一、基本介绍
1.使用的python开发工具是Anaconda中的Spyder 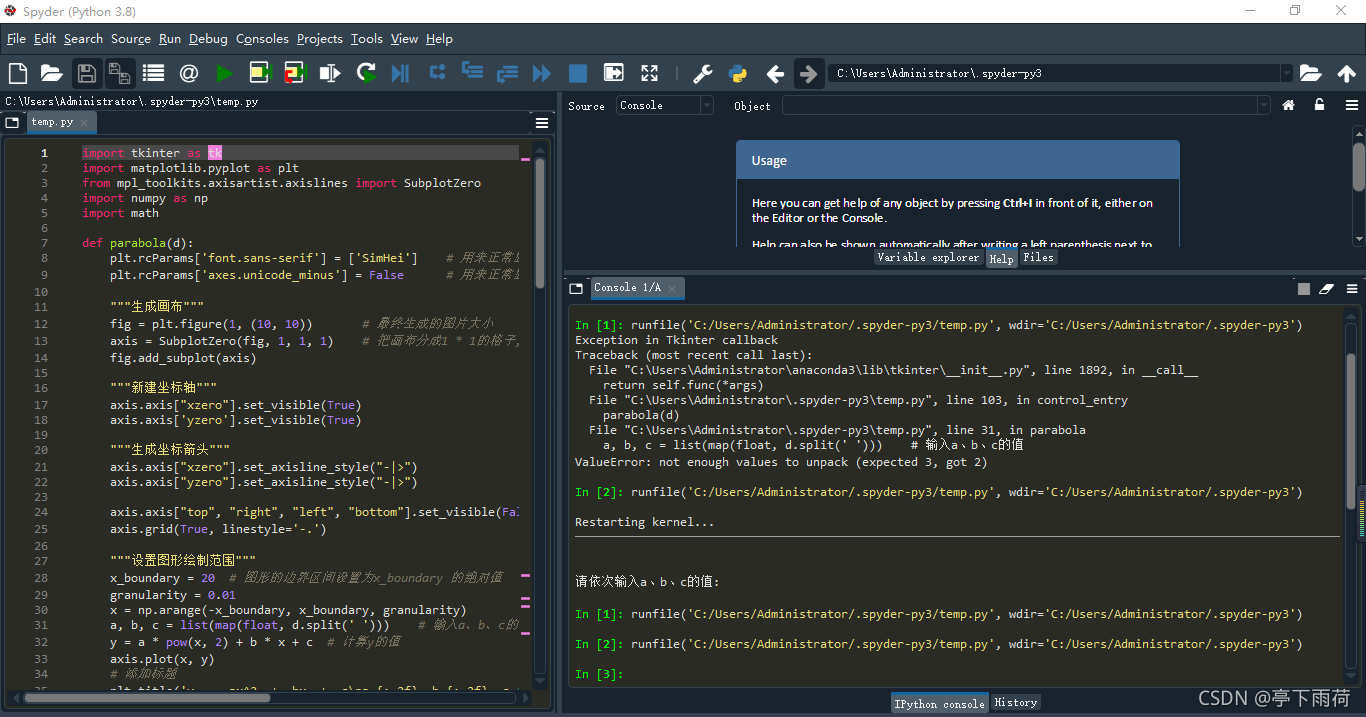 这个工具比较好用,安装步骤直接百度即可。 2.原始代码用到了几个库,用到了matplotlib库等,百度添加相应的库即可。 3.原始代码的主要流程:main函数------>生成界面------>点击按钮调用输入函数------->调用一元二次方程代码函数。
二、具体代码
这样就得到了图形界面与代码的结合。
import tkinter as tk
import matplotlib.pyplot as plt
from mpl_toolkits.axisartist.axislines import SubplotZero
import numpy as np
import math
def parabola(d):
plt.rcParams['font.sans-serif'] = ['SimHei']
plt.rcParams['axes.unicode_minus'] = False
"""生成画布"""
fig = plt.figure(1, (10, 10))
axis = SubplotZero(fig, 1, 1, 1)
fig.add_subplot(axis)
"""新建坐标轴"""
axis.axis["xzero"].set_visible(True)
axis.axis['yzero'].set_visible(True)
"""生成坐标箭头"""
axis.axis["xzero"].set_axisline_style("-|>")
axis.axis["yzero"].set_axisline_style("-|>")
axis.axis["top", "right", "left", "bottom"].set_visible(False)
axis.grid(True, linestyle='-.')
"""设置图形绘制范围"""
x_boundary = 20
granularity = 0.01
x = np.arange(-x_boundary, x_boundary, granularity)
a, b, c = list(map(float, d.split(' ')))
y = a * pow(x, 2) + b * x + c
axis.plot(x, y)
plt.title('y = ax^2 + bx + c\na={:.2f}, b={:.2f}, c={:.2f}'.format(a, b, c),
bbox=dict(facecolor='g', edgecolor='blue', alpha=0.65), fontsize='20')
"""根据a、b、c的值画图"""
if a == 0:
plt.text(0, 0, r'$this\ is\ a\ line\ !!!$', fontdict={'size': '20', 'color': 'red'})
plt.text(0, -1, r'$please\ make\ sure\ the\ first\ number\ is\ not\ 0!!!$', fontdict={'size': '20', 'color': 'red'})
extremum_x = 0
extremum_y = 0
else:
extremum_x = - (b / (2 * a))
extremum_y = (4 * a * c - pow(b, 2)) / (4 * a)
plt.scatter(extremum_x, extremum_y)
if a > 0:
point_describe = "小"
else:
point_describe = "大"
plt.text(extremum_x, extremum_y - (a / a.__abs__()),
'(%.2f, %.2f)是极%s值点' % (extremum_x, extremum_y, point_describe),
fontdict={'size': '18', 'color': 'b'})
delta = pow(b, 2) - 4 * a * c
if delta < 0:
plt.text(extremum_x, extremum_y - 2 * (a / a.__abs__()),
'此方程没有实根',
fontdict={'size': '18', 'color': 'red'})
elif delta == 0:
x1 = (-b + math.sqrt(delta)) / (2 * a)
plt.scatter(x1, 0)
plt.text(x1, 0 + 1 * (a / a.__abs__()),
'(%.2f, 0)是唯一实根' % x1,
fontdict={'size': '18', 'color': '#0cf'})
else:
x1 = (-b + math.sqrt(delta)) / (2 * a)
x2 = (-b - math.sqrt(delta)) / (2 * a)
plt.scatter(x1, 0)
plt.scatter(x2, 0)
plt.text(x1, 0 - 1 * (a / a.__abs__()),
'(%.2f, 0)是第一个根' % x1,
fontdict={'size': '18', 'color': '#000'})
plt.text(x2, 0 + 1 * (a / a.__abs__()),
'(%.2f, 0)是第二个根' % x2,
fontdict={'size': '18', 'color': '#000'})
"""根据极值点动态调整坐标轴范围"""
axis.set_xlim([extremum_x - 10, extremum_x + 10])
axis.set_ylim([-extremum_y.__abs__() - 10, extremum_y.__abs__() + 10])
plt.text(extremum_x + 10, 0.5, 'x', fontdict={'size': '18', 'color': '#000'})
plt.text(0.5, extremum_y.__abs__() + 9.2, 'y', fontdict={'size': '18', 'color': '#000'})
"""显示图片"""
plt.show()
def control_entry():
d = entry_parabola.get()
parabola(d)
if __name__ == '__main__':
root = tk.Tk()
root.title('一元函数画图')
root.geometry('250x100')
label_parabola = tk.Label(root, text='y=ax^2+bx+c,请输入a,b,c的值,用空格隔开')
entry_parabola = tk.Entry(root)
label_parabola.pack()
entry_parabola.pack()
button_gen = tk.Button(
root,
text='点击按钮',
command=control_entry)
button_gen.pack()
root.mainloop()
三、制作软件
直接百度:python打包 即可。 打包完成后,后缀为exe文件,如图: 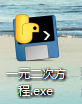
四、总结
如此就可以制作一个简单的python软件。界面做的更美观些,多添加几个函数,就可以做成我的博客中的基本函数画图软件。  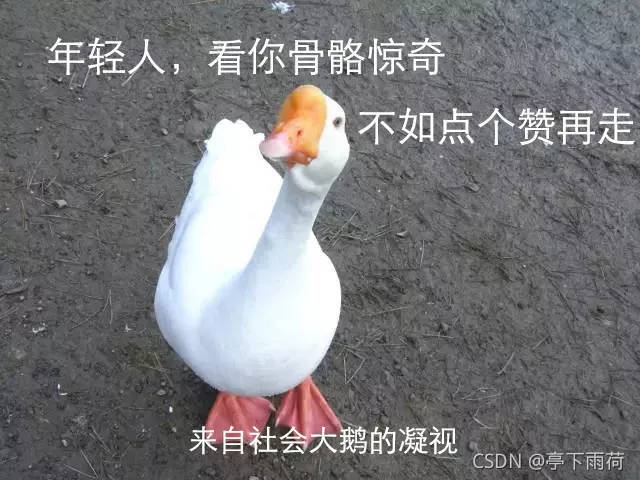 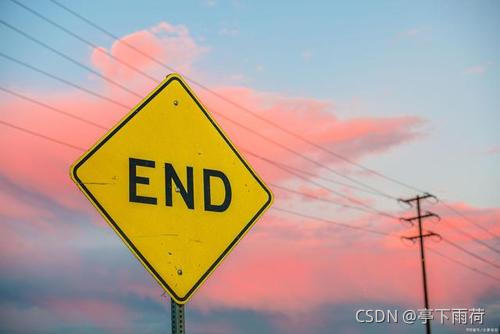
|