https://docs.djangoproject.com/en/3.2/intro/tutorial01/ 3.2版本
创建项目
$ django-admin startproject mysite
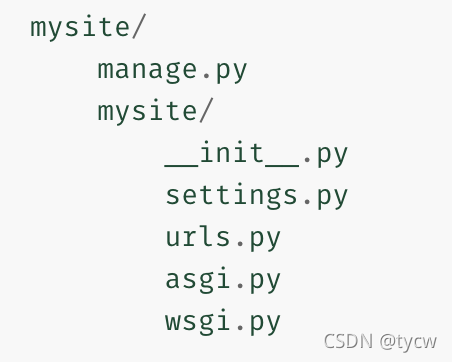
manage.py: 框架提供的命令行启动工具文件,接受参数实现不同的启动配置 mysite/asgi.py和mysite/wsgi.py: 这里需要理解网关的概念 这篇博客 解释的非常清楚 可以理解为web 服务器和 web 应用之间的中间层。 为什么需要中间层 python manage.py runserver,就可以接收请求了? 运行应用程序一般只为了调试,流量大时不能满足需要。
创建app polls
在manage.py 同级目录下运行 python manage.py startapp polls 创建polls文件夹 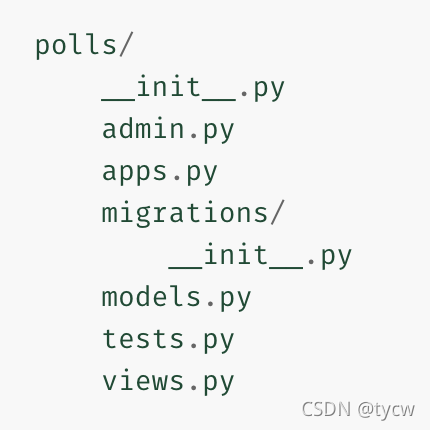
helloworld
from django.http import HttpResponse
def index(request):
return HttpResponse("Hello, world. You're at the polls index.")
from django.contrib import admin
from django.urls import include, path
urlpatterns = [
path('polls/', include('polls.urls')),
path('admin/', admin.site.urls),
]
此时访问http://localhost:8000/polls/ 得到helloworld
配置数据库
在mysite/settings.py下修改默认数据库配置,这篇笔记用MySQL
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'pylearn',
'USER': 'root',
'PASSWORD': '',
'HOST': '127.0.0.1',
'PORT': '3306',
}
}
import pymysql
pymysql.install_as_MySQLdb()
配置好之后运行 python manage.py migrate 然后去查看数据库 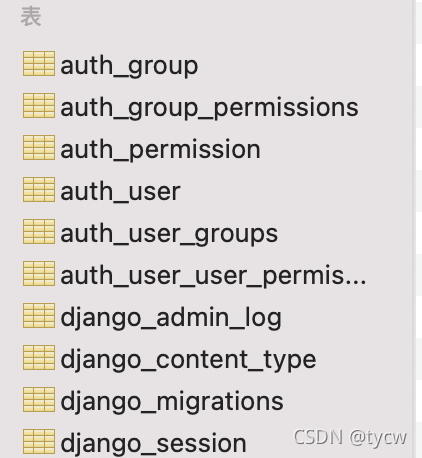
创建model
from django.db import models
class Question(models.Model):
question_text = models.CharField(max_length=200)
pub_date = models.DateTimeField('date published')
class Choice(models.Model):
question = models.ForeignKey(Question, on_delete=models.CASCADE)
choice_text = models.CharField(max_length=200)
votes = models.IntegerField(default=0)
Django的项目和app是包含关系 且app是 pluggable 的 现在我们需要在项目中安装polls这个app 在 mysite/settings.py 下将polls添加
INSTALLED_APPS = [
'polls.apps.PollsConfig',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
]
运行 python manage.py makemigrations polls makemigrations 命令告诉Django model 发生了变化 生成 polls/migrations/0001_initial.py 这个文件 一般不感知其中内容
python manage.py sqlmigrate polls 0001 生成创建 polls 的 model 的数据库表的SQL语句,以便提交dba或者运维建表
python manage.py migrate 根据上面的SQL语句建表
配置admin
python manage.py createsuperuser 访问 http://127.0.0.1:8000/admin/ 登录进去之后发现没有polls的管理选项
需要在polls/admin.py 中注册polls的model
from django.contrib import admin
from .models import Question
admin.site.register(Question)
在admin页面就可以管理Question model了
写view
def detail(request, question_id):
return HttpResponse("You're looking at question %s." % question_id)
def results(request, question_id):
response = "You're looking at the results of question %s."
return HttpResponse(response % question_id)
def vote(request, question_id):
return HttpResponse("You're voting on question %s." % question_id)
from django.urls import path
from . import views
urlpatterns = [
path('', views.index, name='index'),
path('<int:question_id>/', views.detail, name='detail'),
path('<int:question_id>/results/', views.results, name='results'),
path('<int:question_id>/vote/', views.vote, name='vote'),
]
配置好后,访问URL解析过程如下 When somebody requests a page from your website – say, “/polls/34/”, Django will load the mysite.urls Python module because it’s pointed to by the ROOT_URLCONF setting. It finds the variable named urlpatterns and traverses the patterns in order. After finding the match at ‘polls/’, it strips off the matching text (“polls/”) and sends the remaining text – “34/” – to the ‘polls.urls’ URLconf for further processing. There it matches ‘int:question_id/’, resulting in a call to the detail() view like so: detail(request=<HttpRequest object>, question_id=34) The question_id=34 part comes from int:question_id. Using angle brackets “captures” part of the URL and sends it as a keyword argument to the view function. The question_id part of the string defines the name that will be used to identify the matched pattern, and the int part is a converter that determines what patterns should match this part of the URL path. The colon ( : ) separates the converter and pattern name.
view返回httpResponse
view里返回template template的编写 即后端渲染html
新建 polls/templates/polls/index.html
{% if latest_question_list %}
<ul>
{% for question in latest_question_list %}
<li><a href="/polls/{{ question.id }}/">{{ question.question_text }}</a></li>
{% endfor %}
</ul>
{% else %}
<p>No polls are available.</p>
{% endif %}
404和其他业务页面的模板略过 见 Templates Guide 官方文档
以上是tutorial03及之前的主要内容 后面的内容是画view和自动化测试以及定制UI 画view这事和主流的前后端分离完全背离 笔记到此为止
|