一. 要求
编写python程序实现批量发送邮件(含附件)
二. 步骤
1. 开启QQ邮箱SMTP服务
① 登录QQ邮箱,点击“设置”: 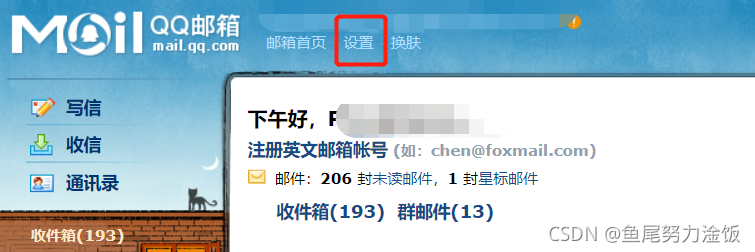 ② 点击“账户”: 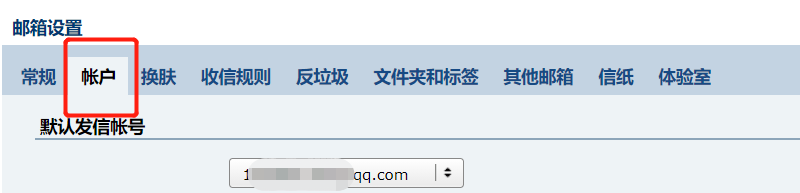 ③ 开启SMTP服务(可全部开启): 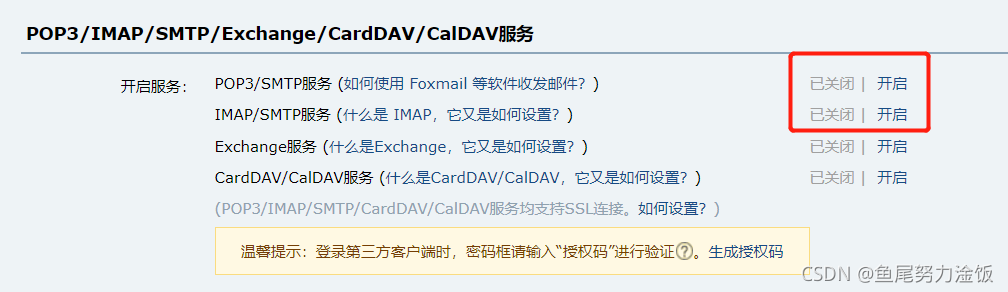 ④ 获取授权码: 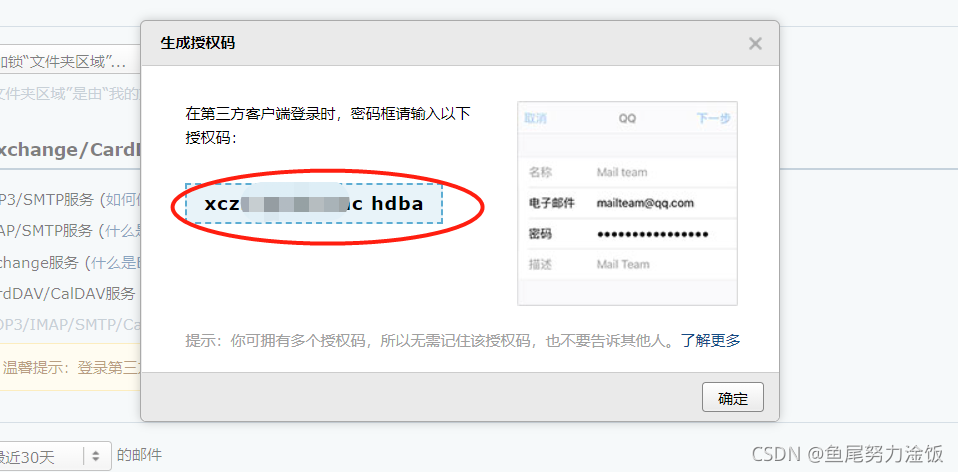
2. 需要发送的邮件
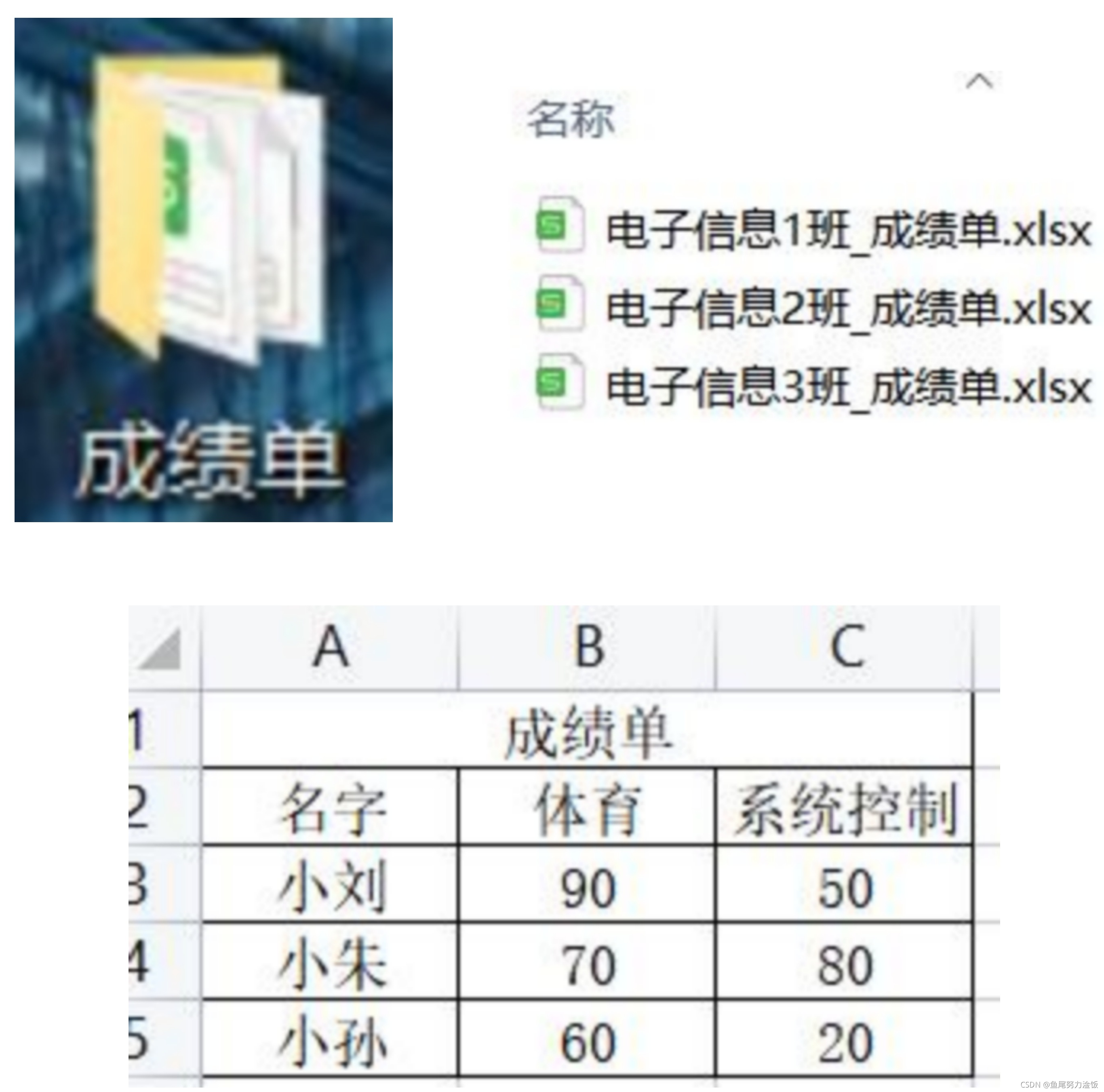
3. 代码分析
三个班级的收件邮箱被存储在名为“邮件发送地址”的一个excel文件中: 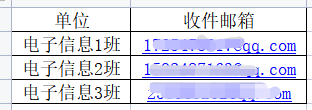 使用load_workbook 打开这个文件夹,并以各班单位为键,收件邮箱为值保存于名为address的字典中:
from openpyxl import load_workbook
wb = load_workbook("邮件发送地址.xlsx")
ws = wb.active
address = {}
for i in range(2,ws.max_row+1):
name = ws["A"+ str(i)].value
to_addr = ws["B"+ str(i)].value
address[name] = [to_addr]
单独打印下address看看效果(邮箱地址做了打码处理):
>>> print(address)
{'电子信息1班': ['1*********7@qq.com'], '电子信息2班': ['1********3@qq.com'], '电子信息3班': ['2********2@qq.com']}
利用pandas库读取文件则不方便进行以上操作,load_workbook 的一些用法可以参考文章:load_workbook 基本用法
import os
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
from email.mime.base import MIMEBase
from email import encoders
from email.header import Header
def Send_mail(to_add, file_path, name):
from_addr = '1592487163@qq.com'
password = 'iieepjqcvsvdbabf'
smtp_server = 'smtp.qq.com'
msg = MIMEMultipart()
msg['From'] = Header(from_addr)
msg['To'] = Header(to_addr)
msg['Subject'] = '成绩单'
msg.attach(MIMEText('你好,\n这是{}的成绩单,请查收!谢谢。\n\n学习部 小蒋'.format(name),'plain','utf-8'))
att = MIMEText(open(os.listdir('成绩单')[0],'rb').read(),'base64','utf-8')
att['Content-Type'] = 'application/octet-stream'
att.add_header('Content-Disposition', 'attachment', filename = '{}成绩单.xlsx'.format(name))
msg.attach(att)
server = smtplib.SMTP_SSL(smtp_server, 465)
server.login(from_addr, password)
server.sendmail(from_addr, to_addr, msg.as_string())
server.quit()
进行批量发送邮件:
for name in address.keys():
to_addr = address[i][0]
os.listdir('成绩单')
file_path = os.getcwd() + '\\成绩单\\{}_成绩单.xlsx'.format(name)
Send_mail(to_addr, file_path)
print("邮件发送完成!")
4. 参考资料
- https://blog.csdn.net/LeoPhilo/article/details/89074232
- https://www.runoob.com/python/python-email.html
- https://zhuanlan.zhihu.com/p/24180606
- https://blog.csdn.net/pptde/article/details/111573494
- https://www.liaoxuefeng.com/discuss/969955749132672/1177449138455872
- https://www.cnblogs.com/zhangxinqi/p/9113859.html#_label2
- https://www.jb51.net/article/65172.htm
- https://blog.csdn.net/zin521/article/details/102582930
|