一、测试阶段
1-安装PyCharm
2-在PyCharm里创建一个项目,如果main.py里有内容,删除
3-在main.py里录入
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if "__main__"==__name__:
app.run(port="5008")
运行后点开链接http://127.0.0.1:5008/?
能看到这个图?
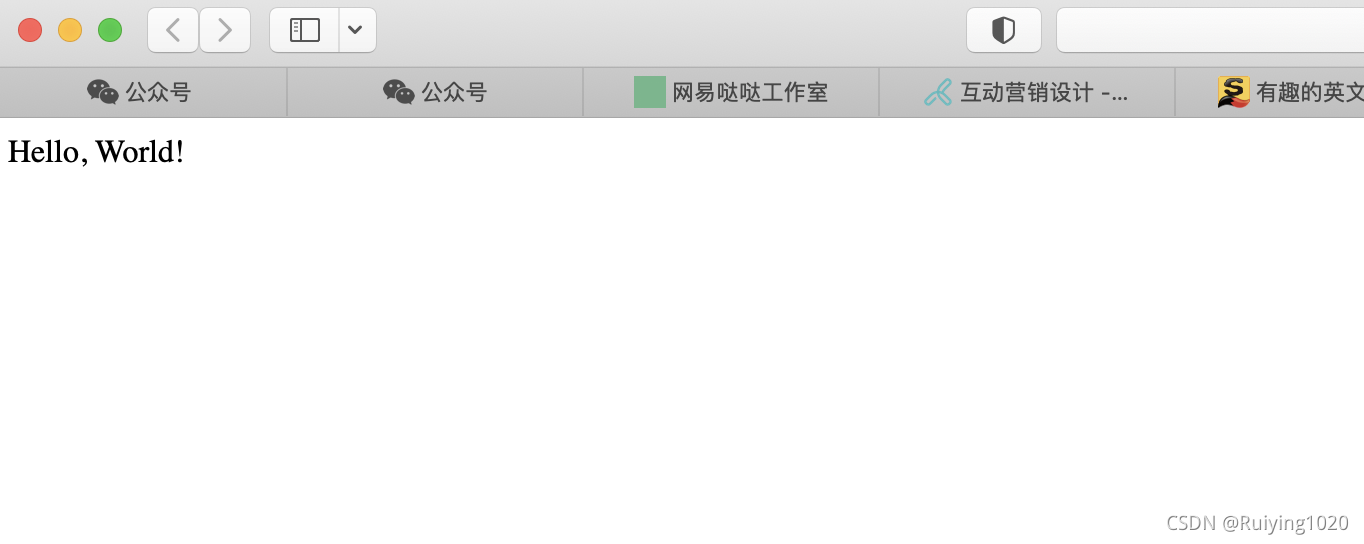
说明运行正常!
二、添加templates和html
?1.?修改main.py
from flask import Flask,render_template
app=Flask(__name__)
@app.route('/')
def index():
#return "Hi,Flask!"
return render_template('index.html')
if "__main__"==__name__:
app.run(port="5008")
2. 在项目中创建文件夹templates
3. 在Templates里新建一个HTML文件,命名为index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Flask分镜</title>
</head>
<body>
视频分镜
</body>
</html>
再次运行main.py
得到结果
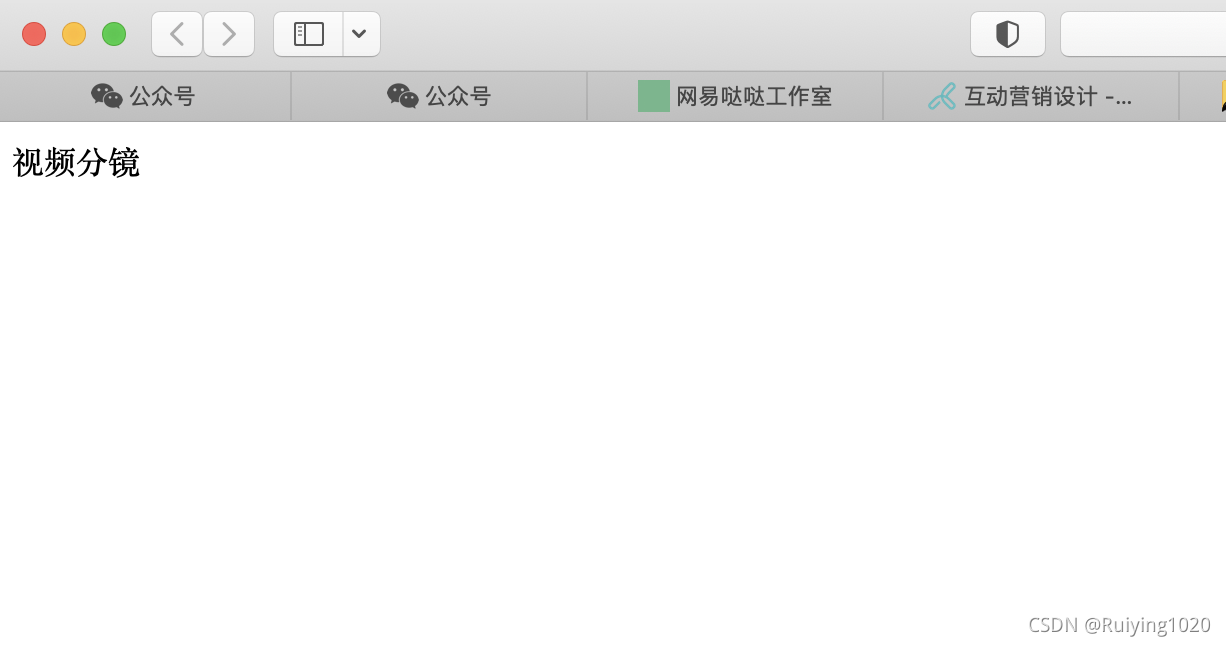
?三、利用flask进行视频分镜
1. 在exercise2新建文件夹static,并导入视频,同时在static里面新建pic文件夹
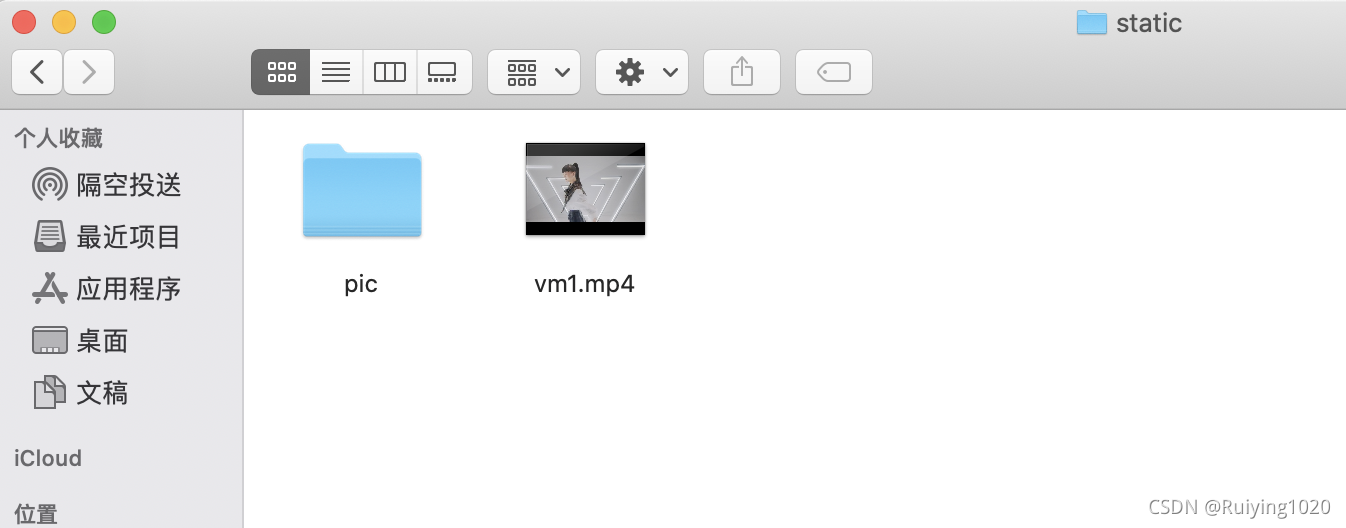
2. 在main.py?进行视频分镜
from flask import Flask,render_template
import os
import cv2
app=Flask(__name__)
def genFrame():
v_path='static/vm1.mp4'
image_save='static/pic'
if not(os.path.exists(image_save)):
os.mkdir(image_save)
cap=cv2.VideoCapture(v_path)
fc=cap.get(cv2.CAP_PROP_FRAME_COUNT)
for i in range(int(fc)):
_,img=cap.read()
cv2.imwrite('static/pic/image{}.jpg'.format(i),img)
@app.route('/')
def index():
#return "Hi,Flask!"
genFrame()
#pic='static/pic/image'
#framecount=825
#return render_template('index.html',pic1=pic,framecount=framecount)
if "__main__"==__name__:
app.run(port="5008")
genFrame()#一定要记得调用,不然没办法分镜
运行后,pic文件夹中就有分好的文件了
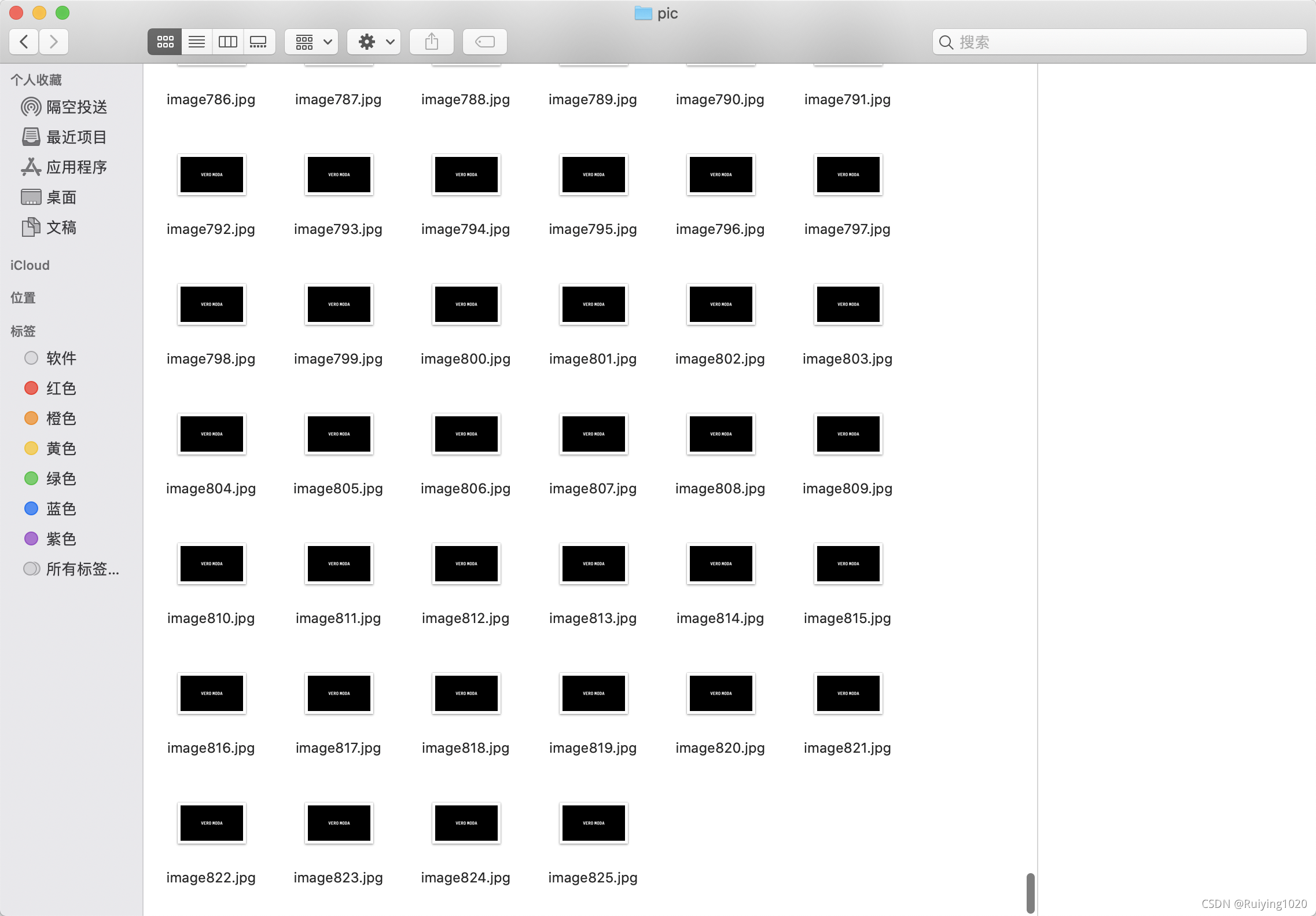
3. 让分镜结果在html中显示
1)先修改main.py文件
from flask import Flask,render_template
import os
import cv2
app=Flask(__name__)
def genFrame():
v_path='static/vm1.mp4'
image_save='static/pic'
if not(os.path.exists(image_save)):
os.mkdir(image_save)
cap=cv2.VideoCapture(v_path)
fc=cap.get(cv2.CAP_PROP_FRAME_COUNT)
for i in range(int(fc)):
_,img=cap.read()
cv2.imwrite('static/pic/image{}.jpg'.format(i),img)
@app.route('/')
def index():
#return "Hi,Flask!"
#genFrame()
pic='static/pic/image'
framecount=825
return render_template('index.html',pic1=pic,framecount=framecount)
if "__main__"==__name__:
app.run(port="5008")
2)再修改html文件
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Flask分镜</title>
</head>
<body>
视频分镜
<br>
<video width="640" height="480" controls autoplay>
<source src="static/vm1.mp4" type="video/mp4">
<object data="static/vm1.mp4" width="640" height="480">
<embed width="640" height="480" src="static/vm1.mp4">
</object>
</video>
<br>
帧数:{{framecount}}<br>
{% for i in range(framecount) %}
<img height="40" src="{{pic1}}{{i}}.jpg" />
{% endfor %}
</body>
</html>
?运行后,即可在网页看到如下结果:
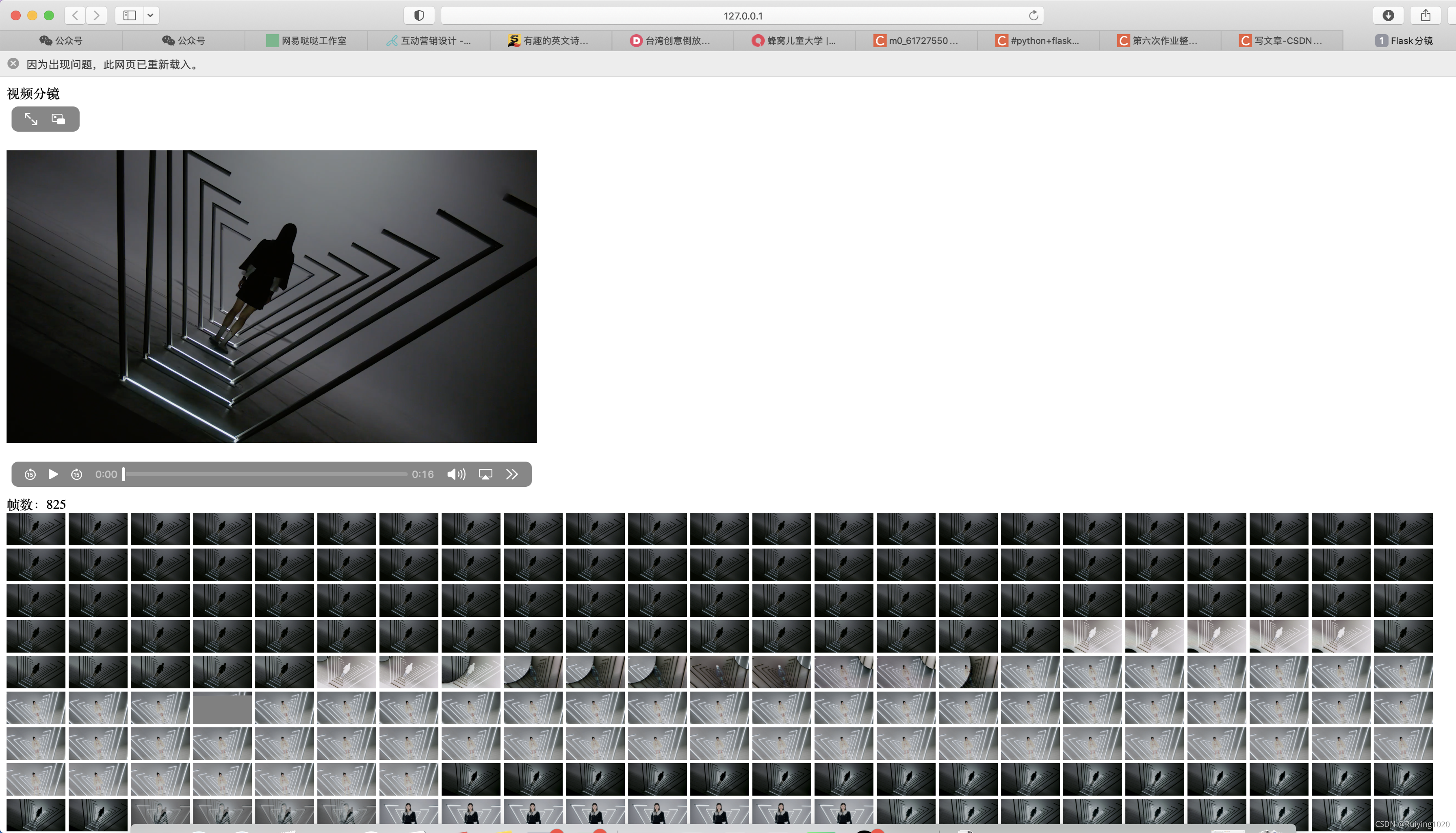
?四、hash均值分镜
1. 新建hash.py
import cv2
import numpy as np
import matplotlib.pyplot as plt
import os
print(os.getcwd())
# 均值哈希算法
def aHash(img):
# 缩放为8*8
# plt.imshow(img)
# plt.axis('off') #去掉坐标轴
# plt.show()
img = cv2.resize(img, (8, 8))
# plt.imshow(img)
# plt.axis('off') #去掉坐标轴
# plt.show()
# 转换为灰度图
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# s为像素和初值为0,hash_str为hash值初值为''
s = 0
hash_str = ''
# 遍历累加求像素和
for i in range(8):
for j in range(8):
s = s + gray[i, j]
# 求平均灰度
avg = s / 64
# 灰度大于平均值为1相反为0生成图片的hash值
for i in range(8):
for j in range(8):
if gray[i, j] > avg:
hash_str = hash_str + '1'
else:
hash_str = hash_str + '0'
return hash_str
# Hash值对比
def cmpHash(hash1, hash2):
n = 0
print(hash1)
print(hash2)
# hash长度不同则返回-1代表传参出错
if len(hash1)!=len(hash2):
return -1
# 遍历判断
for i in range(len(hash1)):
# 不相等则n计数+1,n最终为相似度
if hash1[i] != hash2[i]:
n = n + 1
return n
# img1 = cv2.imread('./pic/image58.jpg') # 11--- 16 ----13 ---- 0.43
# img2 = cv2.imread('./pic/image59.jpg')
#
#
# hash1 = aHash(img1)
# hash2 = aHash(img2)
# n = cmpHash(hash1, hash2)
# print('均值哈希算法相似度:', n)
def genFrame():
v_path='static/vm1.mp4'
image_save='static/hash'
if not(os.path.exists(image_save)):
os.mkdir(image_save)
cap=cv2.VideoCapture(v_path)
fc=cap.get(cv2.CAP_PROP_FRAME_COUNT)
_, img1 = cap.read()
cv2.imwrite('static/hash/image{}.jpg'.format(0), img1)
for i in range(int(fc)-1):
_,img2=cap.read()
hash1 = aHash(img1)
hash2 = aHash(img2)
n = cmpHash(hash1, hash2)
if n>35:
cv2.imwrite('static/hash/image{}.jpg'.format(i),img2)
img1=img2
genFrame()
其实就是从jupyter复制哈希分帧代码过来,但有几个需要注意的点:
1. 所有print需要注释,因为pycharm不能直接读取出来
2. n的大小需要在jupyter里&实际操作中进行测试
例如:我的n最终取值为大于35
第一步:看两个相似照片的哈希数值
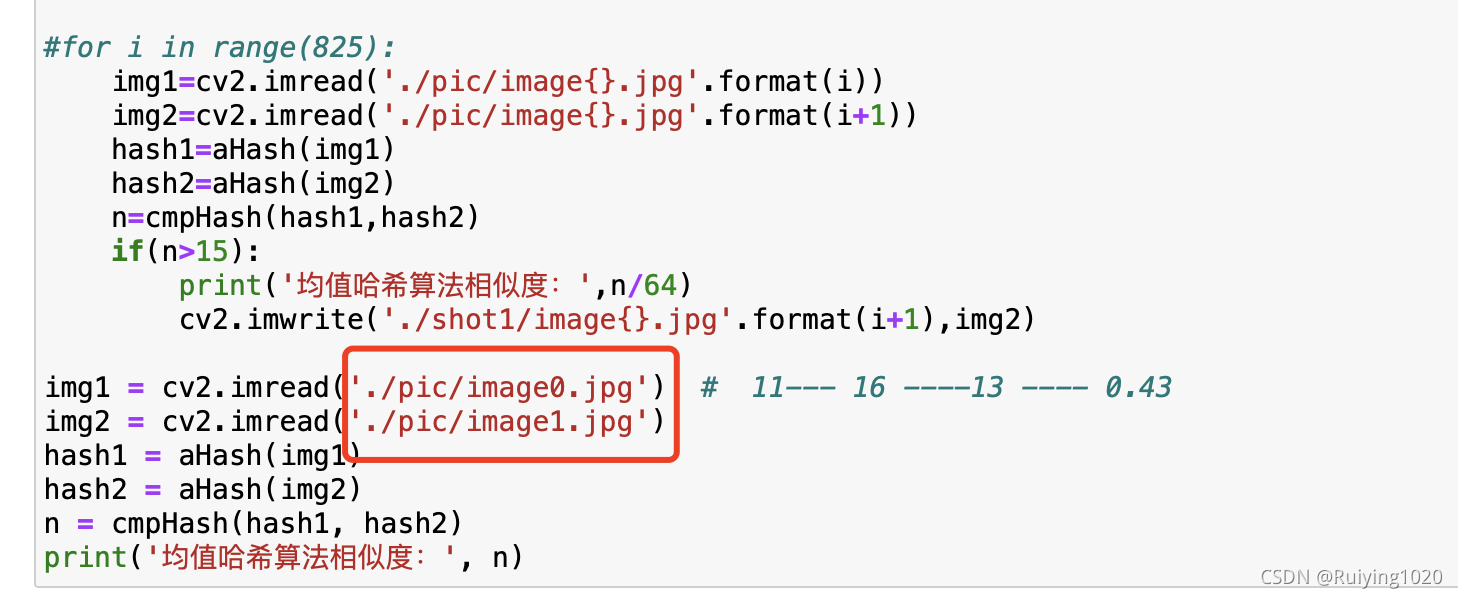
?
第二步:看两个差别巨大的图片的哈希值
?
?
因此:将n的取值放在2和63之间,再一个一个尝试
最终得n>35
调用genFrame()进行分帧 得出如下结果:
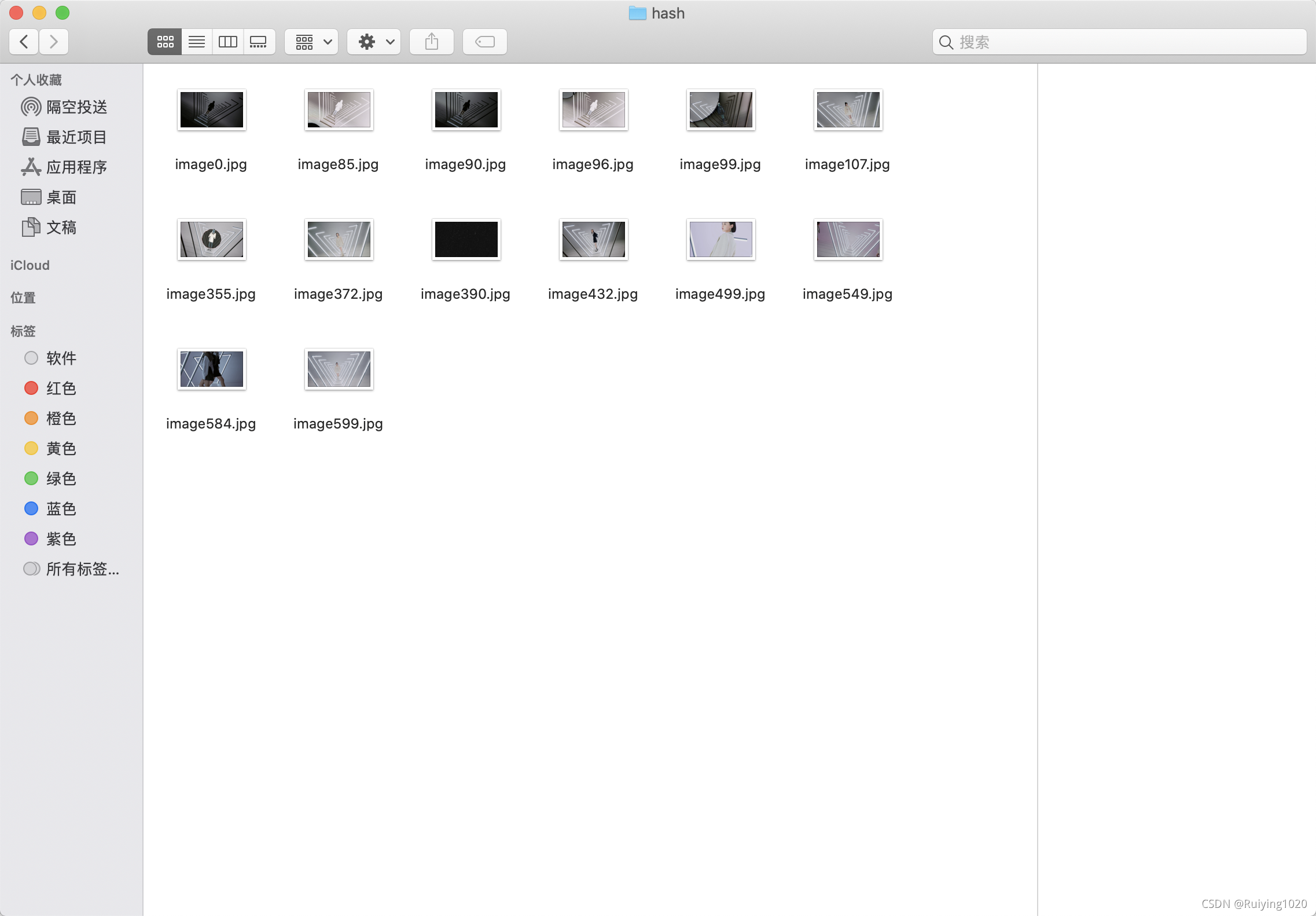
?2. 新建hash.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
</head>
<body>
视频分镜
<br>
<video width="640" height="480" controls autoplay>
<source src="static/vm1.mp4" type="video/mp4">
<object data="static/vm1.mp4" width="640" height="480">
<embed width="640" height="480" src="static/vm1.mp4">
</object>
</video>
<br>
{{imgcount}}<br>
{% for f in filename %}
<img height="40" src="static/hash/{{f}}">
{% endfor %}
</body>
</html>
注意!要单独建hash.html!因为最后是单独的网页 并不是index里能显示的
3. 修改main.py
@app.route('/hash')
def hash():
path='static/hash'
filename=os.listdir(path)
print(type(filename))
print(filename)
imgcount=len(filename)
return render_template('hash.html',imgcount=imgcount,filename=filename)
在main.py里增加hash的命令即可,其他不需要变化
4. 运行main.py
这时你可能还只能看到之前的
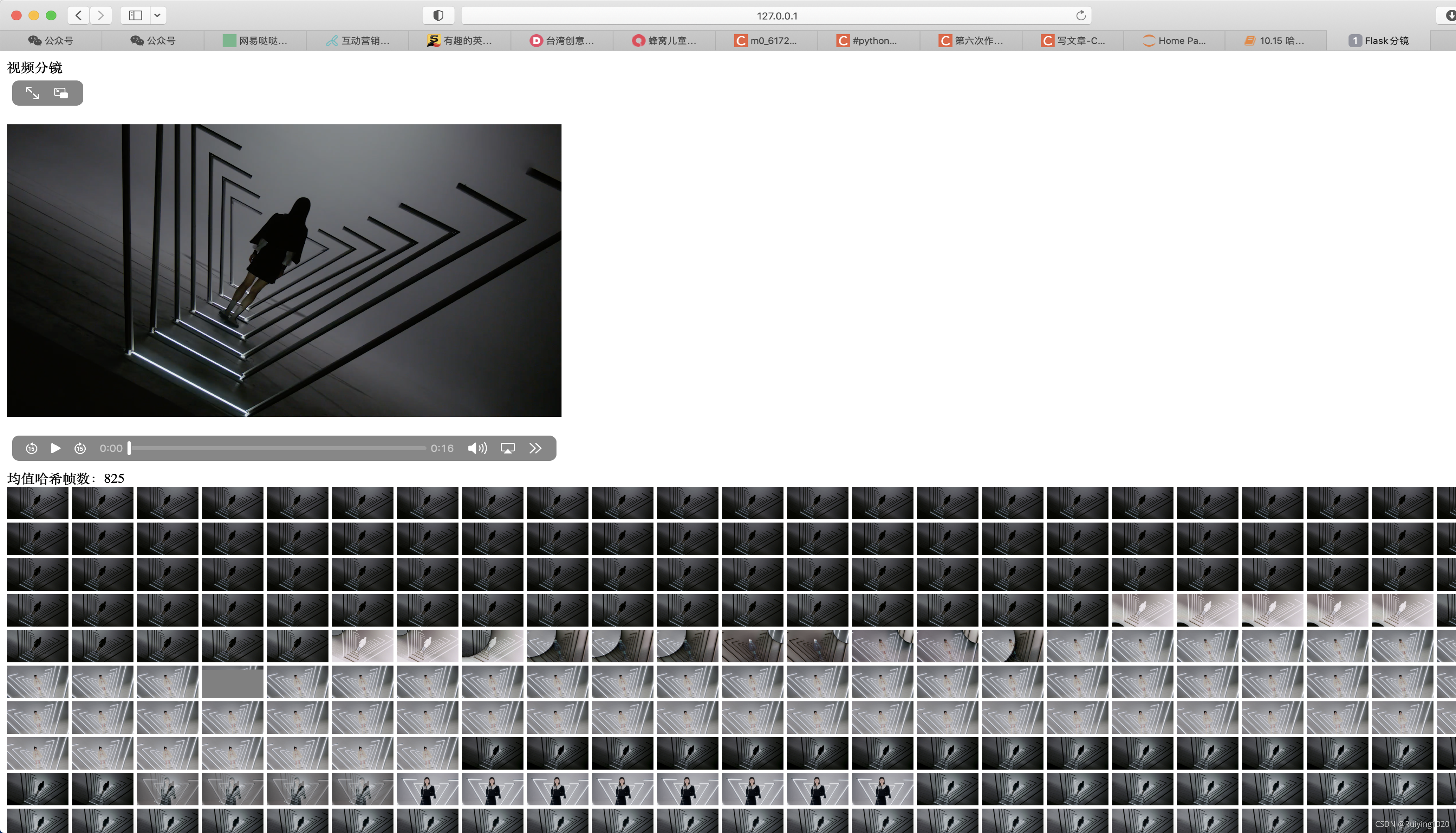
不用慌张,需要修改上面的代码
?
?
?
|