数据结构
Series对象 创建一维数组的对象
s1 = pandas.Series([1, 2, 3, 4])
s2 = pandas.Series([1,2,3],index=['a','b','c'])
s3 =pandas.Series({'a':1,'b':2})
返回值
a 1
b 2
c 3
dtype: int64
DataFrame对象 二维数组对象
s1 = pandas.DataFrame([['student1',12,89],
['student2',21,90]],
index=['001','002'],
columns=['name','age','score'])
返回值
name age score
001 student1 12 89
002 student2 21 0
读取数据 ——Excel表格
使用read_excel
excel = pandas.read_excel('工作簿1.xlsx',
sheet_name=0,
engine='xlrd')
默认引擎是xlrd,使用时需要安装xlrd,此外还需要安装openpyxl模块。
sheet_name=0,表示读取第一个工作表。也可使用具体表名。
指定读取数据的行、列标签
使用参数header和index_col
赋值为0时:自带的列标签没有了
none:没有改变
赋值为1:产品、金额表头没有了
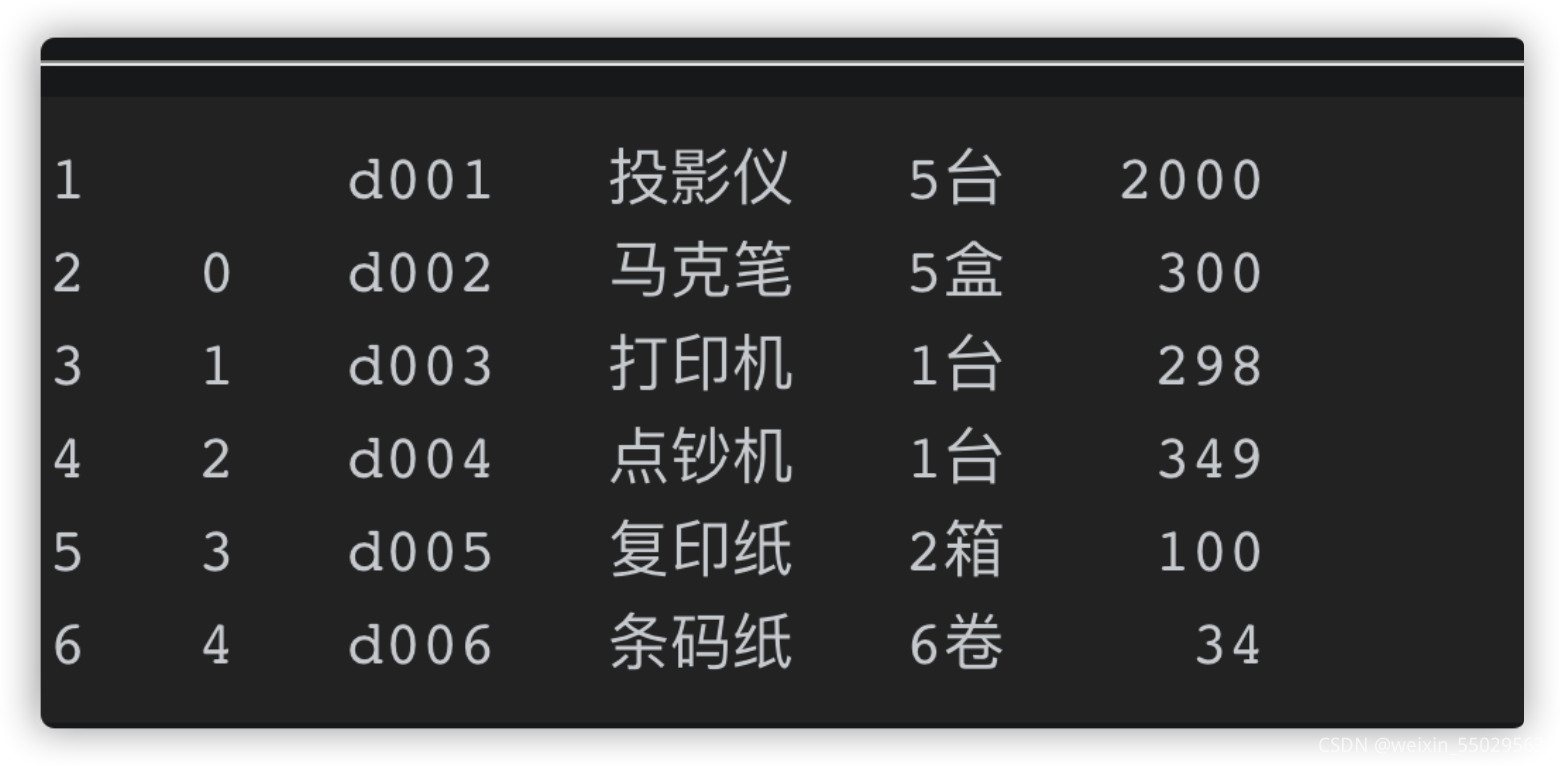
赋值为2以此类推
使用index_col解决行标签同理
读取指定列
usecols=[2,3] 只读取第3,4列
读取前几行
使用head方法,默认是前5行
excel = pandas.read_excel('工作簿1.xlsx')
print(excel.head(3))
print(excel.shape)
查看数据总行数、列数——shape方法
print(excel.shape) #(9,6)
转换数据类型——astype()函数
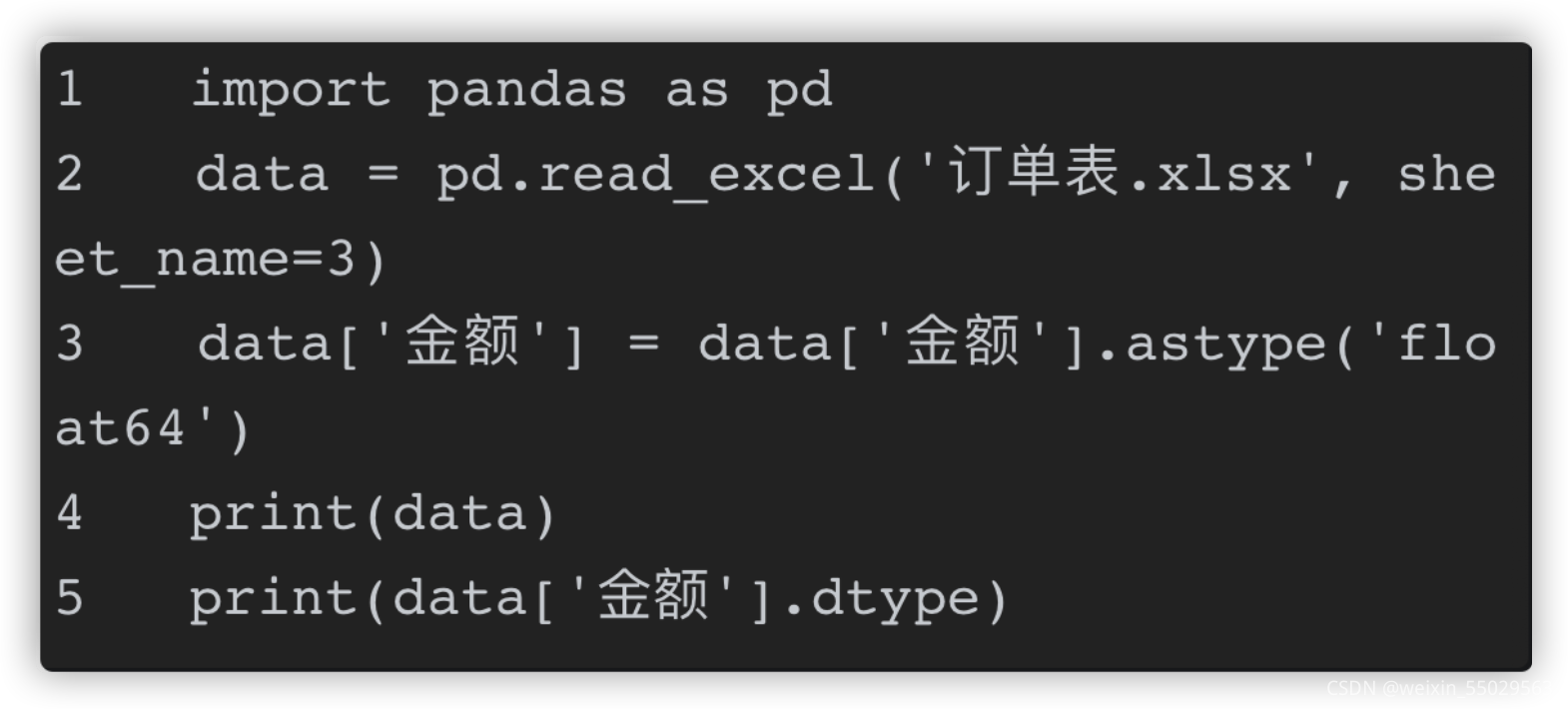
选择单行、多行标签——loc和iloc
loc通过行标签选择
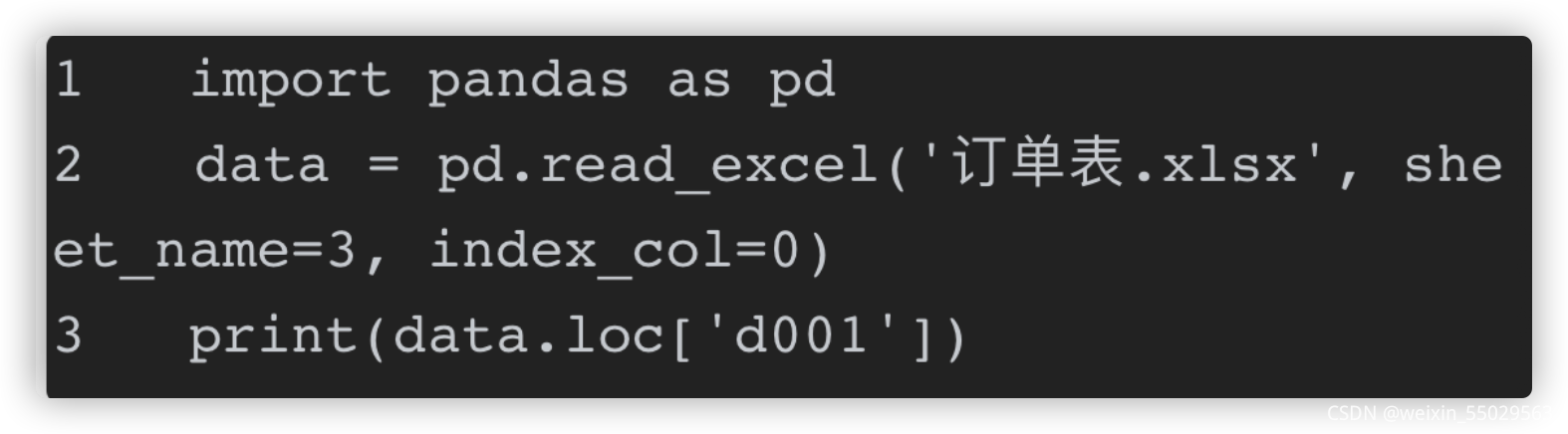
data.loc[['d001','d002']]
iloc通过索引来选择
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-az7C1Vgg-1631366239931)(/Users/wuyang/Library/Application Support/typora-user-images/image-20210823102207198.png)]](https://img-blog.csdnimg.cn/645cd80599874a61b14db7d67999edb1.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBAd2VpeGluXzU1MDI5NTYz,size_20,color_FFFFFF,t_70,g_se,x_16)
data.iloc[['d001','d002']]
data.iloc[1:2]
选择满足条件行
![[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-jVSDYvNl-1631366239935)(/Users/wuyang/Library/Application Support/typora-user-images/image-20210823102349392.png)]](https://img-blog.csdnimg.cn/ee42dec284584dc88407b54c22993e31.png?x-oss-process=image/watermark,type_ZHJvaWRzYW5zZmFsbGJhY2s,shadow_50,text_Q1NETiBAd2VpeGluXzU1MDI5NTYz,size_20,color_FFFFFF,t_70,g_se,x_16)
处理重复数据
planets = pd.read_csv('planets.csv')
print(planets.head(10))
planets.drop_duplicates(subset=['method','year'],keep='first',inplace=True)
print(planets.head(10))
planets.drop_duplicates(subset=[‘method’,‘year’],keep=‘first’,inplace=True)这个是最关键的语句了。 首先subset参数是一个列表,这个列表是需要你填进行相同数据判断的条件。就比如我选的条件是method和year,即 method值和year的值相同就可被判定为一样的数据。keep的取值有三个 分别是 first、last、false keep=first时,保留相同数据的第一条。keep=last时,保存相同数据的最后一条。keep=false时,所有相同的数据都不保留。inplace=True时,会对原数据进行修改。否则,只返回视图,不对原数据修改。
追加文件内容
def append_excel(df, content_list):
"""
excel文件中追加内容
:return:
df:已有表格中数据
content_list:待追加的内容列表
"""
ds = pandas.DataFrame(content_list)
df = df.append(ds, ignore_index=True)
df.to_excel('c.xlsx', index=False, header=False)
append_excel(pandas.read_excel('c.xlsx', header=None), data)
|