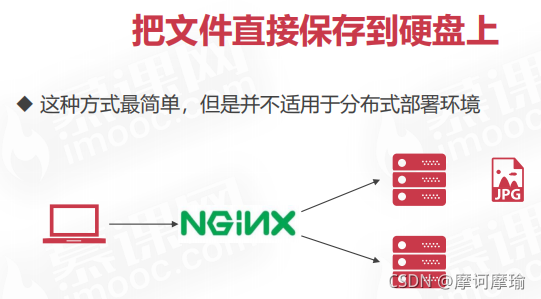 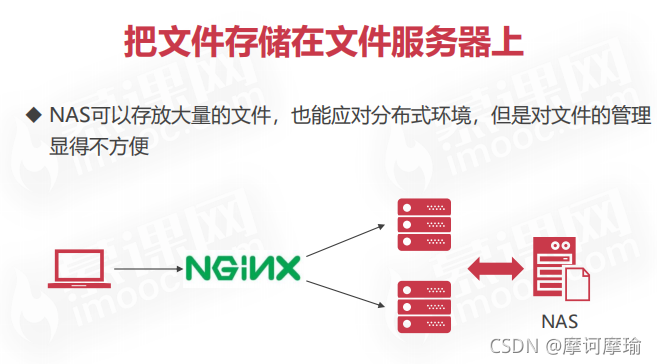 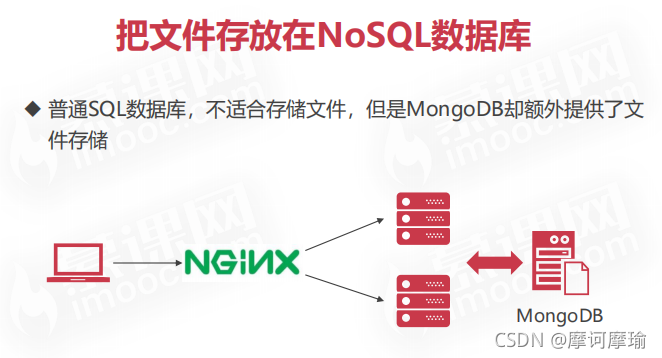 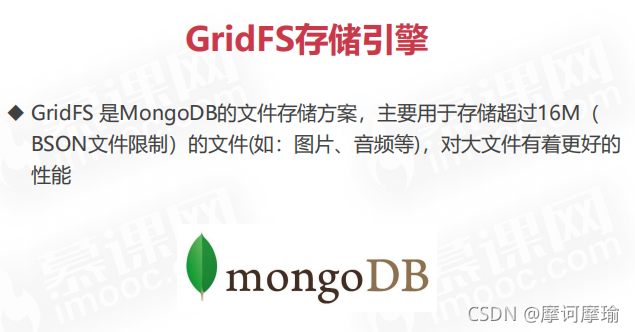 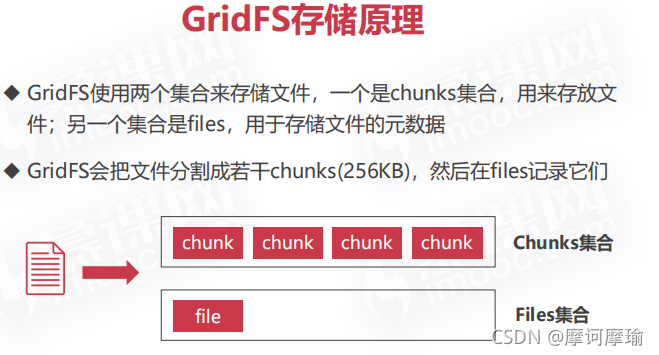 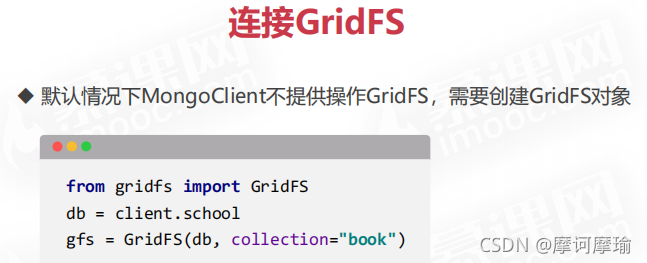 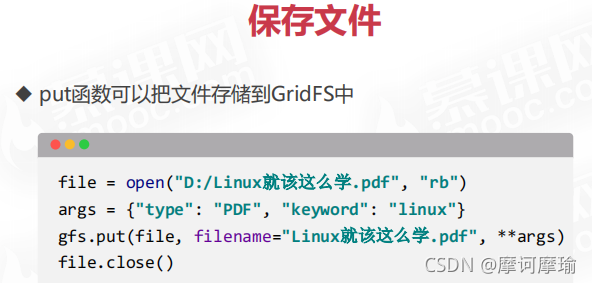
查询文件
from mongo_db import client
from gridfs import GridFS
import math
import datetime
db = client.school
gfs = GridFS(db, collection = "book")
book = gfs.find_one({"filename":"Attention-based_Saliency_Hashing_for_Ophthalmic_Im.pdf"})
print(book.filename)
print(book.type)
print(book.keyword)
print("{}".format(math.ceil(book.length/1024/1024)))
print("-"*100)
books = gfs.find({"type":"PDF"})
for one in books:
uploadDate = one.uploadDate + datetime.timedelta(hours = 8)
uploadDate = uploadDate.strftime("%Y-%m-%d %H:%M:%S")
print(one._id, one.filename, one.type, uploadDate)
print("-"*100)
from bson.objectid import ObjectId
rs = gfs.exists(ObjectId("61371755a775026d74e76855"))
print(rs)
rs = gfs.exists(**{"type":"PDF"})
print(rs)
Attention-based_Saliency_Hashing_for_Ophthalmic_Im.pdf
PDF
hash
3
----------------------------------------------------------------------------------------------------
61371755a775026d74e76855 Attention-based_Saliency_Hashing_for_Ophthalmic_Im.pdf PDF 2021-09-07 15:40:05
----------------------------------------------------------------------------------------------------
True
True
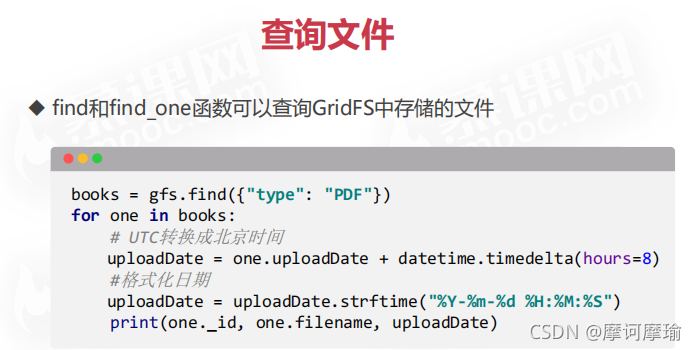 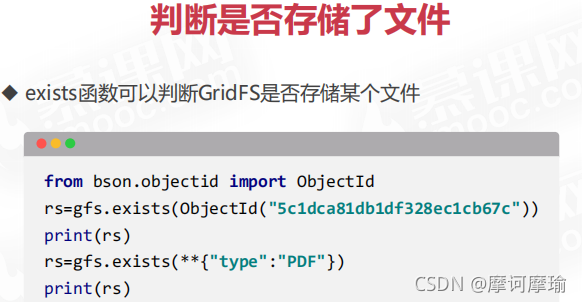 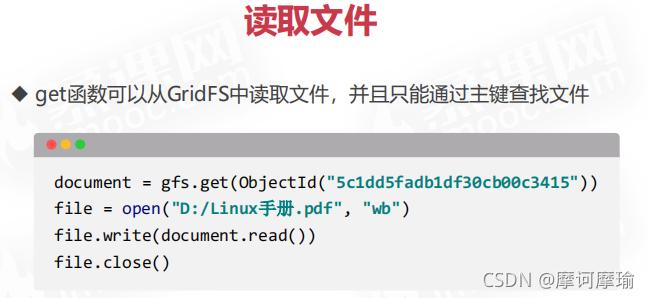 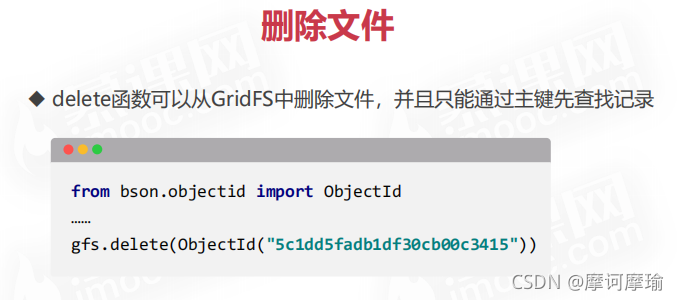 读取文件
from mongo_db import client
from gridfs import GridFS
from bson.objectid import ObjectId
db = client.school
gfs = GridFS(db, collection = "book")
document = gfs.get(ObjectId("61371755a775026d74e76855"))
file = open("./hash.pdf", "wb")
file.write(document.read())
file.close()
删除文件
from mongo_db import client
from gridfs import GridFS
from bson.objectid import ObjectId
db = client.school
gfs = GridFS(db, collection = "book")
gfs.delete(ObjectId("61376d23a775025ce8d0ec3a"))
|