列表
列表按值移除元素
room = ['mushroom','bathroom']
room.remove('mushroom')
del room[0]
列表解析
square = [value**2 for value in range(1,11)]
print(square)
列表复制,采用切片更好
mycheng = square[:]
print(mycheng)
sorted对列表临时排序
for name in sorted(dict.keys()):
print()
检查元素是否存在用in
room = ['mushroom','bathroom']
'mushroom' in room
用户输入输出
message = input("Tell me somethiing,I will repeat it to you: ")
print(message)
number = input("Please input a number:")
int(number)
prompt = "\nPLease tell me something and I will repeat it to you, "
prompt += "\nEnter quit to end the program! "
message=""
空字符串、列表、字典
message = ""
message = []
message = {}
字符串表示
python字符串,单引号双引号括起来都可。
字符大小写
title()首字母大写
upper()全大写
lower()全小写
在字符串中插入变量的值
f字符串
f"{变量1} {变量2}"
注意中间有空格,由此可以得到变量1和变量2连接起来
python3.6引入,如果是之前的版本就需要使用format方法
制表符、换行符
\t \n 反斜杠
删除空白
rstrip() 去除右边空白
lstrip()
strip()
简单的分支结构
age = 21
if age<20:
print("aicc")
elif age>=21:
print("aicc")
else:
print("aicc")
requested_toppings=[]
if requested_toppings:
print("")
else:
print("we are out of peppers")
简单while举例
current_number = 1
while current_number <=5:
current_number+=1
active = True
unconfirmed_users = ['alice','brian','cancade']
conformed_users = []
while unconfirmed_users:
user = unconfirmed_users.pop()
conformed_users.append(user)
元组
dimensions = (200,50)
dimensions1 = (200,)
print(dimensions[0])
print(dimensions[1])
dimensions = (400,500)
字典
dict = {'color':'green','points':5}
print(dict['color'],dict['points'])
dict.get('points','No such key in this dict')
for a,b in dict.items():
print(f"Key:{a}")
print(f"Value:{b}")
函数
def greet_user(username,username1='lcc',middle_name=''):
"""显示简单的问候语"""
print("Hello")
return username
greet_user(username='cc',username1='lcc')
def greet_users(names):
for name in names:
print(f"Hello,{name.title()}")
usenames=['alice','ljc','xcc']
greet_users(usenames)
greet_users(usenames[:])
def make_pizza(*toppings):
print(toppings)
def make_pizza(**user_info):
print(user_info)
import
import numpy as np
python类
class Dog():
"""模拟小狗的简单尝试"""
def __init__(self,name,age):
self.name = name
self.age = age
self.odometer_reading = 0
def sit(self):
"""模拟小狗蹲下"""
print(f"{self.name} is now sitting")
def roll_over(self):
"""打滚"""
print(f"{self.name} is rolling banck")
my_dog = Dog('cc',6)
print(f"my_dog's name is {my_dog.name}")
print(f"my_dog's age is {my_dog.age}")
class ElectDog(Dog,Dog):
def __init__(self,name,age):
super().__init__(name,age)
Dog.__init__(name,age)
异常
try:
5/0
except ZeroDivisionError:
print("nononon")
else:
print()
文件读取
file_path = 'C:/Users/mxt/Desktop/源代码文件/chapter_10/write_mes.txt'
with open(file_path,'w') as file_object:
file_object.write("I love programming")
json模块
import json
numbers = [2,3,5,7,11,13]
filename = 'number.json'
with open(filename,'w') as f:
json.dump(numbers,f)
with open(filename) as f:
numbers = json.load(f)
unittest类
import unittest
from xx import get_formatted_name
class NamesTestCase(unittest.TestCase):
"""测试name_function.py"""
def test_first_last_name(self):
"""能够正确处理像Jains Joplin这样的名字吗"""
formatted_name = get_formatted_name('jains','joplin')
self.assertEqual(formatted_name,'Jains joplin')
if __name__ == '__main__':
unittest.main()
数据可视化
import matplotlib.pyplot as plt
squares = [23,34,56,78]
plt.plot(squares,linewidth = 5)
plt.title("Accuracy",fontsize = 24)
plt.xlabel("Value",fontsize = 14)
plt.ylabel("Square of value",fontsize = 14)
plt.tick_params(axis='both',labelsize = 14)
plt.show()
plt.plot(input_values,squares,linewidth = 5)
散点scatter
import matplotlib.pyplot as plt
plt.scatter(2,4,s = 200)
plt.show()
import matplotlib.pyplot as plt
x = [1,2,3,4,5]
y = [1,4,9,16,25]
plt.scatter(x,y,s = 200)
plt.show()
import matplotlib.pyplot as plt
x = list(range(1,1001))
y = [i**2 for i in x]
plt.scatter(x,y,s = 10)
plt.axis([0,1000,0,110000])
plt.show()
plt.scatter(x,y,c = y, cmap= plt.cm.Blues,edgecolors='none',s = 40)
plt.savefig('scatte.jpg',bbox_inches = 'tight')
随机漫步的生成
"""RandomWalk.py"""
from random import choice
class RandomWalk():
def __init__(self,num_points=5000):
self.num_points = num_points
self.x = [0]
self.y = [0]
def fill_walk(self):
"""计算x,y,即所有点"""
while len(self.x) < self.num_points:
x_dir = choice([1,-1])
x_dis = choice([0,1,2,3,4])
x_step = x_dis*x_dir
y_dir = choice([1, -1])
y_dis = choice([0, 1, 2, 3, 4])
y_step = y_dis * y_dir
if x_step == 0 and y_step == 0:
continue
next_x = self.x[-1] + x_step
next_y = self.y[-1] + y_step
self.x.append(next_x)
self.y.append(next_y)
一次漫步
from RandomWalk import RandomWalk
import matplotlib.pyplot as plt
rw = RandomWalk()
rw.fill_walk()
plt.scatter(rw.x,rw.y,s=15)
plt.show()
多次漫步
from RandomWalk import RandomWalk
import matplotlib.pyplot as plt
while True:
rw = RandomWalk()
rw.fill_walk()
plt.scatter(rw.x, rw.y, s=15)
plt.show()
keep_running = input("Make another walk?(y/n): ")
if keep_running == 'n':
break
颜色变化,绘点着色
from RandomWalk import RandomWalk
import matplotlib.pyplot as plt
while True:
rw = RandomWalk()
rw.fill_walk()
point_numbers = list(range(rw.num_points))
plt.scatter(rw.x, rw.y,c = point_numbers,cmap = plt.cm.Blues, s=15)
plt.show()
keep_running = input("Make another walk?(y/n): ")
if keep_running == 'n':
break
比较完整地进行一系列操作之后的代码
from RandomWalk import RandomWalk
import matplotlib.pyplot as plt
while True:
rw = RandomWalk(50000)
rw.fill_walk()
point_numbers = list(range(rw.num_points))
plt.figure(dpi = 80,figsize=(10,6))
cnaxes = plt.axes()
cnaxes.xaxis.set_visible(False)
cnaxes.yaxis.set_visible(False)
plt.scatter(rw.x, rw.y,c = point_numbers,cmap = plt.cm.Blues, s=15)
plt.scatter(0,0,c = 'green',edgecolors='none',s = 100)
plt.scatter(rw.x[-1],rw.y[-1],c = 'red',edgecolors='none',s = 100)
plt.show()
keep_running = input("Make another walk?(y/n): ")
if keep_running == 'n':
break
掷骰子
pygals骰子类
from random import randint
class Die():
"""表示一个骰子"""
def __init__(self,num_sides = 6):
self.num_sides = num_sides
def roll(self):
return randint(1,self.num_sides)
from pygals import Die
import pygal
die = Die()
res = []
for i in range(100):
res.append(die.roll())
fre = []
for value in range(1,die.num_sides+1):
frequency = res.count(value)
fre.append(frequency)
hist = pygal.Bar()
hist.title = "Result of rolling a D6 100 times"
hist.x_labels = ['1','2','3','4','5','6']
hist.x_title = "Result"
hist.y_title = "Frequency of Results"
hist.add('D6',fre)
hist.render_to_file('die_visual.svg')
读取csv以及json文件
csv文件
import csv
from datetime import datetime
import matplotlib.pyplot as plt
filename = 'C:/Users/mxt/Desktop/sitka_weather_2018_full.csv'
f_name = 'sitka_weather_2018_full.csv'
with open(filename) as f:
reader = csv.reader(f)
header_row = next(reader)
highs,dates = [],[]
for row in reader:
current_time = datetime.strptime(row[2],'%Y-%m-%d')
dates.append(current_time)
if row[4]:
highs.append(int(row[4]))
else:
highs.append(-1)
fig = plt.figure(dpi = 128,figsize=(10,6))
plt.plot(dates,highs)
plt.title('Date and tempature')
plt.xlabel('Date',fontsize = 16)
plt.ylabel('temp',fontsize = 10)
fig.autofmt_xdate()
plt.tick_params(axis='both',which = 'major')
plt.show()
fill_between() 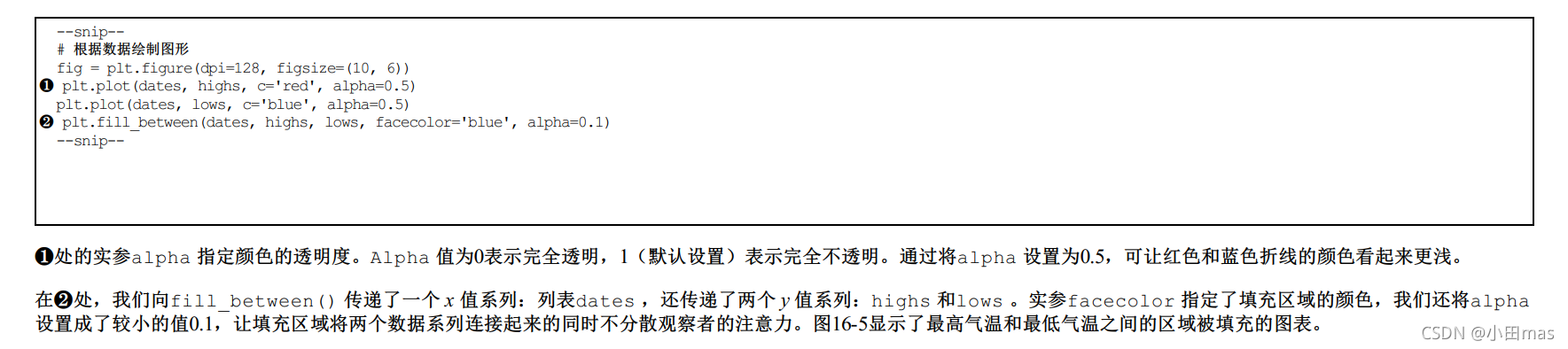 字符串表示的是一个浮点数就不能直接用int转换,需要先采用float()函数,再采用int()函数去掉小数部分。
|