1.前言
实验网站:网易云(https://music.163.com/) 本次实验主要用于练习cookie的使用
2.环境
python 3.8.7 selenium 3.141.0
3.代码
导入库
import json
import os
import re
import time
import pymysql
from selenium.webdriver import Chrome, ChromeOptions
输入账号密码
print("******欢迎使用tzh的程序,输入网易云账号密码自动获取近一周常听歌单******")
user = input("输入账号:")
passw = input("输入密码:")
设置等待时间
driver.implicitly_wait(20)
初始化谷歌驱动
def init_chrome():
driver_path = r"chromedriver.exe"
broswer_path = r"C:\Program Files\Google\Chrome\Application\chrome.exe"
Options = ChromeOptions()
Options.binary_location = broswer_path
Options.add_argument(
'user-agent="Mozilla/5.0 (Windows NT 10.0; Win64; x64) ""AppleWebKit/537.36 '
'(KHTML, like Gecko) Chrome/93.0.4577.63 Safari/537.36"')
Options.add_argument('headless')
return Chrome(options=Options, executable_path=driver_path)
登录操作
def login(username, password):
driver = init_chrome()
driver.implicitly_wait(30)
driver.get("https://music.163.com/")
driver.delete_all_cookies()
driver.maximize_window()
driver.find_element_by_xpath('/html/body/div[1]/div[1]/div/div[1]/a').click()
driver.find_element_by_xpath('//a[@class="u-btn2 other"]').click()
driver.find_element_by_xpath('//*[@id="j-official-terms"]').click()
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[1]/div[1]/div[2]/a').click()
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[3]/div[3]/a').click()
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[1]/div/div/input').send_keys(username)
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[2]/div[1]/input').send_keys(password)
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[4]/a').click()
time.sleep(1)
cookie = driver.get_cookies()
with open("./user_cookies/" + str(user) + ".txt", "w+", encoding="utf-8") as f:
f.write(json.dumps(cookie))
driver.close()
print("获取cookie成功")
进入榜单页面
def get_hobby(username):
driver = init_chrome()
driver.implicitly_wait(20)
driver.get("https://music.163.com/#")
driver.delete_all_cookies()
with open("./user_cookies/" + username + ".txt", "r")as f:
for line in json.loads(f.read()):
driver.add_cookie(line)
driver.get("https://music.163.com/#")
driver.maximize_window()
with open("./user_html/" + str(user) + "_html.txt", "w+", encoding="utf-8") as f:
f.write(driver.page_source)
if len(re.findall("动态", driver.page_source)) == 1:
print("fail")
else:
print("success")
get_top10(driver)
获取本周歌单播放量前十并写入数据库(数据库操作可自行删除省略)
def get_top10(driver):
driver.switch_to.window(driver.window_handles[-1])
id = re.findall(r'GUser={userId:(.*?),nickname:"KeepppGoing",avatarUrl', driver.page_source)
if len(id) == 1:
id = id[0]
driver.get('https://music.163.com/#/user/home?id=' + id)
driver.switch_to.window(driver.window_handles[-1])
driver.switch_to.frame('g_iframe')
time.sleep(1)
song_name = re.findall('<b title="(.*?)">', driver.page_source)
singer_name = re.findall('<span title="(.*?)">', driver.page_source)
listen_time = re.findall('<span class="times f-ff2">(.*?)次</span>', driver.page_source)
if len(song_name) == len(singer_name) == len(listen_time):
for i in range(10):
connect = pymysql.connect(user="", password="",
database="", host="",
port=)
cursor = connect.cursor()
cursor.execute("""
CREATE TABLE IF NOT EXISTS `wyymusic` (
`username` varchar(50) NOT NULL,
`password` varchar(50) NOT NULL,
`song_name` varchar(50) NOT NULL,
`singer_name` varchar(50) NOT NULL,
`listen_time` varchar(50) NOT NULL,
`select_time` varchar(50) NOT NULL
) """)
connect.commit()
cursor.execute('insert into wyymusic values (%s,%s,%s,%s,%s,%s)',
(user, passw, song_name[i], singer_name[i], listen_time[i],
str(time.asctime(time.localtime(time.time())))))
print("歌名:" + song_name[i] + "歌手名::" + singer_name[i] + "听歌次数:" + listen_time[i])
connect.commit()
cursor.close()
connect.close()
driver.close()
定义一个运行自检的function
def judge_exist(username, password):
if username + '.txt' in list(os.walk(r"./user_cookies"))[0][2]:
print("用户cookie存在")
try:
print(get_hobby(username))
except Exception as e:
print(e)
print("cookie失效")
login(username, password)
get_hobby(username)
finally:
print("运行完毕")
else:
print("无用户cookie")
try:
login(username, password)
get_hobby(username)
except Exception as e:
print(e)
print("获取cookie失败")
finally:
print("运行完毕")
调用函数
if __name__ == '__main__':
judge_exist(user, passw)
完整代码
import json
import os
import re
import time
import pymysql
from selenium.webdriver import Chrome, ChromeOptions
print("******欢迎使用tzh的程序,输入网易云账号密码自动获取近一周常听歌单******")
user = input("输入账号:")
passw = input("输入密码:")
def init_chrome():
driver_path = r"chromedriver.exe"
broswer_path = r"C:\Program Files\Google\Chrome\Application\chrome.exe"
Options = ChromeOptions()
Options.binary_location = broswer_path
Options.add_argument(
'user-agent="Mozilla/5.0 (Windows NT 10.0; Win64; x64) ""AppleWebKit/537.36 '
'(KHTML, like Gecko) Chrome/93.0.4577.63 Safari/537.36"')
Options.add_argument('headless')
return Chrome(options=Options, executable_path=driver_path)
def login(username, password):
driver = init_chrome()
driver.implicitly_wait(30)
driver.get("https://music.163.com/")
driver.delete_all_cookies()
driver.maximize_window()
driver.find_element_by_xpath('/html/body/div[1]/div[1]/div/div[1]/a').click()
driver.find_element_by_xpath('//a[@class="u-btn2 other"]').click()
driver.find_element_by_xpath('//*[@id="j-official-terms"]').click()
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[1]/div[1]/div[2]/a').click()
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[3]/div[3]/a').click()
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[1]/div/div/input').send_keys(username)
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[2]/div[1]/input').send_keys(password)
driver.find_element_by_xpath('/html/body/div[6]/div[2]/div/div[1]/div[4]/a').click()
time.sleep(1)
cookie = driver.get_cookies()
with open("./user_cookies/" + str(user) + ".txt", "w+", encoding="utf-8") as f:
f.write(json.dumps(cookie))
driver.close()
print("获取cookie成功")
def get_hobby(username):
driver = init_chrome()
driver.implicitly_wait(20)
driver.get("https://music.163.com/#")
driver.delete_all_cookies()
with open("./user_cookies/" + username + ".txt", "r")as f:
for line in json.loads(f.read()):
driver.add_cookie(line)
driver.get("https://music.163.com/#")
driver.maximize_window()
with open("./user_html/" + str(user) + "_html.txt", "w+", encoding="utf-8") as f:
f.write(driver.page_source)
if len(re.findall("动态", driver.page_source)) == 1:
print("fail")
else:
print("success")
get_top10(driver)
def get_top10(driver):
driver.switch_to.window(driver.window_handles[-1])
id = re.findall(r'GUser={userId:(.*?),nickname:"KeepppGoing",avatarUrl', driver.page_source)
if len(id) == 1:
id = id[0]
driver.get('https://music.163.com/#/user/home?id=' + id)
driver.switch_to.window(driver.window_handles[-1])
driver.switch_to.frame('g_iframe')
time.sleep(1)
song_name = re.findall('<b title="(.*?)">', driver.page_source)
singer_name = re.findall('<span title="(.*?)">', driver.page_source)
listen_time = re.findall('<span class="times f-ff2">(.*?)次</span>', driver.page_source)
if len(song_name) == len(singer_name) == len(listen_time):
for i in range(10):
connect = pymysql.connect(user="", password="",
database="", host="",
port=)
cursor = connect.cursor()
cursor.execute("""
CREATE TABLE IF NOT EXISTS `wyymusic` (
`username` varchar(50) NOT NULL,
`password` varchar(50) NOT NULL,
`song_name` varchar(50) NOT NULL,
`singer_name` varchar(50) NOT NULL,
`listen_time` varchar(50) NOT NULL,
`select_time` varchar(50) NOT NULL
) """)
connect.commit()
cursor.execute('insert into wyymusic values (%s,%s,%s,%s,%s,%s)',
(user, passw, song_name[i], singer_name[i], listen_time[i],
str(time.asctime(time.localtime(time.time())))))
print("歌名:" + song_name[i] + "歌手名::" + singer_name[i] + "听歌次数:" + listen_time[i])
connect.commit()
cursor.close()
connect.close()
driver.close()
def judge_exist(username, password):
if username + '.txt' in list(os.walk(r"./user_cookies"))[0][2]:
print("用户cookie存在")
try:
print(get_hobby(username))
except Exception as e:
print(e)
print("cookie失效")
login(username, password)
get_hobby(username)
finally:
print("运行完毕")
else:
print("无用户cookie")
try:
login(username, password)
get_hobby(username)
except Exception as e:
print(e)
print("获取cookie失败")
finally:
print("运行完毕")
if __name__ == '__main__':
judge_exist(user, passw)
运行结果↓ 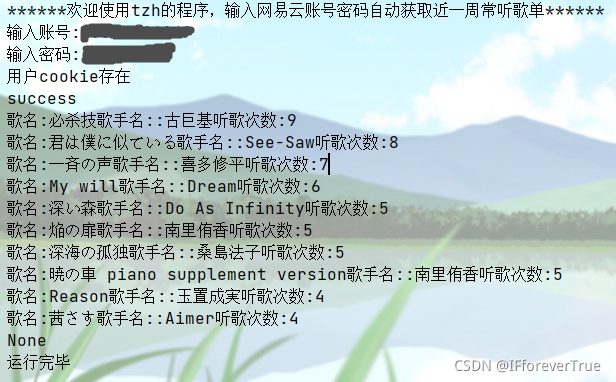 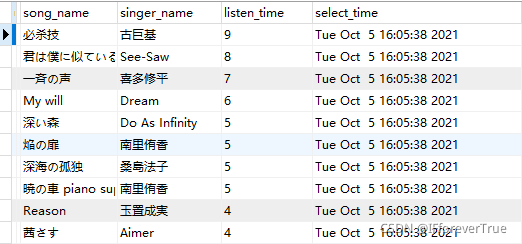 目录结构 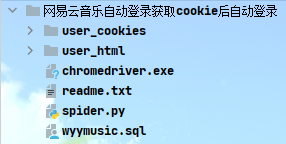
更多功能可自行拓展
谢谢你的浏览(End)
|