Wz的Faster R-CNN代码
一、语法
- for……in……
格式:for x in y: 循环体 执行流程:x依次表示y中的一个元素,遍历完所有元素循环结束 如:
s = 'I love you more than i can say'
for i in s:
print(i)
- enumerate() :函数用于将一个可遍历的数据对象(如列表、元组或字符串)组合为一个索引序列,同时列出数据和数据下标,一般用在 for 循环当中
- try……except……
直接看这个吧https://www.runoob.com/python/python-exceptions.html - with……as……
常用于文件打开读写中,为了防止文件打开出错。 看这个:https://blog.csdn.net/qiqicos/article/details/79200089
二、包
- os(import os)
os.path.exists():判断路径是否存在,存在返回true,不存在返回false。 os.listdir(path):返回path所在路径的所有文件名 如:
print(os.listdir("./"))
得到输出(注意它的格式):
['.idea', 'main.py']
os.path.join():将路径名拼接在一起 如:
s = os.path.join("./", "dataset", "flower")
print(s)
得到:
./dataset\flower
- random(import random)
random.seed(0):设置随机数种子,每次生成相同的随机数,保证结果可复现 random.sample():sample(list, k)返回一个长度为k新列表,新列表存放list所产生k个随机唯一的元素。 如:
f = random.sample(range(0, 30), k=10)
print("采样数据为:" + str(f))
print("采样长度为:" + str(len(f)))
结果:
采样数据为:[2, 20, 9, 27, 29, 4, 0, 14, 24, 16]
采样长度为:10
三、函数
- sorted():
格式:sorted(iterable, cmp=None, key=None, reverse=False) 默认为升序排序,reverse=True时为降序。 - xx.split(“”):将字符串xx(注意xx是个字符串类型哈)中内容按“”中内容拆分开,返回一个字符串列表。
如:
f = os.listdir("./")
for file in f:
print(file.split("."))
得到结果:
['', 'idea']
['main', 'py']
- len():返回对象中项目数量或字符串中字符数。
f = os.listdir("./")
for file in f:
print(file.split("."))
print("该列表长度:"+str(len(file.split("."))))
结果:
['', 'idea']
该列表长度:2
['main', 'py']
该列表长度:2
- assert
如:
s = os.path.join("./", "dataset", "flower")
assert os.path.exists(s), "not found {} file".format(s)
路径不存在时得到红色报错:
Traceback (most recent call last):
File "D:/DUTpostgraduate/pytorch练习/pythonpractice/main.py", line 9, in <module>
assert os.path.exists(s), "not found {} file".format(s)
AssertionError: not found ./dataset\flower file
路径存在则不会报错,什么也不输出。
- open:打开文件操作。
看这个:https://www.runoob.com/python/file-methods.html file.read([size]):从文件读取指定的字节数,如果未给定或为负则读取所有 例子:
with open("./a.txt") as a:
text = a.read()
print(text)
这是文件a: 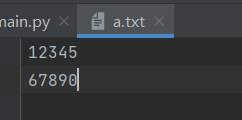 输出结果:
12345
67890
file.readlines([sizeint]):读取所有行(空格和换行符也读)并返回列表,若给定sizeint>0,则是设置一次读多少字节,这是为了减轻读取压力。 例子:
with open("./a.txt") as a:
print(a.readlines())
输出结果:
['12345\n', '67890']
- strip():用于移除字符串头尾指定的字符(默认为空格或换行符)或字符序列。
用法:str.strip([chars]) str.strip()用于去掉末尾空格或换行符。 例子:分别执行上两行和下两行
with open("./a.txt", mode='r') as a:
a_list = [text for text in a.readlines()]
print("没加strip()函数前:" + str(a_list))
输出:
没加strip()函数前:['12345\n', '67890']
加strip()函数后:['12345', '67890']
|