一、python 面向对象编程?
1. 目录
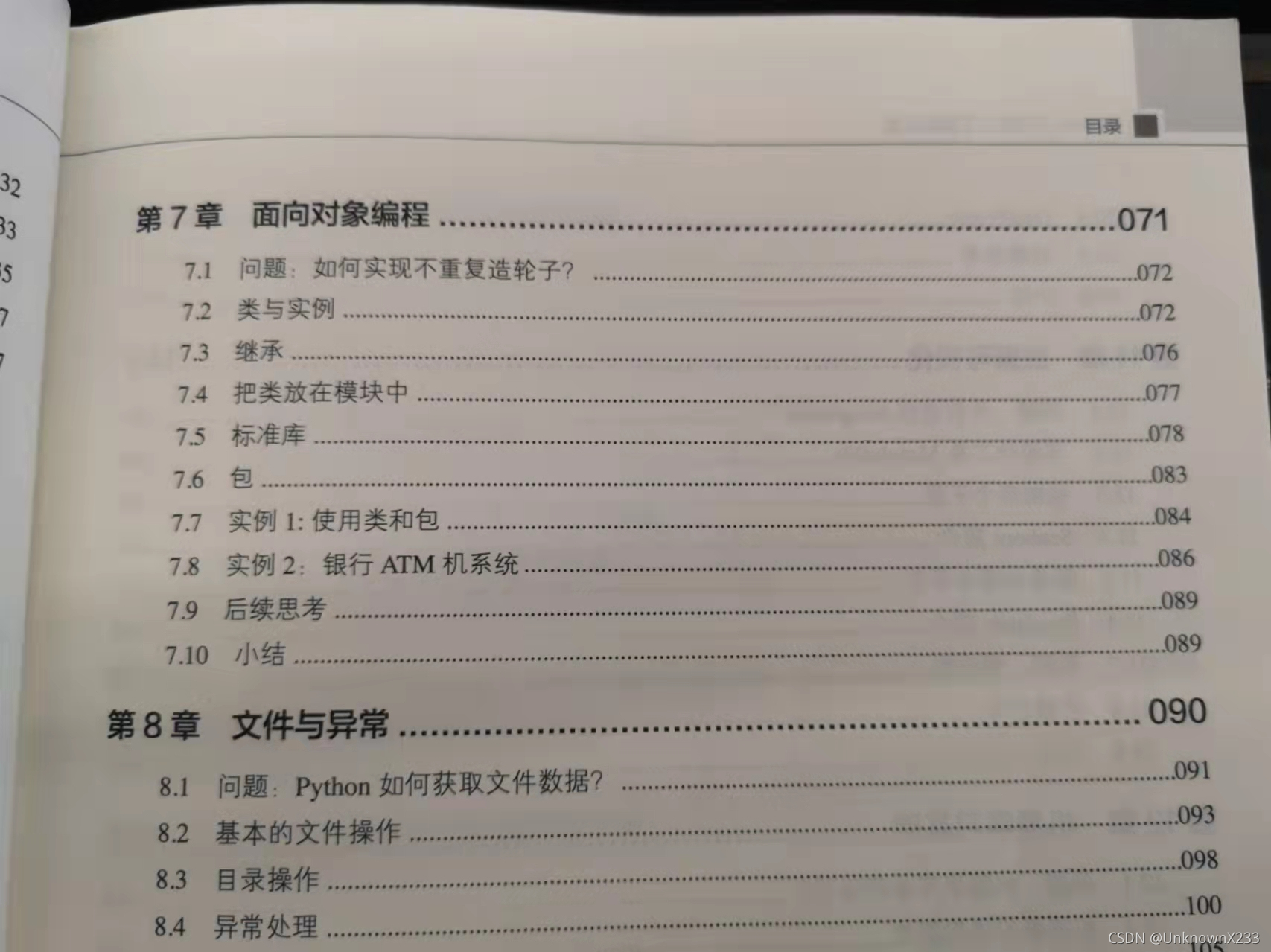
?2. 类
class Person:
def __init__(self,name,age):
self.name = name
self.age = age
def display(self):
print("person(姓名:{},年龄:{})".format(self.name,self.age))
p1=Person("李白",28)
p1.name
p1.display()
p2=Person("张三",30)
print(p2.name)
print(p2.age)
p2.display()
'''
In [5]: p1.name
Out[5]: '李白'
'''
'''
person(姓名:李白,年龄:28)
张三
30
person(姓名:张三,年龄:30)
'''
?3. 类属性
class Person:
pernum = 0 #类属性
def __init__(self,name,age):
self.name = name
self.age = age
Person.pernum+=1
def display(self):
print("person(姓名:{},年龄:{})".format(self.name,self.age))
def display_pernum(self):
print(Person.pernum)
p3=Person("zs",32)
print(p3.pernum)
p3.display_pernum()
p4=Person("qwe", 42)
print(Person.pernum)
print(p4.pernum)
p4.display_pernum()
'''
1
1
2
2
2
'''
4. 继承
class Person:
pernum = 0 #类属性
def __init__(self,name,age):
self.name = name
self.age = age
Person.pernum+=1
def display(self):
print("person(姓名:{},年龄:{})".format(self.name,self.age))
def display_pernum(self):
print(Person.pernum)
class Student(Person): #继承
def __init__(self,name,age,university):
super(Student,self).__init__(name,age)
self.university = university
def display(self):
print("Student(姓名:{},年龄:{},大学:{})".format(self.name,self.age,self.university))
s1=Student("jd", 23, "bjdx")
print(s1.university)
s1.display() #重写
s1.display_pernum() #继承
5. 标准库
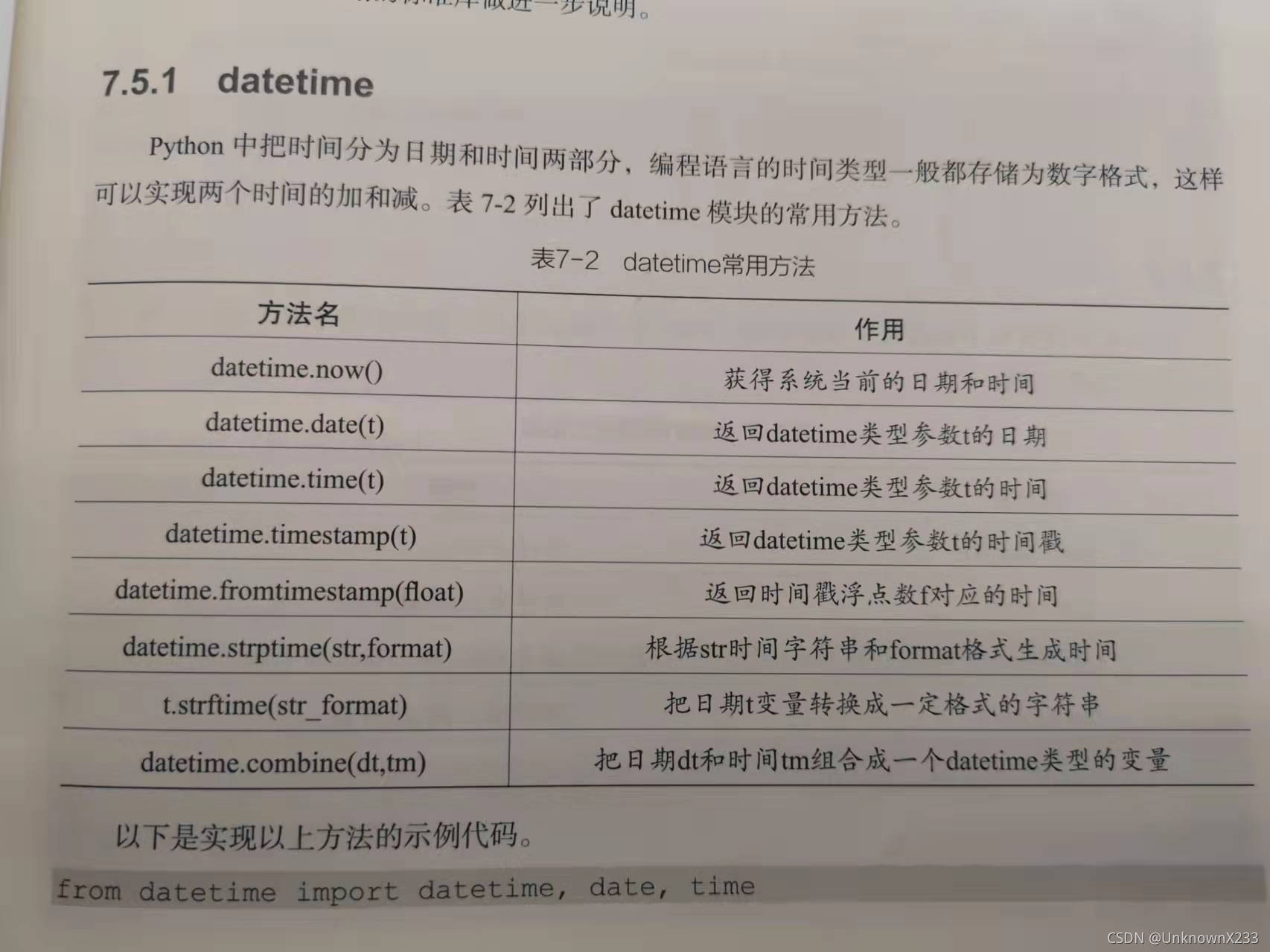
?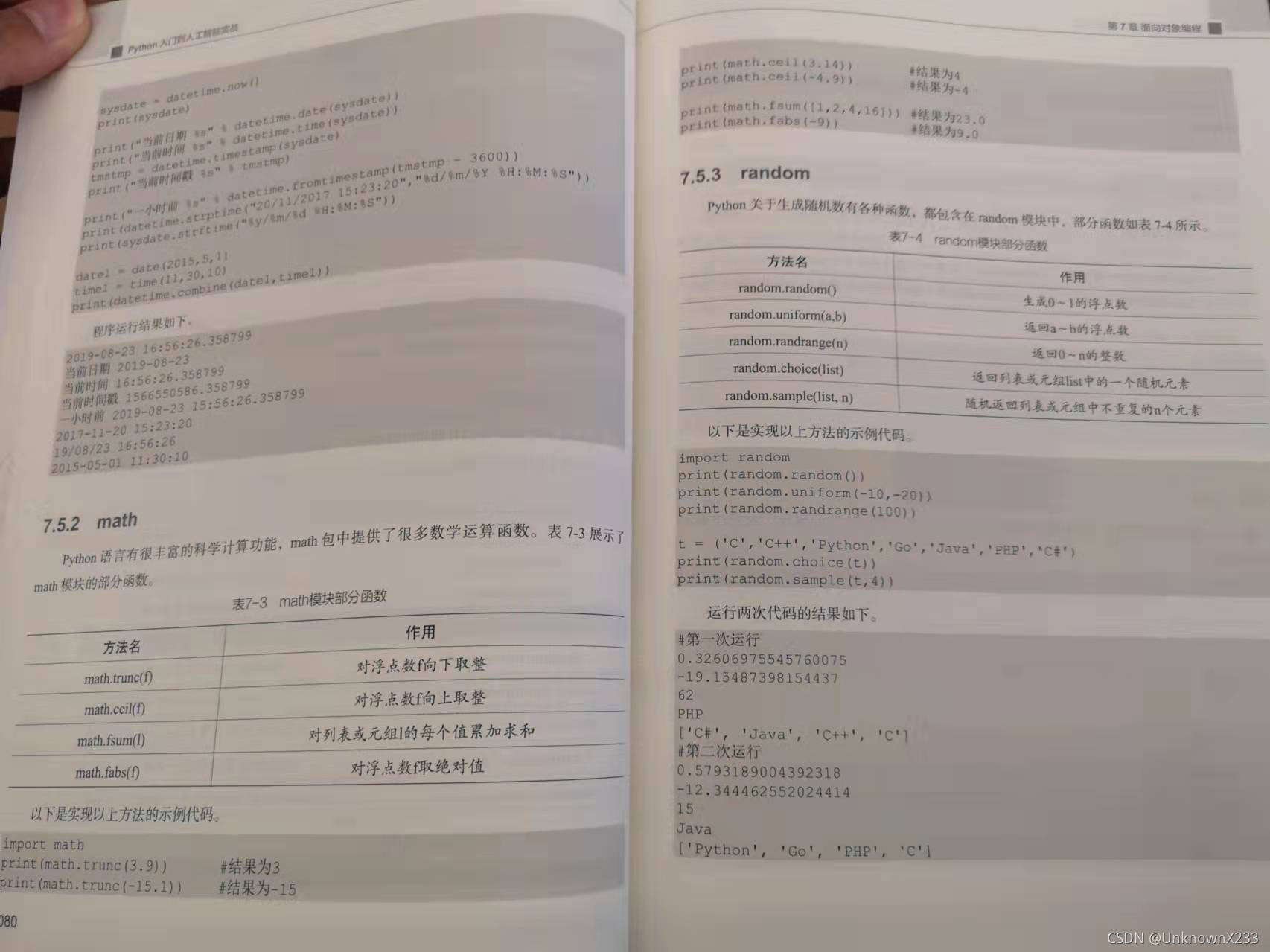
?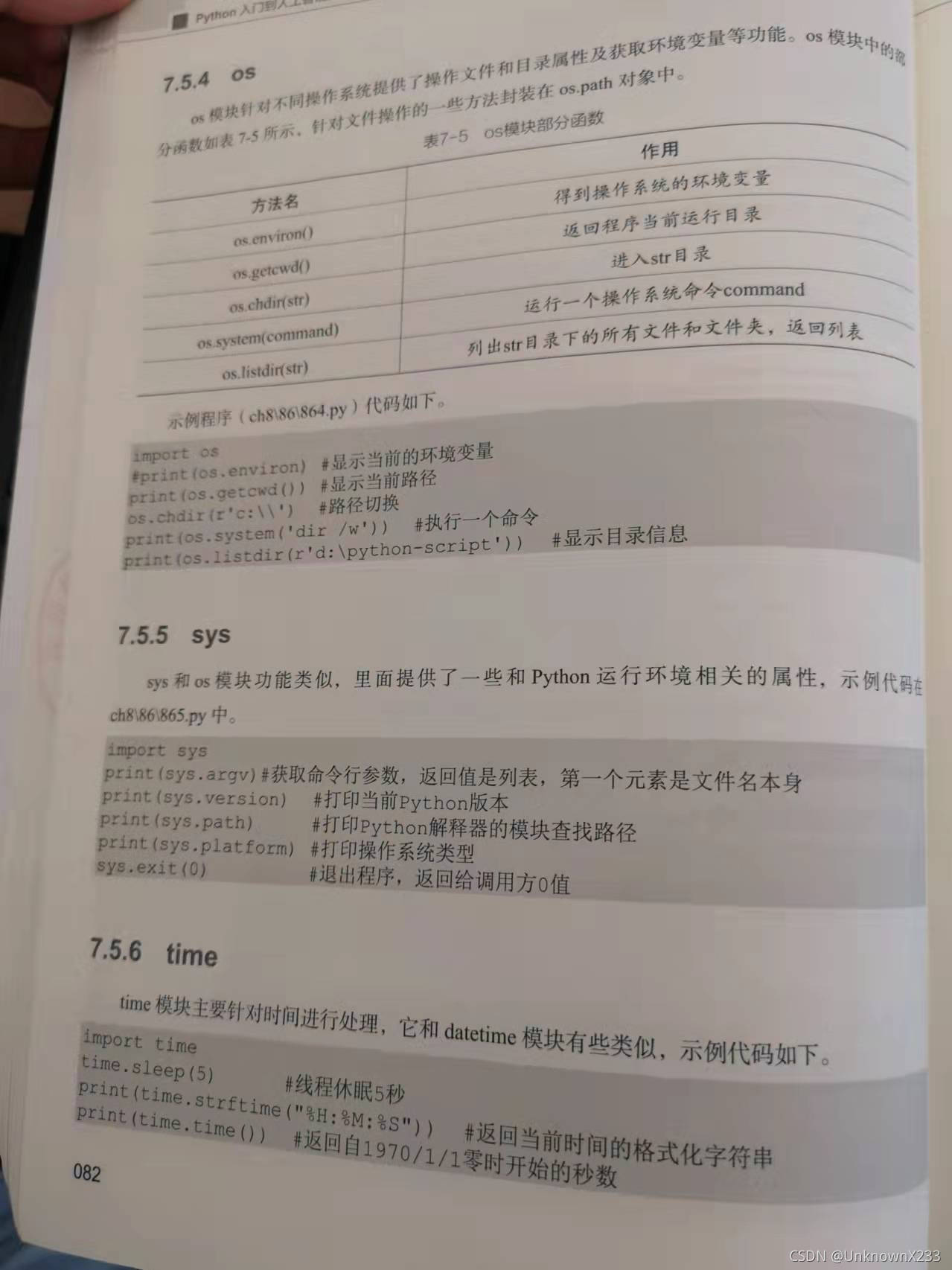
二、c语言学习
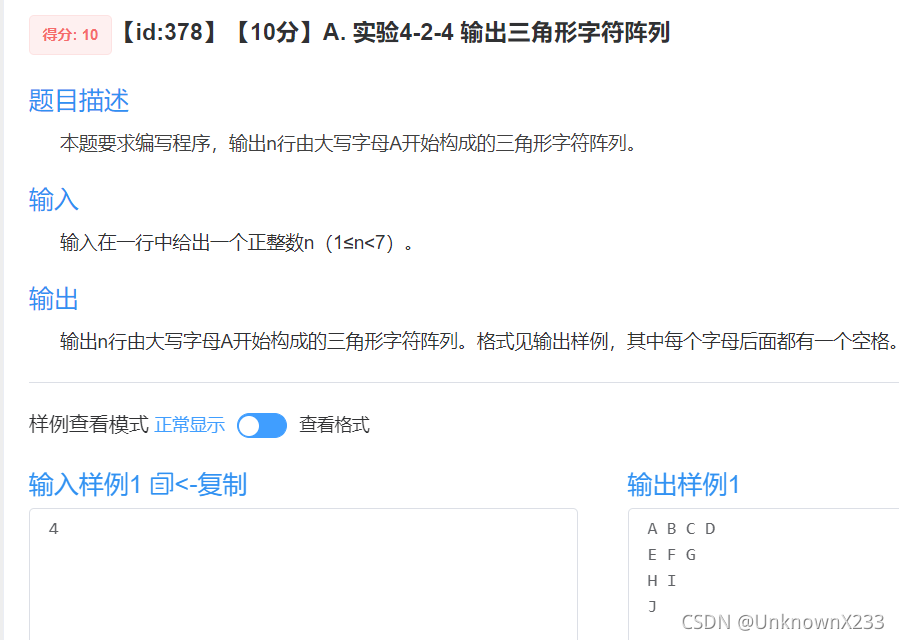
?
#include <stdio.h>
int main(){
int n;
char a='A';
scanf("%d",&n);
for(int i=0;i<=n;i++){
for(int j=n-i;j>0;j--){
printf("%c ",a++);
}
printf("\n");
}
return 0;
}
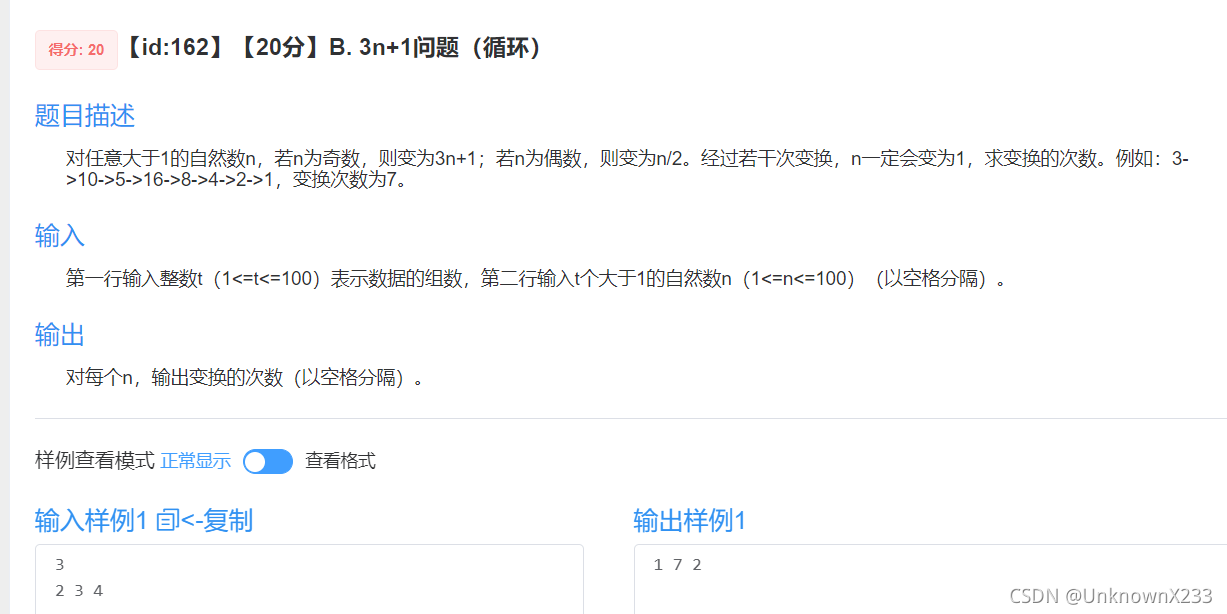
?
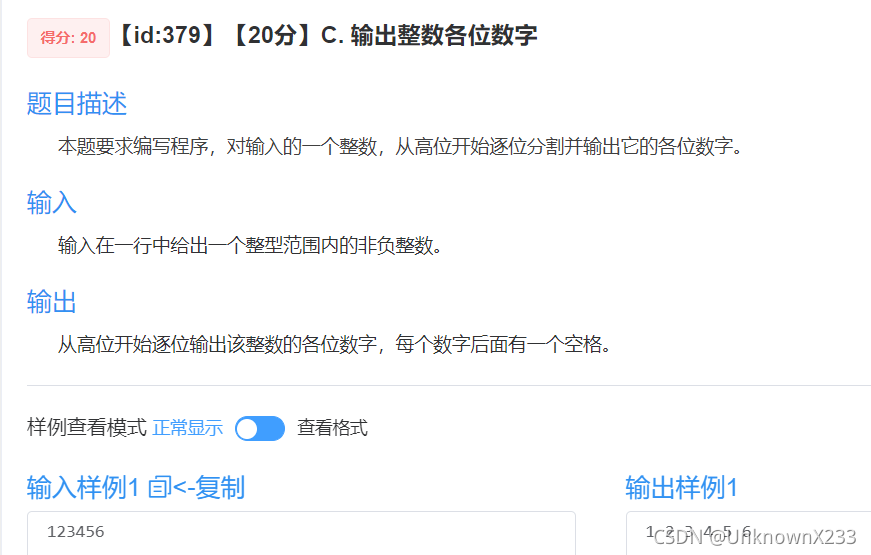
#include <stdio.h>
int main(){
int n,a[100],i=0;
scanf("%d",&n);
do{
a[i]=n%10;
n=n/10;
i++;
}while(n!=0);
for(int j=i-1;j>=0;j--){
printf("%d ",a[j]);
}
return 0;
}
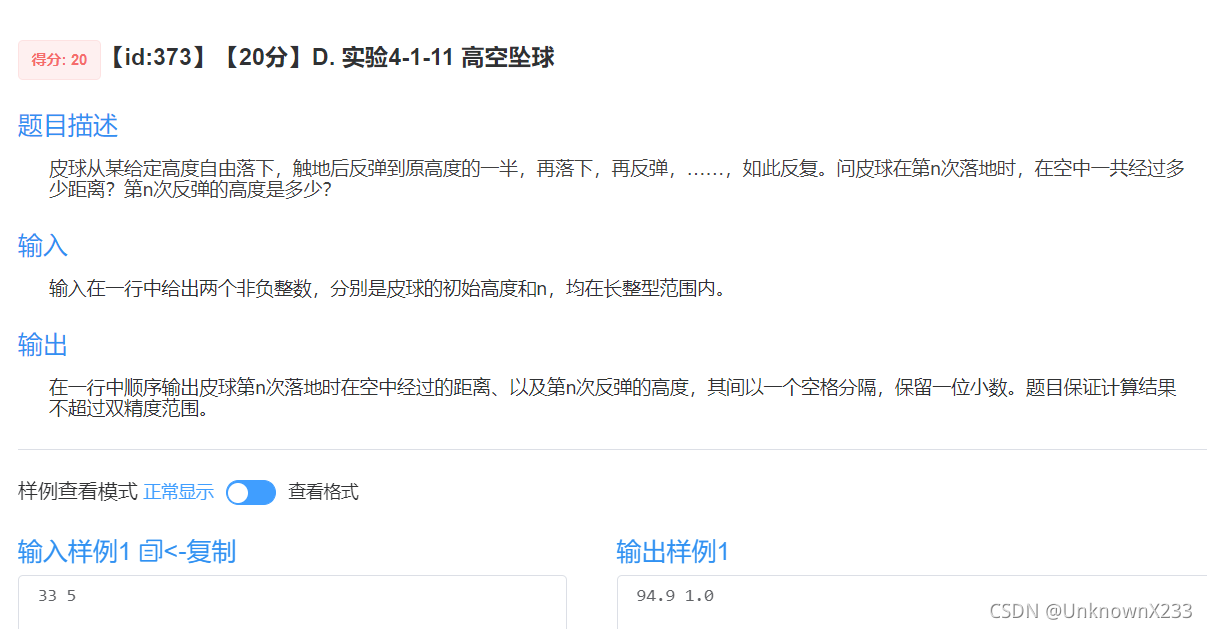
?
#include <stdio.h>
int main(){
long int a,n;
scanf("%ld %ld",&a,&n);
double s=a,h=a;
for(int i=1;i<=n;i++){
s+=h;
h=h/2;
}
s-=2*h;
if(n==0){
printf("0.0 0.0");
}else
printf("%.1f %.1f",s,h);
return 0;
}
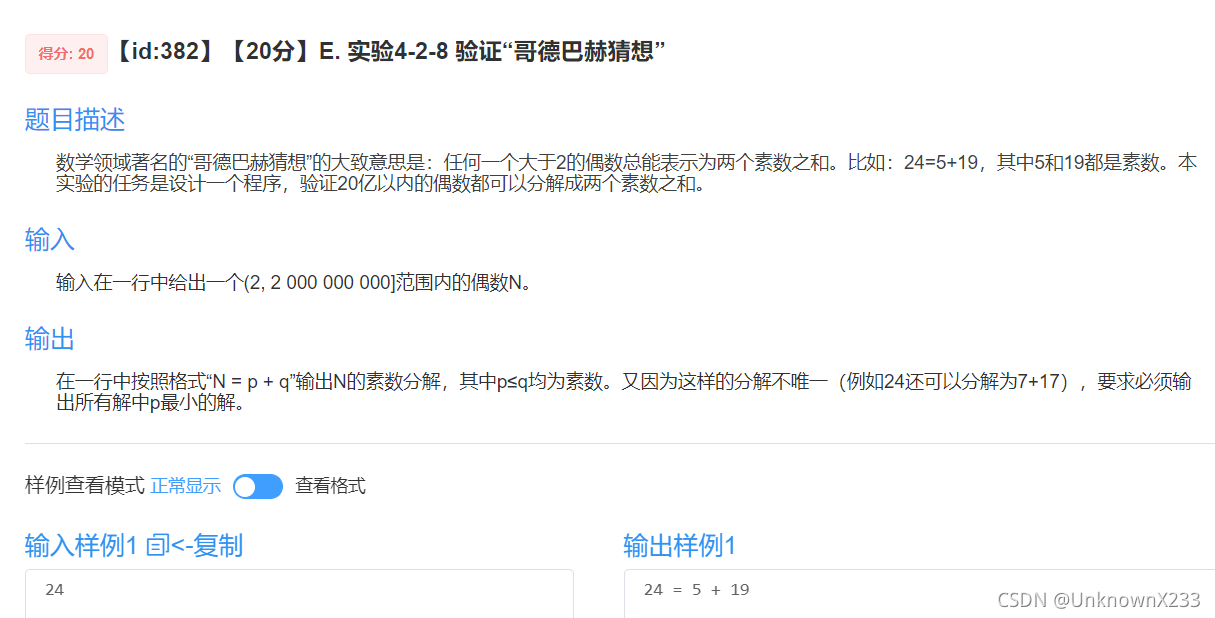
#include <stdio.h>
#include <math.h>
int ss(int x){
if(x==1) return 0;
if(x==2) return 1;
for(int a=2;a<=sqrt(x)+1;a++){
if(x%a==0) return 0;
}
return 1;
}
int main(){
int n;
scanf("%d",&n);
for(int i=1;i<=n;i++){
if(ss(i)&&ss(n-i)) {
printf("%d = %d + %d",n,i,n-i);
break;
}
}
return 0;
}
?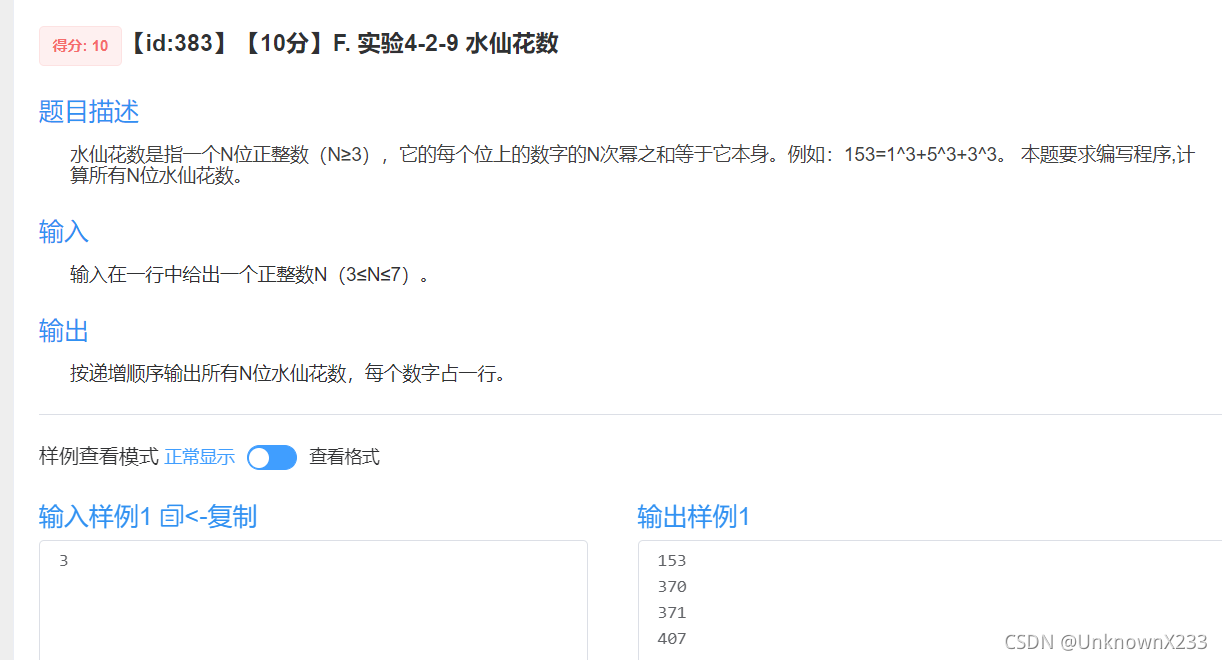
#include <stdio.h>
#include <math.h>
int main(){
int n,a[8];
scanf("%d",&n);
for(int i=pow(10,n-1);i<pow(10,n);i++){
int j=0,sum=0,t=i;
do{
a[j]=t%10;
t=t/10;
j++;
}while(t!=0);
for(int k=0;k<=j-1;k++){
sum+=pow(a[k],n);
}
if(sum==i)
printf("%d\n",i);
}
return 0;
}
?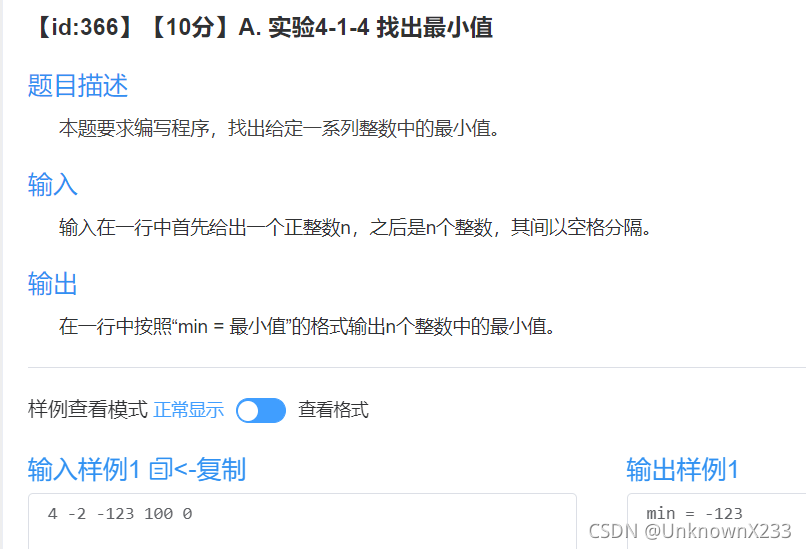
?
#include <stdio.h>
int main(){
int n,min,x;
scanf("%d",&n);
scanf("%d",&x);
min=x;
for(int i=0;i<n-1;i++){
scanf("%d",&x);
if(x<min)
min=x;
}
printf("min = %d",min);
}
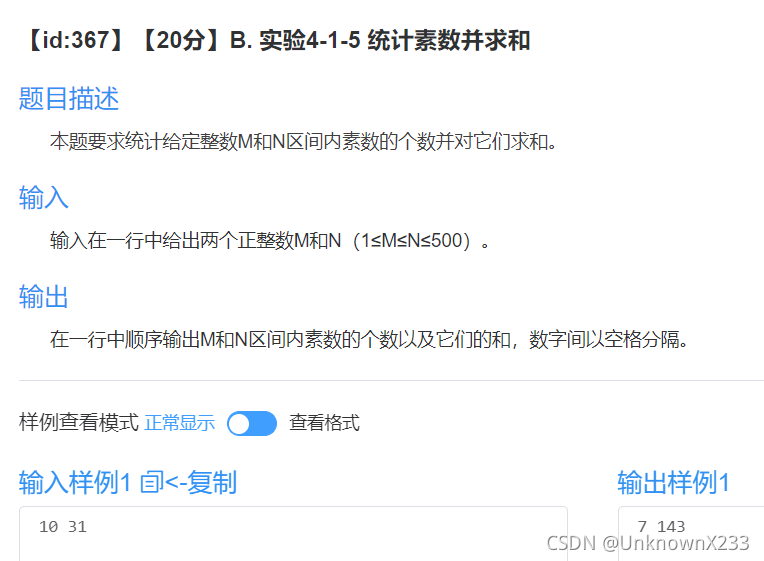
#include <stdio.h>
#include <math.h>
int ss(int a){
if(a==1)
return 0;
else if(a==2)
return 1;
for(int i=2;i<sqrt(a)+1;i++){
if(a%i==0)
return 0;
}
return 1;
}
int main(){
int n,m,x=0,y=0;
scanf("%d %d",&m,&n);
for(int j=m;j<=n;j++){
if(ss(j)){
x++;
y+=j;
}
}
printf("%d %d",x,y);
}
?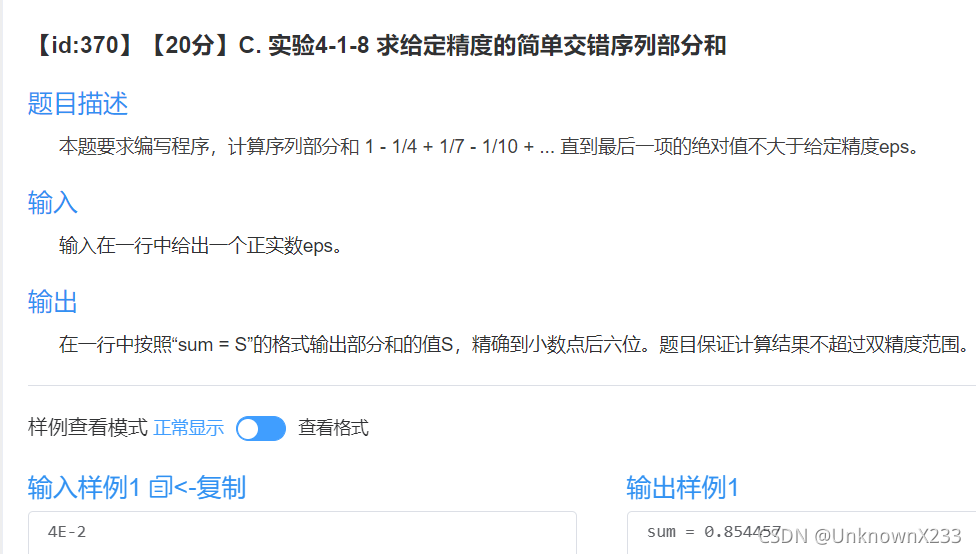
?
#include <stdio.h>
#include <math.h>
int main(){
double n,sum=0,temp;
int flag=1,fm=1;
scanf("%lf",&n);
do{
temp=flag*(1/(double)fm);
sum+=temp;
flag=-flag;
fm+=3;
}while(fabs(temp)>n);
printf("sum = %.6f",sum);
}
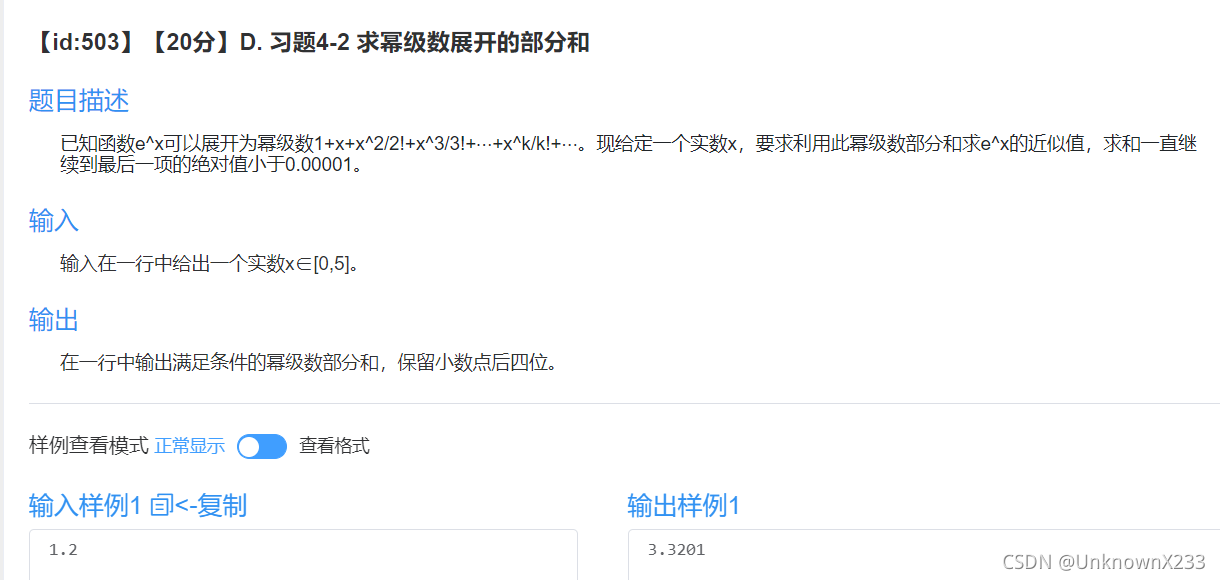
?
#include <stdio.h>
#include <math.h>
double jc(int a){
double s = 1;
for(int i=1;i<=a;i++){
s = s * i;
}
return s;
}
int main(){
double n,sum=1,last=1;
scanf("%lf",&n);
for(int i=1;;i++){
last=pow(n,i)/jc(i);
sum+=last;
if(fabs(last)<0.00001) break;
}
printf("%.4f",sum);
}
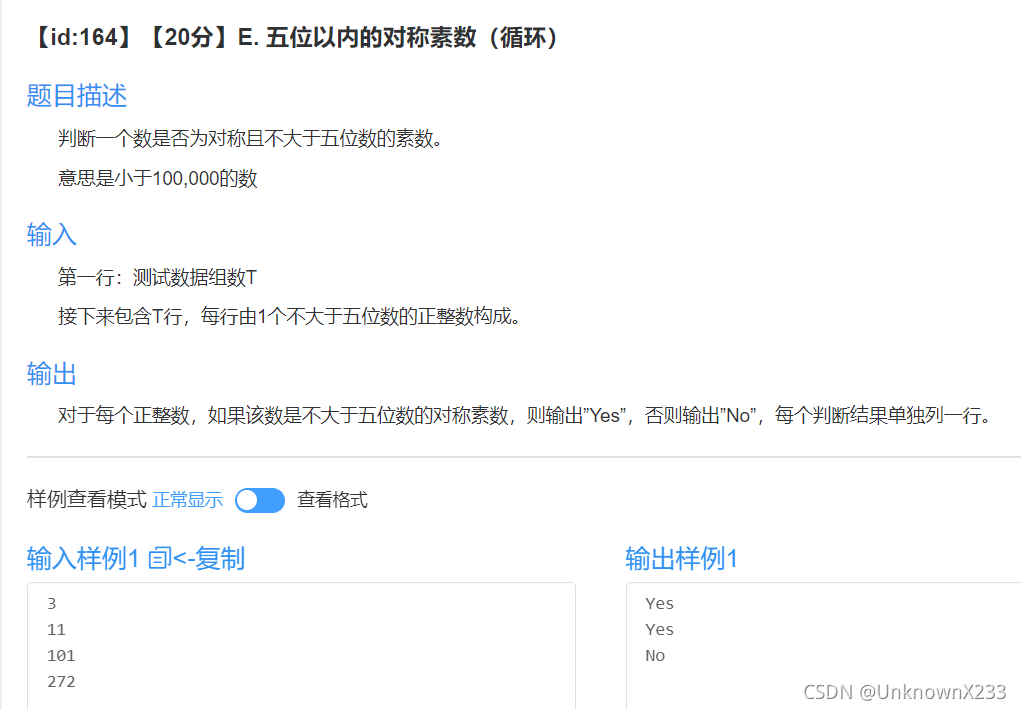
#include <stdio.h>
#include <math.h>
int ss(int a){
if(a==1)
return 0;
else if(a==2)
return 1;
for(int i=2;i<sqrt(a)+1;i++){
if(a%i==0)
return 0;
}
return 1;
}
int dc(int b){
int c[10],j;
for(j=0;;j++){
c[j]=b%10;
b=b/10;
if(b==0) break;
}
for(int i=0;i<=(j/2+1);i++){
if(c[i]!=c[j-i])
return 0;
}
return 1;
}
int main(){
int n,x;
scanf("%d",&n);
for(int i=1;i<=n;i++){
scanf("%d",&x);
if(ss(x)&&dc(x))
printf("Yes\n");
else
printf("No\n");
}
}
?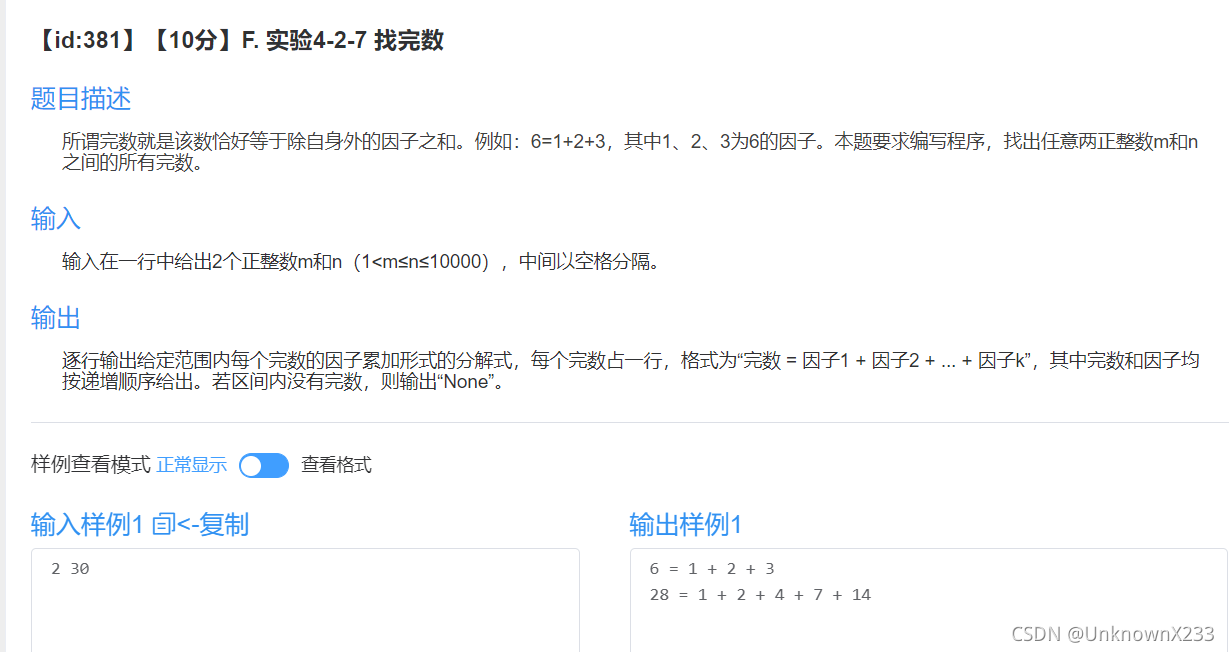
#include <stdio.h>
#include <math.h>
int main(){
int m,n,flag=1;
scanf("%d %d",&m,&n);
for(int i=m;i<=n;i++){
int sum=0,k=0;
int a[100];
for(int j=1;j<i;j++){
if(i%j==0){
sum+=j;
a[k]=j;
k++;
}
}
if(sum==i){
printf("%d =",i);
for(int l=0;l<k-1;l++){
printf(" %d +",a[l]);
}
printf(" %d\n",a[k-1]);
flag=0;
}
}
if(flag)
printf("None");
}
?
|