1.1 Write a function that takes in a number n and returns a one-argument function. The returned function takes in a function that is used to update n. It should return the updated n.
?
def memory(n): """
>>> f = memory(10)
>>> f(lambda x: x * 2)
20
>>> f(lambda x: x - 7)
13
>>> f(lambda x: x > 5)
True
"""
def f(g):
nonlocal n
n = g(n)
return n
return f
2.1 What would Python display? In addition to giving the output, draw the box and pointer diagrams for each list to the right.?
>>> s1 = [1, 2, 3]
>>> s2 = s1
>>> s1 is s2
True
>>> s2.extend([5, 6])
>>> s1[4]
6
>>> s1.append([-1, 0, 1])
>>> s2[5]
[-1, 0, 1]
>>> s3 = s2[:]
>>> s3.insert(3, s2.pop(3))
#在s3 range的3位插入s2弹出的3位,此时s3 = [1,2,3,5,5,6,[-1,0,1]]
>>> len(s1)
5
>>> s1[4] is s3[6]
True
>>> s3[s2[4][1]]
1
>>> s1[:3] is s2[:3]
False
>>> s1[:3] == s2[:3]
True
2.2 Fill in the lines below so that the variables in the global frame are bound to the values below. Note that the image does not contain a full environment diagram. You may only use brackets, commas, colons, p , and q in your answer.
def mystery(p, q): p[1].extend(__________) ___________.append(____[1:]_)
p = [2, 3]
q = [4, [p]]
mystery(_______, __________)
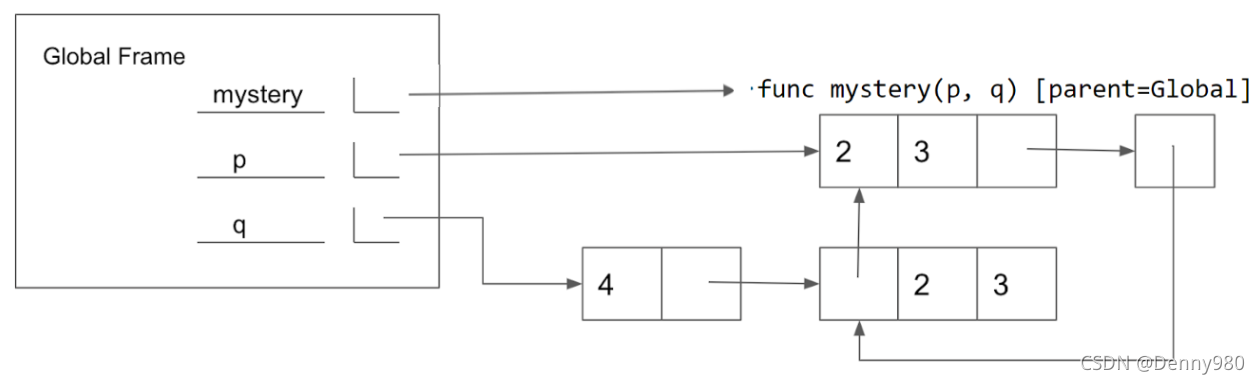
2.3 Tutorial: Write a function that takes in a sequence s and a function fn and returns a dictionary.
?The values of the dictionary are lists of elements from s. Each element e in a list should be constructed such that fn(e) is the same for all elements in that list. The key for each value should be fn(e). For each element e in s, check the value that calling fn(e) returns, and add e to the corresponding group.
def group_by(s, fn): """
>>> group_by([12, 23, 14, 45], lambda p: p // 10)
{1: [12, 14], 2: [23], 4: [45]}
>>> group_by(range(-3, 4), lambda x: x * x)
{9: [-3, 3], 4: [-2, 2], 1: [-1, 1], 0: [0]}
"""
grouped = {}
for p in s:
key = _________________
if ______________________________________:
return____________________________________
else:
grouped[key] = ___________________________________
return _________________________________
grouped = {}
for x in s:
key = fn(x)
if key in grouped:
return grouped[key].append(x)
else:
grouped[key] = [x]
return grouped
2.4 Tutorial:
Write a function that takes in a value x, a value el, and a list s and adds as many el’s to the end of the list as there are x’s.
Make sure to modify the original list using list mutation techniques.
def add_this_many(x, el, s):
""" Adds el to the end of s the number of times x occurs in s.
>>> s = [1, 2, 4, 2, 1]
>>> add_this_many(1, 5, s)
>>> s
[1, 2, 4, 2, 1, 5, 5]
>>> add_this_many(2, 2, s)
>>> s
[1, 2, 4, 2, 1, 5, 5, 2, 2]
"""
count = 0
for element in s:
if element == x:
count += 1
while count > 0:
s.append(el)
count -= 1
3.1 What would Python display?
>>> s = [[1, 2]]
>>> i = iter(s)
>>> j = iter(next(i))
>>> next(j)
1
>>> s.append(3)
>>> next(i)
3
>>> next(j)
2
>>> next(i)
StopIteration
4.1 Implement a generator function called filter(iterable, fn) that only yields elements of iterable for which fn returns True.
def filter(iterable, fn):
"""
>>> is_even = lambda x: x % 2 == 0
>>> list(filter(range(5), is_even)) # a list of the values yielded from the call to filter [0, 2, 4]
>>> all_odd = (2*y-1 for y in range(5))
>>> list(filter(all_odd, is_even))
[]
>>> naturals = (n for n in range(1, 100))
>>> s = filter(naturals, is_even)
>>> next(s)
2
>>> next(s)
4
"""
for x in iterable:
if fn(x):
yield x
python 中 filter的用法_共同学习-CSDN博客?
Python函数式编程(fn)_a栋的博客-CSDN博客
4.2 Tutorial: Write a generator function merge that takes in two infinite generators a and b that are in increasing order without duplicates and returns a generator that has all the elements of both generators, in increasing order, without duplicates.
def merge(a, b):
"""
>>> def sequence(start, step):
...
...
...
>>> a = sequence(2, 3) # 2, 5, 8, 11, 14, ...
>>> b = sequence(3, 2) # 3, 5, 7, 9, 11, 13, 15, ...
>>> result = merge(a, b) # 2, 3, 5, 7, 8, 9, 11, 13, 14, 15
>>> [next(result) for _ in range(10)]
[2, 3, 5, 7, 8, 9, 11, 13, 14, 15]
"""
first_a, first_b = next(a), next(b)
while True:
if first_a == first_b:
yield first_a
first_a, first_b = next(a), next(b)
elif first_a < first_b:
yield first_a
first_a = next(a)
else:
yield first_b
first_b = next(b)
【python】详解pandas库的pd.merge函数_brucewong0516的博客-CSDN博客
|