虽然但是,隔了三天,不过我是去做正事了~ 参加了一个数学建模比赛,本来有把代码和思路放上来的想法,但是因为太不成熟了,就放弃了【doge】
'''冒泡排序'''
def bubbleSort(ListA):
for passNum in range(len(ListA) - 1, 0, -1):
for i in range(passNum):
if ListA[i] > ListA[i + 1]:
ListA[i], ListA[i + 1] = ListA[i + 1], ListA[i]
'''快速排序'''
def quickSort(ListA):
qucikSortHelper(ListA,0,len(ListA)-1)
pass
def qucikSortHelper(ListA,first,last):
if first< last:
splitpoint = partition(ListA,first,last)
qucikSortHelper(ListA,first,splitpoint-1)
qucikSortHelper(ListA,splitpoint+1,last)
pass
def partition(ListA,first,last):
pivotvalue = ListA[first]
leftmark = first+1
rightmark = last
done = False
while not done:
while leftmark <=rightmark and \
ListA[leftmark] < pivotvalue:
leftmark += 1
while leftmark <= rightmark and \
ListA[rightmark] > pivotvalue:
rightmark -= 1
if rightmark < leftmark:
done = True
else:
ListA[leftmark],ListA[rightmark] = ListA[rightmark],ListA[leftmark]
ListA[first],ListA[rightmark] = ListA[rightmark],ListA[first]
return rightmark
pass
'''堆排序'''
def sift(ListA, low, high):
'''
调整根堆
:param ListA:
:param low:
:param high:
:return:
'''
i = low
j = 2 * i + 1
tmp = ListA[low]
while j<=high:
if j+1 <=high and ListA[j + 1]>ListA[j]:
j = j+1
if ListA[j]>tmp:
ListA[i] = ListA[j]
i = j
j = 2*i + 1
else:
ListA[i] = tmp
break
else:
ListA[i] = tmp
def heapSort(ListA):
n = len(ListA)
for i in range((n-2)//2, -1, -1):
sift(ListA, i, n - 1)
for i in range(n-1,-1,-1):
ListA[0], ListA[i] = ListA[i], ListA[0]
sift(ListA, 0, i - 1)
'''希尔排序'''
def insertSortGap(li, gap):
for i in range(gap, len(li)):
tmp = li[i]
j = i-gap
while j >= 0 and li[j] > tmp:
li[j+gap] = li[j]
j -= gap
li[j+gap] = tmp
def shellSort(li):
d = len(li)//2
while d >= 1:
insertSortGap(li,d)
d //= 2
'''二叉树遍历'''
if __name__ == '__main__':
ListA = [2,1,3,5,4]
print(ListA)
shellSort(ListA)
print(ListA)
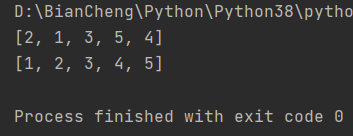
|