一、Hi,Flask!
在pycharm里创建新文件,命名为main.py
from flask import Flask,render_template
app=Flask(__name__)
#__name__是类,区别于app这个实例,app继承类的所有属性和方法
@app.route('/')
def index():
return("Hi,Flask!")
if "__main__"==__name__:
app.run(port="5008")
#5008可改为其他,默认5000
运行结果:

打开该网页即可在前端看到“Hi,Flask!”
二、templates文件夹
新建文件夹,命名为templates(必须命名为templates),用于存放所有html文件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Flask 分镜</title>
</head>
<body>
视频分镜
</body>
</html>
同时将main.py中的代码修改为:
from flask import Flask,render_template
app=Flask(__name__)
#__name__是类,区别于app这个实例,app继承类的所有属性和方法
@app.route('/')
def index():
#return("Hi,Flask!")
return render_template('index.html')
if "__main__"==__name__:
app.run(port="5008")
#5008可改为其他,默认5000
?运行后可在网页端得到“视频分镜”字样
三、视频分镜
新建文件夹static,用于存放视频、图片等文件
定义genFrame()用于对视频进行分帧:
from flask import Flask,render_template
import os
import cv2
app=Flask(__name__)
def genFrame():
v_path='static/视频名.mp4'
image_save='static/pic'
if not(os.path.exists(image_save)):
os.mkdir(image_save)
cap=cv2.VideoCapture(v_path)
fc=cap.get(cv2.CAP_PROP_FRAME_COUNT)
for i in range(int(fc)):
_,img=cap.read()
cv2.imwrite('static/pic/image{}.jpg'.format(i),img)
@app.route('/')
def index():
genFrame()
return render_template('index.html')
if "__main__"==__name__:
app.run()
修改html文件代码为:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Flask 分镜</title>
</head>
<body>
视频分镜
<br>
<video width="640" height="480" controls autoplay>
<source src="ghz.mp4" type="video/mp4">
<object data="ghz.mp4" width="640" height="480">
<embed width="640" height="480" src="ghz.mp4">
</object>
</video>
<br>
帧数:{{framecount}}<br>
{% for i in range(framecount) %}
<img height="20" src="{{pic1}}{{i}}.jpg" />
{% endfor %}
</body>
</html>
其中:{undefined{}}里面是绑定的参数,{%%}里面可以简单使用一些python的语句,是在html里调用python语句的用法
运行结果: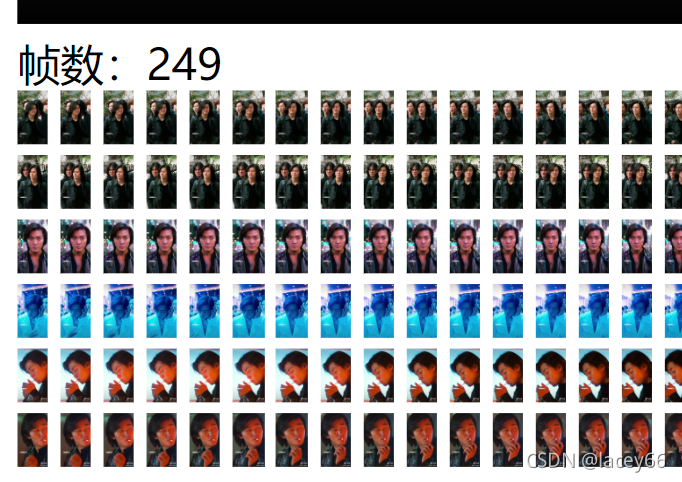
四、使用哈希算法进行视频分镜
在templates新建hash.html文件,并在文件中引用哈希算法:
import cv2
import numpy as np
import matplotlib.pyplot as plt
import os
def aHash(img):
# plt.imshow(img)
# plt.axis('off')
# plt.show()
img = cv2.resize(img, (8, 8))
# plt.imshow(img)
# plt.axis('off')
# plt.show()
# 转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
s = 0
hash_str = ''
# 遍历累加求像素和
for i in range(8):
for j in range(8):
s = s + gray[i, j]
# 求平均灰度
avg = s / 64
# 灰度大于平均值为1相反为0生成图片的hash值
for i in range(8):
for j in range(8):
if gray[i, j] > avg:
hash_str = hash_str + '1'
else:
hash_str = hash_str + '0'
return hash_str
# Hash值对比
def cmpHash(hash1, hash2):
n = 0
print(hash1)
print(hash2)
# hash长度不同则返回-1代表传参出错
if len(hash1) != len(hash2):
return -1
# 遍历判断
for i in range(len(hash1)):
# 不相等则n计数+1,n最终为相似度
if hash1[i] != hash2[i]:
n = n + 1
return n
# img1 = cv2.imread('pic/image0.jpg') # 11--- 16 ----13 ---- 0.43
# img2 = cv2.imread('pic/image1.jpg')
#
# hash1 = aHash(img1)
# hash2 = aHash(img2)
# n = cmpHash(hash1, hash2)
# print('均值哈希算法相似度:', n)
#
# n = classify_hist_with_split(img1, img2)
# print('三直方图算法相似度:', n)
def genFrame():
v_path='static/ghz.mp4'
image_save='static/hash'
if not(os.path.exists(image_save)):
os.mkdir(image_save)
cap=cv2.VideoCapture(v_path)
fc=cap.get(cv2.CAP_PROP_FRAME_COUNT)
_, img1 = cap.read()
cv2.imwrite('static/hash/image{}.jpg'.format(0), img1)
for i in range(int(fc)-1):
_, img2 = cap.read()
hash1 = aHash(img1)
hash2 = aHash(img2)
n = cmpHash(hash1, hash2)
if (n>40):
cv2.imwrite('static/hash/image{}.jpg'.format(i),img2)
img1=img2
genFrame()
注意:n的值随引用视频的不同而变化,n的值不合适可能会报错
index.html代码几乎不变,将main.py修改成:
from flask import Flask,render_template,request
import os
import cv2
import imageColor
app = Flask(__name__)
def genFrame():
v_path = 'ghz.mp4'
image_save = 'pic'
if not (os.path.exists(image_save)):
os.mkdir(image_save)
cap = cv2.VideoCapture(v_path)
fc = cap.get(cv2.CAP_PROP_FRAME_COUNT)
for i in range(int(fc)):
_,img=cap.read()
cv2.imwrite('static/pic/image{}.jpg'.format(i),img)
@app.route('/')
def index():
pic='static/pic/image'
framecount=500
return render_template('index.html',pic1=pic,framecount=framecount)
@app.route('/hash')
def hash():
path='static/hash'
filename=os.listdir(path)
print(type(filename))
print(filename)
imgcount=len(filename)
return render_template('hash.html',imgcount=imgcount,filename=filename)
if "__main__"==__name__:
app.run(port="5008")
?分帧后图片存在hash文件夹中,一共5张
网页端运行结果显示为:
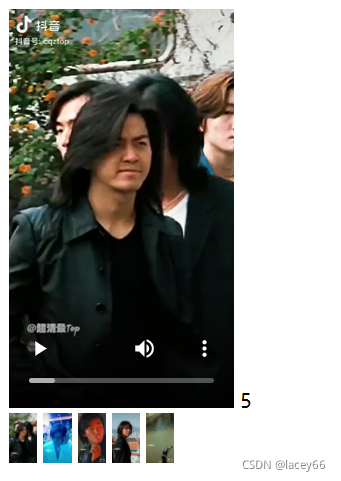
?
|